AI Image Generator using HTML, CSS and JavaScript
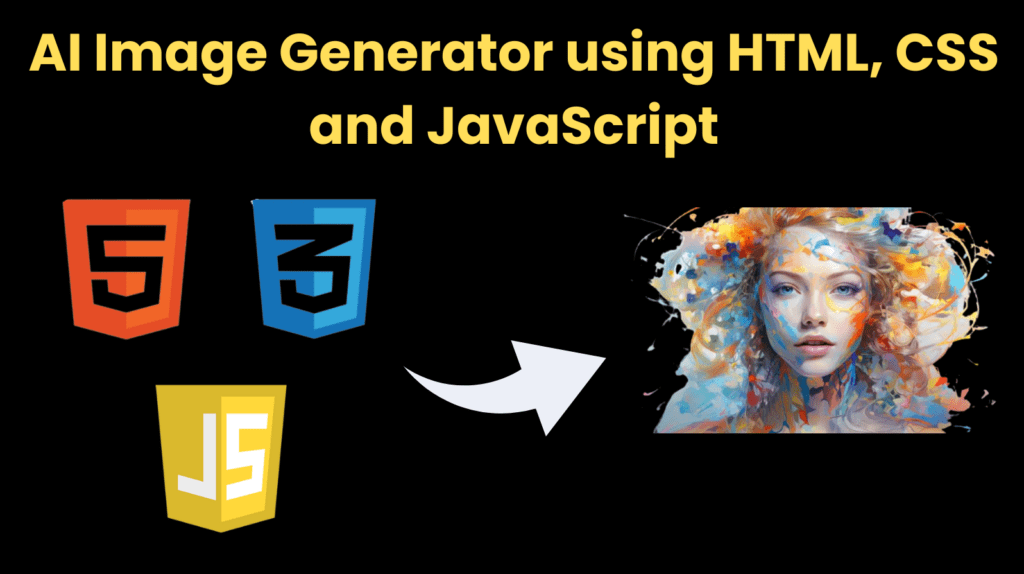
Introduction :
This project is an AI Image Generator built using HTML, CSS, and JavaScript. It allows users to input a text description, and then, using the OpenAI API, generates a set of images based on that description. The generated images are displayed in a gallery format, and users can download the images they like. The application opens with a dedicated section for image generation, featuring a prominent heading introducing the purpose of the tool. A descriptive paragraph outlines the functionality, encouraging users to convert their textual prompts into visually appealing images. A well-designed form allows users to input their desired image description. The form also includes a dropdown menu enabling users to specify the quantity of images they wish to generate. Clear and concise placeholder text guides users on the type of input expected. The form is equipped with interactive controls, including a submit button that triggers the image generation process. A visually intuitive loading state is incorporated, providing users with real-time feedback during the generation phase. Once the images are generated, they are elegantly presented in the gallery section. Each image is encapsulated within a dedicated card, featuring both the generated image and a download button for easy access
Explanation :
HTML Structure:
- The HTML structure includes two main sections: the image generator form and the image gallery.
- The form collects user input, such as the description of the image and the quantity of images to generate.
- The gallery displays the generated images along with download buttons.
JavaScript Logic:
- JavaScript code handles the logic of generating images using the OpenAI API.
- It utilizes the Fetch API to send a POST request to OpenAI’s image generation endpoint.
- The response from the API is processed to extract image data and update the gallery accordingly.
- The OpenAI API key is stored in the
OPENAI_API_KEY
constant. Ensure that you keep your API key secure and consider using server-side logic to handle API requests for increased security.
Image Generation Function (generateAiImages
):
- This asynchronous function takes user input (prompt and image quantity) and sends a request to the OpenAI API.
- The API response is then processed to update the image cards in the gallery.
Image Card Update Function (updateImageCard
):
- This function is responsible for updating the image cards in the gallery.
- It takes an array of image objects, extracts the base64-encoded image data, and updates the image source and download button attributes.
Form Submission Event Listener:
- The form submission is prevented from triggering the default behavior (page reload).
- User input is obtained from the form fields, and a loading state is displayed in the gallery while images are being generated.
- The
generateAiImages
function is called to initiate the image generation process.
SOURCE CODE :
HTML (index.html)
CSS (style.css)
/* Importing Google font - Poppins */
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;700&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
.image-generator {
height: 40vh;
display: flex;
align-items: center;
justify-content: center;
position: relative;
background-color: #565656;
background-size: cover;
background-position: center;
}
.image-generator::before {
content: "";
position: absolute;
left: 0;
top: 0;
width: 100%;
height: 100%;
opacity: 0.5;
background: #121212;
}
.image-generator .content {
position: relative;
color: #fff;
padding: 0 15px;
max-width: 760px;
text-align: center;
}
.image-generator h1 {
font-size: 2.5rem;
font-weight: 700;
}
.image-generator p {
margin-top: 10px;
font-size: 1.35rem;
}
.image-generator .generate-form {
height: 56px;
padding: 6px;
display: flex;
margin-bottom: 15px;
background: #fff;
align-items: center;
border-radius: 30px;
margin-top: 45px;
justify-content: space-between;
}
.generate-form .prompt-input {
width: 100%;
height: 100%;
outline: none;
padding: 0 17px;
border: none;
background: none;
font-size: 1rem;
border-radius: 30px;
}
.generate-form .controls {
display: flex;
height: 100%;
gap: 15px;
}
.generate-form .img-quantity {
outline: none;
border: none;
height: 44px;
background: none;
font-size: 1rem;
}
.generate-form .generate-btn {
font-size: 1rem;
outline: none;
border: none;
font-weight: 500;
color: #fff;
cursor: pointer;
height: 100%;
padding: 0 25px;
border-radius: 30px;
background: #4949E7;
}
.generate-form .generate-btn[disabled] {
opacity: 0.6;
pointer-events: none;
}
.generate-form button:hover {
background: #1d1de2;
}
.image-gallery {
display: flex;
gap: 15px;
padding: 0 15px;
flex-wrap: wrap;
justify-content: center;
margin: 50px auto;
max-width: 1250px;
}
.image-gallery .img-card {
display: flex;
position: relative;
align-items: center;
justify-content: center;
background: #f2f2f2;
border-radius: 4px;
overflow: hidden;
aspect-ratio: 1 / 1;
width: 285px;
}
.image-gallery .img-card img {
height: 100%;
width: 100%;
object-fit: cover;
}
.image-gallery .img-card.loading img {
width: 80px;
height: 80px;
}
.image-gallery .img-card .download-btn {
bottom: 15px;
right: 15px;
height: 36px;
width: 36px;
display: flex;
align-items: center;
justify-content: center;
text-decoration: none;
background: #fff;
border-radius: 50%;
position: absolute;
opacity: 0;
pointer-events: none;
transition: 0.2s ease;
}
.image-gallery .img-card .download-btn img {
width: 14px;
height: 14px;
}
.image-gallery .img-card:not(.loading):hover .download-btn {
opacity: 1;
pointer-events: auto;
}
@media screen and (max-width: 760px) {
.image-generator {
height: 45vh;
padding-top: 30px;
align-items: flex-start;
}
.image-generator h1 {
font-size: 1.8rem;
}
.image-generator p {
font-size: 1rem;
}
.image-generator .generate-form {
margin-top: 30px;
height: 52px;
display: block;
}
.generate-form .controls {
height: 40px;
margin-top: 15px;
justify-content: end;
align-items: center;
}
.generate-form .generate-btn[disabled] {
opacity: 1;
}
.generate-form .img-quantity {
color: #fff;
}
.generate-form .img-quantity option {
color: #000;
}
.image-gallery {
margin-top: 20px;
}
.image-gallery .img-card:not(.loading) .download-btn {
opacity: 1;
pointer-events: auto;
}
}
@media screen and (max-width: 500px) {
.image-gallery .img-card {
width: 100%;
}
}
JavaScript (app.js)
const generateForm = document.querySelector(".generate-form");
const ImageGallery = document.querySelector(".image-gallery");
const OPENAI_API_KEY = "ENTER YOUR API KEY HERE FROM OPENAI API SITE"
async function generateAiImages(userPrompt, userImageQuantity) {
function updateImageCard(imgDataArray) {
imgDataArray.forEach((imgObject, index) => {
const imgCard = ImageGallery.querySelectorAll(".img-card")[index];
const imgElement = imgCard.querySelector("img");
const downloadBtn = imgCard.querySelector(".download-btn");
const aiGeneratedImg = `data:image/jpeg;base64,${imgObject.b64_json}`;
imgElement.src = aiGeneratedImg;
imgElement.onload = () => {
imgCard.classList.remove("loading");
downloadBtn.setAttribute("href", aiGeneratedImg);
downloadBtn.setAttribute("download", `${userPrompt}.jpg`);
}
});
}
try {
const response = await fetch("https://api.openai.com/v1/images/generations", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${OPENAI_API_KEY}`
},
body: JSON.stringify({
prompt: userPrompt,
n: parseInt(userImageQuantity),
size: "512x512",
response_format: "b64_json"
})
});
if (!response.ok) throw new Error("Failed to generate Images")
const { data } = await response.json();
console.log(data);
updateImageCard([...data])
} catch (error) {
alert(error.message)
}
}
generateForm.addEventListener("submit", function (event) {
// user input and image quantity values from the form
event.preventDefault()
const userPrompt = event.srcElement[0].value;
const userImageQuantity = event.srcElement[1].value;
// creating HTML markup for image cars with loading state
const imgCardMarkup = Array.from({ length: userImageQuantity }, function () {
return (
` `
)
}
).join("");
// console.log(imgCardMarkup)
ImageGallery.innerHTML = imgCardMarkup;
generateAiImages(userPrompt, userImageQuantity);
})
OUTPUT :
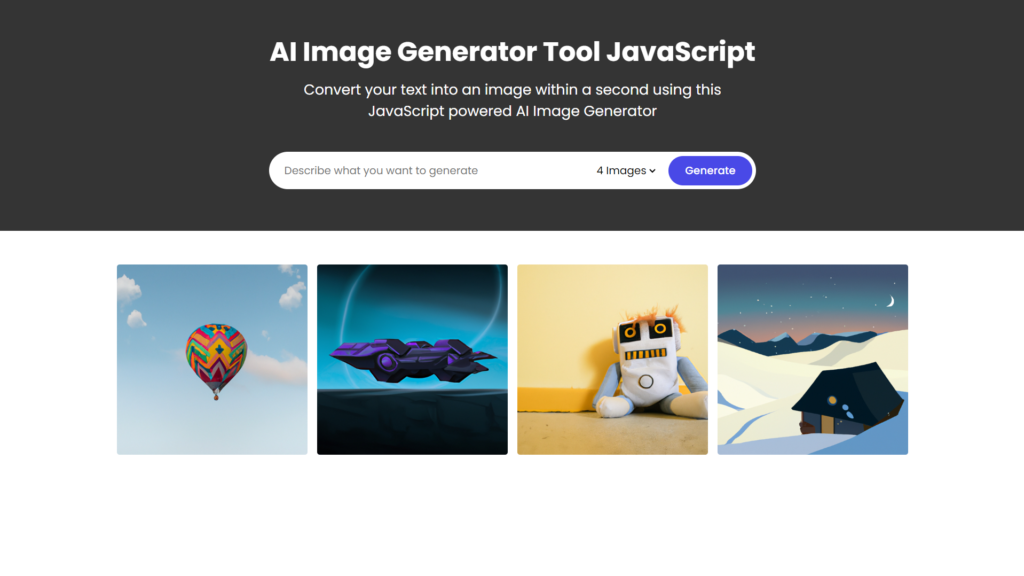
More HTML CSS JS Projects
Get Huge Discounts
Get Discount on Top EdTech Compnies
Find More Projects
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …