Web Based Guess the Number Using HTML , CSS & JavaScript With Source Code
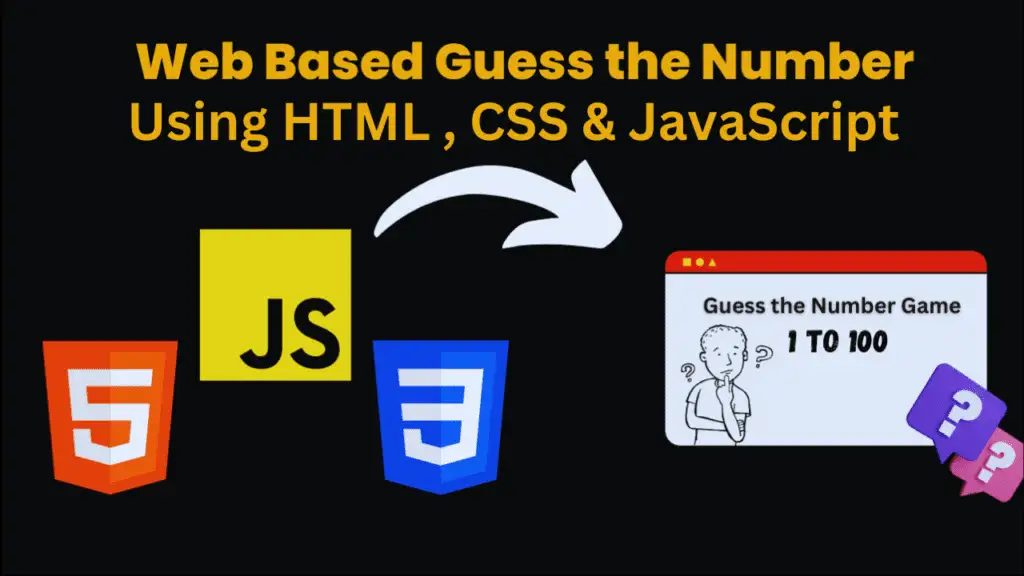
Introduction:
The “Guess the Number” game is a simple web-based game where players have to guess a random number between 1 and 100. It is designed to provide an enjoyable and interactive experience for users of all ages. The game interface is user-friendly and responsive, ensuring compatibility across different devices.
Key Features:
Random Number Generation: The game generates a random number between 1 and 100 at the start of each round, ensuring a unique guessing challenge for each player.
User Input: Players can enter their guess in an input field provided in the game interface. The input field only allows numeric values, restricting any non-numeric entries.
Feedback Messages: After submitting a guess, players receive feedback on their guess. The game interface displays messages indicating whether the guess is too low, too high, or the correct answer. The messages are displayed in a visually prominent manner, making it easy for players to understand their progress.
Attractive User Interface: The game features an attractive user interface with a responsive design. It utilizes the Bootstrap framework to provide a visually appealing layout, including a centered container, stylish buttons, and clear instructions.
Easy to Play: The game is simple and easy to play, making it accessible for players of all skill levels. With just a few clicks, players can participate and enjoy the guessing challenge.
Engaging Gameplay: The “Guess the Number” game offers an engaging gameplay experience. Players can make multiple attempts to guess the correct number and experience the excitement of getting closer to the answer with each try.
Responsive Design: The game interface is designed to be responsive, allowing players to enjoy the game seamlessly on various devices, including desktops, tablets, and mobile phones.
The “Guess the Number” game provides an entertaining and interactive experience for players, challenging their ability to guess the correct number within a specified range. Its simplicity and user-friendly interface make it an ideal choice for casual gaming and can be easily customized and expanded upon to add additional features and complexity.
Source Code:
Get Discount on Top Educational Courses
HTML:
Guess the Number Game
Guess the Number Game
Guess a number between 1 and 100:
CSS (style.css):
body {
font-family: Arial, sans-serif;
background-color: #f7f7f7;
}
.container {
max-width: 400px;
margin: 0 auto;
text-align: center;
padding-top: 50px;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
padding: 20px;
}
h1 {
color: #333;
font-weight: bold;
margin-bottom: 20px;
}
.lead {
color: #555;
margin-bottom: 20px;
}
.btn-primary {
width: 100%;
background-color: #4CAF50;
border-color: #4CAF50;
}
.btn-primary:hover {
background-color: #45a049;
border-color: #45a049;
}
#message {
font-weight: bold;
margin-top: 20px;
color: #333;
}
JavaScript (script.js):
// Generate a random number between 1 and 100
const randomNumber = Math.floor(Math.random() * 100) + 1;
// Function to check the user's guess
function checkGuess() {
// Get the user's guess from the input field
const userGuess = parseInt(document.getElementById('userGuess').value);
// Get the message element
const message = document.getElementById('message');
// Check if the user's guess is correct, too high, or too low
if (userGuess === randomNumber) {
message.style.color = 'green';
message.textContent = 'Congratulations! You guessed the correct number!';
} else if (userGuess < randomNumber) {
message.style.color = 'red';
message.textContent = 'Too low. Try again.';
} else {
message.style.color = 'red';
message.textContent = 'Too high. Try again.';
}
}
Output:
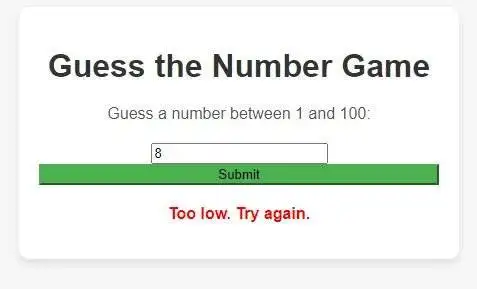
Find More Projects
MathGenius Pro – AI-Powered Math Solver Using Python Introduction: From simple arithmetic to more complicated college-level subjects like integration, differentiation, algebra, matrices, …
CipherMaze: The Ultimate Code Cracking Quest Game Using Python Introduction: You can make CipherMaze, a fun and brain-boosting puzzle game, with Python …
Warp Perspective Using Open CV Python Introduction: In this article, we are going to see how to Create a Warp Perspective System …
Custom AI Story Generator With Emotion Control Using Python Introduction: With the help of this AI-powered story generator, users can compose stories …
AI Powered PDF Summarizer Using Python Introduction: AI-Powered PDF Summarizer is a tool that extracts and summarizes research papers from ArXiv PDFs using Ollama (Gemma 3 LLM). The …
AI Based Career Path Recommender Using Python Introduction: One of the most significant and frequently perplexing decisions in a person’s life is …