Ping pong game using python
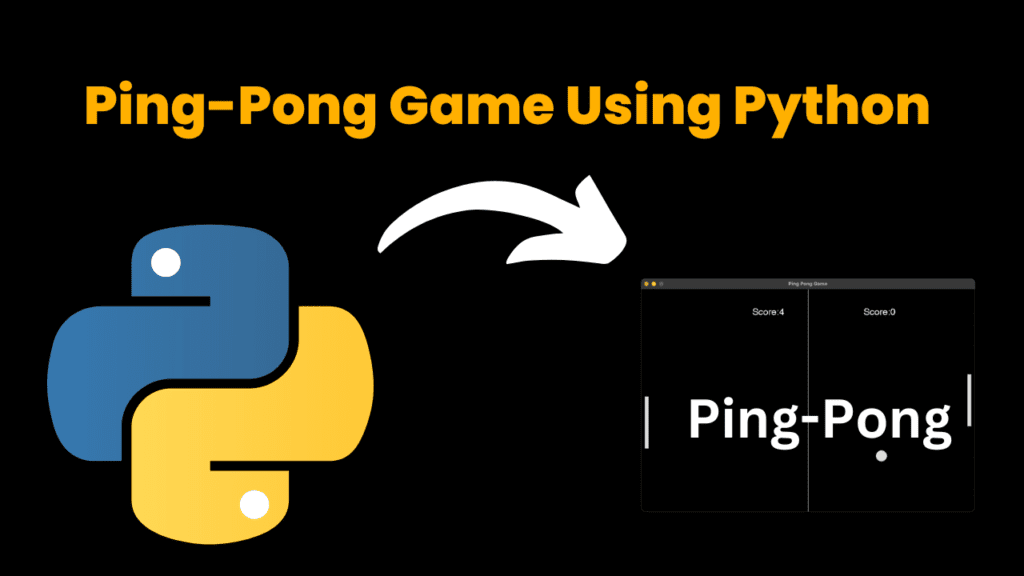
Introduction:
In this project, we have tried to create a ping pong game via using the “pygame” module of python. If we talk about the game then, in this game, there are two sides considered as player1 and player2, and a ball is present which needed to get hit by the paddle. If any one of the sides gets fails to hit the ball then the opposite side will score 1 point.
Source Code:
# Importing the library codewithcurious.com
import pygame
import sys
import random
import time
# Initialising the pygame
pygame.init()
# frames per second
c = pygame.time.Clock()
# Dimensions for window
width = 900
height = 600
# creating game window
screen = pygame.display.set_mode((width, height))
# Title and icon
pygame.display.set_caption("Ping Pong Game")
# Game rectangle
ball = pygame.Rect(width / 2 - 15, height / 2 - 15, 30, 30, )
player1 = pygame.Rect(width - 20, height / 2 - 70, 10, 140)
player2 = pygame.Rect(10, height / 2 - 70, 10, 140)
# Game variables
ball_speedx = 6 * random.choice((1, -1))
ball_speedy = 6 * random.choice((1, -1))
player1_speed = 0
player2_speed = 6
player1_score = 0
player2_score = 0
# Function for ball to move
def ball_movement():
global ball_speedx, ball_speedy, player1_score, player2_score
ball.x += ball_speedx
ball.y += ball_speedy
# Bouncing the ball
if ball.top <= 0 or ball.bottom >= height:
ball_speedy *= -1
if ball.left <= 0:
player1_score += 1
ball_restart()
if ball.right >= width:
player2_score += 1
ball_restart()
if ball.colliderect(player1) or ball.colliderect(player2):
ball_speedx *= -1
# function for the movement of player1
def player1_movement():
global player1_speed
player1.y += player1_speed
if player1.top <= 0:
player1.top = 0
if player1.bottom >= height:
player1.bottom = height
# function for the movement of player2
def player2_movement():
global player2_speed
if player2.top < ball.y:
player2.top += player2_speed
if player2.bottom > ball.y:
player2.bottom -= player2_speed
if player2.top <= 0:
player2_top = 0
if player2.bottom >= height:
player2.bottom = height
# Function to reset the ball position
def ball_restart():
global ball_speedx, ball_speedy
ball.center = (width / 2, height / 2)
ball_speedy *= random.choice((1, -1))
ball_speedx *= random.choice((1, -1))
# Font variable
font = pygame.font.SysFont("calibri", 25)
# Game Loop
while True:
for event in pygame.event.get():
# Checking for quit event
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# checking for key pressed event
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_DOWN:
player1_speed += 8
if event.key == pygame.K_UP:
player1_speed -= 8
# checking for key released event
if event.type == pygame.KEYUP:
if event.key == pygame.K_DOWN:
player1_speed -= 8
if event.key == pygame.K_UP:
player1_speed += 8
# calling the functions
ball_movement()
player1_movement()
player2_movement()
# setting the score condition
if ball.x < 0:
player1_score += 1
elif ball.x > width:
player2_score += 1
# Visuals
screen.fill((0, 0, 0))
pygame.draw.rect(screen, (220, 220, 220), player1)
pygame.draw.rect(screen, (220, 220, 220), player2)
pygame.draw.ellipse(screen, (220, 220, 220), ball)
pygame.draw.aaline(screen, (220, 220, 220), (width / 2, 0), (width / 2, height))
# To draw score font
player1_text = font.render("Score:" + str(player1_score), False, (255, 255, 255))
screen.blit(player1_text, [600, 50])
player2_text = font.render("Score:" + str(player2_score), False, (255, 255, 255))
screen.blit(player2_text, [300, 50])
# Updating the game window
pygame.display.update()
# 60 frames per second
c.tick(60)
Explanation:
→ The very first step is to import the modules and in this project, we have imported the following modules: “pygame”, “time”,” Sys”, and “random”. Then we will initialize the pygame module by using the “.init( )” method. Now, we have to set the frames per second to be shown with the help of “pygame.time.Clock()” which is available in the “time” module in python
→ The basic need to develop this project is to create a game window and with the help of “pygame. display.set_mode(width, height)” we will do so. Also to make it user-friendly we have set the title and icon for the game window via “pygame. display.set_caption( )” & “pygame.display.set_icon( )” methods respectively. To load the image in our project for icons we have used “pygame.image.load(file name)” method
→ Pygame uses rect objects to store and manipulate rectangular areas and we have considered every element of this project as a rectangle and thus for this, we have used “pygame. rect(left, top, width, height)”. With this, we have created the ball area, player1 area, and player2 area by specifying that particular coordinates. To get this area to be drawn on the screen we used “pygame.draw.rect(surface, color, value)” with this we created the player’s area and then “pygame.draw.ellipse(surface, color, value)” we have created ball and “pygame.draw.aaline(surface, color, parameter)” to draw a straight antialiased line.
→ Before talking about the main loop let’s discuss the objectives we need to cover under this loop to make this project
- First of all, we need to move the ball with a particular speed and for the same, we need to bounce the ball whenever it will get hit by any of the rectangular areas
- We need to code for the movement of both players
- The third step is to reset the position of the ball if hits the boundaries rather than the paddle
- The last step is to create a font variable and display the score by following the game rules
→ In this program, now we have created a user-defined function named “ball_movement()”. We are setting the scope of the variable as global so that the variables can be used throughout, in the first place we are just increasing the ball’s speed concerning x and y coordinates. Now, to bounce the ball we are checking the conditions that if the ball hits boundaries by .top and .bottom functions, then updating the speed following that axis. Under this code, we are also checking that if the opponent won’t be able to hit the ball then increase the score of that particular player, and thus after that we are calling the “ball_restart( )” function to reset the ball position.
→ Creating a UDF named as “player1_movement( )” is next in the program’s sequence. In this, we have just simply put the condition that the paddle should not move out of the screen. We have set the conditions for the top and bottom. The same process is followed by another UDF “player2_movement( )”
→ In the “ball_restart( )” function we have set the ball’s speed to be chosen randomly between and 1 and -1 by “.choice( )” method in the “random” module.
→ In the next step we have declared a font variable and set the font by using “pygame.font.SysFont(“font name”,” size”)” method of the “Sys” module
→ Until now, we have completed all the basic needs of our game and now the main step is to be followed, i.e. to create a game loop under which we will call all these functions to get executed
→ With the help of the “while” loop will create a game loop under which we will run this loop until we will get an event, to check the event condition we will use “pygame.event.get()” method. Under this, we will make use of the if-else ladder to get to know the type of event.
To do so we will use “.type”
→ Firstly we have checked the quit event, by “pygame. QUIT”, if the user has clicked the cross button of the game window then the window will get close
In the next series, we checked the event, if any keyboard keys are pressed or not for that we used “pygame. KEYDOWN”. Since the games will make use of arrow keys(down arrow key and up arrow key) and to check the arrow keys we have used “pygame.K_DOWN”, “pygame.K_UP”.
To check the event if the keyboard keys are released we make use of “pygame.KEYUP”
→ In the next step, we have called all the functions which we have made. We have set the scoring conditions for the game as well
→ To draw the score, firstly will render it with the help of “.render()” then we will draw it by using the “.blit(value, color)” function
→ In the end, we will give the command to update the mentioned changes in the game window by using the “pygame.display.update()”.
By using all of these functions and methods, and following all these steps, a simple ping-pong game is ready!
Output:
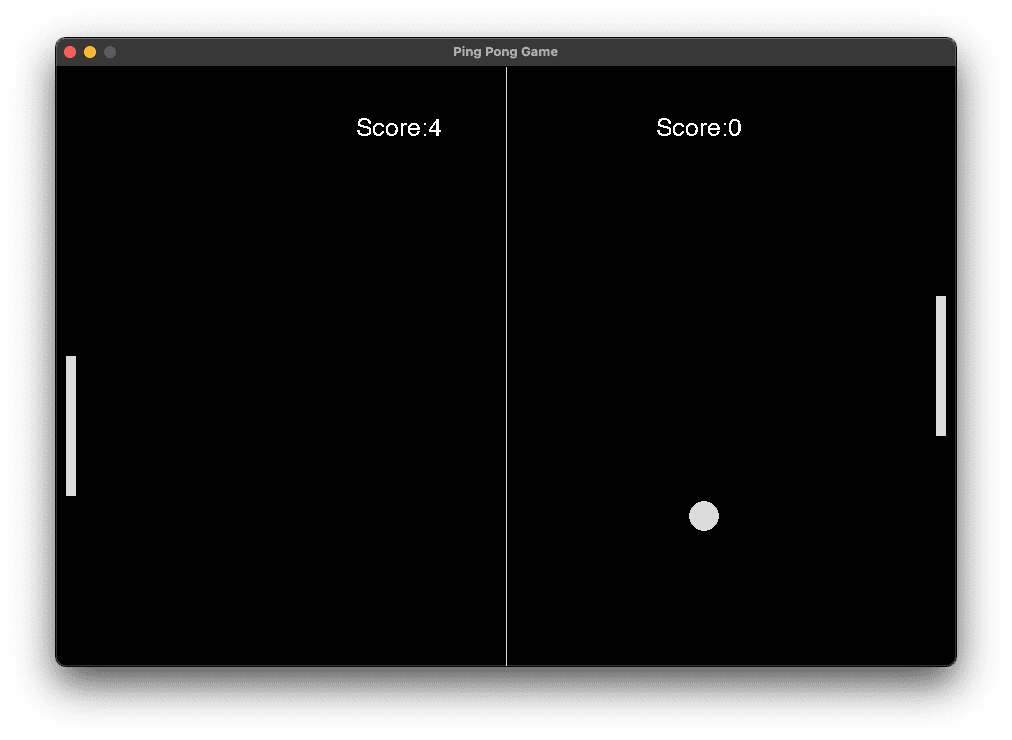
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …