Notes app using HTML, CSS and JavaScript
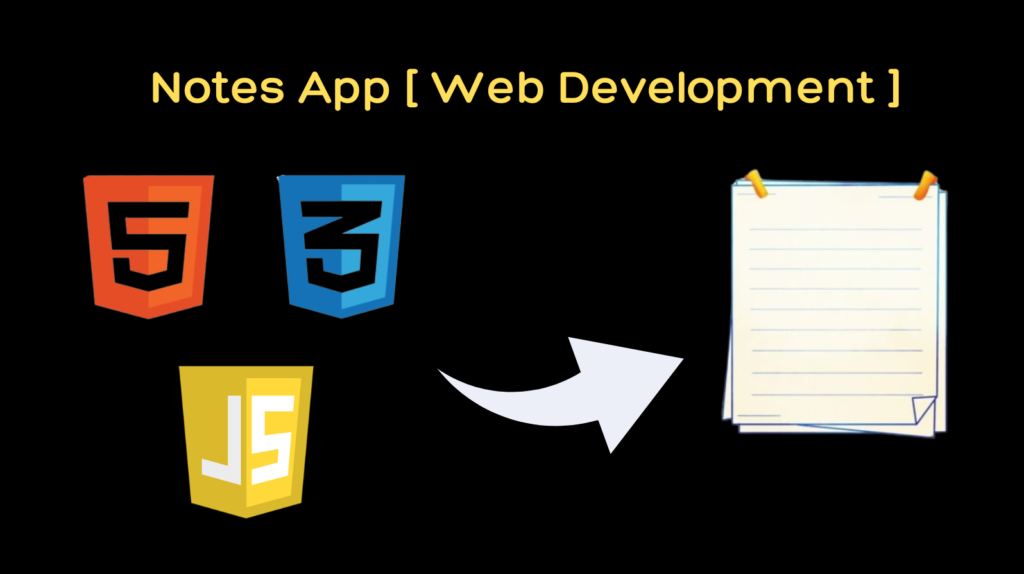
Introduction :
The Notes App is a web application that allows users to create, view, and delete notes. Developed using HTML, CSS and JavaScript, this app provides a simple and intuitive interface for managing personal notes. Using this app a user can make his/her notes and store them as well as edit them as per his need. Use of localstorage database helps to store the written tasks in the browser. In this project the localstorage plays very important role to store the data of notes. This app is compatible with all the devices which has successful accessibility of browser as well as internet connection. This app is build for both laptop as well as mobile devices so any user who is using this app in mobile phones can easily take advantage of its feature by hassle-free experience. The user interface of this app is developed in such way that any user that is new to this app can understand the structure as well as working of this app. This app help you to record your daily tasks and delete them as soon as you complete them.
Explanation :
HTML Structure:
- A navigation bar (commented out) for future enhancements.
- A container for adding and displaying notes.
- A form for adding new notes with a text area and a button.
- A section to display existing notes with a delete button for each.
CSS Styling:
- Layout adjustments using Bootstrap for responsiveness.
- A clean and organized design for a user-friendly interface.
JavaScript Logic:
- DOM elements are selected using
document.getElementById and
document.getElementsByClassName
. - Event listeners are set for the “Add Note” button and the search input.
- Local storage is used to store and retrieve notes.
Features of this project are as follows :
- Add a new note with a text description.
- View and delete existing notes.
- Responsive design using Bootstrap for a user-friendly experience.
- Search functionality to filter notes based on content.
Source Code :
HTML (index.html)
Notes App
Record your notes here
Add a note
Your Notes
CSS (style.css)
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
display: flex;
justify-content: center;
}
.navbar {
background-color: #343a40;
padding: 1rem;
}
.navbar-brand {
color: #ffffff;
font-size: 1.5rem;
font-weight: bold;
}
.navbar-toggler {
background-color: #ffffff;
}
.navbar-toggler-icon {
color: #343a40;
}
.navbar-nav {
margin-top: 10px;
}
.nav-item {
margin-right: 10px;
}
.form-inline {
display: flex;
align-items: center;
}
.form-control {
margin-right: 10px;
}
.container {
max-width: 800px;
}
.card {
margin-top: 20px;
}
.card-body {
padding: 20px;
}
.btn-primary {
background-color: #007bff;
color: #ffffff;
border: none;
padding: .8rem;
border-radius: .3rem;
}
.btn-outline-success {
color: #28a745;
border-color: #28a745;
}
hr {
border: 1px solid #343a40;
margin: 20px 0;
}
h1 {
font-size: 2rem;
opacity: .6;
}
.container{
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
width: 100%;
background-color: #eeeeee;
}
textarea{
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
font-size: 1rem;
padding: .5rem;
}
JavaScript (app.js)
console.log("Welcome to notes app. This is app.js");
showNotes();
// If user adds a note, add it to the localStorage
let addBtn = document.getElementById("addBtn");
addBtn.addEventListener("click", function (e) {
let addTxt = document.getElementById("addTxt");
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
notesObj.push(addTxt.value);
localStorage.setItem("notes", JSON.stringify(notesObj));
addTxt.value = "";
showNotes();
});
// Function to show elements from localStorage
function showNotes() {
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
let html = "";
notesObj.forEach(function (element, index) {
html += `
Note ${index + 1}
${element}
`;
});
let notesElm = document.getElementById("notes");
if (notesObj.length != 0) {
notesElm.innerHTML = html;
} else {
notesElm.innerHTML = `Nothing to show! Use "Add a Note" section above to add notes.`;
}
}
// Function to delete a note
function deleteNote(index) {
// console.log("I am deleting", index);
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
notesObj.splice(index, 1);
localStorage.setItem("notes", JSON.stringify(notesObj));
showNotes();
}
let search = document.getElementById('searchTxt');
search.addEventListener("input", function () {
let inputVal = search.value.toLowerCase();
// console.log('Input event fired!', inputVal);
let noteCards = document.getElementsByClassName('noteCard');
Array.from(noteCards).forEach(function (element) {
let cardTxt = element.getElementsByTagName("p")[0].innerText;
if (cardTxt.includes(inputVal)) {
element.style.display = "block";
}
else {
element.style.display = "none";
}
// console.log(cardTxt);
})
})
const sample = () => {
console
}
Functions :
showNotes()
: Retrieves and displays notes from local storage.addBtn
Event Listener: Adds a new note to local storage and updates the display.deleteNote(index)
: Deletes a selected note from local storage and updates the display.search
Event Listener: Filters notes based on the search input.
OUTPUT :
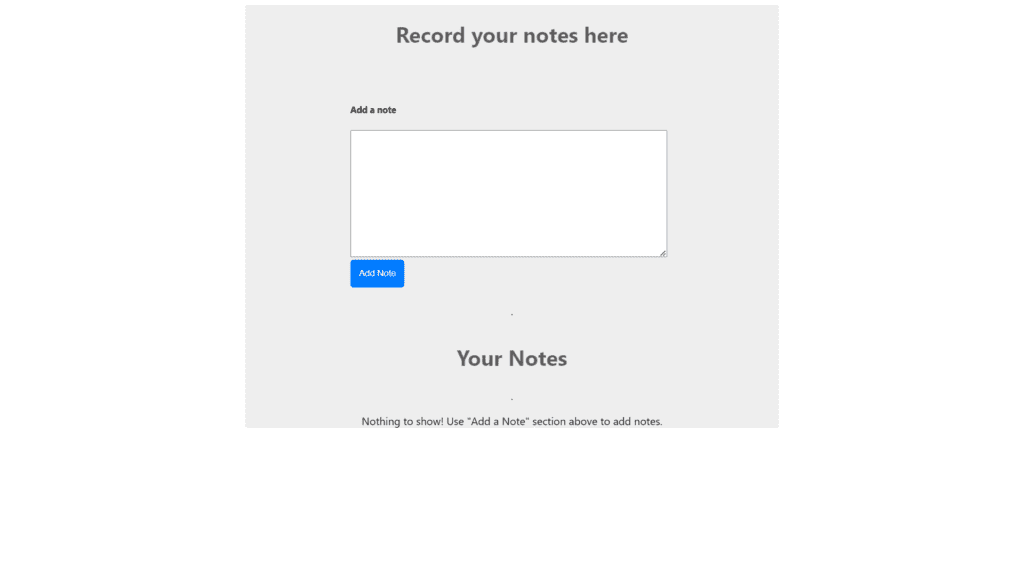
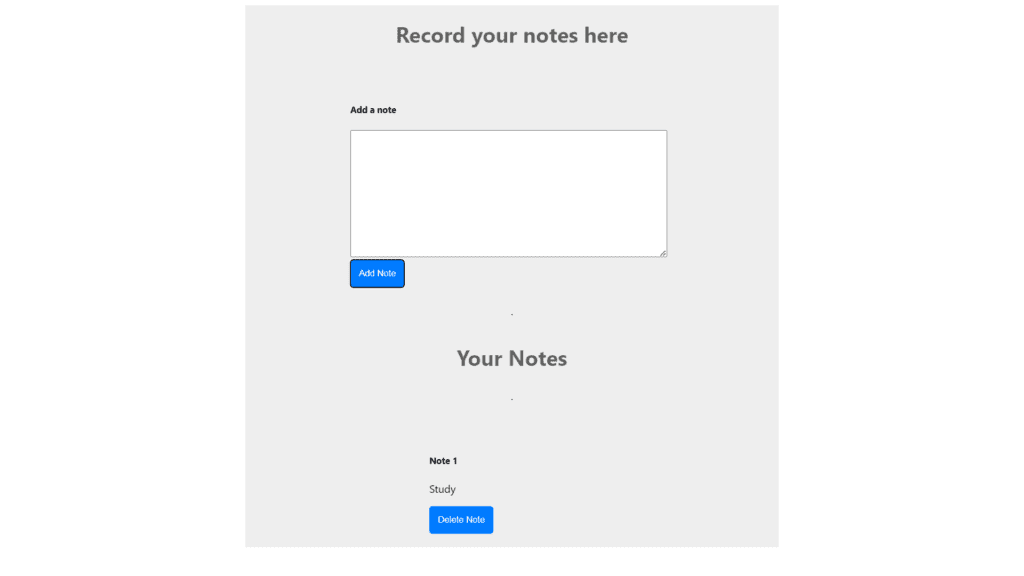
Conclusion :
The Notes App is a straightforward and effective solution for managing personal notes. With its clean design, responsive layout, and basic features, it provides a foundation that can be extended with additional functionalities in the future. The use of HTML, CSS and JavaScript ensures compatibility and ease of use for users across different devices.
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …