Dictionary App using HTML, CSS, JavaScript & ReactJs
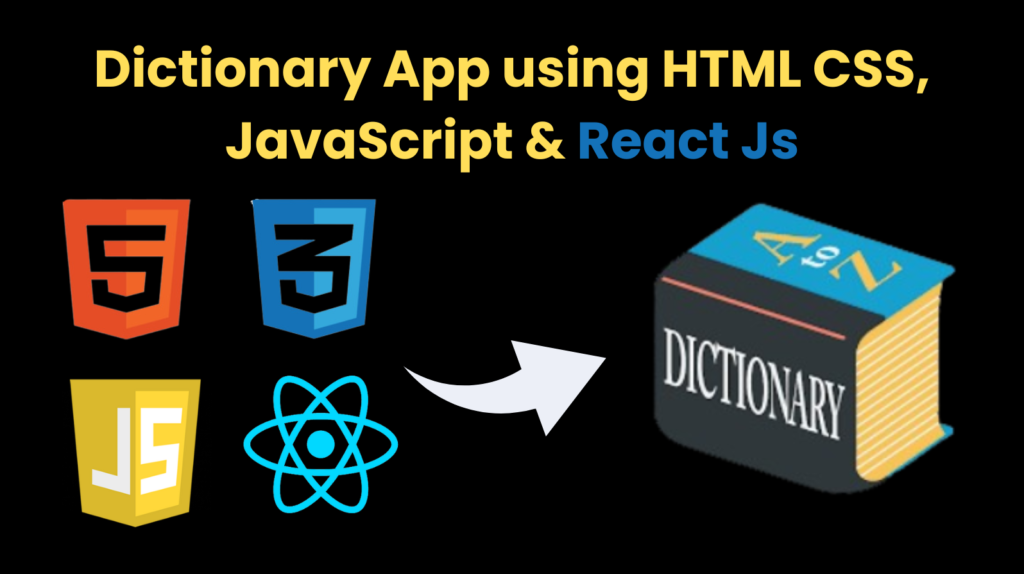
Introduction :
The React dictionary app is a web application designed to provide users with a seamless and intuitive experience for exploring word definitions. Leveraging the power of React, a popular JavaScript library for building user interfaces, the app offers a modern and dynamic platform for accessing a wealth of lexical information.
Core Features:
Search Functionality: Users can easily search for the definitions of words they’re interested in. The app fetches and displays comprehensive definitions from a reliable source, offering users accurate and up-to-date information.
Bookmarking System: To enhance user convenience, the app incorporates a robust bookmarking system. Users can save their favorite words for quick access later, streamlining the process of revisiting terms of interest.
Responsive Design: With a responsive layout, the app ensures a consistent and enjoyable experience across various devices and screen sizes. Whether accessed on a desktop, tablet, or smartphone, users can seamlessly interact with the app’s features.
Material-UI Integration: The app leverages Material-UI, a popular React UI framework, for its visual design and components. This integration not only enhances the aesthetic appeal of the app but also ensures consistency and accessibility in its user interface.
Technologies:
React: At the heart of the application lies React, a powerful JavaScript library maintained by Facebook. React’s component-based architecture facilitates the creation of reusable and modular user interface components, enabling developers to build complex applications with ease.
LocalStorage: The app utilizes the browser’s localStorage API to persist user bookmarks locally. This ensures that users can access their saved words even after closing and reopening the app, enhancing the overall user experience.
Routing with React Router: To enable seamless navigation between different views of the app, React Router is employed. This powerful routing library allows for declarative routing within a React application, enabling the creation of dynamic and intuitive navigation structures.
The React dictionary app prioritizes user experience, striving to provide a frictionless and enjoyable journey for users seeking word definitions. From its clean and intuitive interface to its efficient search and bookmarking functionalities, every aspect of the app is meticulously crafted to meet the needs and expectations of its users.
Explanation :
App.js
useState and useEffect:
useState
is a React hook used to manage state within functional components. Here, it initializes thebookmarks
state with data retrieved from local storage or an empty object if no data is found.useEffect
is another React hook used to perform side effects in functional components. In this case, it’s used to synchronize thebookmarks
state with local storage whenever it changes.
addBookmark Function:
- This function takes two parameters:
word
anddefinitions
. - It updates the
bookmarks
state by adding a new entry where the word is the key and its definitions are the value.
- This function takes two parameters:
removeBookmark Function:
- This function takes a single parameter:
word
. - It updates the
bookmarks
state by removing the entry corresponding to the provided word.
- This function takes a single parameter:
index.js
- ReactDOM.render:
- This function renders the
<App />
component into the DOM. - It is wrapped in
<React.StrictMode>
to enable additional checks and warnings for potential issues in development mode.
- This function renders the
theme.js
- Theme Definition:
- This file defines the Material-UI theme used throughout the application.
- It specifies colors, typography, and mixins to ensure consistent styling across components.
Purpose of Functions
addBookmark Function:
- Purpose: To add a word and its definitions to the bookmarks.
- It facilitates the addition of new words to the user’s bookmark list, allowing them to easily access and revisit their favorite terms.
removeBookmark Function:
- Purpose: To remove a word from the bookmarks.
- It provides the functionality to remove unwanted or no longer relevant words from the user’s bookmark list, ensuring efficient management of saved terms.
Instructions to run this project :
Step 01 : Download the zip file of source code (given below) and extract it.
Step 02 : Navigate to the Project Directory:
Once the repository is cloned, navigate into the project directory using the cd command: cd <project_directory>
Replace <project_directory> with the name of the directory where the project was cloned.
Step 03 : Install Dependencies:
Inside the project directory, run the following command to install project dependencies using Yarn : yarn install
This command will read the package.json file and install all required dependencies listed in it.
Step 04 : Run the Project:
After installing dependencies, you can start the development server using the following command: yarn start
This command will start the development server and automatically open the project in your default web browser.
Once the development server is up and running, you can access the project by opening your web browser and navigating to the specified address (usually http://localhost:3000).
You can now explore the e-commerce app, navigate through different pages, interact with components, and experience the functionality firsthand.
SOURCE CODE :
OUTPUT :
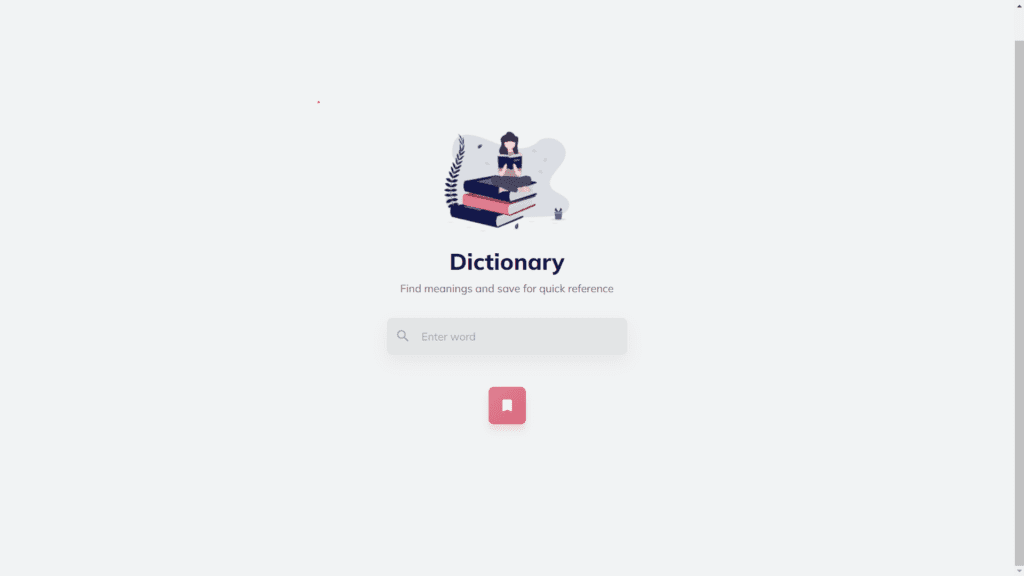
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …