Creating GUI Piano Using Python With Source Code
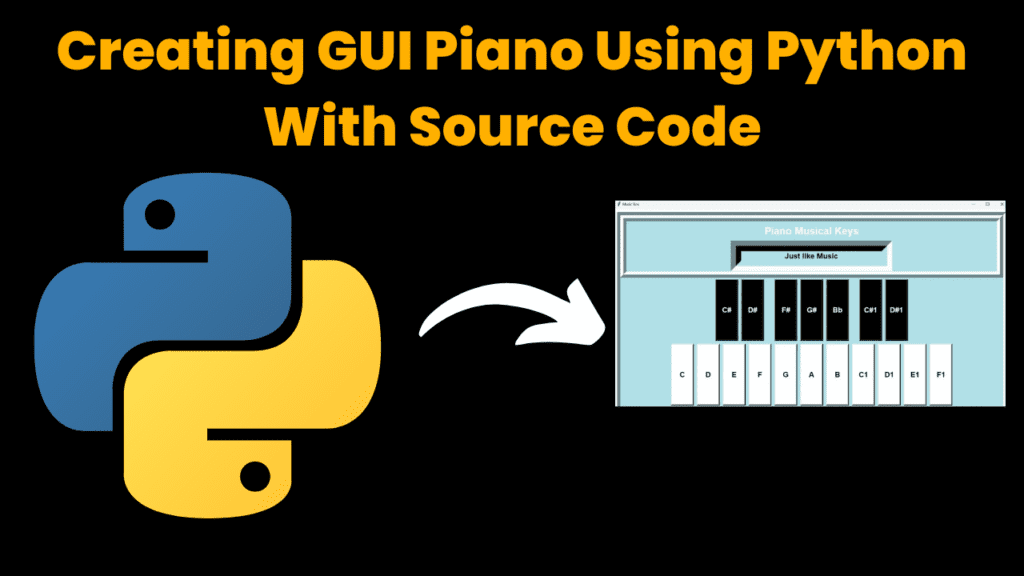
Introduction:
In this project, we are going to build a GUI piano by using different libraries of Python. With the help of the tkinter library, we will create the GUI for the project. As the name suggests, several keynotes of the piano will be there and by clicking them the sound will get produced accordingly. For playing the sound we will use the mixer module of the pygame library.
Explanation:
The main objectives which will be needed to cover this project are:
- Building the frame widgets
- Creating the keynotes as Buttons
- Adding the sound
First of all, we will import all the necessary libraries, then we will create the instance of the tkinter frame i.e. Tk(). With this, we will also set the title and geometry for our window by using “.title()” & “.geometry()”. Adding to this, we will also set the background color of the window by the “.configure(background=<color name>)” function.
Source Code:
# Importing the libraries
from tkinter import *
import pygame
# initializing the pygame
pygame.init()
root = Tk()
# Setting the title and geometry
root.title("Music Box")
root.geometry("1352x700+0+0")
root.configure(background="white")
# creating the Frames
abc = Frame(root, bg="powder blue", bd=20, relief=RIDGE)
abc.grid()
abc1 = Frame(abc, bg="powder blue", bd=20, relief=RIDGE)
abc1.grid()
abc2 = Frame(abc, bg="powder blue", relief=RIDGE)
abc2.grid()
abc3 = Frame(abc, bg="powder blue", relief=RIDGE)
abc3.grid()
str = StringVar()
str.set("Just like Music")
#Functions for sound
def value_Cs():
str.set("C#")
sound = pygame.mixer.Sound("C#.wav")
sound.play()
def value_A():
str.set("A")
sound = pygame.mixer.Sound("A.wav")
sound.play()
def value_B():
str.set("B")
sound = pygame.mixer.Sound("B.wav")
sound.play()
def value_C():
str.set("C")
sound = pygame.mixer.Sound("C.wav")
sound.play()
def value_Bb():
str.set("Bb")
sound = pygame.mixer.Sound("Bb.wav")
sound.play()
def value_Gs():
str.set("G#")
sound = pygame.mixer.Sound("G#.wav")
sound.play()
def value_Ds():
str.set("D#")
sound = pygame.mixer.Sound("D#.wav")
sound.play()
def value_Fs():
str.set("F#")
sound = pygame.mixer.Sound("F#.wav")
sound.play()
def value_G():
str.set("G")
sound = pygame.mixer.Sound("G.wav")
sound.play()
def value_D():
str.set("D")
sound = pygame.mixer.Sound("D.wav")
sound.play()
def value_E1():
str.set("E1")
sound = pygame.mixer.Sound("E.mp3")
sound.play()
def value_E():
str.set("E")
sound = pygame.mixer.Sound("E!.mp3")
sound.play()
def value_F():
str.set("F")
sound = pygame.mixer.Sound("F!.mp3")
sound.play()
def value_F1():
str.set("F1")
sound = pygame.mixer.Sound("F.mp3")
sound.play()
def value_C1():
str.set("C1")
sound = pygame.mixer.Sound("C1.mp3")
sound.play()
def value_D1():
str.set("D1")
sound = pygame.mixer.Sound("D1.mp3")
sound.play()
def value_Cs1():
str.set("C#1")
sound = pygame.mixer.Sound("C#1.mp3")
sound.play()
def value_Ds1():
str.set("C#1")
sound = pygame.mixer.Sound("D#1.mp3")
sound.play()
# Label
Label(abc1, text="Piano Musical Keys", font=("arial", 25, "bold"), padx=8, pady=8, bd=4, width=59, bg="powder blue",
fg="white", height=1,
justify=CENTER).grid(row=0, column=0, columnspan=11)
display = Entry(abc1, textvariable=str, font=("arial", 18, "bold"), width=35, bd=34, bg="powder blue", fg="black",
justify=CENTER).grid(row=1, column=5, pady=1)
# Buttons for keynotes
btnCs = Button(abc2, height=6, width=4, bd=4, text="C#", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Cs)
btnCs.grid(row=0, column=0, padx=5, pady=5)
btnDs = Button(abc2, height=6, width=4, bd=4, text="D#", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Ds)
btnDs.grid(row=0, column=1, padx=5, pady=5)
btnSpace1 = Button(abc2, state=DISABLED, height=6, width=2, bg="powder blue", relief=FLAT)
btnSpace1.grid(row=0, column=3, padx=0, pady=0)
btnFs = Button(abc2, height=6, width=4, bd=4, text="F#", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Fs)
btnFs.grid(row=0, column=4, padx=5, pady=5)
btnGs = Button(abc2, height=6, width=4, bd=4, text="G#", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Gs)
btnGs.grid(row=0, column=6, padx=5, pady=5)
btnBb = Button(abc2, height=6, width=4, bd=4, text="Bb", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Bb)
btnBb.grid(row=0, column=8, padx=5, pady=5)
btnSpace5 = Button(abc2, state=DISABLED, height=6, width=2, bg="powder blue", relief=FLAT)
btnSpace5.grid(row=0, column=9, padx=0, pady=0)
btnCs1 = Button(abc2, height=6, width=4, bd=4, text="C#1", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Cs1)
btnCs1.grid(row=0, column=10, padx=5, pady=5)
btnDs1 = Button(abc2, height=6, width=4, bd=4, text="D#1", font=("arial", 18, "bold"), bg="black", fg="white",
command=value_Ds1)
btnDs1.grid(row=0, column=12, padx=5, pady=5)
btnC = Button(abc3, bd=4, width=4, height=6, text="C", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_C)
btnC.grid(row=0, column=0, padx=5, pady=5)
btnD = Button(abc3, bd=4, width=4, height=6, text="D", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_D)
btnD.grid(row=0, column=1, padx=5, pady=5)
btnE = Button(abc3, bd=4, width=4, height=6, text="E", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_E)
btnE.grid(row=0, column=2, padx=5, pady=5)
btnF = Button(abc3, bd=4, width=4, height=6, text="F", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_F)
btnF.grid(row=0, column=3, padx=5, pady=5)
btnG = Button(abc3, bd=4, width=4, height=6, text="G", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_G)
btnG.grid(row=0, column=4, padx=5, pady=5)
btnA = Button(abc3, bd=4, width=4, height=6, text="A", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_A)
btnA.grid(row=0, column=5, padx=5, pady=5)
btnB = Button(abc3, bd=4, width=4, height=6, text="B", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_B)
btnB.grid(row=0, column=6, padx=5, pady=5)
btnC1 = Button(abc3, bd=4, width=4, height=6, text="C1", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_C1)
btnC1.grid(row=0, column=7, padx=5, pady=5)
btnD1 = Button(abc3, bd=4, width=4, height=6, text="D1", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_D1)
btnD1.grid(row=0, column=8, padx=5, pady=5)
btnE1 = Button(abc3, bd=4, width=4, height=6, text="E1", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_E1)
btnE1.grid(row=0, column=9, padx=5, pady=5)
btnF1 = Button(abc3, bd=4, width=4, height=6, text="F1", bg="white", fg="black", font=("arial", 18, "bold"),
command=value_F1)
btnF1.grid(row=0, column=10, padx=5, pady=5)
# Mainloop
root.mainloop()
Now we have to create the Frame widget, it is one of the most important steps. The frame widget is for the process of grouping and organizing other widgets in a somehow friendly way. It works like a container, which is responsible for arranging the position of other widgets. In this project, we will create 4 frame widgets. The need for the 4 will get discussed further. The syntax for creating the frame widget is as follows:
“Frame(root, bg=,bd=,width=,height=,….)” and with the help of “.grid()” will place it on our window.
With the frame widget, we will also create a label widget [“Label()”]and an Entry widget [“Entry()”] for the top heading and to get to know the pressed keynote respectively.
The next most important step is to create buttons to show the keynotes for the piano. The syntax is as follows: “Buttons(root, bg=,font=,height=,width=,text=…., command=)” Within the “text” parameter we will mention the name of the keynote that is to be present on the button and with the help of “command” parameter will direct it to the function to be called. In the end, with the help of “.grid()” will place the buttons accordingly on the window screen. In such a way, for this project, we have created the following keynotes of the piano.
- C# (C sharp)
- D# (D sharp)
- F# (F sharp)
- G# (G sharp)
- Bb
- C#1
- D#1
- C
- D
- E
- F
- G
- A
- B
- C1
- D1
- E1
- F1
The next step is to add the actual functionality of this project, that is, adding the sound such that whenever the buttons are clicked by the user the sound of that particular keynote will be played. For doing so, we will make user-defined functions that will make use of the “mixer” module. With the help of the “pygame.mixer.Sound()” function, this will pass the name of the sound file in either .wav or .mp3 format. Finally, with the help of “.play()” will give the command to play the sound.
In the end, we will run the mainloop for everything to get executed.
By following all these steps, we can create a GUI Piano!
Output:
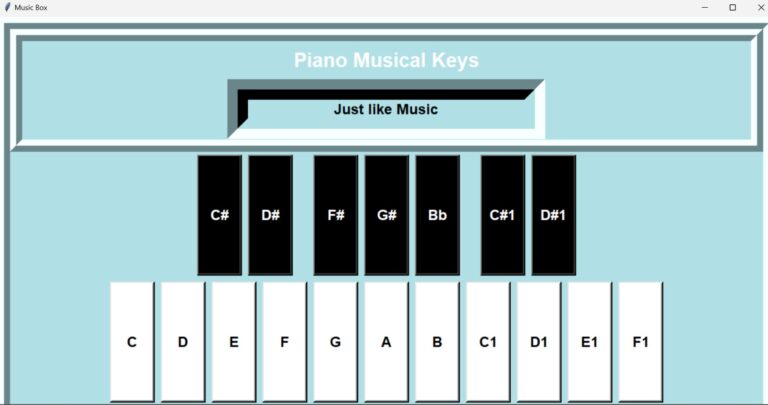
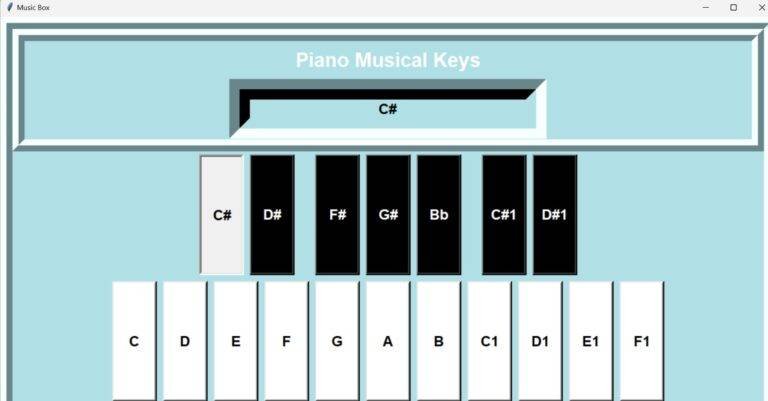
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …