NotePad Using Python
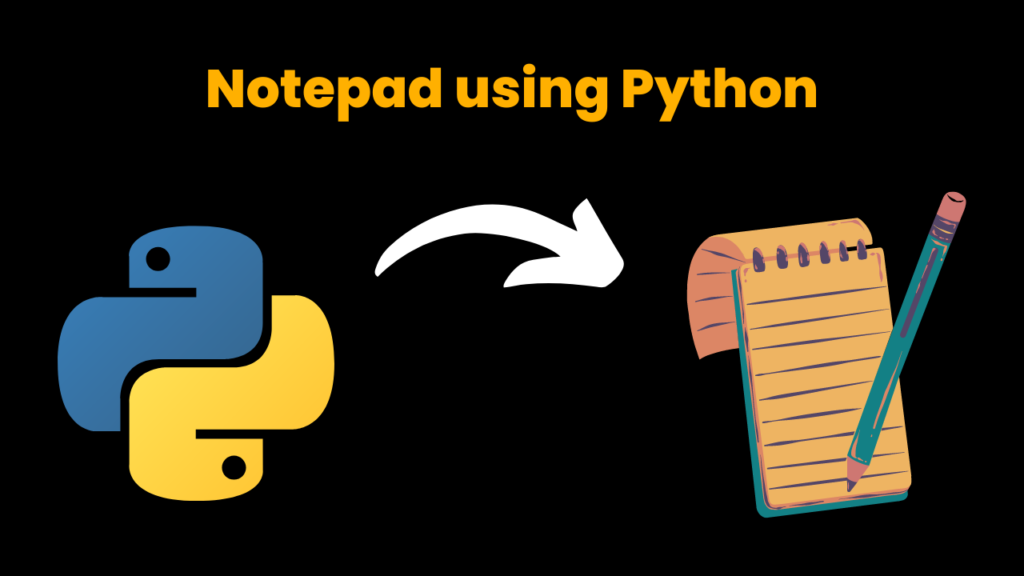
Introduction:
tkinter is a GUI library provided by Python to create GUI applications. In this project, with the help of this library, we are going to build up the notepad, a text editor. The notepad will have two main menu items: File & edit. The functionalities of these menu items will also be there.
Explanation:
First of all, we will import the tkinter library. With this, we will also import the “filedailog” module from tkinter which will provide the classes and factory functions for creating file selection windows.
Now, we will initiate a class named “Notepad ” by using the “class” keyword. During this process, we will pass the parameter as “tk. Tk” which will create the root object for our GUI application. Under this, we will define a “__init__” constructor with self,*args,**kwargs as parameters.
Under this constructor, we will define the title by using “.title” and create a Text widget with the help of the “.Text()” method and will make this widget fill the entire frame with the “.pack()” method.
For the notepad to show the menu bar we will create the menu widget with the help of “.Menu()”.
The functionalities provided by the File menu in this project will be: New, Open, save and exit.
and the functionalities provided by the edit menu will be: cut, copy, and paste
The following options will be created by using the “.add_cascade()” method. This method will create a new hierarchical menu by associating the given menu with the parent menu. While doing so, we will pass the user-defined functions which will carry the particular functionality, under the “command” parameter. By this, both main menu items will get created.
Source Code
# Find More Python Projects at Codewithcurious.com
import tkinter as tk
from tkinter import filedialog
class Notepad(tk.Tk):
def __init__(self, *args, **kwargs):
tk.Tk.__init__(self, *args, **kwargs)
# Set the title for the notepad
self.title("Notepad")
# Create a text widget
self.text = tk.Text(self, wrap="word")
self.text.pack(side="top", fill="both", expand=True)
# Create a menu bar
self.menu = tk.Menu(self)
self.config(menu=self.menu)
# Create a file menu
file_menu = tk.Menu(self.menu)
self.menu.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="New", command=self.new_file)
file_menu.add_command(label="Open", command=self.open_file)
file_menu.add_command(label="Save", command=self.save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=self.quit)
# Create an edit menu
edit_menu = tk.Menu(self.menu)
self.menu.add_cascade(label="Edit", menu=edit_menu)
edit_menu.add_command(label="Cut", command=self.cut)
edit_menu.add_command(label="Copy", command=self.copy)
edit_menu.add_command(label="Paste", command=self.paste)
def new_file(self):
self.text.delete("1.0", "end")
self.title("Notepad")
def open_file(self):
file = filedialog.askopenfile(parent=self, mode="rb", title="Open a file")
if file:
contents = file.read()
self.text.delete("1.0", "end")
self.text.insert("1.0", contents)
file.close()
self.title(file.name + " - Notepad")
def save_file(self):
file = filedialog.asksaveasfile(mode="w", defaultextension=".txt", filetypes=[("Text Documents", "*.txt"), ("All Files", "*.*")])
if file:
contents = self.text.get("1.0", "end")
file.write(contents)
file.close()
self.title(file.name + " - Notepad")
def cut(self):
self.text.event_generate("<>")
def copy(self):
self.text.event_generate("<>")
def paste(self):
self.text.event_generate("<>")
if __name__ == "__main__":
notepad = Notepad()
notepad.mainloop()
Let’s discuss all the user-defined functions which will be needed to add functionalities to this project.
- new_file(): To open the new file. For this, we will clear the content of the text widget by using “.delete()” and then will set the title again as Notepad.
- open_file(): To open the existing file. For this, we will make use of the “.askopenfile()” method of the filedialog module to show a dialog that allows a single file selection. After that, it will read the file by the “.read()” method and then give the functionality to insert the data by using the “.insert()” method.
- save_file(): To save the file. For this, we will make the save as dialog by using the “.asksaveasfile()” method, then to get the text for further processing will make use of “.get()” and then write the content of the file by using the “.write()” method and then set the title of the text editor with the addition of the file name.
- cut(): To remove the text. For this, we will generate a cut event that shows some action and bring the procedure of removal of text into existence by using the “.event_generate(<<event-name>>)” method.
- copy(): To copy the text. We will follow the same procedure for generating the event and then will pass the event name which we want to generate as Copy.
- paste(): To paste the text. For this as well, we will follow the same procedure for generating the event and name the event Paste
.
In the end, we will call the main method and under this method, we will create the object of the Notepad class named “notepad” and will run the mainloop by using “.mainloop()”.
By following the above-mentioned procedure, you can create Notepad!
Output:
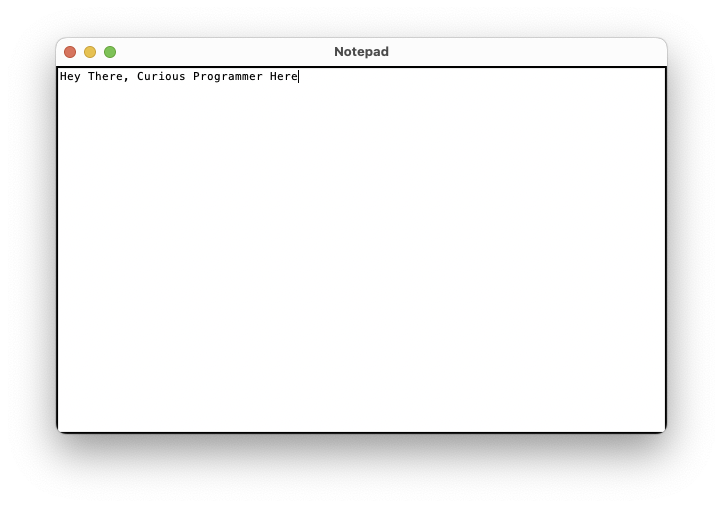
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …