Online Library Management System
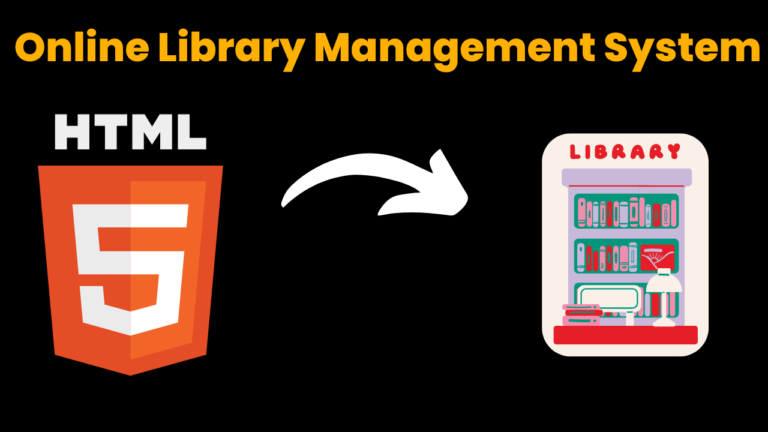
Introduction:
This Projects Online Library Management System introduces a System that is created by using the latest tech stacks i.e. HTML5, Bootstrap, and JavaScript(ES6)
One who has a basic understanding of JavaScript and familiar with JS Functions concepts can understand easily.
You can customize this project’s animation as well as logic as per requirement.
It’s one of the major projects you can use for your personal use as well as to present in your college.
This is about a Library which manages the records of the books its author and the type of book you want to store.
EXPLANATION:
For this project, we have used the VS Code editor.
And instead of CSS3 for styling we have used another technology that is Bootstrap.
Bootstrap is used for applying styling the user interface components.
To include Bootstrap add it from https://getbootstrap.com/docs/4.0/getting-started/introduction/.
Then include the following code in the body section, before the closing </body> tag:
Bootstrap also provides us with many components that can be used to provide a good user experience and user interactions on a web page like cards, navigation bars, dropdowns, icons, buttons, forms, and also sizing options for different DOM elements.
In this system, the user can store a book’s name its author as well as the type of book.
Now let us discuss the functions we have used in this project:
The first function we have used is function Book() this is the function that is used to take the name of the book, the name of the author, and the type of book you want to store.
After executing the code first of all this function is called.
The second function comes that is function Display() this function is created to display the books in the order the user has entered.
Here we have written a conditional code about if the user has typed only the book name and not mentioned its author or type of book then it shows the message
‘Sorry you cannot add this book’
And when the user enters all the details of the book its name, author, and its type, and then clicks the button to add the book is recorded successfully and it shows the message
‘Your book has been successfully added
Here we have also a feature for searching the book the one who enters the name in the search box can find the book only by its name, not by its author or its type.
We have only the logics we have discussed above.
There is no other hard part that one can’t understand.
Source Code:
index.html
Welcome to Library
Manage Library
Your books
Name
Author
Type
index.js
console.log("This is index.js");
// Todos"
// 1. Store all the data to the localStorage
// 2. Give another column as an option to delete the book
// 3. Add a scroll bar to the view
// Constructor
function Book(name, author, type) {
this.name = name;
this.author = author;
this.type = type;
}
// Display Constructor
function Display() {
}
// Add methods to display prototype
Display.prototype.add = function (book) {
console.log("Adding to UI");
tableBody = document.getElementById('tableBody');
let uiString = `
${book.name}
${book.author}
${book.type}
`;
tableBody.innerHTML += uiString;
}
// Implement the clear function
Display.prototype.clear = function () {
let libraryForm = document.getElementById('libraryForm');
libraryForm.reset();
}
// Implement the validate function
Display.prototype.validate = function (book) {
if (book.name.length < 2 || book.author.length < 2) {
return false
}
else {
return true;
}
}
Display.prototype.show = function (type, displayMessage) {
let message = document.getElementById('message');
message.innerHTML = `
Messge: ${displayMessage}
`;
setTimeout(function () {
message.innerHTML = ''
}, 2000);
}
// Add submit event listener to libraryForm
let libraryForm = document.getElementById('libraryForm');
libraryForm.addEventListener('submit', libraryFormSubmit);
function libraryFormSubmit(e) {
console.log('YOu have submitted library form');
let name = document.getElementById('bookName').value;
let author = document.getElementById('author').value;
let type;
let fiction = document.getElementById('fiction');
let programming = document.getElementById('programming');
let cooking = document.getElementById('cooking');
if (fiction.checked) {
type = fiction.value;
}
else if (programming.checked) {
type = programming.value;
}
else if (cooking.checked) {
type = cooking.value;
}
let book = new Book(name, author, type);
console.log(book);
let display = new Display();
if (display.validate(book)) {
display.add(book);
display.clear();
display.show('success', 'Your book has been successfully added')
}
else {
// Show error to the user
display.show('danger', 'Sorry you cannot add this book');
}
e.preventDefault();
}
index.js
console.log("This is index.js");
// Todos"
// 1. Store all the data to the localStorage
// 2. Give another column as an option to delete the book
// 3. Add a scroll bar to the view
// Constructor
function Book(name, author, type) {
this.name = name;
this.author = author;
this.type = type;
}
// Display Constructor
function Display() {
}
// Add methods to display prototype
Display.prototype.add = function (book) {
console.log("Adding to UI");
tableBody = document.getElementById('tableBody');
let uiString = `
${book.name}
${book.author}
${book.type}
`;
tableBody.innerHTML += uiString;
}
// Implement the clear function
Display.prototype.clear = function () {
let libraryForm = document.getElementById('libraryForm');
libraryForm.reset();
}
// Implement the validate function
Display.prototype.validate = function (book) {
if (book.name.length < 2 || book.author.length < 2) {
return false
}
else {
return true;
}
}
Display.prototype.show = function (type, displayMessage) {
let message = document.getElementById('message');
message.innerHTML = `
Messge: ${displayMessage}
`;
setTimeout(function () {
message.innerHTML = ''
}, 2000);
}
// Add submit event listener to libraryForm
let libraryForm = document.getElementById('libraryForm');
libraryForm.addEventListener('submit', libraryFormSubmit);
function libraryFormSubmit(e) {
console.log('YOu have submitted library form');
let name = document.getElementById('bookName').value;
let author = document.getElementById('author').value;
let type;
let fiction = document.getElementById('fiction');
let programming = document.getElementById('programming');
let cooking = document.getElementById('cooking');
if (fiction.checked) {
type = fiction.value;
}
else if (programming.checked) {
type = programming.value;
}
else if (cooking.checked) {
type = cooking.value;
}
let book = new Book(name, author, type);
console.log(book);
let display = new Display();
if (display.validate(book)) {
display.add(book);
display.clear();
display.show('success', 'Your book has been successfully added')
}
else {
// Show error to the user
display.show('danger', 'Sorry you cannot add this book');
}
e.preventDefault();
}
indexes6.js
console.log('This is ES6 version of Project 2');
class Book {
constructor(name, author, type) {
this.name = name;
this.author = author;
this.type = type;
}
}
class Display {
add(book) {
console.log("Adding to UI");
let tableBody = document.getElementById('tableBody');
let uiString = `
${book.name}
${book.author}
${book.type}
`;
tableBody.innerHTML += uiString;
}
clear() {
let libraryForm = document.getElementById('libraryForm');
libraryForm.reset();
}
validate(book) {
if (book.name.length < 2 || book.author.length < 2) {
return false
}
else {
return true;
}
}
show(type, displayMessage) {
let message = document.getElementById('message');
let boldText;
if(type==='success'){
boldText = 'Success';
}
else{
boldText = 'Error!';
}
message.innerHTML = `
${boldText}: ${displayMessage}
`;
setTimeout(function () {
message.innerHTML = ''
}, 5000);
}
}
// Add submit event listener to libraryForm
let libraryForm = document.getElementById('libraryForm');
libraryForm.addEventListener('submit', libraryFormSubmit);
function libraryFormSubmit(e) {
console.log('YOu have submitted library form');
let name = document.getElementById('bookName').value;
let author = document.getElementById('author').value;
let type;
let fiction = document.getElementById('fiction');
let programming = document.getElementById('programming');
let cooking = document.getElementById('cooking');
if (fiction.checked) {
type = fiction.value;
}
else if (programming.checked) {
type = programming.value;
}
else if (cooking.checked) {
type = cooking.value;
}
let book = new Book(name, author, type);
console.log(book);
let display = new Display();
if (display.validate(book)) {
display.add(book);
display.clear();
display.show('success', 'Your book has been successfully added')
}
else {
// Show error to the user
display.show('danger', 'Sorry you cannot add this book');
}
e.preventDefault();
}
tut34.js
console.log("This is tutorial 34");
setTimeout(() => {
for (let index = 0; index < 4005; index++) {
const element = index;
console.log("This is index number" + index);
}
}, 100);
console.log('done printing');
Output:
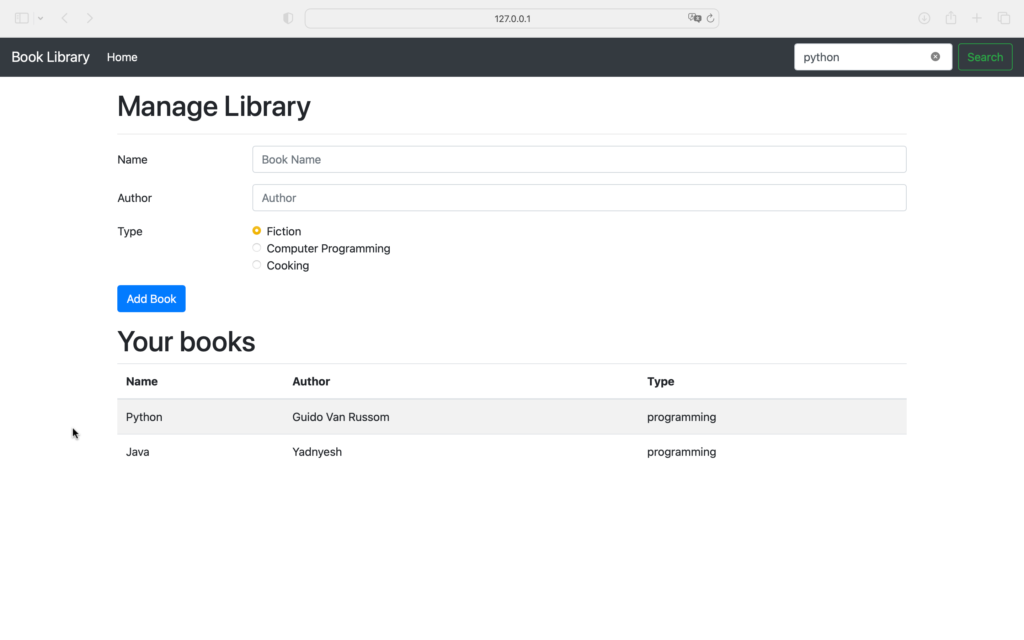
Find More Projects
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …