QR Code Generator using HTML, CSS and JavaScript
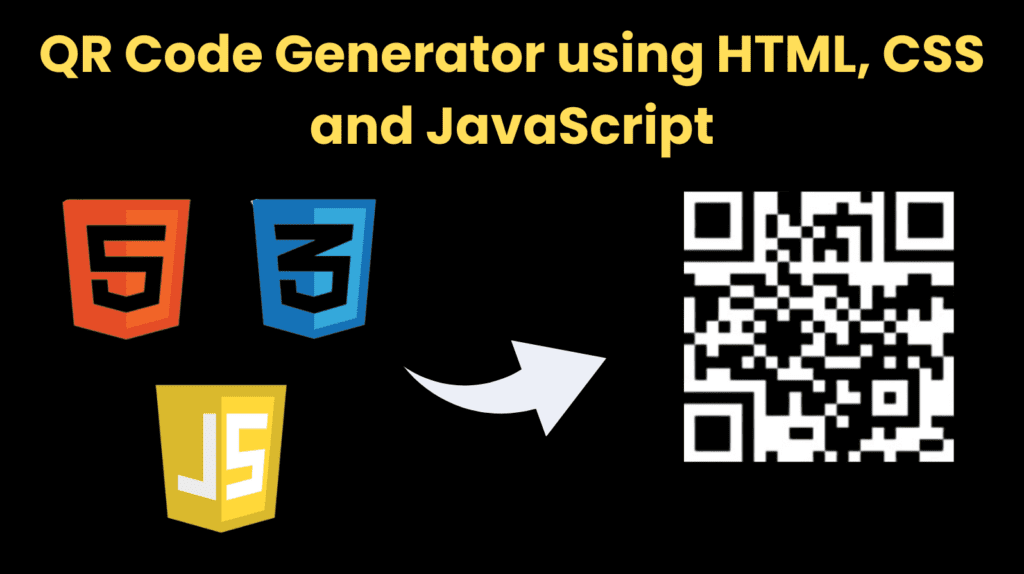
Introduction :
The QR Code Generator project is a web application designed to facilitate the quick and effortless creation of QR codes from user-provided text or URLs. Developed using a combination of HTML, CSS, and JavaScript, this application offers a user-friendly interface that streamlines the QR code generation process. The primary goal of this project is to empower users to convert any arbitrary text or URL into a scannable QR code, enhancing accessibility and convenience in sharing information.
The user interface comprises a clean and intuitive design, featuring a prominent header that succinctly introduces the application as the “QR Code Generator.” Users are guided through the process with a clear call-to-action, prompting them to paste the desired text or URL in the designated input field. This input field benefits from real-time updates, responding dynamically to user interactions through event listeners.
The underlying logic, implemented in JavaScript, ensures a seamless experience. Upon clicking the “Generate QR Code” button, the application triggers an asynchronous process to fetch a QR code image from an external API. The integration of an event listener not only facilitates the timely update of the UI but also ensures that repetitive generation requests are efficiently managed, preventing unnecessary API calls.
In summary, the QR Code Generator project blends functionality with user experience, offering a straightforward solution for generating QR codes. Whether for sharing URLs, contact information, or any other text-based data, this web application provides a valuable tool for users seeking a quick and efficient QR code creation process.
Explanation :
JavaScript Logic (script.js
):
Querying DOM Elements:
const wrapper = document.querySelector(".wrapper")
: Selects the main content wrapper.const qrInput = wrapper.querySelector(".form input")
: Selects the input field.const generateBtn = wrapper.querySelector(".form button")
: Selects the generate button.const qrImg = wrapper.querySelector(".qr-code img")
: Selects the image tag where the QR code will be displayed.let preValue
: Holds the previous value of the input.
Event Listeners:
generateBtn.addEventListener("click", () => {...})
: Listens for a click on the generate button.- It checks if the input value is not empty and is different from the previous value.
- Updates
preValue
and changes the button text to indicate QR code generation. - Dynamically sets the
src
attribute of theqrImg
to the QR code generated from the entered value using an API. - Adds a load event listener to the
qrImg
to handle the completion of image loading. - Updates the UI by adding the “active” class and resetting the button text.
qrInput.addEventListener("keyup", () => {...})
: Listens for keyup events in the input field.- If the input field is empty, removes the “active” class and resets
preValue
.
Purpose of Functions:
- Purpose: To generate the QR code when the button is clicked.
- Steps:
- Get the trimmed value from the input.
- Check if the value is not empty and has changed.
- Update
preValue
to the current value. - Change the button text to indicate QR code generation.
- Set the
src
attribute ofqrImg
to the generated QR code. - Add a load event listener to handle UI updates after image loading.
Keyup Event Listener:
- Purpose: To reset the UI when the input field is empty.
- Steps:
- Check if the input field is empty.
- If empty, remove the “active” class and reset
preValue
.
SOURCE CODE :
HTML (index.html)
QR Code Generator | CodingNepal
QR Code Generator
Paste the URL/Text here
CSS (style.css)
@import url('https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;700&display=swap');
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Poppins', sans-serif;
}
body {
display: flex;
padding: 0 10px;
min-height: 100vh;
align-items: center;
background: #a6aeb4;
justify-content: center;
flex-direction: column;
}
::selection {
color: #fff;
background: #3498DB;
}
.wrapper {
height: 265px;
max-width: 410px;
background: #e7d4d4;
border-radius: 7px;
padding: 20px 25px 0;
transition: height 0.2s ease;
box-shadow: 0 10px 30px rgba(0, 0, 0, 0.1);
}
.wrapper.active {
height: 530px;
}
header {
margin: 2rem;
background-color: #c7d8e4;
padding: 1rem;
border-radius: 1.5rem;
}
header h1 {
font-size: 40px;
font-weight: 500;
text-align: center;
}
header p {
margin-top: 5px;
color: #575757;
font-size: 25px;
text-align: center;
}
.wrapper .form {
margin: 20px 0 25px;
}
.form :where(input, button) {
width: 100%;
height: 55px;
border: none;
outline: none;
border-radius: 5px;
transition: 0.1s ease;
}
.form input {
font-size: 18px;
padding: 0 17px;
border: 1px solid #999;
}
.form input:focus {
box-shadow: 0 3px 6px rgba(0, 0, 0, 0.13);
}
.form input::placeholder {
color: #999;
}
.form button {
color: #fff;
cursor: pointer;
margin-top: 20px;
font-size: 17px;
background: #44e14e;
}
.qr-code {
opacity: 0;
display: flex;
padding: 33px 0;
border-radius: 5px;
align-items: center;
pointer-events: none;
justify-content: center;
border: 1px solid #ccc;
}
.wrapper.active .qr-code {
opacity: 1;
pointer-events: auto;
transition: opacity 0.5s 0.05s ease;
}
.qr-code img {
width: 170px;
}
@media (max-width: 430px) {
.wrapper {
height: 255px;
padding: 16px 20px;
}
.wrapper.active {
height: 510px;
}
header p {
color: #696969;
}
.form :where(input, button) {
height: 52px;
}
.qr-code img {
width: 160px;
}
}
JavaScript (script.js)
const wrapper = document.querySelector(".wrapper"),
qrInput = wrapper.querySelector(".form input"),
generateBtn = wrapper.querySelector(".form button"),
qrImg = wrapper.querySelector(".qr-code img");
let preValue;
generateBtn.addEventListener("click", () => {
let qrValue = qrInput.value.trim();
if(!qrValue || preValue === qrValue) return;
preValue = qrValue;
generateBtn.innerText = "Generating QR Code...";
qrImg.src = `https://api.qrserver.com/v1/create-qr-code/?size=200x200&data=${qrValue}`;
qrImg.addEventListener("load", () => {
wrapper.classList.add("active");
generateBtn.innerText = "Generate QR Code";
});
});
qrInput.addEventListener("keyup", () => {
if(!qrInput.value.trim()) {
wrapper.classList.remove("active");
preValue = "";
}
});
OUTPUT :
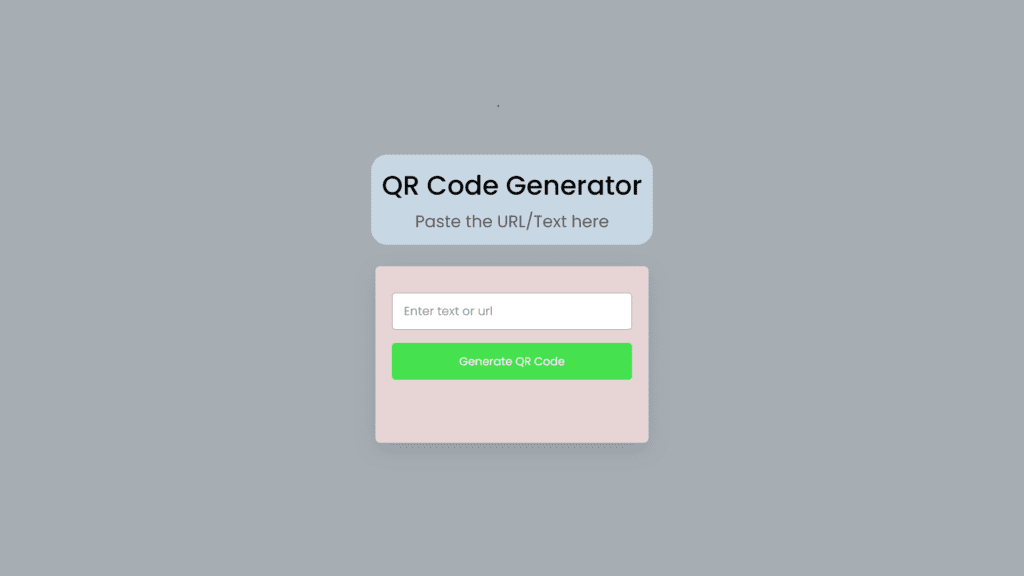
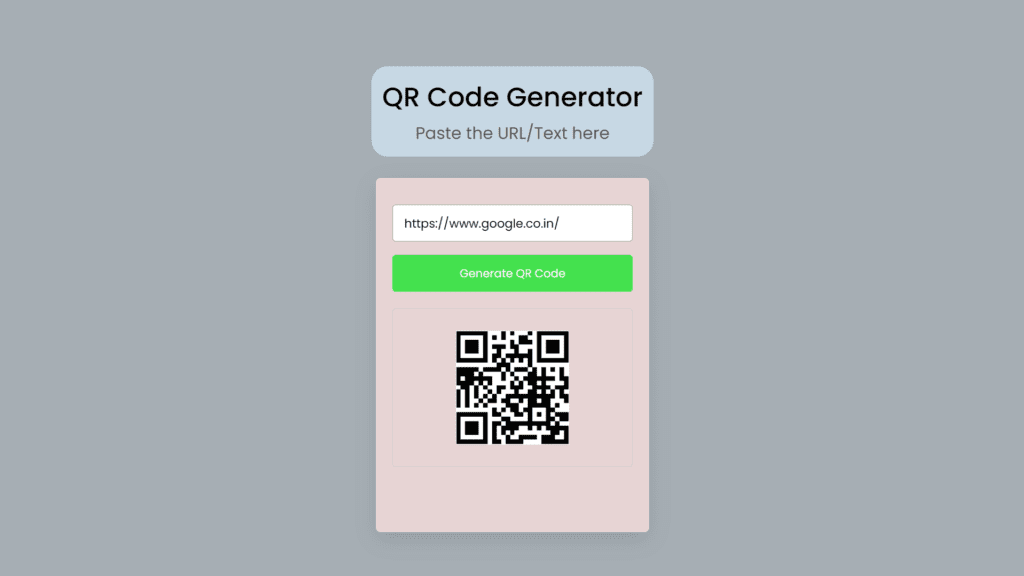
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …