Valentine Day Project using Python Turtle With Source Code
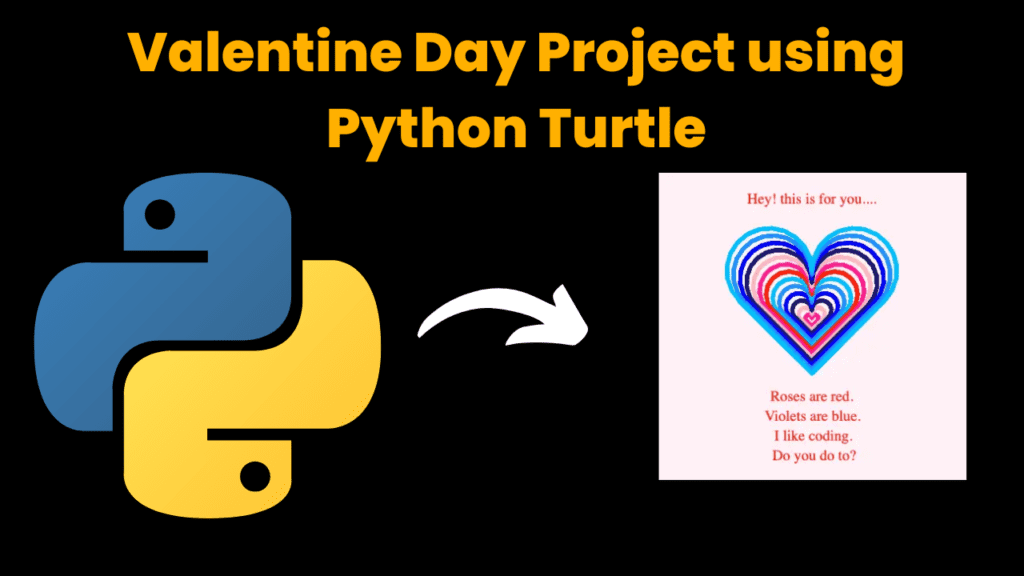
Introduction :
Valentine’s Day, a day of love and affection, is just around the corner. Why not celebrate it in a unique way this year? Let’s create a beautiful animation of hearts using Python’s Turtle module. This project is not only fun but also a great way to learn and understand Python programming.
Required Python Modules :
This project requires two Python modules: turtle
and math
. The turtle
module is a part of Python’s standard library and is used for creating graphics. The math
module provides mathematical functions and is used in this project to calculate the shape of the heart.
Code Explanation :
Let’s break down the code into smaller parts for better understanding:
Importing Modules: We start by importing the necessary modules.
turtle
for graphics andmath
for mathematical functions.Setting up the Environment: We create a turtle object
t
and a screenwn
. We set the turtle’s shape to “turtle”, the background color to “lavender blush”, and the turtle’s speed to 4.Color Function: This function sets the pen color based on the iteration number. We have a list of colors, and we choose the color by taking the remainder of the iteration number divided by the length of the color list.
At Function: This function moves the turtle to a specific location on the screen without drawing anything.
Heart Function: This function draws a heart. It takes two parameters:
size
andshape
. Thesize
determines the size of the heart, and theshape
determines the shape of the heart. We use thesin
function from themath
module and some geometry to calculate the radius of the circles that form the heart.Hearts Function: This function draws a series of hearts. For each iteration, it changes the color, moves the turtle down, and draws a heart with increasing size.
Displaying the Message: We move the turtle to specific locations and use the
write
method to display a message.
Get Discount on Top Educational Courses
Source Code :
from math import sin, pi
import turtle
t = turtle.Turtle()
wn = turtle.Screen()
t.shape("turtle")
wn.bgcolor("lavender blush")
t.speed(4)
colors = ["red", "deep pink", "light pink", "midnight blue", "blue", "dodger blue", "deep sky blue"]
def color(iteration):
t.pencolor(colors[iteration % len(colors)])
def at(x, y):
t.penup()
t.home()
t.forward(x)
t.left(90)
t.forward(y)
t.pendown()
def heart(size, shape):
t.pensize(5)
radius = size * sin(shape * pi / 180) / (1 + sin((90 - shape) * pi / 180))
t.right(shape)
t.forward(size)
t.circle(radius, 180 + shape)
t.right(180)
t.circle(radius, 180 + shape)
t.forward(size)
t.left(180 - shape)
def hearts():
turtle.delay(0)
for iteration in range(1, 14):
color(iteration)
at(0, iteration * -5)
heart(iteration * 10, 45)
t.penup()
t.goto(0,150)
t.color("red")
t.write("Hey! this is for you....", None, "center", "14pt bold")
t.goto(1,-125)
hearts()
t.penup()
t.goto(0,-100)
t.color("red")
t.write("Roses are red.", None, "center", "14pt bold")
t.goto(1,-125)
t.write("Violets are blue.", None, "center", "14pt bold")
t.goto(2,-150)
t.write("I like coding.", None, "center", "14pt bold")
t.goto(3,-175)
t.write("Do you do to?", None, "center", "14pt bold")
t.color("white")
t.goto(0,-300)
Output :
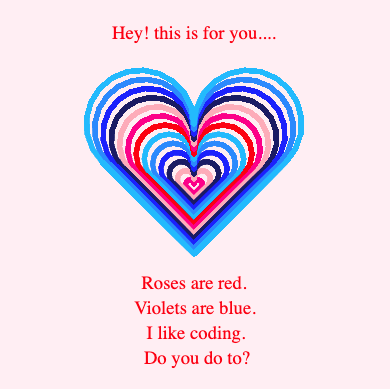
Find More Projects
MathGenius Pro – AI-Powered Math Solver Using Python Introduction: From simple arithmetic to more complicated college-level subjects like integration, differentiation, algebra, matrices, …
CipherMaze: The Ultimate Code Cracking Quest Game Using Python Introduction: You can make CipherMaze, a fun and brain-boosting puzzle game, with Python …
Warp Perspective Using Open CV Python Introduction: In this article, we are going to see how to Create a Warp Perspective System …
Custom AI Story Generator With Emotion Control Using Python Introduction: With the help of this AI-powered story generator, users can compose stories …
AI Powered PDF Summarizer Using Python Introduction: AI-Powered PDF Summarizer is a tool that extracts and summarizes research papers from ArXiv PDFs using Ollama (Gemma 3 LLM). The …
AI Based Career Path Recommender Using Python Introduction: One of the most significant and frequently perplexing decisions in a person’s life is …