Interactive Login and Registration Form Using HTML , CSS & JavaScript With Source Code
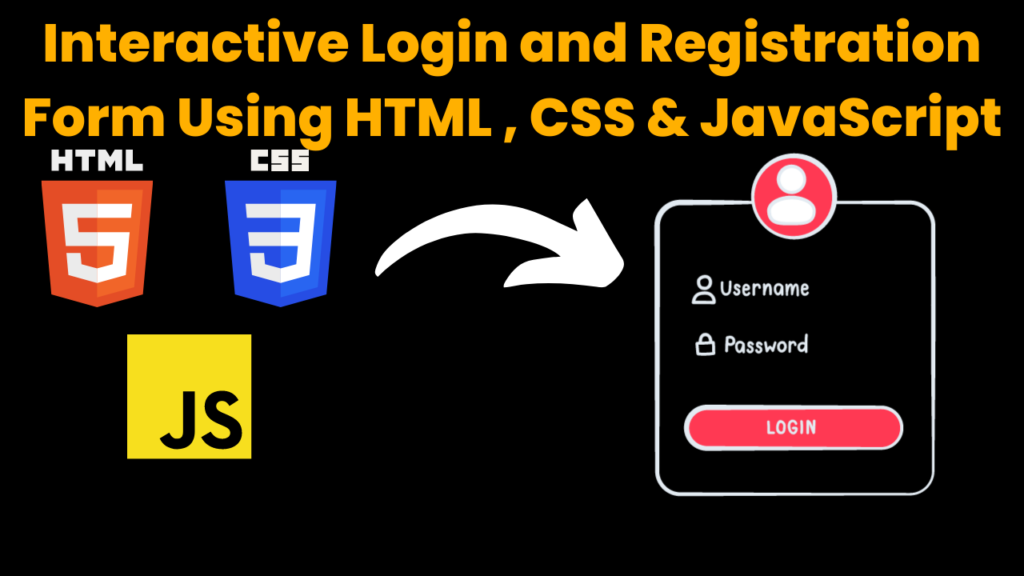
Introduction:
The Login and Registration Form project aims to create a user authentication system where users can log in using their credentials or register for a new account. The project utilizes HTML for structuring the form, CSS for styling and layout, and JavaScript for form validation and submission.
Key Features:
- User Registration: Users can create a new account by providing their desired username, email address, and password.
- Form Validation: The form validates user inputs to ensure that the required fields are filled correctly.
- Password Strength Meter: The password field includes a strength meter to indicate the strength of the user’s chosen password.
- Password Confirmation: The registration form includes a password confirmation field to ensure that users enter the same password twice.
- Error Handling: The form provides error messages to guide users in case of invalid inputs or missing fields.
- User Login: Existing users can log in using their registered username and password.
- Persistence: The project can be extended to include backend functionality to store and authenticate user credentials.
Source Code:
HTML:
Login and Registration Form
Login and Registration Form
CSS (style.css):
body {
font-family: Arial, sans-serif;
background-color: #f5f5f5;
text-align: center;
padding: 20px;
}
h1 {
color: #333;
}
.form-container {
display: flex;
justify-content: space-around;
margin-top: 30px;
}
form {
background-color: #fff;
border-radius: 5px;
padding: 20px;
width: 300px;
}
h2 {
margin-top: 0;
color: #333;
}
label {
display: block;
margin-bottom: 5px;
}
input {
width: 100%;
padding: 8px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 4px;
}
button {
width: 100%;
padding: 10px;
background-color: #4caf50;
color: #fff;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
JavaScript (script.js):
document.getElementById('loginForm').addEventListener('submit', function(event) {
event.preventDefault();
const username = document.getElementById('loginUsername').value;
const password = document.getElementById('loginPassword').value;
// Check if username and password are valid
if (username === 'admin' && password === 'password') {
// Successful login
alert('Login Successful');
} else {
// Invalid login
alert('Invalid username or password');
}
});
document.getElementById('registrationForm').addEventListener('submit', function(event) {
event.preventDefault();
const username = document.getElementById('regUsername').value;
const email = document.getElementById('regEmail').value;
const password = document.getElementById('regPassword').value;
const confirmPassword = document.getElementById('regConfirmPassword').value;
// Check if all fields are filled
if (username && email && password && confirmPassword) {
// Check if passwords match
if (password === confirmPassword) {
// Successful registration
alert('Registration Successful');
// Reset the form
document.getElementById('registrationForm').reset();
} else {
// Passwords don't match
alert('Passwords do not match');
}
} else {
// Missing fields
alert('Please fill in all fields');
}
});
Output :
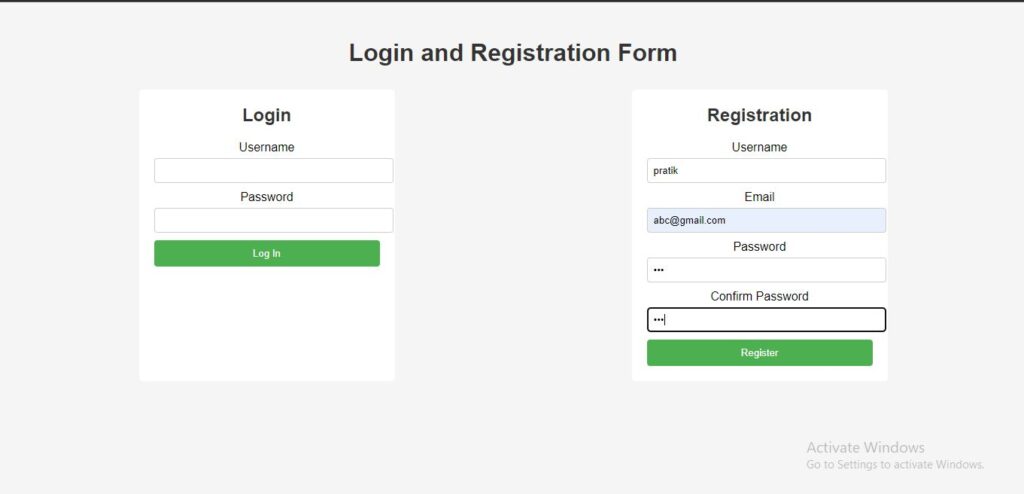
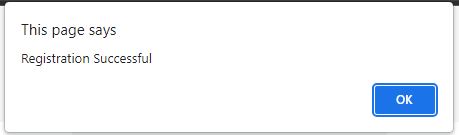
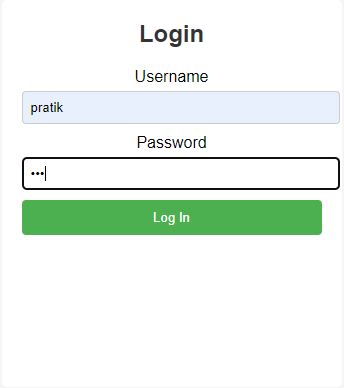
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …