Flappy Bird Using HTML, CSS and JS
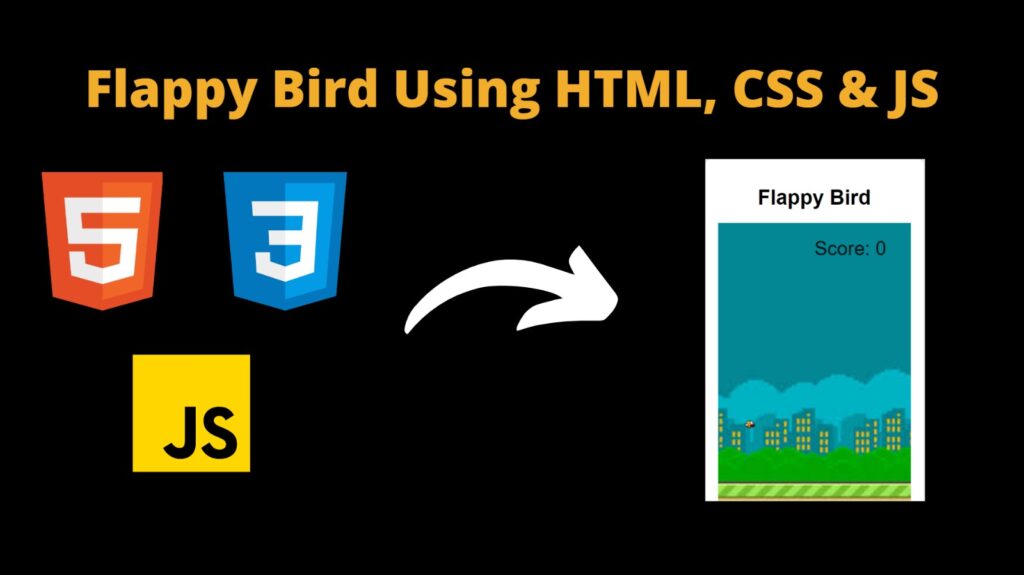
Introduction
In this project, we delve into the fascinating world of game development by creating a classic arcade-style game known as Flappy Bird. Inspired by the immensely popular mobile game, our project aims to provide an engaging and interactive experience for players of all ages. Using HTML, CSS, and JavaScript, we embark on a journey to build the foundation of Flappy Bird, incorporating essential game mechanics such as player control, obstacle avoidance, and scoring systems. Let’s dive into the intricacies of game development and witness the magic unfold as we bring Flappy Bird to life on the web platform.
Steps to create Flappy Bird
Set Up HTML Structure:
- Create an HTML file (e.g., index.html).
- Inside the HTML file, set up the basic structure including
<html>
,<head>
,<title>
, and<body>
tags.
Create Canvas Element:
- Within the
<body>
tag, add a<canvas>
element to serve as the game board. - Give the canvas an id attribute (e.g., “gameCanvas”).
- Within the
Style with CSS:
- Create a separate CSS file (e.g., styles.css).
- Add styles to the canvas element to define its dimensions and appearance.
- Customize styles for the bird, pipes, background, and any other game elements.
Draw Game Elements with JavaScript:
- Create a JavaScript file (e.g., game.js).
- Select the canvas element using JavaScript.
- Use the canvas API (getContext) to get the drawing context.
- Write functions to draw the bird, pipes, background, and any other game elements on the canvas.
Implement Game Logic:
- Define variables to store the bird’s position, velocity, and gravity.
- Implement functions to handle user input (e.g., spacebar for bird flap).
- Write logic to move the bird up and down based on user input and gravity.
- Generate pipes at regular intervals and move them horizontally across the screen.
- Detect collisions between the bird and pipes to determine when the game ends.
Add Scoring System:
- Create a variable to keep track of the player’s score.
- Increase the score each time the bird successfully passes through a set of pipes.
Handle Game Over:
- Implement logic to detect when the bird collides with a pipe or goes out of bounds.
- Display a “Game Over” message on the canvas.
- Allow the player to restart the game.
Test and Debug:
- Test the game thoroughly to ensure all features work as expected.
- Debug any issues with game mechanics, collision detection, or user input.
Explanation
HTML
index.html
- Document Declaration:The HTML code begins with the declaration
<!DOCTYPE html>
, signifying that the document adheres to the HTML5 standard. Additionally, it specifies the language as English, ensuring proper interpretation by web browsers.
- Head Section: Within the
<head>
section, essential meta-information and external resource links are housed. This includes metadata tags for character encoding and viewport configuration, crucial for rendering the content correctly across various devices and screen sizes. - Canvas Element: A
<canvas>
element with the id “gameCanvas” is introduced within the<body>
section. This serves as the game board where Flappy Bird and game elements will be drawn dynamically using JavaScript. - Game Container: A
<div>
element with the id “game-container” encloses the game canvas and provides a container for organizing and styling game-related content. - Game Title: An
<h1>
heading displaying the title “Flappy Bird” serves as the prominent identifier for the game, providing users with a clear indication of the game’s identity and purpose. - JavaScript Integration: A
<script>
tag is included at the end of the<body>
section, linking an external JavaScript file named “game.js”. This file contains the game logic and functionality responsible for controlling the behavior of Flappy Bird, including player input, obstacle generation, and scoring. - External Stylesheet: The HTML document links an external CSS file named “styles.css” using the
<link>
tag within the<head>
section. This stylesheet is responsible for styling various game elements, ensuring consistency in appearance and enhancing the visual aesthetics of the game interface.
In summary, the HTML code establishes the foundational structure and layout for the Flappy Bird game, incorporating essential elements such as the canvas element for rendering graphics, JavaScript integration for game logic, and external styling for visual enhancement.
CSS Styling
Body Styling:
- The
body
selector applies styling to the entire webpage. margin: 0;
andpadding: 0;
remove any default margins and padding, ensuring consistent spacing.font-family: Arial, sans-serif;
specifies the font family for text content, prioritizing Arial and falling back to a generic sans-serif font if Arial is not available.background-color: #f1f1f1;
sets the background color of the webpage to a light gray (#f1f1f1), providing a neutral backdrop for content.
- The
Game Container Styling:
- The
#game-container
selector targets a specific container element with the id “game-container”. position: relative;
specifies that the container’s position is relative to its normal position, allowing absolute positioning of child elements.width: fit-content;
sets the width of the container to fit its content, ensuring that it expands or contracts based on the content inside.margin: 50px auto;
centers the container horizontally on the page with a top and bottom margin of 50 pixels, auto-calculating the left and right margins.background-color: #fff;
sets the background color of the container to white (#fff), creating a clean and minimalistic appearance.border-radius: 10px;
adds rounded corners to the container with a border radius of 10 pixels, adding a subtle visual effect.box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
applies a shadow effect to the container, providing depth and dimensionality to the layout.padding: 20px;
adds internal spacing of 20 pixels to the container, ensuring adequate space between the content and container edges.
- The
Canvas Styling:
- The
canvas
selector targets the canvas element within the game container. display: block;
sets the display property to block, making the canvas a block-level element that fills its container’s width.margin: 0 auto;
centers the canvas horizontally within its container by setting left and right margins to auto.background-image: url('bg.jpg');
specifies a background image for the canvas, using the file “bg.jpg”.background-size: cover;
ensures that the background image covers the entire canvas, maintaining aspect ratio and filling any empty space.
- The
Additional Styling:
- The
#instructions
selector targets an element with the id “instructions” to provide styling for game instructions. - The
h1
selector styles heading elements to have centered text alignment.
- The
game.js
Canvas Initialization:
- The
canvas
variable holds the reference to the HTML canvas element with the id “gameCanvas”. - The
ctx
variable stores the 2D rendering context of the canvas, enabling drawing operations.
- The
Bird Object Definition:
- The
bird
object encapsulates properties such as position (x
andy
), size (radius
), gravity, velocity, and lift. - These properties control the behavior of the bird within the game environment, including its movement and response to user input.
- The
Pipes and Scoring:
- The
pipes
array stores information about the pipes in the game, including their position and whether the bird has passed them. - The
pipeGap
variable defines the vertical gap between upper and lower pipes. - The
pipeWidth
variable specifies the width of the pipes. - The
score
variable keeps track of the player’s score as they successfully navigate through pipes.
- The
Game State Management:
- The
isGameOver
flag indicates whether the game is over. - The
attempts
variable counts the number of attempts the player has made, limiting the number of retries. - The
maxAttempts
constant determines the maximum number of attempts allowed.
- The
Image Loading:
- The
birdImg
andpipeImg
variables load image files for the bird and pipes, respectively, using theImage
constructor. - These images are later drawn on the canvas to represent game elements.
- The
Drawing Functions:
drawBird()
anddrawPipes()
functions render the bird and pipes on the canvas using thedrawImage()
method of the canvas context (ctx
).- These functions are responsible for visually representing game elements to the player.
Game Logic:
updateBird()
andupdatePipes()
functions handle the game’s core mechanics, including bird movement, pipe generation, collision detection, and scoring.- These functions update the game state based on user input and game rules, ensuring proper gameplay mechanics.
Game Over Handling:
- The
gameOver()
function is triggered when the game ends due to collision or reaching the maximum number of attempts. - It displays appropriate messages on the canvas, indicating whether the player can restart the game or if it’s over.
- The
Restart Functionality:
- The
restartListener()
function listens for the spacebar key press to restart the game after it has ended. - It resets game variables and starts the game loop again upon player input.
- The
Game Loop and Event Listeners:
- The
gameLoop()
function runs continuously to update the game state and render the game elements on the canvas. - Event listeners are added to handle user input (e.g., spacebar for bird flap) and restart the game after game over.
- The
Initialization and Start:
- The
gameLoop()
function is initially called to start the game loop and render the game on the canvas.
- The
This JavaScript code orchestrates the Flappy Bird game, managing game state, user input, rendering, and game mechanics to provide an immersive gaming experience for players.
Source Code
HTML (index.html)
Flappy Bird
Flappy Bird
CSS (styles.css)
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
background-color: #f1f1f1;
}
#game-container {
position: relative;
width: fit-content;
margin: 50px auto;
background-color: #fff;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
padding: 20px;
}
canvas {
display: block;
margin: 0 auto;
background-image: url('bg.jpg'); /* Set your background image here */
background-size: cover;
}
#instructions {
text-align: center;
margin-top: 20px;
}
h1 {
text-align: center;
}
JS (game.js)
const canvas = document.getElementById('gameCanvas');
const ctx = canvas.getContext('2d');
const bird = {
x: 50,
y: canvas.height / 2,
radius: 15,
gravity: 0.6,
velocity: 0,
lift: -12
};
const pipes = [];
const pipeGap = 250;
const pipeWidth = 100;
let score = 0;
let isGameOver = false;
let attempts = 0;
const maxAttempts = 3;
// Load the bird image
const birdImg = new Image();
birdImg.src = 'bird.png'; // Set your bird image file path here
function drawBird() {
ctx.drawImage(birdImg, bird.x - bird.radius, bird.y - bird.radius, bird.radius * 2, bird.radius * 2);
}
// Load the pipe image
const pipeImg = new Image();
pipeImg.src = 'pipe.png'; // Set your pipe image file path here
function drawPipes() {
for (let i = 0; i = canvas.height || bird.y <= 0) {
gameOver();
}
}
function updatePipes() {
if (pipes.length === 0 || pipes[pipes.length - 1].x < canvas.width - 200) {
pipes.push({
x: canvas.width,
topHeight: Math.random() * (canvas.height - pipeGap - 300) + 150
});
}
for (let i = 0; i < pipes.length; i++) {
pipes[i].x -= 2;
if (pipes[i].x + pipeWidth pipes[i].x &&
bird.x - bird.radius < pipes[i].x + pipeWidth &&
(bird.y - bird.radius pipes[i].topHeight + pipeGap)) {
gameOver();
}
if (pipes[i].x + pipeWidth = maxAttempts) {
ctx.font = '50px Arial';
ctx.fillStyle = '#000';
ctx.fillText('Game Over', canvas.width / 2 - 150, canvas.height / 2);
} else {
ctx.font = '30px Arial';
ctx.fillStyle = '#000';
ctx.fillText('Start Again', canvas.width / 2 - 150, canvas.height / 2);
}
// Add event listener for restart
document.addEventListener('keydown', restartListener);
}
function restartListener(e) {
if (e.key === ' ' && attempts = maxAttempts) return;
if (!isGameOver) {
ctx.clearRect(0, 0, canvas.width, canvas.height);
drawBird();
drawPipes();
updateBird();
updatePipes();
drawScore();
requestAnimationFrame(gameLoop);
} else {
drawInstructions();
}
}
document.addEventListener('keydown', function (e) {
if (e.key === ' ' && attempts < maxAttempts) {
if (isGameOver) {
restartGame();
} else {
bird.velocity = bird.lift;
}
}
});
function restartGame() {
pipes.length = 0;
bird.y = canvas.height / 2;
score = 0;
isGameOver = false;
gameLoop();
}
gameLoop();
Output
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …