Spotify Clone Using HTML, CSS and JS
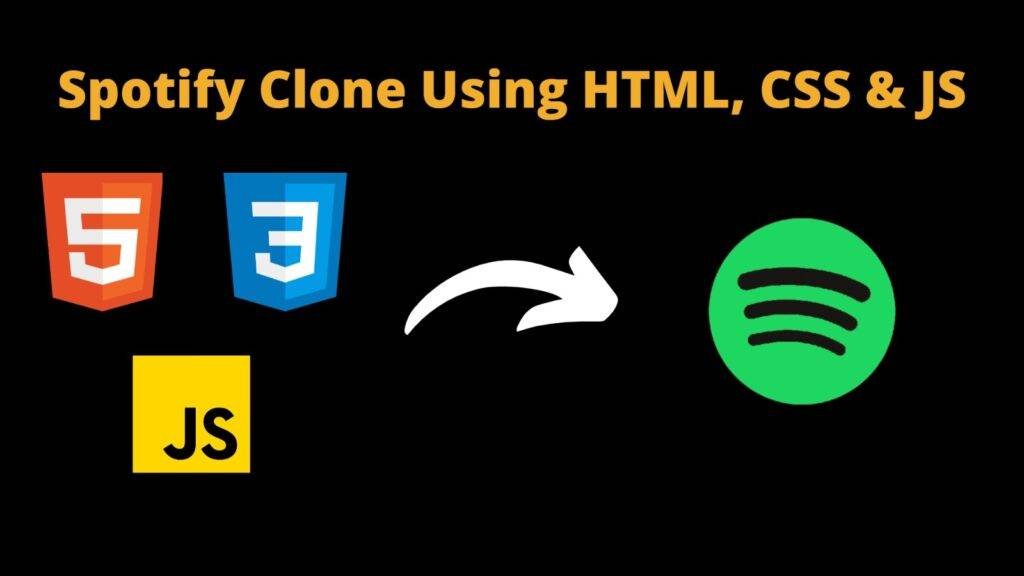
Introduction
In this project, we have built a dynamic Spotify-inspired music streaming platform using the trio of HTML, CSS, and JavaScript. Our goal was to create a user-friendly interface that seamlessly navigates through artists, albums, and playlists, providing an immersive music listening experience.
With HTML, we’ve structured the foundation of our platform, ensuring clarity and ease of use in every interaction. CSS has enhanced the visual appeal, with stylish designs and responsive layouts that adapt flawlessly to various devices.
JavaScript has powered interactivity, enabling dynamic features such as real-time song recommendations and instant playlist updates. Through client-side scripting, we’ve provided users with a seamless and engaging music streaming journey.
Together, these technologies have allowed us to craft a comprehensive music streaming experience that brings the joy of Spotify to life.
“Welcome to our project – where music meets innovation”
Steps to create a Spotify Clone
- Project Setup:Create a new project folder on your computer.Inside the folder, create three files: index.html, style.css, and script.js.
- HTML Structure: Open the index.html file and set up the basic HTML structure. Create sections for header, main content, footer, and any additional sections as needed. Within the main content section, include placeholders for displaying albums, playlists, and player controls.
- Styling with CSS:Open the styles.css file and begin styling the layout and components.Define the overall look and feel of your Spotify Clone, including colors, typography, and spacing.Use CSS to create responsive designs that adapt to different screen sizes.
- Adding Content:Populate the HTML file with placeholder content, such as sample albums, artist names, and playlist titles.Use dummy text or images to represent album covers and artist profiles for now.
- Implementing Interactive Features with JavaScript:Open the script.js file and start writing JavaScript code to add interactivity to your Spotify Clone.
- Testing and Debugging:Regularly test your project in different web browsers to ensure cross-browser compatibility. Debug any issues or errors that arise during testing. Use browser developer tools to inspect and troubleshoot CSS styles and JavaScript code.
Explanation
HTML
index.html
Our Spotify Clone project’s HTML structure forms the backbone of our music streaming platform. Let’s dissect the essentials:
The document type declaration (<!DOCTYPE html>) ensures compliance with HTML5 standards, while the HTML element (<html lang=”en”>) sets the document’s language to English.
Within the head section (<head>), metadata and external resource links are crucial. These include character encoding (<meta charset=”UTF-8″>) and document title (<title>Spotify Clone</title>).
External resource links (<link>) bring additional functionality and styling. We integrate Font Awesome, Bootstrap libraries, and a custom stylesheet (style.css).
The body section (<body>) houses visible content, with the application container (<div id=”root”></div>) serving as the canvas for our Spotify Clone. This container is targeted for dynamic content rendering via JavaScript.
JavaScript inclusions (<script>) link React libraries (react.production.min.js and react-dom.production.min.js) for interactive UI development. Additionally, a custom JavaScript file (script.js) tailors specific functionalities.
CSS Styling
- Body Styles:
In our Spotify Clone project, the body styles lay the groundwork for visual appeal and readability. We utilize a standard font stack including “Poppins” and fallbacks to sans-serif, ensuring consistent legibility across various platforms. The chosen background color of #0d0d0d sets a dark tone, enhancing the platform’s modern aesthetic. - Menu Bar Styling:
The .menu-bar class defines the appearance of our menu bar. With a fixed height and width of 14em, it remains visible and accessible at all times. Positioned fixed at the top, its black background color provides a sleek contrast against the overall dark theme. Icons such as the podcast icon and heart icon are styled with specific colors for visual differentiation. - Main Content Header Styles:
Our main content header design exudes professionalism and clarity. Positioned fixed at the top, it features a vibrant green background color (#1DB954) to complement Spotify’s brand identity. The hamburger menu icon offers intuitive navigation options for users on smaller screens. - Main Content Section Styling:
Within the .main-content main section, we define the layout and styling for the primary content area. Utilizing margins and auto margins, we ensure optimal alignment and spacing. The content is positioned to the right with a margin-top of 4.5em, allowing for smooth scrolling and easy access to the header. - Recent Activity Display Styling:
The .recent-activity class governs the appearance of the recent activity section. Displayed in a grid layout, it showcases user interactions in a visually appealing manner. Each activity card is styled with a dark background color (#1a1a1a) and subtle hover effects for enhanced interactivity. - Category Section Styles:
Within the main content, categories are displayed in a visually organized manner. Utilizing grid layout and auto margins, we ensure consistent spacing and alignment across different screen sizes. Each category card features an image, title, and author, providing users with relevant information at a glance. - Responsive Design:
To ensure optimal user experience across devices, our CSS code includes media queries targeting various screen sizes. These queries adjust layout, spacing, and font sizes to maintain readability and functionality on smaller screens while maximizing content visibility.
script.js
JavaScript code defines the structure and functionality of the Spotify clone application using React components. It handles user interactions, such as menu toggling and dropdown menu visibility, and dynamically generates content based on user actions and time of the day.
- App Component:The App component is a class component that serves as the main component of the application.It renders two child components: Menu and MainContent.
- Menu Component:The Menu component is a functional component responsible for rendering the navigation menu of the application. It consists of a menu bar containing links to different sections like Home, Search, and Your Library.Each link is represented by an <a> tag with an associated SVG icon.Additionally, there is a user collection section containing links for creating playlists, accessing liked songs, and viewing episodes.
- MainContent Component:The MainContent component is a functional component responsible for rendering the main content of the application.It consists of two child components: Header and Body.
- Header Component:The Header component is a functional component responsible for rendering the header section of the application.It includes a hamburger menu icon for toggling the menu visibility and a user dropdown menu for accessing user-related options like account settings, profile, and logout.The visibility of the dropdown menu is controlled by state variables (profileVisibility and menuBarVisibility) using React hooks (useState).Event handlers are defined to toggle the visibility of the dropdown menu and the menu bar.
- Body Component:The Body component is a functional component responsible for rendering the main content body of the application.It dynamically generates content based on the time of the day (morning, afternoon, or evening) and displays recent activities, categories, and popular shows.
Source Code
HTML (index.html)
Spotify Clone
Spotify - Web Player
CSS (style.css)
@import url("https://fonts.googleapis.com/css2?family=Poppins&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
background-color: #0d0d0d;
color: #fff;
}
.menu-bar {
height: 100vh;
width: 14em;
position: fixed;
z-index: 1;
top: 0;
background-color: #000;
}
.menu-bar img {
height: 3.75em;
width: 9.3em;
margin: 0.5em 0 0 0.9em;
}
.menu-bar .fa-podcast {
color: green;
}
.menu-bar .fa-heart {
color: #ff3333;
}
.menu-bar nav ul {
padding: 0;
margin: 0;
list-style: none;
}
.menu-bar nav ul li {
margin: 1em 0;
}
.menu-bar nav ul li a {
text-decoration: none;
color: #cccccc;
padding: 0.5em 0.5em;
margin-left: 1.2em;
}
.menu-bar nav ul li a:hover {
color: #fff;
}
.menu-bar nav ul li a svg {
margin: 0 1em 0.3em 0;
}
.menu-bar nav ul li a .fa {
margin-right: 1em;
}
.menu-bar .user-collection {
margin-bottom: 6em;
}
.menu-bar .install-app a {
text-decoration: none;
color: #cccccc;
padding: 0.5em 0.5em;
width: 2em;
}
.menu-bar .install-app a:hover {
color: #fff;
}
.menu-bar .install-app a .fa {
width: 2em;
margin-left: 1em;
}
.main-content header {
position: fixed;
display: flex;
justify-content: center;
align-items: center;
justify-content: flex-end;
top: 0;
width: 100%;
height: 4em;
padding: 1em;
background-color: #1DB954;
z-index: 2;
}
.main-content header .hamburger {
visibility: hidden;
position: absolute;
left: 2em;
top: 1.45em;
cursor: pointer;
}
.main-content header .hamburger div {
height: 3px;
width: 25px;
margin-bottom: 0.3em;
background-color: #fff;
}
.main-content header button {
border: none;
padding: 0.5em 0.5em 0.5em 0;
border-radius: 1.2em;
background-color: #000;
color: #fff;
}
.main-content header button .fa {
margin: 0 0.5em;
}
.main-content header ul {
padding: 0;
margin: 0;
list-style: none;
position: fixed;
right: 0;
margin: 0.3em 1.2em 0 0;
background-color: #262626;
border-radius: 3%;
padding: 0.5em;
text-align: left;
}
.main-content header ul li a {
text-decoration: none;
color: #cccccc;
padding: 0.5em 0.5em;
display: block;
padding: 0.5em 1em;
}
.main-content header ul li a:hover {
color: #fff;
}
.main-content header ul li a:hover {
background-color: #404040;
border-radius: 3%;
}
.main-content header ul li .fa {
position: relative;
margin-left: 2em;
top: 0.1em;
}
.main-content main {
margin-left: 16em;
margin-top: 4.5em;
}
.main-content main .greeting h2 {
font-weight: bold;
margin-bottom: 0.6em;
}
.main-content main .recent-activity {
margin-bottom: 1em;
display: grid;
grid-template-columns: auto auto auto;
grid-gap: 0 1.5em;
}
.main-content main .recent-activity .activity-info {
height: 5em;
width: 21em;
border-radius: 0.3em;
background-color: #1a1a1a;
margin-bottom: 1em;
display: flex;
align-items: center;
}
.main-content main .recent-activity .activity-info:hover {
transition: background-color 0.3s;
background-color: #262626;
cursor: pointer;
}
.main-content main .recent-activity .activity-info .img-div {
height: 100%;
width: 5em;
border-radius: 0;
display: flex;
justify-content: center;
align-items: center;
margin-right: 1em;
background-color: #333333;
}
.main-content main .recent-activity .activity-info .img-div img {
height: 100%;
width: 100%;
border-radius: 0;
}
.main-content main .recent-activity .activity-info p {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
width: 75%;
margin: 0;
}
.main-content main .category .title {
display: flex;
justify-content: space-between;
margin: 0;
}
.main-content main .category .title .popular-shows {
display: flex;
flex-direction: column;
}
.main-content main .category .title .popular-shows p {
font-size: 0.8em;
margin-bottom: 0.2em;
}
.main-content main .category .title .popular-shows h3 {
font-size: 1em;
margin-bottom: 0;
}
.main-content main .category .title a {
text-decoration: none;
color: #cccccc;
padding: 0.5em 0.5em;
font-size: 0.85em;
margin-right: 2em;
}
.main-content main .category .title a:hover {
color: #fff;
}
.main-content main .category .title a:hover {
text-decoration: underline;
}
.main-content main .category div {
margin-bottom: 1em;
display: grid;
grid-template-columns: auto auto auto;
grid-gap: 0 1.5em;
grid-template-columns: auto auto auto auto auto;
overflow-x: auto;
overflow-y: hidden;
/* Hide scrollbar for Chrome, Safari */
/* Hide scrollbar for IE, Edge and Firefox */
}
.main-content main .category div::-webkit-scrollbar {
display: none;
}
.main-content main .category div {
-ms-overflow-style: none;
/* IE and Edge */
scrollbar-width: none;
/* Firefox */
}
.main-content main .category div .category-info {
height: 16em;
width: 12em;
border-radius: 0.3em;
margin-top: 0.5em;
background-color: #1a1a1a;
padding: 1em;
display: flex;
flex-direction: column;
}
.main-content main .category div .category-info:hover {
transition: background-color 0.3s;
background-color: #262626;
cursor: pointer;
}
.main-content main .category div .category-info .img-div {
height: 10em;
width: 10em;
border-radius: 6%;
background-color: #333333;
display: flex;
justify-content: center;
align-items: center;
}
.main-content main .category div .category-info .img-div img {
height: 100%;
width: 100%;
border-radius: 6%;
}
.main-content main .category div .category-info p {
margin: 0;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
.main-content main .category div .category-info .category-name {
margin-bottom: 0.5em;
font-size: 0.95em;
}
.main-content main .category div .category-info .author {
color: #bfbfbf;
font-size: 0.83em;
}
.main-content main .final-category {
margin-bottom: 1em;
}
.main-content main .final-category .title .popular-shows p {
font-size: 0.7em;
}
.main-content main .final-category .title .popular-shows h3 {
padding-bottom: 0.1em;
}
@media (min-width: 1400px) {
.main-content main .category .title a {
margin-right: 3em;
}
}
@media (max-width: 1000px) {
.main-content main {
margin-left: 15em;
}
}
@media (min-width: 1100px) and (max-width: 1200px) {
.main-content main .recent-activity {
grid-template-columns: auto auto;
}
.main-content main .recent-activity .activity-info {
height: 5em;
width: 25em;
border-radius: 0.3em;
}
}
@media (min-width: 1000px) and (max-width: 1100px) {
.main-content main .recent-activity {
grid-template-columns: auto auto;
justify-content: center;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 21em;
border-radius: 0.3em;
}
}
@media (min-width: 900px) and (max-width: 1000px) {
.main-content main .recent-activity {
grid-template-columns: auto auto;
justify-content: center;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 19em;
border-radius: 0.3em;
}
}
@media (min-width: 800px) and (max-width: 900px) {
.main-content main .recent-activity {
grid-template-columns: auto auto;
grid-gap: 0.5em;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 17em;
border-radius: 0.3em;
}
.main-content main .recent-activity .activity-info:nth-child(n+5) {
display: none;
}
.main-content main .recent-activity .activity-info .img-div {
margin-right: 0.5em;
}
}
@media (min-width: 700px) and (max-width: 800px) {
.main-content main .recent-activity {
grid-template-columns: auto auto;
grid-gap: 0.5em;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 14em;
border-radius: 0.3em;
}
.main-content main .recent-activity .activity-info:nth-child(n+5) {
display: none;
}
.main-content main .recent-activity .activity-info .img-div {
margin-right: 0.5em;
}
}
@media (max-width: 700px) {
.menu-bar {
visibility: hidden;
}
.main-content header .hamburger {
visibility: visible;
}
.main-content main {
margin-left: 0;
padding: 0.5em;
}
.main-content main .category .title a {
margin-right: 0;
}
.main-content main .recent-activity {
justify-content: center;
grid-template-columns: auto auto;
grid-gap: 0.5em;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 16em;
border-radius: 0.3em;
margin-bottom: 0.2em;
}
.main-content main .recent-activity .activity-info:nth-child(n+5) {
display: none;
}
.main-content main .recent-activity .activity-info .img-div {
margin-right: 0.5em;
}
.main-content main .category div .category-info {
margin-top: 0.2em;
}
.main-content main .greeting h2 {
margin-bottom: 0.5em;
}
}
@media (max-width: 530px) {
.main-content main .recent-activity .activity-info {
height: 4em;
width: 12em;
border-radius: 0.3em;
margin-bottom: 0.2em;
}
}
@media (max-width: 400px) {
.main-content main .recent-activity {
justify-content: center;
grid-template-columns: auto;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 12em;
border-radius: 0.3em;
margin-bottom: 0.2em;
}
.main-content main .recent-activity .activity-info {
height: 4em;
width: 16em;
border-radius: 0.3em;
margin-bottom: 0.2em;
}
.main-content main .recent-activity .activity-info:nth-child(n+3) {
display: none;
}
}
JS (script.js)
class App extends React.Component {
constructor(props) {
super(props);
}
render() {
return /*#__PURE__*/(
React.createElement("div", null, /*#__PURE__*/
React.createElement(Menu, null), /*#__PURE__*/
React.createElement(MainContent, null)));
}}
const Menu = () => {
return /*#__PURE__*/(
React.createElement("div", { id: "menu-bar", class: "menu-bar" }, /*#__PURE__*/
React.createElement("span", null, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("img", { src: "https://getheavy.com/wp-content/uploads/2019/12/spotify2019-830x350.jpg", alt: "Spotify Logo" }))), /*#__PURE__*/
React.createElement("nav", { class: "navbar" }, /*#__PURE__*/
React.createElement("ul", null, /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { class: "active", href: "#" }, /*#__PURE__*/React.createElement("svg", { viewBox: "0 0 512 512", width: "24", height: "24", xmlns: "http://www.w3.org/2000/svg" }, /*#__PURE__*/React.createElement("path", { d: "M448 463.746h-149.333v-149.333h-85.334v149.333h-149.333v-315.428l192-111.746 192 110.984v316.19z", fill: "currentColor" })), "Home")), /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("svg", { viewBox: "0 0 512 512", width: "24", height: "24", xmlns: "http://www.w3.org/2000/svg" }, /*#__PURE__*/React.createElement("path", { d: "M349.714 347.937l93.714 109.969-16.254 13.969-93.969-109.969q-48.508 36.825-109.207 36.825-36.826 0-70.476-14.349t-57.905-38.603-38.603-57.905-14.349-70.476 14.349-70.476 38.603-57.905 57.905-38.603 70.476-14.349 70.476 14.349 57.905 38.603 38.603 57.905 14.349 70.476q0 37.841-14.73 71.619t-40.889 58.921zM224 377.397q43.428 0 80.254-21.461t58.286-58.286 21.461-80.254-21.461-80.254-58.286-58.285-80.254-21.46-80.254 21.46-58.285 58.285-21.46 80.254 21.46 80.254 58.285 58.286 80.254 21.461z", fill: "currentColor", "fill-rule": "evenodd" })), "Search")), /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("svg", { viewBox: "0 0 512 512", width: "24", height: "24", xmlns: "http://www.w3.org/2000/svg" }, /*#__PURE__*/React.createElement("path", { d: "M291.301 81.778l166.349 373.587-19.301 8.635-166.349-373.587zM64 463.746v-384h21.334v384h-21.334zM192 463.746v-384h21.334v384h-21.334z", fill: "currentColor" })), "Your Library")))), /*#__PURE__*/
React.createElement("nav", { class: "user-collection" }, /*#__PURE__*/
React.createElement("ul", null, /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("i", { class: "fa fa-plus-square fa-lg", "aria-hidden": "true" }), "Create Playlist")), /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("i", { class: "fa fa-heart fa-lg", "aria-hidden": "true" }), "Liked Songs")), /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("i", { class: "fa fa-podcast fa-lg", "aria-hidden": "true" }), "Your Episodes")))), /*#__PURE__*/
React.createElement("span", { class: "install-app" }, /*#__PURE__*/React.createElement("a", { href: "#" }, /*#__PURE__*/React.createElement("i", { class: "fa fa-arrow-circle-down fa-lg", "aria-hidden": "true" }), "Install App"))));
};
const MainContent = () => {
return /*#__PURE__*/(
React.createElement("div", { class: "main-content" }, /*#__PURE__*/
React.createElement(Header, null), /*#__PURE__*/
React.createElement(Body, null)));
};
const Header = () => {
const [profileVisibility, setProfileVisibility] = React.useState('hidden');
const handleClick = () => {
if (profileVisibility == 'hidden') {
setProfileVisibility('visible');
} else
{
setProfileVisibility('hidden');
}
};
const [menuBarVisibility, setMenuBarVisibility] = React.useState('visible');
const handleMenuBar = () => {
if (menuBarVisibility == 'hidden') {
setMenuBarVisibility('visible');
document.getElementById('bar1').style.transform = 'none';
document.getElementById('bar1').style.transition = 'transform .4s ease';
document.getElementById('bar2').style.opacity = 1;
document.getElementById('bar2').style.transition = 'opacity .5s ease';
document.getElementById('bar3').style.transform = 'none';
document.getElementById('bar3').style.transition = 'transform .4s ease';
document.getElementById('menu-bar').style.transition = 'visibility .2s ease-in-out';
} else
{
setMenuBarVisibility('hidden');
document.getElementById('bar1').style.transform = 'rotate(-45deg) translate(-9px, 6px)';
document.getElementById('bar1').style.transition = 'transform .4s ease';
document.getElementById('bar2').style.opacity = 0;
document.getElementById('bar2').style.transition = 'opacity .5s ease';
document.getElementById('bar3').style.transform = 'rotate(45deg)translate(-5px, -3px)';
document.getElementById('bar3').style.transition = 'transform .4s ease';
document.getElementById('menu-bar').style.transition = 'visibility .2s ease-n-out';
}
document.getElementById('menu-bar').style.visibility = menuBarVisibility;
};
return /*#__PURE__*/(
React.createElement("header", null, /*#__PURE__*/
React.createElement("div", null, /*#__PURE__*/
React.createElement("div", { onClick: handleMenuBar, class: "hamburger" }, /*#__PURE__*/
React.createElement("div", { id: "bar1", class: "bar1" }), /*#__PURE__*/
React.createElement("div", { id: "bar2", class: "bar2" }), /*#__PURE__*/
React.createElement("div", { id: "bar3", class: "bar3" })), /*#__PURE__*/
React.createElement("div", { class: "dropdown" }, /*#__PURE__*/
React.createElement("button", { onClick: handleClick }, /*#__PURE__*/
React.createElement("i", { class: "fa fa-user-circle fa-lg", "aria-hidden": "true" }), "curious_coder", /*#__PURE__*/
React.createElement("i", { className: `fa fa-caret-${profileVisibility == 'hidden' ? 'down' : 'up'}`, "aria-hidden": "true" })), /*#__PURE__*/
React.createElement("ul", { style: { visibility: `${profileVisibility}` } }, /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#", target: "_blank" }, "Account", /*#__PURE__*/React.createElement("i", { class: "fa fa-external-link", "aria-hidden": "true" }))), /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, "Profile")), /*#__PURE__*/
React.createElement("li", null, /*#__PURE__*/React.createElement("a", { href: "#" }, "Log Out")))))));
};
const Body = () => {
const hours = new Date().getHours();
const greeting = hours < 12 ? "Morning" : hours < 17 ? "Afternoon" : "Evening";
return /*#__PURE__*/(
React.createElement("main", null, /*#__PURE__*/
React.createElement("div", { class: "greeting" }, /*#__PURE__*/
React.createElement("h2", null, "Good ", greeting)), /*#__PURE__*/
React.createElement("div", { class: "recent-activity" }, /*#__PURE__*/
React.createElement("div", { class: "activity-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://seed-mix-image.spotifycdn.com/v6/img/pop/4AK6F7OLvEQ5QYCBNiQWHq/en/default", alt: "Pop Mix playlist cover photo" })), /*#__PURE__*/
React.createElement("p", null, "Pop Mix")), /*#__PURE__*/
React.createElement("div", { class: "activity-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1f854bce22cb0e6890dba92dd8", alt: "The Athletic Football Tactics Podcast cover photo" })), /*#__PURE__*/
React.createElement("p", null, "The Athletic Football Tactics Podcast")), /*#__PURE__*/
React.createElement("div", { class: "activity-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f00000002b75cdf3f088c129cc350c0f8", alt: "This Is One Direction playlist cover photo" })), /*#__PURE__*/
React.createElement("p", null, "This Is One Direction")), /*#__PURE__*/
React.createElement("div", { class: "activity-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab6761610000e5eb6e9a17ce6d67c02312e3fb89", alt: "Alessia Cara cover photo" })), /*#__PURE__*/
React.createElement("p", null, "Alessia Cara")), /*#__PURE__*/
React.createElement("div", { class: "activity-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://dailymix-images.scdn.co/v2/img/ab6761610000e5eb26dbdbdacda5c30dc95e0c2c/3/en/default", alt: "Daily Mix 3 playlist cover photo" })), /*#__PURE__*/
React.createElement("p", null, "Daily Mix 3")), /*#__PURE__*/
React.createElement("div", { class: "activity-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f000000021373358fa4ff03aac54f188e", alt: "All Out 10s playlist cover photo" })), /*#__PURE__*/
React.createElement("p", null, "All Out 10s"))), /*#__PURE__*/
React.createElement("div", { class: "category" }, /*#__PURE__*/
React.createElement("div", { class: "title" }, /*#__PURE__*/
React.createElement("h3", null, "Your Shows"), /*#__PURE__*/
React.createElement("a", { href: "#" }, "SEE ALL")), /*#__PURE__*/
React.createElement("div", null, /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/a3313c9ff4f806345e71728b502022782e92cf34", alt: "HTML All The Things podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "HTML All The Things"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. Matt & Mike")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1f854bce22cb0e6890dba92dd8", alt: "The Athletic Football Podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "The Athletic Football Podcast"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. The Athletic")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1fcf5b0c37fe67ebbcdceb930b", alt: "Headline: Breaking Football News playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Headline: Breaking Football News"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. The Athletic")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/fedc8e1c8b93cc9b8e49e8e101ec9d9b8795d1fe", alt: "Raj Prakash Paul playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Raj Prakash Paul"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. Raj Prakash Paul")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1f6d655e4364ad1ed1dad7a83d", alt: "The Here We Go Podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "The Here We Go Podcast"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. Here We Go")))), /*#__PURE__*/
React.createElement("div", { class: "category" }, /*#__PURE__*/
React.createElement("div", { class: "title" }, /*#__PURE__*/
React.createElement("h3", null, "Made For curious_coder"), /*#__PURE__*/
React.createElement("a", { href: "#" }, "SEE ALL")), /*#__PURE__*/
React.createElement("div", null, /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://dailymix-images.scdn.co/v2/img/ab6761610000e5eb031619e5eb9ed3b9806b648b/1/en/default", alt: "Daily Mix 1 cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Daily Mix 1"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Ella Mai, Shawn Mendes, Jason Derulo")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://dailymix-images.scdn.co/v2/img/ab6761610000e5eb6e9a17ce6d67c02312e3fb89/2/en/default", alt: "Daily Mix 2 cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Daily Mix 2"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Alessia Cara, 5 Seconds Of Summer")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://dailymix-images.scdn.co/v2/img/ab6761610000e5eb26dbdbdacda5c30dc95e0c2c/3/en/default", alt: "Daily Mix 3 cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Daily Mix 3"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Taylor Swift, Fifth Harmony")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://dailymix-images.scdn.co/v2/img/ab6761610000e5eb7aff8a274fcec288dd534abc/4/en/default", alt: "Daily Mix 4 cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Daily Mix 4"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Jesus Culture, Bethel Music")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://dailymix-images.scdn.co/v2/img/ab6761610000e5eb70859a2e628fd00e8be3a696/5/en/default", alt: "Daily Mix 5 cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Daily Mix 5"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Benny Joshua, Allen Ganta")))), /*#__PURE__*/
React.createElement("div", { class: "category" }, /*#__PURE__*/
React.createElement("div", { class: "title" }, /*#__PURE__*/
React.createElement("h3", null, "Charts"), /*#__PURE__*/
React.createElement("a", { href: "#" }, "SEE ALL")), /*#__PURE__*/
React.createElement("div", null, /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://charts-images.scdn.co/assets/locale_en/regional/daily/region_in_default.jpg", alt: "Top 50 India playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Top 50 India")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f00000002b545db24c5864981ff896f07", alt: "Hot Hits India playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Hot Hits India")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://charts-images.scdn.co/assets/locale_en/regional/daily/region_global_default.jpg", alt: "Top 50 Global playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Top 50 Global")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706c0000da84fc156bed23ef2df5814fb190", alt: "Top Albums - Global playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Top Albums - Global")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://charts-images.scdn.co/assets/locale_en/viral/daily/region_global_default.jpg", alt: "Viral 50 - India playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Viral 50 - India")))), /*#__PURE__*/
React.createElement("div", { class: "category" }, /*#__PURE__*/
React.createElement("div", { class: "title" }, /*#__PURE__*/
React.createElement("h3", null, "Best Of Artists"), /*#__PURE__*/
React.createElement("a", { href: "#" }, "SEE ALL")), /*#__PURE__*/
React.createElement("div", null, /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f000000021230c7f75023a90181e914a0", alt: "This is Alessia Cara playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "This Is Alessia Cara")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f00000002a0a577ed169a7792c9363d6c", alt: "This is Hillsong Worship playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "This Is Hillsong Worship")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f00000002181896dd694bc09e4a0f13c8", alt: "This is Camila Cabello playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "This Is Camila Cabello")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f000000027988283d13d5654287988494", alt: "This is Shawn Mendes playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "This Is Shawn Mendes")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67706f00000002b75cdf3f088c129cc350c0f8", alt: "This is One Direction playlist cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "This Is One Direction")))), /*#__PURE__*/
React.createElement("div", { class: "category final-category" }, /*#__PURE__*/
React.createElement("div", { class: "title" }, /*#__PURE__*/
React.createElement("div", { class: "popular-shows" }, /*#__PURE__*/
React.createElement("p", null, "POPULAR WITH LISTENERS OF"), /*#__PURE__*/
React.createElement("h3", null, "Headline: Breaking Football News")), /*#__PURE__*/
React.createElement("a", { href: "#" }, "SEE ALL")), /*#__PURE__*/
React.createElement("div", null, /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1f6070c8c3beddfeef90cd9044", alt: "Football Cliches podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Football Cliches"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. The Athletic")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1fff3db692e1f2dbe7c73951e2", alt: "The Athletic Football Podcast podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "The Athletic Football Podcast"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. The Athletic")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1fea8a7821ffed11a7bfe73c71", alt: "Beyond the Headline podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "Beyond the Headline"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. The Athletic")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/ab67656300005f1f31a9464d4951d231128babc6", alt: "The Next Big Thing podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "The Next Big Thing"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. The Athletic")), /*#__PURE__*/
React.createElement("div", { class: "category-info" }, /*#__PURE__*/
React.createElement("div", { class: "img-div" }, /*#__PURE__*/
React.createElement("img", { src: "https://i.scdn.co/image/bdd990bddb85baa44c320b2ffba328549e184643", alt: "The Scouted Football Podcast podcast cover photo" })), /*#__PURE__*/
React.createElement("p", { class: "category-name" }, "The Scouted Football Podcast"), /*#__PURE__*/
React.createElement("p", { class: "author" }, "Show. Scouted Football"))))));
};
ReactDOM.render( /*#__PURE__*/React.createElement(App, null), document.getElementById('root'));
Output
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …