Task Manager Using HTML , CSS , JavaScript
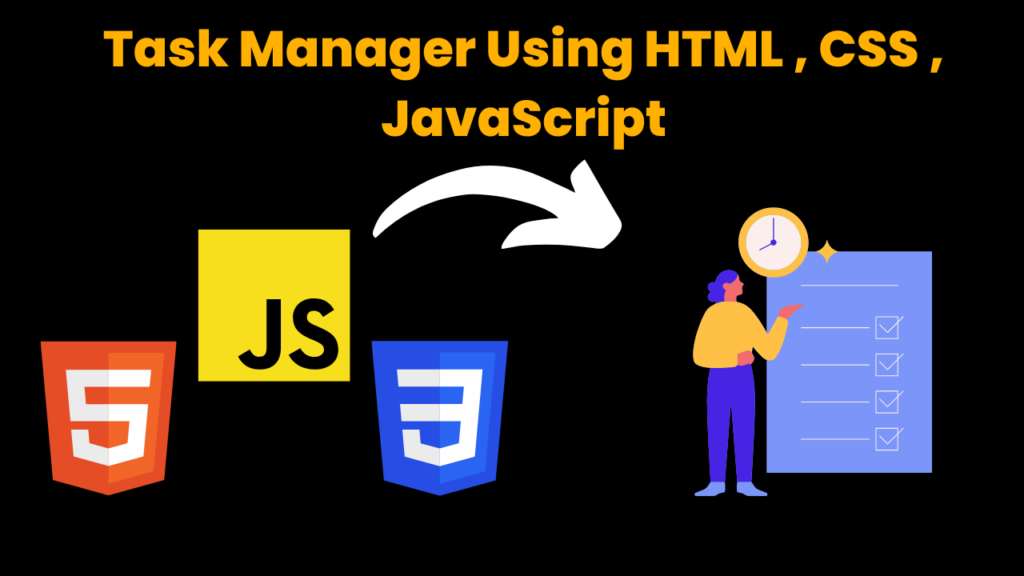
Introduction:
Project Introduction: The to-do list application is a simple and efficient tool that helps users manage their tasks effectively. It allows users to create, delete, and organize tasks based on their priorities and deadlines. This application aims to provide a user-friendly interface that can be accessed from any device, ensuring seamless task management across different platforms.
Key Features:
- Task Creation: Users can add tasks with a title, description, and due date.
- Task Organization: Tasks can be categorized into different lists or tags, allowing users to group related tasks together.
- Task Deletion: Users can easily delete tasks that are no longer needed.
- Task Priority: Users can assign priority levels to tasks to highlight their importance.
- Task Sorting: Users can sort tasks based on due date, priority, or alphabetical order to organize their to-do list efficiently.
- Task Search: Users can search for specific tasks using keywords or tags to quickly locate and manage them.
- Task Completion: Users can mark tasks as complete, providing a visual indication of progress.
- Responsive Design: The application is designed to be responsive and compatible with different devices and screen sizes.
Source Code:
HTML:
<--@codewithcurious.com-->-->
To-Do List Application
To-Do List
CSS (style.css):
/* @codewithcurious.com */
body {
font-family: 'Roboto', sans-serif;
background-color: #f2f2f2;
}
.container {
max-width: 600px;
margin: 0 auto;
padding: 20px;
background-color: #f9f9f9;
border-radius: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
color: #333;
margin-bottom: 20px;
}
.input-container {
display: flex;
margin-bottom: 20px;
}
input[type="text"] {
flex-grow: 1;
padding: 10px;
border-radius: 5px 0 0 5px;
border: none;
background-color: #fff;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
button {
padding: 10px 20px;
background-color: #ff5851;
color: #fff;
border: none;
border-radius: 0 5px 5px 0;
cursor: pointer;
transition: background-color 0.3s ease;
}
button:hover {
background-color: #ff403a;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: flex;
align-items: center;
padding: 10px;
margin-bottom: 10px;
background-color: #fff;
border-radius: 5px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
li:hover {
background-color: #f2f2f2;
}
li span {
flex-grow: 1;
margin-right: 10px;
}
li button {
background-color: transparent;
border: none;
color: #888;
cursor: pointer;
transition: color 0.3s ease;
}
li button:hover {
color: #333;
}
JavaScript (script.js):
// @codewithcurious.com/
function addTask() {
var input = document.getElementById("taskInput");
var task = input.value;
input.value = "";
if (task === "") {
alert("Please enter a task!");
return;
}
var taskList = document.getElementById("taskList");
var li = document.createElement("li");
li.appendChild(document.createTextNode(task));
taskList.appendChild(li);
}
Output:
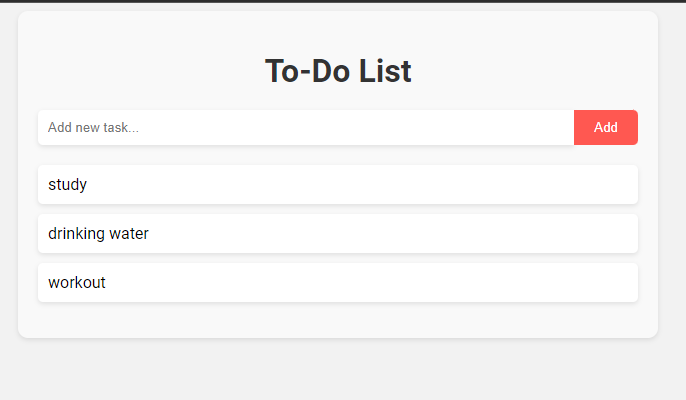
Find More Projects
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …