Photo Collage Maker using Python
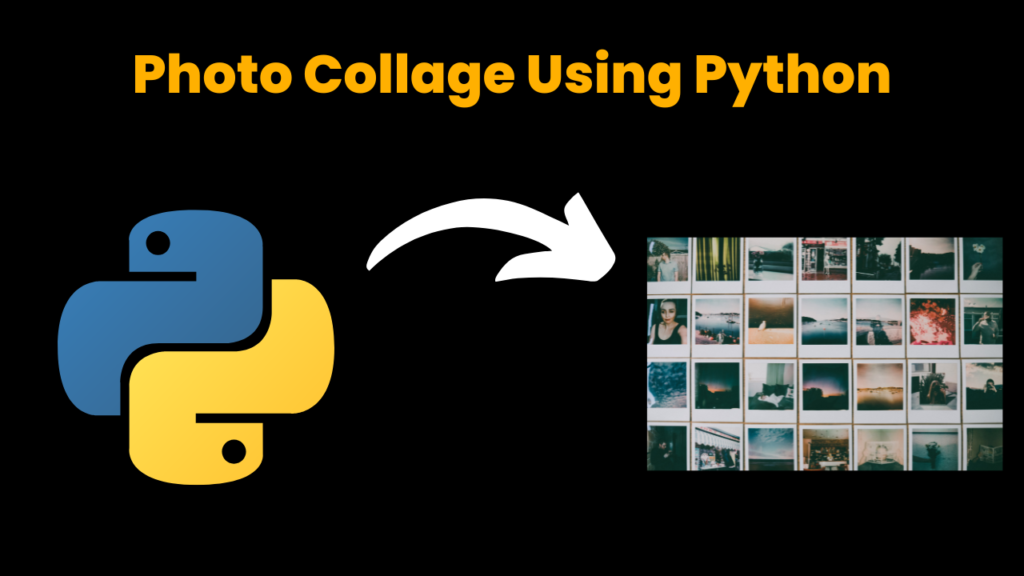
Introduction
Hello Curious Coders,
In this Project we are going to discuss how to form a collage of images using python. The Photo Collage is a group of multiple photographs or images arranged and displayed together in a single composition. The PIL packeg is used to generate a collage in Python as follows….
Code
#Import required Libraries
from PIL import Image
# Open the images and resize them to equal size to ensure collage looks beautiful
image1 = Image.open("image1.png")
image1=image1.resize((500,500))
image2 = Image.open("image2.png")
image2=image2.resize((500,500))
image3 = Image.open("image3.png")
image3=image3.resize((500,500))
image4 = Image.open("image4.png")
image4=image4.resize((500,500))
image5 = Image.open("image5.png")
image5=image5.resize((500,500))
# Creation of Image which enables users to paste the images
collage= Image.new("RGBA",(1500,1500),color="black")
collage.paste(image1,(0,0))
collage.paste(image3,(1000,0))
collage.paste(image2,(500,500))
collage.paste(image4,(0,1000))
collage.paste(image5,(1000,1000))
# Save the newly genrated collage image
collage.save("Photo_Collage.png")
Code Explanation
- First we imported required PIL package
- Later using Image.open() fucntion we are going to open images.
- Using Image.resize() fucntion we resized images to same resolution to ensure collage looks good.
- Next we created a simple image using Image.new() function which is able to store all the opened images.
- Finally using Image.save() function we saved our image(Photo_Collage.png)
Output
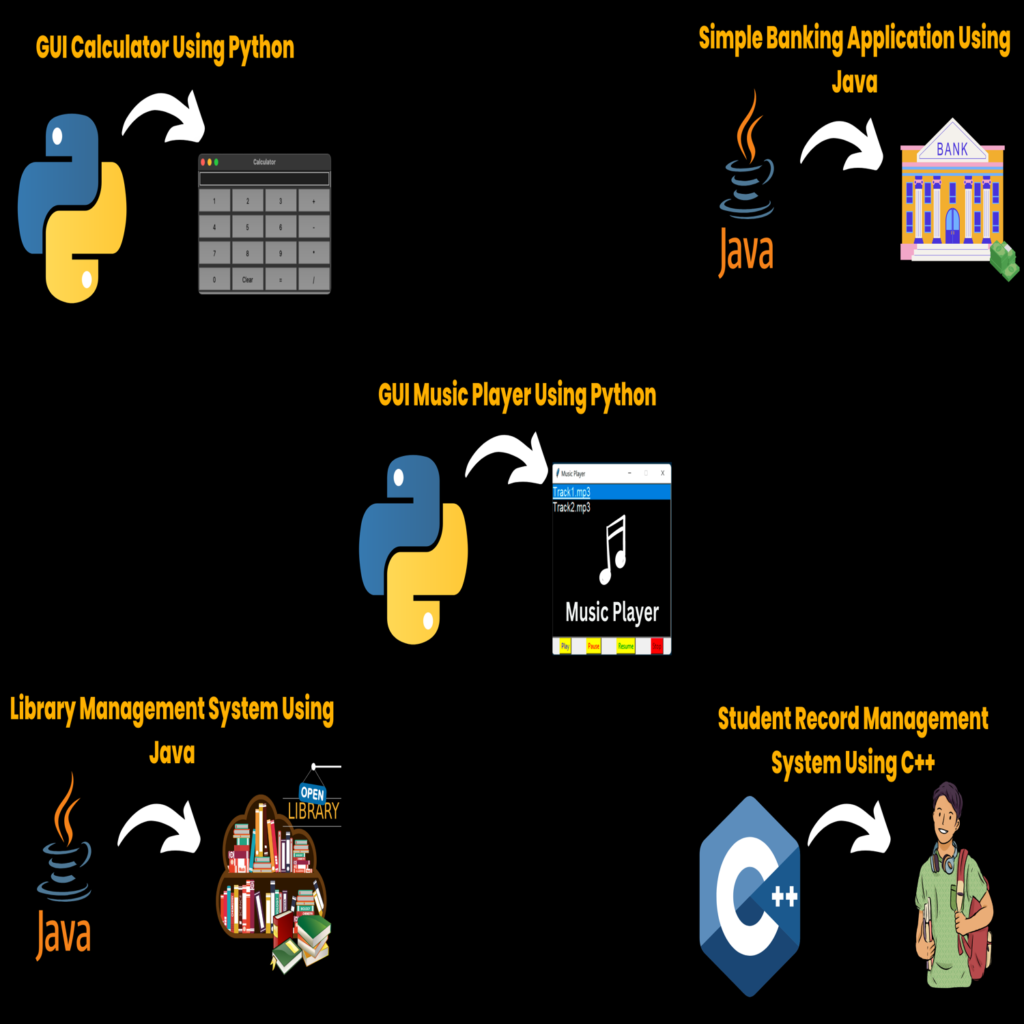
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …