Facebook Clone Using HTML, CSS and JS
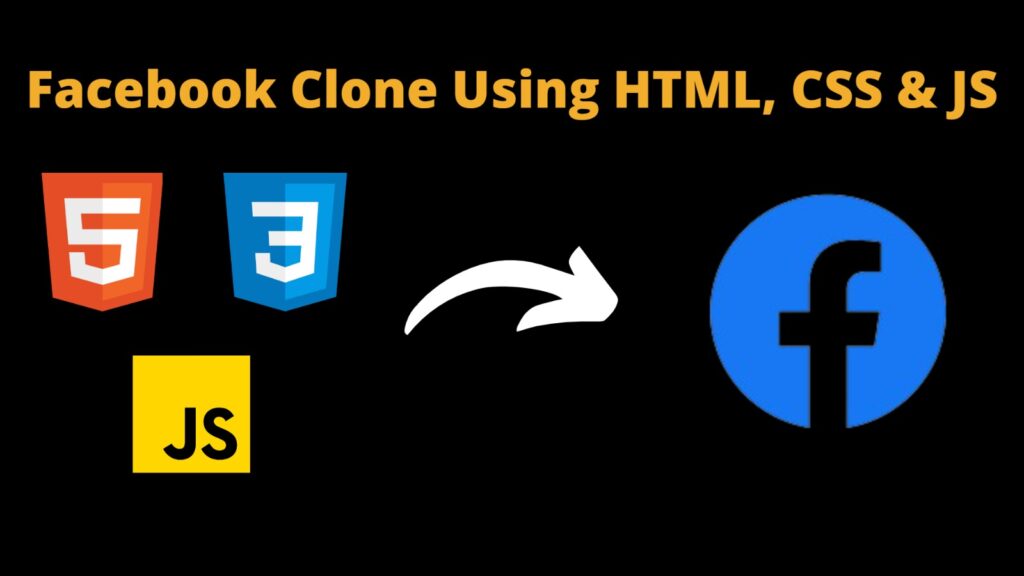
Introduction
In this project, we have recreated the essence of Facebook in a dynamic and engaging clone using the powerful combination of HTML, CSS, JavaScript, and Bootstrap. Our goal was to develop a platform that captures the essence of social networking, providing users with a familiar yet enhanced experience that keeps them connected and engaged.
HTML forms the backbone of our Facebook clone, laying the foundation for a seamless user interface that prioritizes simplicity and accessibility. With carefully crafted markup, we’ve created a user-friendly environment that facilitates easy navigation and interaction, allowing users to explore and engage with content effortlessly.
CSS, with the assistance of Bootstrap, elevates the visual appeal of our platform to new heights. From sleek designs to responsive layouts, CSS ensures that our Facebook clone looks stunning across all devices, captivating users with its modern and polished appearance.
By leveraging Bootstrap for both CSS and JavaScript, we’ve been able to harness its extensive library of components and utilities to streamline development and enhance functionality. From responsive grids to interactive modals, Bootstrap has played a vital role in shaping our Facebook clone into a polished and professional product.
Steps to create Facebook Clone
Planning and Research:
- Analyze the Facebook interface to understand its layout, components, and functionality.
- Identify the key features such as the news feed, user profile, navigation bar, etc.
Set Up Your Project:
- Create a new directory for your project.
- Set up your HTML file (
index.html
) and CSS file (style.css
). - Link Bootstrap CDN or download Bootstrap and link it to your HTML file.
Design the Layout:
- Create the basic structure of your HTML file.
- Use Bootstrap’s grid system to create a responsive layout.
- Design the header/navbar, sidebar, main content area, and footer using Bootstrap components and classes.
Header/Navbar:
- Use Bootstrap’s Navbar component for the header.
- Customize the Navbar to include the Facebook logo, search bar, user profile picture, and navigation links.
Sidebar:
- Design the sidebar containing user information, friend requests, and other relevant widgets.
- Utilize Bootstrap’s card components or list groups for styling.
Main Content Area:
- Create the news feed section where posts from friends appear.
- Use Bootstrap cards or panels to display each post.
- Include options for liking, commenting, and sharing posts.
User Profile Section:
- Design the user profile section displaying profile picture, cover photo, and user information.
- Utilize Bootstrap’s grid system for organizing profile information.
Styling with CSS:
- Add custom styles using CSS to enhance the appearance of your Facebook clone.
- Customize colors, fonts, and spacing to match the Facebook theme.
- Use media queries for responsive design to ensure your clone looks good on different devices.
Testing and Debugging:
- Test your Facebook clone on various devices and browsers to ensure compatibility.
- Debug any layout or functionality issues.
Explanation
HTML Structure Breakdown with Bootstrap Integration (index.html)
- Document Declaration:The HTML code begins with a declaration (<!DOCTYPE html>), indicating the document type as HTML5, and specifies the language as English.This sets the standard for the browser to interpret the following code accurately.
- Head Section:The <head> section contains crucial meta-information and external resource links necessary for the webpage. It includes links to external CSS files, JavaScript libraries, and meta tags for SEO optimization and viewport configuration.
- Bootstrap Introduction: Bootstrap is a robust front-end framework used for building responsive and mobile-friendly websites. It offers a comprehensive suite of pre-designed HTML, CSS, and JavaScript components that expedite web development and ensure consistent design across various devices and screen sizes. In this code snippet, Bootstrap is seamlessly integrated to leverage its styling classes and grid system for structuring the layout and enhancing the visual aesthetics of the Facebook clone.
- Topbar Section: The topbar segment encompasses elements like the Facebook logo, search bar, and user navigation links. Bootstrap classes such as input-group, form-control, and input-group-addon are applied to style and align the search bar seamlessly within the layout.
- Left Content Area: This section presents global links, shortcuts, explore options, and create links for user engagement. Leveraging Bootstrap’s responsive grid system (col-md-4, col-md-8), the content is arranged in a visually appealing and organized manner across different viewport sizes.
- Feed Content: Here, recent posts and user activities are displayed. Bootstrap’s navigation tabs are utilized to categorize and structure post headers and footers, offering users a familiar and intuitive browsing experience.
- Third Column Content: The third column showcases user-related pages, event invitations, trending topics, and sponsored content. By employing Bootstrap’s styling components, the content is presented uniformly and responsively, ensuring optimal display regardless of the device or screen resolution.
- Right Content Area: This segment showcases users who have interacted with the current user’s posts, along with links to user profiles and contacts. Bootstrap styling ensures that the content is visually appealing and well-organized, contributing to an enhanced user experience.
- Script Integration: External JavaScript libraries, including Bootstrap and custom scripts (script.js), are integrated to augment the webpage’s functionality and interactivity. These scripts enhance user engagement and provide dynamic features to the Facebook clone.
- Image Usage: Various images, such as logos (profile.png, fb.png), profile pictures, and post images, are incorporated to enrich the visual presentation of the Facebook clone. These images contribute to the overall aesthetic appeal and user engagement of the webpage.
In summary, the seamless integration of Bootstrap within the HTML code enables the creation of a visually appealing, responsive, and user-friendly Facebook clone. Bootstrap’s extensive toolkit empowers developers to expedite the development process while ensuring consistent design standards and optimal user experience across diverse platforms and devices.
Bootstrap Classes Utilized in the Facebook Clone
Topbar Section:
- navbar: Applied to create a navigation bar structure.
- navbar-brand: Used for the Facebook logo to establish brand identity.
- navbar-form: Employed for creating a form within the navigation bar.
- form-group: Wraps form elements to apply styling and spacing.
- form-control: Styles form inputs like text fields and buttons.
- input-group: Utilized for grouping form elements together.
- input-group-addon: Adds an element before or after the form input, such as an icon or text.
Left Content Area:
- col-md-4 and col-md-8: Utilized for creating a responsive grid layout, where content occupies different proportions of the available space based on the screen size. Here, it divides the content into two columns on medium-sized screens.
- list-group and list-group-item: Employed for creating a list group with items, used for global links and shortcuts.
- nav and nav-tabs: Applied for creating navigation tabs to categorize and organize content.
Feed Content:
- nav-tabs: Used for the navigation tabs to categorize and structure post headers and footers.
- media and media-body: Employed for displaying media content such as posts, comments, and user activities.
Third Column Content:
- panel and panel-default: Utilized for creating a panel with default styling for user-related pages, event invitations, trending topics, and sponsored content.
- panel-heading and panel-body: Applied for structuring the panel’s header and body content.
Right Content Area:
- thumbnail: Used for displaying images with a border and padding.
- caption: Employed for adding a caption to the thumbnail image.
- list-group and list-group-item: Utilized for creating a list group with items, used for displaying users who have interacted with the current user’s posts, along with links to user profiles and contacts.
Script Integration:
- bootstrap.min.css and bootstrap.min.js: External CSS and JavaScript files provided by Bootstrap, integrated to apply Bootstrap’s styling and functionality to the webpage.
- script.js : Custom JavaScript file for additional functionality and interactivity.
Significance of Bootstrap Classes
- Responsiveness: Classes like
col-md-*
ensure that content adapts and displays appropriately across various screen sizes, enhancing the website’s responsiveness. - Consistent Styling: Bootstrap classes offer predefined styling, ensuring a consistent look and feel throughout the webpage without the need for custom CSS.
- Component Reusability: Components like navigation bars (
navbar
), forms (form-control
), and panels (panel
) streamline development by providing reusable building blocks for common UI elements. - Grid System: The grid classes facilitate the creation of complex layouts by dividing content into responsive columns, enabling developers to design flexible and visually appealing interfaces.
- Interactivity: Bootstrap’s JavaScript components and plugins enhance user interaction, providing features like dropdowns, modals, and tooltips to enrich the user experience.
By leveraging these Bootstrap classes effectively, developers can expedite the development process, ensure design consistency, and create a visually appealing and user-friendly Facebook clone that performs optimally across diverse devices and screen sizes.
CSS Styling
Global Styles:
- The
body
selector sets the background color of the entire webpage to a light grayish-blue (#e9ebee). - Textareas and form controls have their borders removed, border radius set to 0, and resizing disabled.
Topbar Styles:
- The
.topbar
class styles the top navigation bar to be fixed at the top of the viewport with a blue background and a dark blue bottom border. - The right group within the topbar is floated to the right, containing link groups and notification groups.
- Links within the topbar have white text, padding, and a font size of 12 pixels. On hover, the background color changes to a darker blue (#355486) and text decoration is removed.
- Link groups within the right group are displayed as inline-block elements with no padding.
- Image icons within links are displayed inline-block with a specific width and height.
Left Content and Feed Content:
- The left content section is fixed on the left side of the viewport with a specific width, background color, and overflow set to auto.
- Global links within the left content have specific styling for text and icons, along with a counter for notifications.
- The feed content section is positioned to the right of the left content with padding, background color, and overflow set to auto.
Third Column Content:
- The third column content is fixed on the right side of the viewport with a specific width, background color, and overflow set to auto.
- It contains various sections with borders, headings, and links.
Media Queries:
- Media queries are used to adjust the layout and styling based on the screen width.
- When the screen width is less than 700px, the left content is hidden and certain link groups in the topbar are hidden.
- When the screen width is less than 1000px, the third column content is hidden.
- When the screen width is greater than or equal to 1260px, additional content is displayed on the right side.
Technical Buzzwords:
- Selectors:
body
,.topbar
,.right-group
,.link-group
,a
,img
,textarea
,.form-control
. - Properties:
position
,background-color
,color
,font-size
,padding
,margin
,border
,width
,height
,overflow
,float
,display
,transition
,outline
,text-align
,list-style
,border-radius
. - Values: Colors in hexadecimal format (#e9ebee), lengths in pixels (px), positions (fixed, relative), and various keywords (none, inline-block, auto).
script.js
Bootstrap Library: The first
<script>
tag loads the Bootstrap library from a Content Delivery Network (CDN) hosted at//cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.0.0-alpha.6/js/bootstrap.min.js
. This library provides pre-designed components and styles for building responsive web pages.Custom Script: The second
<script>
tag loads a custom JavaScript file namedscript.js
from the current directory (./
). This is where you would typically place your own JavaScript code to add interactivity or functionality to your webpage.Anonymous Function: The JavaScript code inside
script.js
is wrapped in an immediately invoked function expression (IIFE) using(function() { ... })();
. This encapsulates the code within its own scope, preventing it from polluting the global namespace and avoiding conflicts with other scripts.Function Invocation: The IIFE is immediately invoked using
.call(this)
to execute its code as soon as the script is loaded. This ensures that any initialization or setup logic inside the function is executed promptly.Execution Context: By using
.call(this)
, the current execution context (this
) is passed into the IIFE. This allows the code inside the function to access properties and methods of the current context, which could be the globalwindow
object or another context depending on where the script is loaded.
In summary, this HTML setup loads the Bootstrap library for styling and functionality, along with a custom JavaScript file (script.js
) containing code wrapped in an IIFE for encapsulation and immediate execution. This structure ensures that your webpage has access to Bootstrap features and your custom JavaScript logic as soon as it loads.
Source Code
HTML (index.html)
Facebook Design Clone
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Cupiditate sunt quasi in quam asperiores! Optio voluptate impedit eos ex nisi, molestias facilis sint cupiditate, dolores veritatis cum? Enim vel, qui!
We lose 48 football fields of forest every minute. :-(
By using "Ecosia.org" for your web searches you can help stop this trend.
Let's plant some trees together!
Trending
-
Alex Honnold
'Free solo' climber conquers El Capitan without rope, safety gear ‑ msn.com
-
Michael Bloomberg
Michael Bloomberg Pledges $15 Million For Paris Climate Pact ‑ huffingtonpost.com
-
Theresa May
UK PM May's lead cut to just 1 point over Labour - Survation poll ‑ reuters.com
Sponsored
Create Ad
-
First Name likes First Name's post
-
First Name likes First Name's post
-
First Name likes First Name's post
-
First Name likes First Name's post
-
First Name likes First Name's post
-
First Name likes First Name's post
Your Pages
-
Page Name 1
-
Page Name
-
Page Name 2
Contacts
-
First Name
-
First Name
-
First Name
-
First Name
-
First Name
CSS (style.css)
body {
background-color: #e9ebee;
}
textarea,
.form-control {
border: 0;
border-radius: 0;
resize: none;
}
.topbar {
position: fixed;
top: 0;
left: 0;
right: 0;
z-index: 1;
padding: 6px 26px;
height: 42px;
background: #3b5e95;
border-bottom: 1px solid #294c7b;
overflow: hidden;
}
.topbar .right-group {
float: right;
}
.topbar .right-group .link-group {
position: relative;
top: -4px;
display: inline-block;
padding: 0;
}
.topbar .right-group .link-group a {
padding: 9px 8px;
color: #fff;
font-size: 12px;
}
.topbar .right-group .link-group a img {
display: inline-block;
width: 24px;
height: 24px;
margin-right: 9px;
}
.topbar .right-group .link-group a:hover {
background: #355486;
text-decoration: none;
}
.topbar .right-group .link-group:nth-child(2) a {
margin-right: 16px;
border-left: 1px solid #355485;
}
.topbar .right-group .notification-group {
display: inline-block;
position: relative;
top: 3px;
}
.topbar .right-group .notification-group .link-group a {
margin: 0;
border: 0;
}
.topbar .right-group .notification-group a {
color: #17253c;
font-size: 21px;
}
.topbar .right-group .notification-group a:hover {
color: #111;
background: transparent;
}
.topbar .right-group .link-group:nth-child(4) a {
margin-left: 16px;
border-left: 1px solid #355485;
}
.topbar .search-box {
display: inline-block;
width: calc(100vw - 72%);
}
.topbar .search-box .input-group * {
font-size: 12px;
height: 25px;
border: 1px solid #fff;
background: #fff;
outline: 0;
}
.topbar .search-box .input-group button {
color: #7a7a7a;
transition: all ease 150ms;
}
.topbar .search-box .input-group button:hover {
color: #000;
}
.left-content {
left: 0;
}
.left-content {
position: fixed;
top: 42px;
bottom: 0;
width: 250px;
background: #e9ebee;
overflow: auto;
}
.left-content .global-links {
padding: 10px;
margin-left: 10px;
}
.left-content .global-links a {
display: inline-block;
color: #000;
font-size: 12px;
width: 100%;
padding: 2px 8px;
}
.left-content .global-links a img {
width: 20px;
height: 20px;
margin-right: 6px;
}
.left-content .global-links .counter {
float: right;
color: #90949c;
}
.left-content .global-links .counter .fa-ellipsis-h {
font-size: 20px;
}
.left-content .global-links .activepage {
padding-top: 5px;
background: #fff;
border-radius: 2px;
border: 1px solid #dddfe2;
}
.left-content .group-content h4 {
margin: 20px 0 8px 0;
font-size: 13px;
color: #444;
text-transform: uppercase;
}
.left-content a:last-child .fa-caret-down {
margin-right: 12px;
}
.feed-content {
position: absolute;
top: 42px;
bottom: 0;
left: 250px;
right: 0;
padding: 12px 7px;
background: #e9ebee;
overflow: auto;
}
.feed-content .recentcontainer {
border-radius: 2px;
border: 1px solid #dddfe2;
margin-bottom: 15px;
overflow: hidden;
}
.feed-content .recentcontainer .newpostheader,
.feed-content .recentcontainer .newpostfooter {
padding: 4px 5px;
background: #f6f7f9;
}
.feed-content .recentcontainer .newpostheader li,
.feed-content .recentcontainer .newpostfooter li {
display: inline-block;
width: 100%;
text-align: center;
}
.feed-content .recentcontainer .newpostheader li:first-child,
.feed-content .recentcontainer .newpostfooter li:first-child {
float: left;
}
.feed-content .recentcontainer .newpostheader li:last-child,
.feed-content .recentcontainer .newpostfooter li:last-child {
float: rght;
}
.feed-content .recentcontainer .newpostheader h4,
.feed-content .recentcontainer .newpostfooter h4 {
font-size: 14px;
margin: 10px 5px;
color: #666;
}
.feed-content .recentcontainer .newpostheader a:first-child,
.feed-content .recentcontainer .newpostfooter a:first-child {
margin-right: 5px;
}
.feed-content .recentcontainer .newpostheader a,
.feed-content .recentcontainer .newpostfooter a {
font-size: 14px;
}
.feed-content .recentcontainer .newpostheader a img,
.feed-content .recentcontainer .newpostfooter a img {
width: 40px;
height: 40px;
margin: 5px 5px 0 5px;
}
.feed-content .recentcontainer .newpostheader a .name,
.feed-content .recentcontainer .newpostfooter a .name {
position: relative;
top: -6px;
}
.feed-content .recentcontainer .newpostheader a .fa,
.feed-content .recentcontainer .newpostfooter a .fa {
display: inline-block;
margin: 5px;
}
.feed-content .recentcontainer .newpostheader > span,
.feed-content .recentcontainer .newpostfooter > span {
position: relative;
top: -6px;
left: -4px;
font-size: 14px;
}
.feed-content .recentcontainer .newpostheader p,
.feed-content .recentcontainer .newpostfooter p {
position: relative;
top: -22px;
left: 55px;
font-size: 12px;
}
.feed-content .recentcontainer .newpostheader p a,
.feed-content .recentcontainer .newpostfooter p a {
font-size: 12px;
color: #4b4f56;
margin-right: 5px;
}
.feed-content .recentcontainer .newpostheader .rightsideofpost,
.feed-content .recentcontainer .newpostfooter .rightsideofpost {
position: relative;
top: -73px;
float: right;
}
.feed-content .recentcontainer .newpostheader .rightsideofpost .personpostmenu,
.feed-content .recentcontainer .newpostfooter .rightsideofpost .personpostmenu {
color: #4b4f56;
}
.feed-content .recentcontainer .newpostheader li a,
.feed-content .recentcontainer .newpostfooter li a {
font-size: 14px;
color: #4b4f56;
margin-right: 5px;
}
.feed-content .recentcontainer .newpostheader li a .fa,
.feed-content .recentcontainer .newpostfooter li a .fa {
display: inline-block;
margin: 5px;
}
.feed-content .recentcontainer .community-counter {
padding: 4px 5px;
background: #f6f7f9;
}
.feed-content .recentcontainer .community-counter li a {
font-size: 14px;
color: #4b4f56;
margin-right: 5px;
}
.feed-content .recentcontainer .community-counter li a .fa {
display: inline-block;
margin: 5px;
}
.feed-content .recentcontainer .newpost textarea,
.feed-content .recentcontainer .newpost .postcontent {
display: inherit;
padding: 10px;
width: 100%;
height: 100%;
background: #f6f7f9;
}
.feed-content .recentcontainer .newpost .postcontent {
position: relative;
margin-top: -40px;
padding-bottom: 40px;
white-space: pre-line;
}
.feed-content .recentcontainer .newpostfooter {
margin-top: 1px;
}
.feed-content .recentcontainer .commentpost {
display: inherit;
background: #f6f7f9;
margin-top: -1px;
}
.feed-content .recentcontainer .commentpost img {
margin: 10px;
height: 40px;
}
.feed-content .recentcontainer .commentpost .input-group-btn {
display: inline-block;
background: #fff;
}
.feed-content .recentcontainer .commentpost .input-group-btn a {
margin-top: 10px;
}
.feed-content .recentcontainer:nth-child(1) .newpostfooter li:first-child .fa {
color: #96b756;
}
.feed-content .recentcontainer:nth-child(1) .newpostfooter li:nth-child(2) .fa {
color: #f1c04e;
}
.feed-content .recentcontainer:nth-child(1) .newpostfooter li:last-child a:last-child {
font-size: 20px;
}
.thirdcol-content {
position: fixed;
top: 42px;
bottom: 0;
width: 250px;
background: #e9ebee;
right: 0;
display: block;
width: 300px;
padding: 12px 7px;
background: #e9ebee;
overflow-x: auto;
}
.thirdcol-content > div > a,
.thirdcol-content > div > p {
font-size: 12px;
}
.thirdcol-content .section-content {
border-radius: 2px;
border: 1px solid #dddfe2;
margin: 0 0 15px 0;
overflow-y: hidden;
padding: 4px 5px;
background: #f6f7f9;
}
.thirdcol-content .section-content h4:nth-child(1) {
font-size: 14px;
margin: 6px;
padding: 6px;
color: #888;
}
.thirdcol-content .section-content h4:nth-child(1) a {
float: right;
color: #8a8a8a;
}
.thirdcol-content .section-content p a {
display: block;
}
.thirdcol-content .section-content p a span:not(:first-child) {
display: inline-block;
margin-left: 4px;
font-size: 9px;
padding: 1px 2px;
color: #545454;
background: #fff;
border: 1px solid #b8b8b8;
}
.thirdcol-content .section-content a {
font-size: 14px;
margin-right: 5px;
}
.thirdcol-content .section-content a img {
width: 64px;
height: 64px;
margin: 5px 5px 0 5px;
}
.thirdcol-content .section-content a .fa {
display: inline-block;
margin: 5px;
}
.thirdcol-content .section-content p:nth-child(3) {
text-align: right;
margin: -20px 0 4px 0;
padding: 2px;
width: 80%;
}
.thirdcol-content .section-content p:nth-child(3) a {
display: inline-block;
position: relative;
top: -18px;
}
.thirdcol-content .section-content p:nth-child(4) {
margin-top: -64px;
margin-left: 75px;
font-size: 12px;
}
.thirdcol-content .section-content p:nth-child(4) a:not(:nth-child(1)) {
font-size: 12px;
color: #4b4f56;
margin-right: 5px;
}
.thirdcol-content .section-content p:nth-child(7) {
text-align: center;
}
.thirdcol-content .section-content p:nth-child(7) a {
width: 20%;
display: inline-block;
color: #333;
}
.thirdcol-content .section-content p:nth-child(7) .fa {
display: block;
}
.thirdcol-content .section-content .activetrend,
.thirdcol-content .section-content .activetrend .fa {
color: #1273b8;
}
.thirdcol-content .section-content .trend-feed ul {
margin: 0 30px;
padding: 0;
list-style: square url("http://i.imgur.com/Etzny0O.png");
}
.thirdcol-content .section-content .trend-feed ul li {
font-size: 12px;
}
.thirdcol-content .section-content .trend-feed ul li span:nth-child(1) {
color: #999;
}
.thirdcol-content .section-content .trend-feed div a {
float: left;
font-size: 12px;
margin-top: 4px;
}
.thirdcol-content .section-content .trend-feed div a:first-child {
padding: 4px 0 0 4px;
}
.thirdcol-content .section-content .trend-feed div a:last-child {
float: right;
padding: 4px 0 0 4px;
}
.thirdcol-content .section-content:nth-child(1) > a:nth-child(2) {
float: right;
color: #8a8a8a;
}
.thirdcol-content .section-content:nth-child(4) {
font-size: 12px;
}
.thirdcol-content .section-content:nth-child(4) h4 {
float: left;
}
.thirdcol-content .section-content:nth-child(4) a:nth-child(2) {
margin-top: 10px;
float: right;
}
.thirdcol-content .section-content:nth-child(4) div {
padding: 5px;
}
.thirdcol-content .section-content:nth-child(4) div a {
display: block;
}
.thirdcol-content .section-content:nth-child(4) div a img {
width: 100%;
height: auto;
}
.thirdcol-content .section-content:nth-child(4) div a:hover {
text-decoration: none;
}
.thirdcol-content .section-content:nth-child(4) div div {
padding: 5px 5px 0 5px;
font-size: 12px;
}
.thirdcol-content .section-content:nth-child(4) div div div {
padding: 0;
}
.thirdcol-content .section-content:nth-child(4) div div:nth-child(3) {
color: #878787;
}
.thirdcol-content .section-content:nth-child(5) a {
font-size: 12px;
}
.feed-content {
right: 300px;
}
.right-content {
display: none;
position: fixed;
top: 42px;
bottom: 0;
width: 250px;
background: #e9ebee;
right: 0;
width: 275px;
padding: 12px 7px;
background: #e9ebee;
overflow-x: auto;
border-left: 1px solid #a3a3a3;
}
.right-content ul {
list-style: none;
padding: 0;
margin: 0;
height: 50%;
overflow: auto;
}
.right-content ul li {
display: block;
padding: 5px 5px 15px 5px;
width: 100%;
border-bottom: 1px solid #a3a3a3;
cursor: pointer;
}
.right-content ul:first-child {
list-style: none;
padding: 0;
height: 50%;
overflow: auto;
}
.right-content ul:first-child li {
display: block;
padding: 5px 5px 15px 5px;
width: 100%;
border-bottom: 1px solid #a3a3a3;
cursor: pointer;
}
.right-content ul:first-child li a {
padding: 5px;
font-size: 12px;
}
.right-content ul:first-child li img {
display: inline-block;
width: 32px;
height: 32px;
}
.right-content ul:first-child li b,
.right-content ul:first-child li span {
color: #000;
}
.right-content ul:first-child li span {
color: #545454;
}
.right-content ul:first-child li a:hover {
text-decoration: none;
}
.right-content ul:first-child li:hover {
background: #ccc;
}
.right-content ul:nth-child(2) {
list-style: none;
padding: 0;
height: 50%;
overflow: auto;
}
.right-content ul:nth-child(2) h4 {
font-size: 12px;
margin-top: 15px;
color: #474747;
text-transform: uppercase;
}
.right-content ul:nth-child(2) li {
display: block;
padding: 5px 5px 15px 5px;
width: 100%;
border-bottom: 1px solid #a3a3a3;
cursor: pointer;
}
.right-content ul:nth-child(2) li a {
padding: 5px;
font-size: 12px;
}
.right-content ul:nth-child(2) li img {
display: inline-block;
width: 32px;
height: 32px;
}
.right-content ul:nth-child(2) li b,
.right-content ul:nth-child(2) li span {
color: #000;
}
.right-content ul:nth-child(2) li span {
display: block;
float: right;
position: relative;
top: 8px;
font-size: 9px;
padding: 1px 2px;
color: #545454;
background: #fff;
border: 1px solid #b8b8b8;
}
.right-content ul:nth-child(2) li .fa {
float: right;
position: relative;
top: 8px;
color: #1f0;
}
.right-content ul:nth-child(2) li a:hover {
text-decoration: none;
}
.right-content ul:nth-child(2) li:hover {
background: #ccc;
}
@media all and (max-width: 700px) {
.left-content {
display: none;
}
.feed-content {
left: 0;
}
.topbar .right-group > .link-group {
display: none;
}
}
@media all and (max-width: 1000px) {
.thirdcol-content {
display: none;
}
.feed-content {
right: 0;
}
}
@media all and (min-width: 1260px) {
.feed-content {
right: 250px;
}
.right-content {
right: 0;
}
}
@media all and (min-width: 1260px) {
.feed-content {
right: 575px;
}
.thirdcol-content {
right: 275px;
}
.right-content {
display: block;
}
}
.pointer {
cursor: pointer;
}
.ib {
display: inline-block;
}
.fl {
float: left;
}
.fr {
float: right;
}
.tc {
text-align: center;
}
JS (script.js)
(function() {
}).call(this);
Output
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …