Binary to Decimal & Decimal to Binary Converter Using Python With Source Code
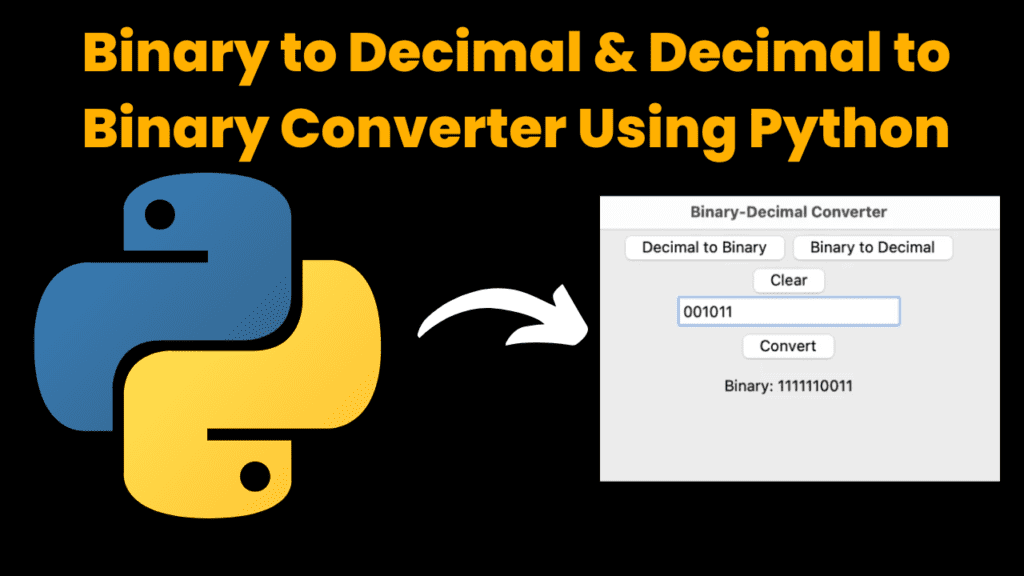
Introduction:
The “Binary-Decimal Converter” Python project is an ideal starting point for individuals venturing into the world of programming, especially those who are new to Python. This project introduces the basics of graphical user interface (GUI) development using the tkinter
library while offering a practical application in the form of a number conversion tool.
The project is structured intuitively, featuring clear buttons to select the conversion direction: “Decimal to Binary” and “Binary to Decimal”. These buttons act as the gateway to initiate the conversion process. The interface further comprises an input field where users can enter the number they wish to convert, followed by a “Convert” button that triggers the conversion calculation. Upon conversion, the result is displayed in an output label, providing immediate feedback.
One remarkable aspect of the project is its error handling. The system gracefully manages unexpected inputs, such as non-numeric characters or invalid binary digits, ensuring that the user experience remains frustration-free.
Required Modules & Modules Installation:
For the “Binary-Decimal Converter” project using the tkinter
library, you won’t need to install any additional modules because tkinter
is a standard library that comes with most Python installations. However, you can ensure that tkinter
is available on your system by trying to run a simple check.
Here’s how you can do it:
Check for
tkinter
Installation:Open a terminal or command prompt and type the following command:
python -m tkinter
If a window with a button appears (even if it’s a simple window), then
tkinter
is already installed and available on your system.If you get an error message, it might indicate that
tkinter
is not installed or accessible. In that case, you might need to install it based on your operating system.Install Python:
If you don’t have Python installed, make sure to download and install it from the official Python website: Python Downloads.
Install
tkinter
on Linux/Ubuntu:On some Linux distributions,
tkinter
might not be installed by default. You can install it using the following command:sudo apt-get install python3-tk
Install
tkinter
on macOS:tkinter
is typically included in the default Python installation on macOS. However, if you face any issues, you can use the built-in Python from Terminal:/usr/bin/python3 -m tkinter
If you’re using a virtual environment, make sure it’s configured to use the system’s Python installation that comes with
tkinter
Install
tkinter
on Windows:If you’re using Windows,
tkinter
should be available by default with your Python installation. If it’s not, you might need to repair your Python installation or ensure thattkinter
is selected during installation.
Source Code :
import tkinter as tk
def decimal_to_binary():
try:
decimal_num = int(input_field.get())
binary_num = bin(decimal_num).replace("0b", "")
output_label.config(text=f"Binary: {binary_num}")
except ValueError:
output_label.config(text="Invalid input. Please enter a decimal number.")
def binary_to_decimal():
try:
binary_num = input_field.get()
decimal_num = int(binary_num, 2)
output_label.config(text=f"Decimal: {decimal_num}")
except ValueError:
output_label.config(text="Invalid input. Please enter a valid binary number.")
def clear_output():
output_label.config(text="")
root = tk.Tk()
root.title("Binary-Decimal Converter")
menu_frame = tk.Frame(root)
menu_frame.pack()
decimal_to_binary_button = tk.Button(menu_frame, text="Decimal to Binary", command=decimal_to_binary)
decimal_to_binary_button.pack(side=tk.LEFT)
binary_to_decimal_button = tk.Button(menu_frame, text="Binary to Decimal", command=binary_to_decimal)
binary_to_decimal_button.pack(side=tk.LEFT)
clear_button = tk.Button(root, text="Clear", command=clear_output)
clear_button.pack()
input_field = tk.Entry(root)
input_field.pack()
convert_button = tk.Button(root, text="Convert", command=lambda: None) # Placeholder for now
convert_button.pack()
output_label = tk.Label(root, text="", pady=10)
output_label.pack()
root.mainloop()
Explanation:
Importing the Library:
At the beginning, we’re telling Python to use a special toolbox of code called
tkinter
. It’s like a kit that helps us make windows and buttons in our program.Functions for Conversion:
We define two functions:
decimal_to_binary()
: This function takes a regular number (decimal) and turns it into a binary number. For example, it changes 10 to “1010”.binary_to_decimal()
: This function takes a binary number and turns it into a regular number. So, “1010” becomes 10.
Clearing Output:
We also have a function called
clear_output()
. This one just cleans up the space where the result appears.Creating the Window:
We create the main window (the program’s screen) and give it a name, “Binary-Decimal Converter”.
Buttons for Conversion:
We make a little section at the top with buttons: “Decimal to Binary” and “Binary to Decimal”. These buttons will help us choose what kind of conversion we want to do.
Input Field:
Then we create a spot where you can type in a number. It’s like a text box.
Convert Button:
Next is a “Convert” button. Right now, it doesn’t do anything, but we’ll make it work soon.
Output Label:
Below that, there’s a spot where the result will show up. It starts out empty.
Putting Everything Together:
The
root.mainloop()
part keeps the window open. It’s like saying, “Hey, computer, show this window and let us click buttons and type things.”
Output:
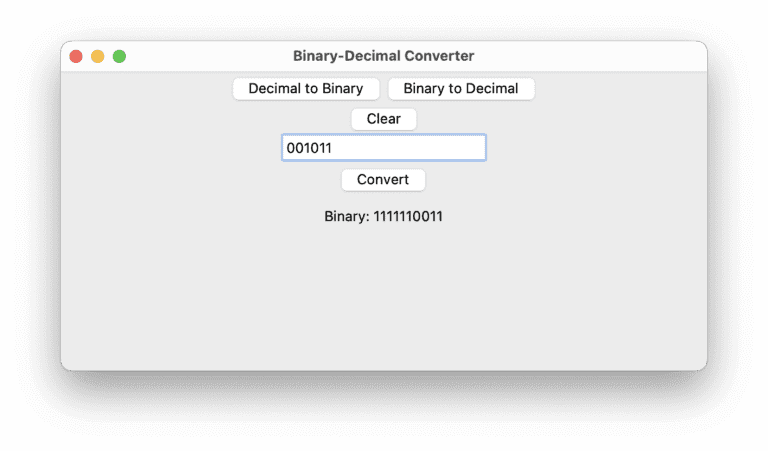
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …