Countdown Timer Using HTML , CSS & JavaScript
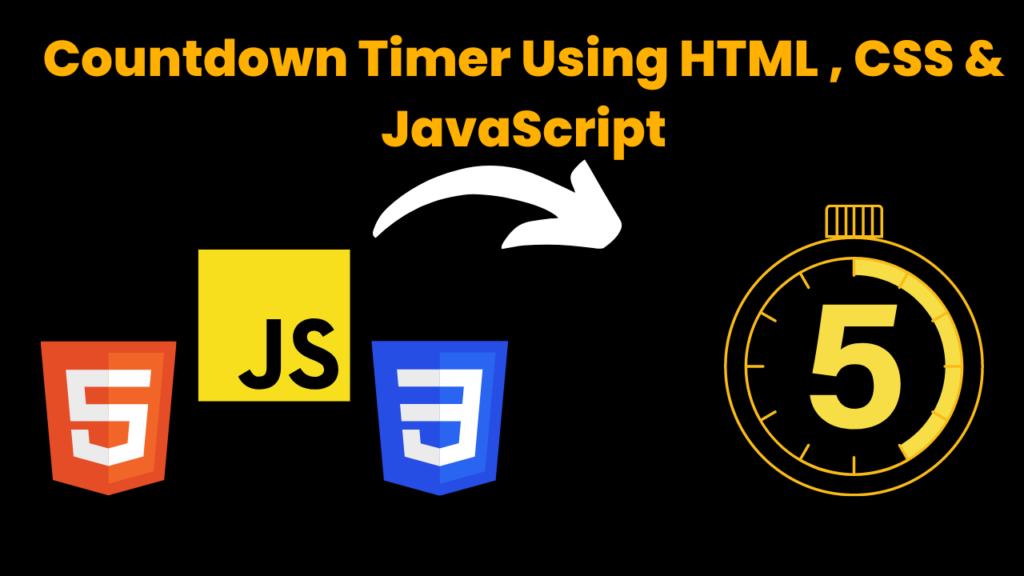
Introduction:
The Countdown Timer project is an interactive web application that allows users to set a specific date and time for an upcoming event, product launch, or special occasion. It provides a visual representation of the time remaining until the specified event occurs, building excitement and anticipation among users.
This web-based countdown timer utilizes HTML, CSS, and JavaScript to create an intuitive and engaging user experience. Users can input the desired date and time through a user-friendly interface, and the countdown timer dynamically calculates and displays the remaining time in days, hours, minutes, and seconds.
The project aims to provide a versatile tool that can be easily customized to suit various purposes. It allows users to personalize the countdown timer by adding event titles, background images, and additional features based on their specific requirements.
Key Features:
User-friendly interface: The countdown timer features a simple and intuitive user interface, allowing users to easily set the target date and time.
Real-time countdown: The timer updates in real-time, accurately displaying the remaining time until the event occurs. It dynamically calculates the time difference between the current date and time and the user-specified target.
Countdown display: The timer showcases the remaining time in days, hours, minutes, and seconds, creating a sense of anticipation for the upcoming event.
Customization options: Users can personalize the countdown timer by adding event titles, selecting background images, and modifying the appearance and style of the timer to align with their preferences and branding.
Responsive design: The countdown timer is designed to be responsive and compatible with various screen sizes and devices, ensuring a seamless user experience across desktops, tablets, and mobile devices.
Benefits:
- Engaging user experience: The visual representation of the countdown timer builds excitement and anticipation among users, creating a sense of anticipation for the upcoming event or occasion.
- Versatile and customizable: The countdown timer can be easily customized and adapted for different purposes, such as product launches, special occasions, or event reminders.
- Simple setup and usage: Users can quickly set the target date and time through the user-friendly interface, making it accessible to a wide range of users.
- Mobile-friendly: The responsive design ensures that the countdown timer can be accessed and enjoyed on various devices, providing flexibility and convenience.
The Countdown Timer project provides a valuable tool for individuals, businesses, and organizations looking to create a sense of anticipation and excitement around upcoming events or occasions. Its simplicity, customizability, and engaging user experience make it a valuable addition to any web development portfolio.
Explanation:
The Countdown Timer project is a web application that allows users to set a specific date and time for an upcoming event, product launch, or special occasion. It provides a visual representation of the time remaining until the specified event occurs, creating anticipation and excitement among users. The project utilizes HTML, CSS, and JavaScript to create an intuitive and engaging user experience. Users can input the desired date and time through a user-friendly interface, and the countdown timer dynamically calculates and displays the remaining time in days, hours, minutes, and seconds. Customization options are available to personalize the timer, including event titles, background images, and styling.
Source Code:
HTML:
Countdown Timer
Countdown Timer
0
Days
0
Hours
0
Minutes
0
Seconds
CSS (style.css):
#countdown {
text-align: center;
}
#timer {
display: flex;
justify-content: center;
}
#timer div {
margin: 0 10px;
}
#timer span {
display: block;
font-size: 24px;
}
JavaScript (script.js):
// Set the target date and time for the countdown
const targetDate = new Date("June 10, 2023 15:00:00").getTime();
// Update the countdown every second
const countdownInterval = setInterval(() => {
// Get the current date and time
const now = new Date().getTime();
// Calculate the time difference between the current date/time and the target date/time
const timeDifference = targetDate - now;
// Calculate days, hours, minutes, and seconds
const days = Math.floor(timeDifference / (1000 * 60 * 60 * 24));
const hours = Math.floor(
(timeDifference % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)
);
const minutes = Math.floor((timeDifference % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((timeDifference % (1000 * 60)) / 1000);
// Display the calculated time in the HTML elements
document.getElementById("days").textContent = days;
document.getElementById("hours").textContent = hours;
document.getElementById("minutes").textContent = minutes;
document.getElementById("seconds").textContent = seconds;
// Check if the countdown has expired
if (timeDifference < 0) {
clearInterval(countdownInterval);
document.getElementById("timer").innerHTML = "Countdown expired";
}
}, 1000);
Make sure to save the HTML file as index.html
, the CSS file as style.css
, and the JavaScript file as script.js
in the same directory. You can then open the index.html
file in a web browser to see the countdown timer in action.
Output:
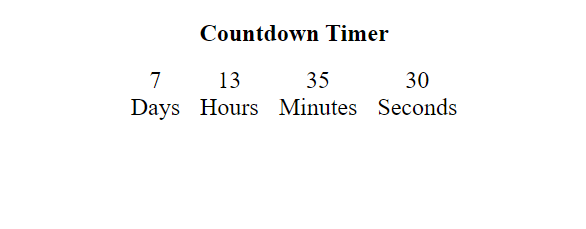
Find More Projects
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …
Chat Application Using HTML , CSS And Javascript Introduction Hello developers friends, all of you are welcome to this new and wonderful …
Travel Planner Website Using HTML , CSS And Javascript Introduction Hello friends, welcome to all of you developer friends, in this new …
Dictionary App using HTML, CSS, JavaScript & ReactJs Introduction : The React dictionary app is a web application designed to provide users …
Weather App using HTML, CSS, JavaScript & ReactJs Introduction : The React Weather App is a web application designed to provide users …