Real Time Chat App Using HTML, CSS, JavaScript & React With Source Code
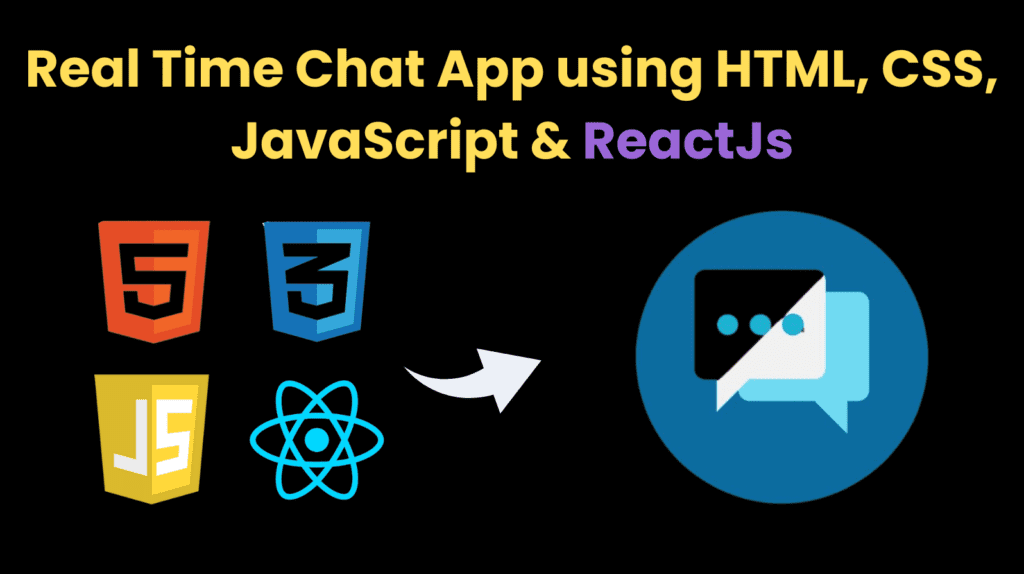
Introduction :
The real-time chat application built with React.js allows users to join chat rooms and communicate in real-time. It leverages socket.io
for real-time bidirectional communication, ensuring messages are instantly delivered to all participants in a chat room. The application is structured with several React components, each serving a specific purpose in the chat interface.
Explanation :
Project Structure
App.js
Purpose: The main entry point for the application, responsible for setting up routing.
Structure and Logic:
- Router Setup: Uses
BrowserRouter
fromreact-router-dom
to enable routing. - Route Definitions: Defines routes for the join page (/) and the chat page (/chat).
/
: Renders the Join component./chat
: Renders the Chat component.
Index.js
Purpose: The entry point of the application, responsible for rendering the main App component into the DOM.
Structure and Logic:
- Render Method: Uses
ReactDOM.render
to mount the App component into the root DOM node.
Chat.js
Purpose: Manages the core chat functionality, including user connections, message handling, and user interactions within the chat room.
State Management:
name
,room
: Store the user’s name and the chat room name.users
: Track users currently in the chat room.message
,messages
: Handle the current message being typed and the list of all messages in the chat.
Effects and Logic:
- Initial Connection: Parses query parameters to extract the user’s name and room, connects to the socket server, and joins the room.
- Message Handling: Listens for new messages and updates the state accordingly, ensuring the chat interface displays the latest messages.
- Room Data: Listens for updates on the room’s user list, ensuring the user list is always up-to-date.
Functions:
sendMessage
: Emits a socket event to send a message and clears the input field after sending.
InfoBar.js
Purpose: Displays the current chat room name and provides a button to leave the chat.
Structure and Logic:
- Room Name: Displays the name of the chat room.
- Online Icon: Indicates that the user is online.
- Close Button: Provides a link to return to the join page, effectively leaving the chat.
Input.js
Purpose: Provides an input field for typing messages and a send button to submit them.
Structure and Logic:
- Input Field: Captures the user’s message input and updates the state.
- Send Button: Triggers the function to send the message when clicked.
- Keyboard Interaction: Allows users to send messages by pressing the Enter key.
Join.js
Purpose: Allows users to join a chat room by entering their name and the room name.
State Management:
name
,room
: Store the user’s inputs for their name and the room name.
Structure and Logic:
- Input Fields: Capture the user’s name and room inputs, updating the state accordingly.
- Link: Navigates to the chat page with the provided name and room as query parameters, ensuring valid inputs before allowing navigation.
Messages.js
Purpose: Displays a list of messages in the chat.
Structure and Logic:
- Message List: Maps through the messages state to render each message, ensuring the chat displays all messages in order.
- Scroll Management: Uses a utility to ensure the chat window scrolls to the bottom, keeping the latest message in view.
TextContainer.js
Purpose: Displays the list of users currently in the chat room.
Structure and Logic:
- User List: Shows a list of active users in the chat room, each with an online icon to indicate their status.
- Chat Information: Provides additional details about the chat application, encouraging user engagement.
JavaScript Logic
App.js
The App component sets up the foundational routing for the application using React Router. It defines two primary routes:
- The root path (
/
) which renders the Join component, allowing users to input their name and room. - The
/chat
path which renders the Chat component, where users participate in real-time messaging.
Index.js
Index.js is the entry point of the React application. It utilizes ReactDOM.render
to mount the App component into the root DOM node identified by the id
“root”. This file ensures that the App component is properly rendered and the routing is enabled.
Chat.js
The Chat component is the heart of the chat functionality:
- State Variables:
name
androom
store the current user’s name and the chat room they have joined.users
holds the list of users currently in the room.message
andmessages
manage the current input message and the array of all messages.
- Effects:
- The first
useEffect
handles the initial connection. It parses the query string to extract the user’s name and room, then connects to the socket server and emits a ‘join’ event. - The second
useEffect
listens for incoming messages and updates the messages state, ensuring new messages are displayed. It also listens for updates to the room’s user list.
- The first
- sendMessage Function: This function sends the current message to the socket server and clears the input field.
InfoBar.js
The InfoBar component displays the name of the chat room and provides an interface element to leave the chat:
- Room Name: Displays the name of the current chat room.
- Online Icon: Indicates the user is online.
- Close Button: Provides a link to navigate back to the join page, effectively leaving the chat room.
Input.js
The Input component allows users to type and send messages:
- Input Field: Captures user input and updates the message state as the user types.
- Send Button: Triggers the sendMessage function when clicked.
- Keyboard Interaction: Allows users to send a message by pressing the Enter key, providing a seamless messaging experience.
Join.js
The Join component is the entry interface for the chat application:
- State Variables:
name
stores the user’s name input.room
stores the room name input.
- Input Fields: Capture the user’s name and room, updating the state accordingly.
- Link: Uses
react-router-dom
to navigate to the chat page with the name and room as query parameters, ensuring valid inputs before allowing navigation.
Messages.js
The Messages component is responsible for rendering the list of messages:
- Message List: Maps through the messages state to render each message using the Message component.
- Scroll Management: Ensures the chat window scrolls to the bottom, keeping the latest message in view.
Get Discount on Top Educational Courses
Source Code :
Output :
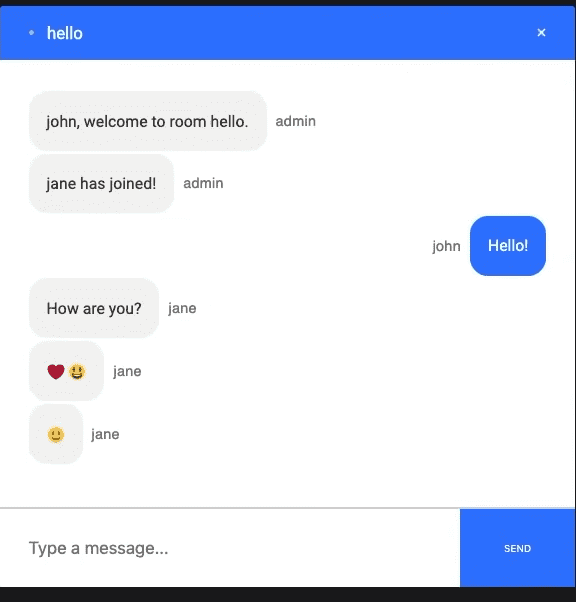
Find More Projects
how to create a video background with html CSS JavaScript Introduction Hello friends, you all are welcome to today’s new blog post. …
Auto Text Effect Animation Using Html CSS & JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …