Creating Pong Game Using HTML CSS Javascript
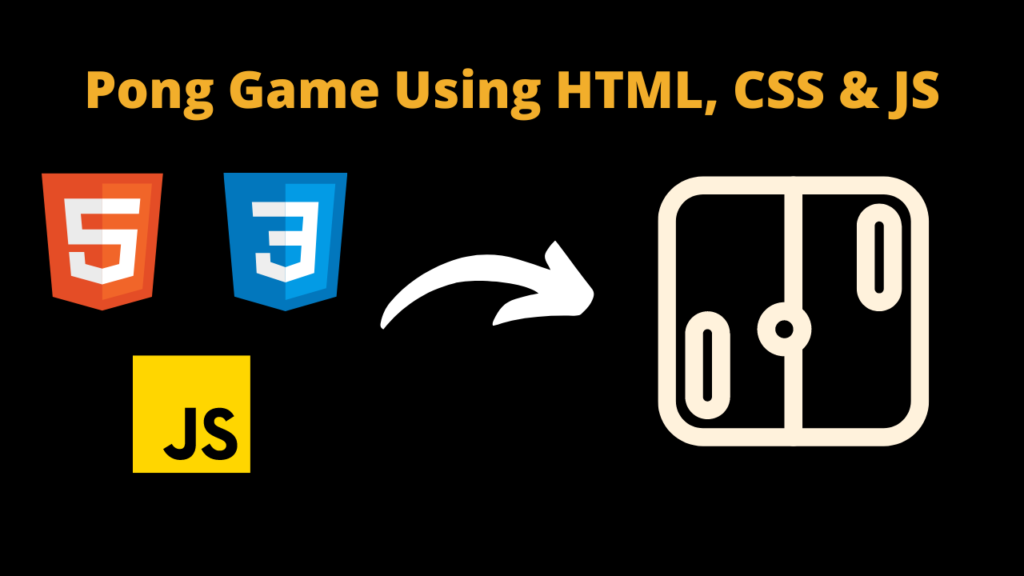
Introduction:
Welcome to the world of game development! Have you ever wanted to create your own video game? Well, you’re in luck because today we’re going to learn how to build a classic game called Pong using three essential web technologies: HTML, CSS, and JavaScript.
Pong is a simple but fun game where two players control paddles on opposite sides of the screen. The goal is to hit a ball back and forth, trying to make the other player miss it. It’s like virtual ping-pong!
In this tutorial, we’ll guide you through the process of creating your own Pong game from scratch. Don’t worry if you’re new to programming or game development – we’ll explain everything step by step in a simple and easy-to-understand way.
By the end of this tutorial, you’ll have your very own playable Pong game that you can share with your friends or even customize to make it your own. So let’s get started and have some fun building our own Pong game together!
Code Explanation:
Let’s break down the provided code:
HTML Structure:
- The HTML file starts with the usual
<!DOCTYPE html>
declaration and<html>
,<head>
, and<body>
tags. - Within the
<head>
, there’s a<style>
block defining the CSS styles for the canvas element.
Canvas Setup:
- A canvas element is created with the id “pongCanvas” and dimensions 800×400 pixels.
JavaScript:
- The JavaScript begins with obtaining a reference to the canvas and its context.
- It defines constants and variables for paddle dimensions, paddle positions, ball dimensions, ball positions, and ball speeds.
Drawing Functions:
- Two drawing functions are defined:
drawRect
: To draw rectangles (used for paddles and clearing the canvas).drawCircle
: To draw circles (used for the ball).
- These functions set the fill style and draw shapes based on given parameters.
Drawing Function:
- The
draw()
function is responsible for drawing the game elements on the canvas:- Clears the canvas.
- Draws the paddles and ball.
Update Function:
- The
update()
function is responsible for updating the game state:- Moves the ball.
- Handles collisions with walls and paddles.
- Resets the ball if it goes out of bounds.
Reset Ball Function:
- The
resetBall()
function resets the ball position to the center of the canvas and changes its direction.
Mouse Move Handler:
- An event listener is added to the canvas for the ‘mousemove’ event.
- It updates the position of the player’s paddle based on the mouse movement.
Calculate Mouse Position:
- The
calculateMousePos()
function calculates the position of the mouse relative to the canvas.
Game Loop:
- The
gameLoop()
function runs continuously usingrequestAnimationFrame()
:- Draws the game.
- Updates the game state.
- Requests the next animation frame.
Start Game:
- The game loop is started by calling
gameLoop()
.
How to Run Below Code:
Source Code:
Pong Game
Output:
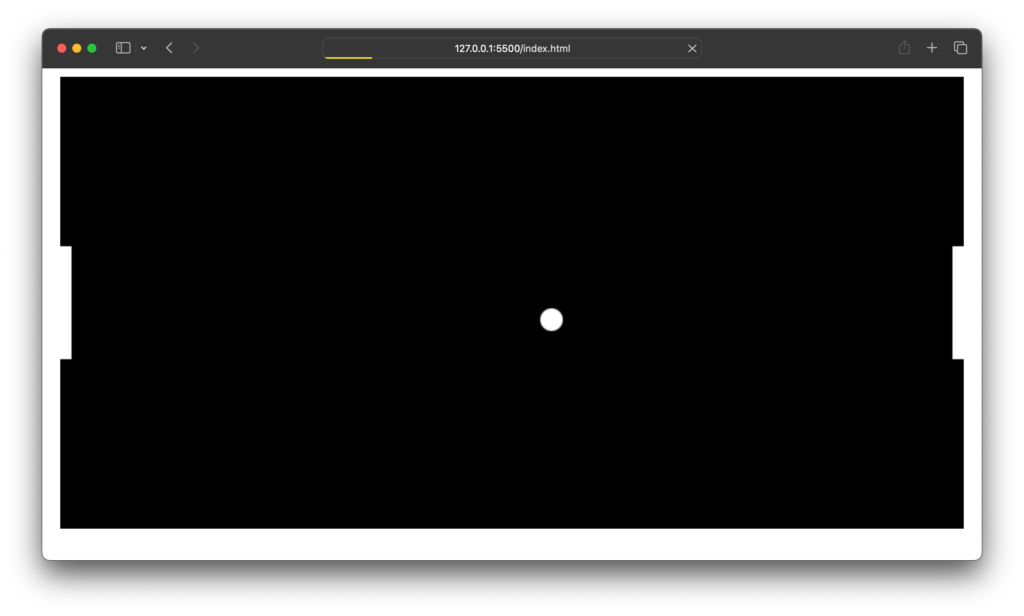
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …