Drum Kit using HTML, CSS and JavaScript
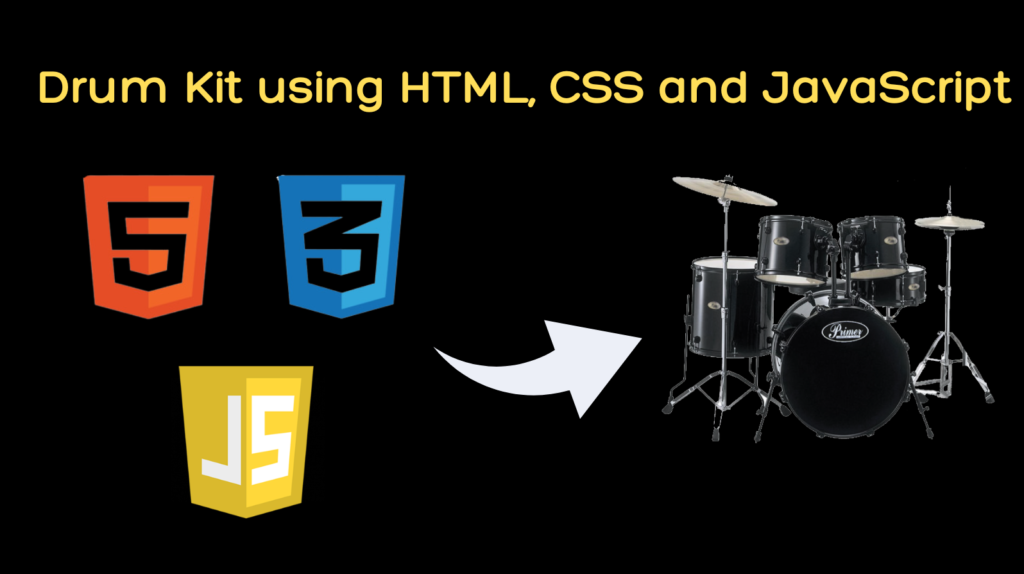
Introduction :
This project is a simple drum kit created using HTML, CSS, and JavaScript. It allows users to play different drum sounds by pressing corresponding keys on the keyboard. Each key is associated with a specific drum sound, and when a key is pressed, the associated sound is played along with a visual animation on the screen. The purpose of this project is to create an interactive and dynamic digital drum kit that allows users to engage with a virtual percussion instrument using their computer keyboard. By associating each key on the keyboard with a specific drum sound, users can experience the joy of creating rhythmic patterns and beats in a user-friendly and entertaining manner. The drum kit is designed with accessibility in mind, utilizing keyboard input, a familiar and widely accessible input method. This makes the application user-friendly, allowing individuals with varying levels of technological proficiency to engage with and enjoy the virtual drumming experience. Beyond its entertainment value, this drum kit project also serves as an educational tool for those learning web development. It demonstrates the integration of HTML, CSS, and JavaScript in a real-world context, showcasing how these technologies can be harmoniously combined to create an engaging and interactive web application.
Explanation :
HTML Structure :
- The HTML file defines a basic structure with a head containing meta tags and links to external stylesheets and favicon.
- The body contains a
<div>
with the class “keys,” which contains individual keys for each drum sound, represented by<div>
elements with the class “key.” - Each key has a data-key attribute representing the corresponding key code, a
<kbd>
element for displaying the associated keyboard key, and a<span>
element for displaying the drum sound name. - Following the keys, there are
<audio>
elements for each sound with a data-key attribute and a source pointing to the respective sound file. - The script.js file is linked at the end of the body.
Styling using CSS :
- The external stylesheet (style.css) likely contains styles for the keys and their animations. Unfortunately, the CSS code is not provided, so specific details about the styling cannot be covered here.
JavaScript Logic :
removeTransition
(e)
Function :
- his function is called when a CSS transition on a key element ends.
- It checks if the ended transition property is “transform” (ensuring it is related to the animation).
- If true, it removes the “playing” class from the key element, which stops the visual animation.
playSound(e)
Function:
- This function is called when a key is pressed (
keydown
event). - It retrieves the
<audio>
element associated with the pressed key using thee.keyCode
attribute. - It retrieves the
<div>
(key) associated with the pressed key using the same attribute. - If there is no audio element for the pressed key, the function exits.
- It adds the “playing” class to the key, triggering a visual animation.
- It sets the audio’s current time to 0 to allow rapid consecutive playbacks.
- It plays the associated audio.
Event Listeners:
- The script converts the NodeList of keys (selected using
document.querySelectorAll(".key")
) to an array usingArray.from()
. - For each key, an event listener for the
transitionend
event is added, calling theremoveTransition
function. - A global event listener for the
keydown
event is added to the window, triggering theplaySound
function when a key is pressed.
SOURCE CODE :
HTML (index.html)
Piano-Project
A
clap
S
hihat
D
kick
F
openhat
G
boom
H
ride
j
snare
k
tom
l
tink
CSS (style.css)
html {
font-size: 10px;
background-image: url("bg.png");
background-size: cover;
}
body,
html {
margin: 0;
padding: 0;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
.keys {
display: flex;
flex-grow: 1;
min-height: 100vh;
align-items: center;
justify-content: center;
}
.key {
border: 0.4rem solid rgb(177, 177, 177);
border-radius: 0.5rem;
margin: 1rem;
font-size: 1.5rem;
padding: 1rem 0.5rem;
transition: all 0.07s ease;
width: 10rem;
text-align: center;
background: rgba(0, 0, 0, 0.4);
color: white;
/* text-shadow: 0 0 0.5rem black; */
}
.playing {
transform: scale(1.1);
border-color: #ffc600;
box-shadow: 0 0 1rem #ffc600;
}
kbd {
display: block;
font-size: 4rem;
}
.sound {
font-size: 1.2rem;
text-transform: uppercase;
letter-spacing: 1px;
color: #d6c588;
font-weight: bold;
}
JavaScript (script.js)
function removeTransition(e){
if(e.propertyName!=="transform") return;
e.target.classList.remove("playing")
}
function playSound(e){
const audio = document.querySelector(`audio[data-key="${e.keyCode}"]`)
const key = document.querySelector(`div[data-key="${e.keyCode}"]`)
if(!audio) return;
key.classList.add("playing");
audio.currentTime=0;
audio.play();
}
const keys= Array.from(document.querySelectorAll(".key"));
keys.forEach(key=>
key.addEventListener("transitionend",removeTransition));
window.addEventListener("keydown",playSound);
Purpose of functions :
removeTransition(e): Ensures that the “playing” class is removed only when the “transform” transition ends. This avoids interference with other transitions that might be applied to the keys.
playSound(e): Handles the logic for playing the associated audio when a key is pressed. Adds a visual cue by applying the “playing” class to the key element, triggering a CSS animation.
OUTPUT :
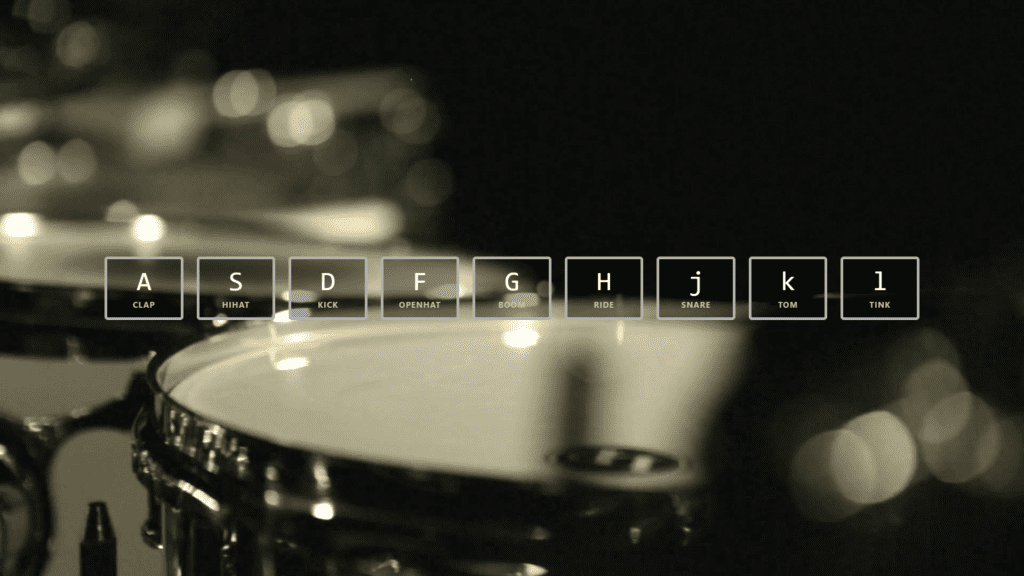
Conclusion :
This drum kit project combines HTML for structure, CSS for styling and animations (not provided), and JavaScript for handling key events and playing sounds. The code is concise and modular, making it easy to understand and maintain. Users can enjoy creating drum beats by interacting with the keyboard keys.
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …