Dairy management system using C++
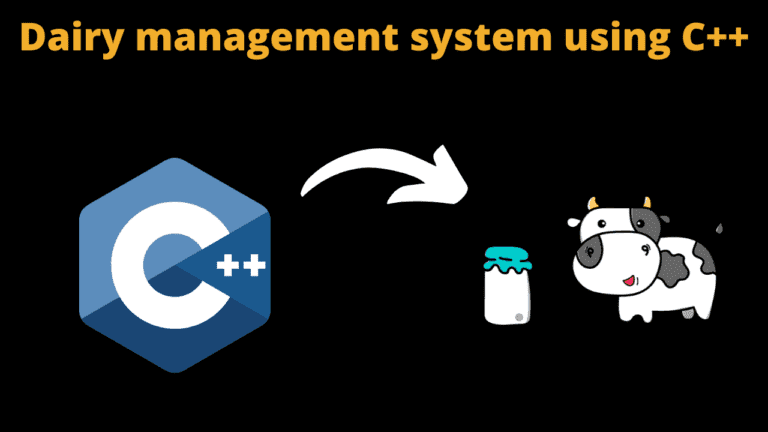
Introduction:
The “Dairy Management System” showcased here represents a cutting-edge C++ project designed to revolutionize the very core of your milk dairy enterprise. This exceptional system empowers you to masterfully oversee every crucial facet that ensures the seamless operation of your dairy. From meticulously tracking milk deposits to effortlessly managing animal meals, facilitating loan applications, and effortlessly sharing vital dairy-related information, this system paves the way for unparalleled dairy excellence.
Features:
1. Login System:
– Users are required to provide a username and a password to access the dairy management system.
– A predefined password (“3003”) is used for authentication. Successful login leads to access to the main menu.
2. Milk Deposit:
– Users can input information about milk deposits, including the name, animal type (cow/buffalo), quantity of milk, and milk fat.
– The system calculates the milk’s fat rate and the corresponding bill. The user receives a detailed bill receipt.
3. Main Menu:
– After successful login, users are presented with a main menu.
– The menu options include managing milk deposits, animal meal, loan applications, and learning more about the dairy.
4. Animal Meal:
– Users can select different types of animal meal products along with their quantities.
– The system calculates the total cost of the selected products and generates a bill receipt.
5. Loan Application:
– Users can apply for different types of loans: home loan, car loan, and agriculture loan.
– The system calculates the loan interest and generates a loan receipt detailing the loan amount, interest, total due, and monthly EMI.
6. About Dairy:
– This option provides information about the dairy, including its establishment date and various benefits for associates.
– Details about different loan options and associated benefits are also highlighted.
7. User-Friendly Interface:
– The system is designed with clear prompts and messages for user interaction.
– Menus and bill receipts are organized with appropriate formatting for easy readability.
8. Date and Time Display:
– The system displays the current date and time on the main menu to keep users informed.
9. Error Handling:
– The code includes error handling mechanisms, such as handling incorrect input values and providing error messages.
10. Looping Mechanism:
– The system allows users to navigate between different functionalities without exiting the program.
11. User-Friendly Prompts:
– Users are guided with prompts and instructions at each step to ensure smooth interaction.
Source code:
#include
#include
#include
using namespace std;
void login();
void deposit_milk();
void menu();
void meal();
void loan();
void about();
class info
{
public:
char name[50];
int no, fat;
float milk;
char day[10];
public:
void getdata()
{
cout << "\nEnter Your Name (without space): ";
cin >> name;
cout << "\nEnter Your No.: ";
cin >> no;
cout << "\nEnter Morning OR Evening: ";
cin >> day;
cout << "\nEnter Milk in liters: ";
cin >> milk;
cout << "\nEnter Milk fat: ";
cin >> fat;
}
void putdata();
};
void info::putdata()
{
float fat_rate = 6.43, total;
float fat_t = (fat * fat_rate);
total = (fat * fat_rate) * milk;
system("cls");
cout << "\n\tYOUR BILL-";
cout << "\n\t\t ====================================";
cout << "\n\t\t| SUNITA milk dairy |" << endl;
cout << "\n\t\t Name : " << name << " No:" << no << endl;
cout << "\n\t\t Milk session: " << day << endl;
cout << "\n\t\t Milk : " << milk << " Litre"
<< " Fat: " << fat << endl;
cout << "\n\t\t| Fat rate: " << fat_t << " Total: " << total << "rs |";
cout << "\n\t\t ====================================" << endl;
}
char user[50];
int pass;
int i, opt;
int main()
{
login();
menu();
}
void login()
{
cout << "\n\n\t\t\t|--------------LOGIN----------------|" << endl;
cout << "\n\t\t\t Enter User_name: ";
cin >> user;
cout << "\n\t\t\t Enter Password[3003]: ";
cin >> pass;
cout << "\n\t\t\t|-----------------------------------|" << endl;
if (pass == 3003)
{
system("cls");
cout << "\n\tSuccessfully logged in";
for (int i = 0; i < 10; i++)
{
cout << ".";
Sleep(250);
}
Beep(523, 900);
}
else
{
cout << "\nERROR.....! PLEASE CHECK YOUR PASSWORD.";
main();
}
}
void deposit_milk()
{
info n;
int animal;
char back;
cout << "\n\n__________________________________________\n";
cout << "\nEnter your Milk animal NO. from below";
cout << "\n\n\t|1. BUFFALO |2. COW |\n";
cin >> animal;
if (animal == 1)
cout << "\nYou choose 'BUFFALO' \n";
else if (animal != 1 && animal != 2)
{
cout << "\nError! Choose only option [1/2] ....." << endl;
Sleep(2500);
system("cls");
deposit_milk();
}
else
cout << "\nYou choose 'COW' \n";
n.getdata();
n.putdata();
cout << "\nWant to go back to the Menu [y/n]-";
cin >> back;
if (back == 'y' || back == 'Y')
{
menu();
}
else
{
deposit_milk();
}
}
void menu()
{
system("cls");
cout << "\n\n\t\tWelcome to SUNITA milk dairy [ " << user << " ]";
time_t my_time = time(NULL);
cout << "\t DATE: " << ctime(&my_time);
cout << "\n\n1. Milk";
cout << "\n2. Animal MEAL";
cout << "\n3. Apply loan";
cout << "\n4. About our Dairy";
cout << "\n5. Logout";
cout << "\n\nEnter option: ";
cin >> opt;
switch (opt)
{
case 1:
deposit_milk();
break;
case 2:
meal();
break;
case 3:
loan();
break;
case 4:
about();
break;
case 5:
cout << "\n\t\t\tThank you | keep visiting";
exit(0);
default:
break;
}
}
void meal()
{
system("cls");
long int prod, how;
char go;
long int totalval;
cout << "\n\t\t\t-- OUR ANIMAL MEAL PRODUCTS --";
cout << "\n\t\t ============================================";
cout << "\n\t\t| 1.Mahalakshmi Animal MEAL - 1100rs |";
cout << "\n\t\t| 2.Mahalakshmi Bhusa - 1000rs |";
cout << "\n\t\t| 3.For growth in Milk-Products - 350rs |";
cout << "\n\t\t ============================================";
cout << "\n\n\t*Enter Any Above Option for buying Products: ";
cin >> prod;
switch (prod)
{
case 1:
cout << "\n\n\tYou choose - Mahalakshmi Animal MEAL";
cout << "\n\n\tEnter how many meal bags you want :- ";
cin >> how;
totalval = 1100 * how;
cout << "\n\tYour Bill-";
cout << "\n\t\t _________________________________________";
cout << "\n\t\t| SUNITA MILK DAIRY |";
cout << "\n\n\t\t Product name Quantity ";
cout << "\n\n\t\t *Mahalakshmi Animal MEAL " << how;
cout << "\n\n\t\t Total Bill-" << totalval << "rs";
cout << "\n\t\t|_________________________________________|";
break;
case 2:
cout << "\nYou choose - Mahalakshmi Bhusa ";
cout << "\n\nEnter how many bhusa bags you want :- ";
cin >> how;
totalval = 1000 * how;
cout << "\n\tYour Bill-";
cout << "\n\t\t _________________________________________";
cout << "\n\t\t| SUNITA MILK DAIRY |";
cout << "\n\n\t\t Product name Quantity ";
cout << "\n\n\t\t *Mahalakshmi Animal Bhusa " << how;
cout << "\n\n\t\t Total Bill-" << totalval << "rs";
cout << "\n\t\t|_________________________________________|";
break;
case 3:
cout << "\nYou choose - Milk growth products ";
cout << "\n\nEnter how many product you want :- ";
cin >> how;
totalval = 350 * how;
cout << "\n\tYour Bill-";
cout << "\n\t\t _________________________________________";
cout << "\n\t\t| SUNITA MILK DAIRY |";
cout << "\n\n\t\t Product name Quantity ";
cout << "\n\n\t\t *growth in Milk-Product " << how;
cout << "\n\n\t\t Total Bill-" << totalval << "rs";
cout << "\n\t\t|_________________________________________|";
break;
default:
cout << "\n\t\tPlz Enter option between 1 to 3";
Sleep(1000);
meal();
break;
}
cout << "\n\nWant to go back to the main menu [y/n] : ";
cin >> go;
if (go == 'y' || go == 'Y')
menu();
else
meal();
}
void loan()
{
int loanopt;
long long int principal, tenure, emi;
long long int interest, totaldue;
char goback;
system("cls");
cout << "\n\t\t ----*loan*-----------*rate*----";
cout << "\n\t\t| 1.Home loan 12% |";
cout << "\n\t\t| 2.Car loan 10% |";
cout << "\n\t\t| 3.Agriculture loan 7% |";
cout << "\n\t\t -------------------------------";
cout << "\n\tEnter which loan option you choose: ";
cin >> loanopt;
switch (loanopt)
{
case 1:
cout << "\n\nYou Choose- Home loan";
cout << "\n\nEnter loan amount: ";
cin >> principal;
if (principal > 1000000)
{
cout << "\n\t\tError! Maximum amount of loan is 10,00,000 rs";
Sleep(2600);
loan();
}
cout << "\nEnter tenure(in years): ";
cin >> tenure;
interest = (principal * 12 * tenure) / 100;
totaldue = principal + interest;
emi = totaldue / (tenure * 12);
cout << "\n\tYour Loan receipt-";
cout << "\n\t\t _________________________________________";
cout << "\n\t\t| SUNITA MILK DAIRY-- |";
cout << "\n\n\t\t Home loan[on 12%] -" << principal << "rs";
cout << "\n\n\t\t Interest -" << interest << "rs";
cout << "\n\n\t\t Total loan due -" << totaldue << "rs";
cout << "\n\n\t\t YOUR EMI -" << emi << "rs";
cout << "\n\t\t|_________________________________________|";
break;
case 2:
cout << "\n\nYou Choose- Car loan";
cout << "\n\nEnter loan amount: ";
cin >> principal;
if (principal > 1000000)
{
cout << "\nMaximum amount of loan is 10,00,000 rs";
Sleep(2600);
loan();
}
cout << "\nEnter tenure(in years): ";
cin >> tenure;
interest = (principal * 10 * tenure) / 100;
totaldue = principal + interest;
emi = totaldue / (tenure * 10);
cout << "\n\tYour Loan receipt-";
cout << "\n\t\t _________________________________________";
cout << "\n\t\t| SUNITA MILK DAIRY-- |";
cout << "\n\n\t\t Car loan[on 10%] -" << principal << "rs";
cout << "\n\n\t\t Interest -" << interest << "rs";
cout << "\n\n\t\t Total loan due -" << totaldue << "rs";
cout << "\n\n\t\t YOUR EMI -" << emi << "rs";
cout << "\n\t\t|_________________________________________|";
break;
case 3:
cout << "\n\nYou Choose- Agriculture loan";
cout << "\n\nEnter loan amount: ";
cin >> principal;
if (principal > 1000000)
{
cout << "\nMaximum amount of loan is 10,00,000 rs";
Sleep(2600);
loan();
}
cout << "\nEnter tenure(in years): ";
cin >> tenure;
interest = (principal * 7 * tenure) / 100;
totaldue = principal + interest;
emi = totaldue / (tenure * 7);
cout << "\n\tYour Loan receipt-";
cout << "\n\t\t _________________________________________";
cout << "\n\t\t| SUNITA MILK DAIRY-- |";
cout << "\n\n\t\t Agriculture loan[on 7%] -" << principal << "rs";
cout << "\n\n\t\t Interest -" << interest << "rs";
cout << "\n\n\t\t Total loan due -" << totaldue << "rs";
cout << "\n\n\t\t YOUR EMI -" << emi << "rs";
cout << "\n\t\t|_________________________________________|";
break;
default:
cout << "\nEnter option between 1 to 3";
Sleep(1000);
loan();
break;
}
cout << "\n\nWant to go back to the main menu [y/n] : ";
cin >> goback;
if (goback == 'y' || goback == 'Y')
menu();
else
loan();
}
void about()
{
system("cls");
cout << "\n\n\t\tAbout SUNITA milk dairy";
cout << "\n\t\t ============================================";
cout << "\n\t\t| SUNITA milk dairy is a leading dairy |";
cout << "\n\t\t| in the region, providing quality dairy |";
cout << "\n\t\t| products to our customers. We are |";
cout << "\n\t\t| committed to delivering fresh and |";
cout << "\n\t\t| nutritious milk products. |";
cout << "\n\t\t ============================================";
cout << "\n\nPress 'M' to go back to the main menu: ";
char back;
cin >> back;
if (back == 'M' || back == 'm')
menu();
else
about();
}
Explanation:
Header Files:
#include <iostream> #include <windows.h> #include <ctime>
These lines include necessary header files for input/output (
iostream
), Windows-specific functions (windows.h
forSleep
andBeep
), and time-related functions (ctime
for getting the current time).Function Prototypes:
void login(); void deposit_milk(); void menu(); void meal(); void loan(); void about();
These lines declare the prototypes of functions that will be defined later in the code. These functions handle different aspects of a milk dairy management system.
Class
info
:class info { public: char name[50]; int no, fat; float milk; char day[10]; public: void getdata(); void putdata(); };
This class
info
is used to store information about a milk deposit, including the customer’s name, customer number, milk fat percentage, milk quantity, and whether it’s morning or evening milk. It also contains member functionsgetdata
andputdata
for inputting and displaying this information.info
Member Function Definitions:void info::getdata() { ... } void info::putdata() { ... }
These functions are the implementations of the
getdata
andputdata
member functions of theinfo
class. They allow you to input and display customer milk deposit information.Global Variables:
char user[50]; int pass; int i, opt;
These are global variables used throughout the program to store the user’s name, password, an integer
i
, and an optionopt
for menu choices.main()
Function:int main() { login(); menu(); }
The
main
function is the entry point of the program. It calls thelogin
function to authenticate the user and then proceeds to themenu
function, which displays the main menu.login()
Function:void login() { ... }
This function handles user authentication by asking for a username and password. If the correct password (3003) is entered, it clears the screen, displays a success message, and makes a beeping sound. Otherwise, it shows an error message and recalls
main()
.deposit_milk()
Function:void deposit_milk() { ... }
This function allows users to deposit milk by choosing whether it’s from a buffalo or cow and entering relevant information about the milk deposit. It then displays a bill and asks if the user wants to go back to the main menu or continue depositing milk.
menu()
Function:void menu() { ... }
The
menu
function displays the main menu of options for the user, such as depositing milk, ordering animal meal, applying for a loan, viewing information about the dairy, and logging out. It uses aswitch
statement to handle different menu choices.meal()
Function:void meal() { ... }
This function handles ordering animal meal products. It presents a menu of meal options, calculates the total cost based on the quantity selected, and displays a bill. It also allows the user to return to the main menu.
loan()
Function:void loan() { ... }
The
loan
function handles loan applications. It offers different types of loans (home loan, car loan, agriculture loan) with different interest rates. It calculates the total loan amount, interest, and monthly EMI. Users can choose a loan type, input loan details, and view their loan receipt.about()
Function:void about() { ... }
The
about
function displays information about the SUNITA milk dairy. It provides a brief description of the dairy and allows users to return to the main menu by pressing ‘M’.
Code Functions:
1. `void login()`:
– This function handles the user authentication process.
– It prompts the user for a username and password.
– If the password is correct (3003), it displays a success message with a loading animation and a beep sound.
– If the password is incorrect, it displays an error message and recursively calls itself for the user to retry.
2. `void deposite_milk()`:
– This function allows users to input details about milk deposits.
– Users select the type of animal (buffalo or cow) for which milk is being deposited.
– It creates an instance of the `info` class, gathers input data like name, animal type, milk quantity, and fat content.
– It then displays a bill using the `putdata` function of the `info` class and offers an option to return to the main menu.
3. `void menu()`:
– This function displays the main menu of the dairy management system.
– It shows options like managing milk deposits, animal meal, loan applications, dairy information, and logout.
– Users input a choice, and based on the choice, the corresponding function is called (e.g., `deposite_milk`, `meal`, `loan`, `about`), or the program exits if the user chooses to logout.
4. `void meal()`:
– This function allows users to purchase animal meal products.
– It displays available meal options along with their prices.
– Users choose a product and specify the quantity they want to purchase.
– The function calculates the total cost, displays a bill receipt, and offers an option to return to the main menu.
5. `void loan()`:
– This function provides loan options and calculates loan details.
– It presents loan options like home loan, car loan, and agriculture loan, along with their interest rates.
– Users choose a loan option and input the loan amount and tenure.
– The function calculates interest, total due amount, and monthly EMI. It then displays a loan receipt and offers an option to return to the main menu.
6. `void about()`:
– This function displays information about the dairy establishment, benefits, and loan options.
– It provides details about the dairy’s services, benefits for associates, and available loan options.
– Users can choose to return to the main menu or continue reading.
7. `int main()`:
– The main function initializes the program execution.
– It starts by calling the `login` function to authenticate the user.
– If the login is successful, it proceeds to the `menu` function to display the main menu and handle user interactions.
These functions collectively create an interactive dairy management system where users can log in, manage milk deposits, purchase animal meal, apply for loans, and access information about the dairy. The program showcases basic control flow, user input, function calls, and class usage to simulate dairy management operations.
Output:
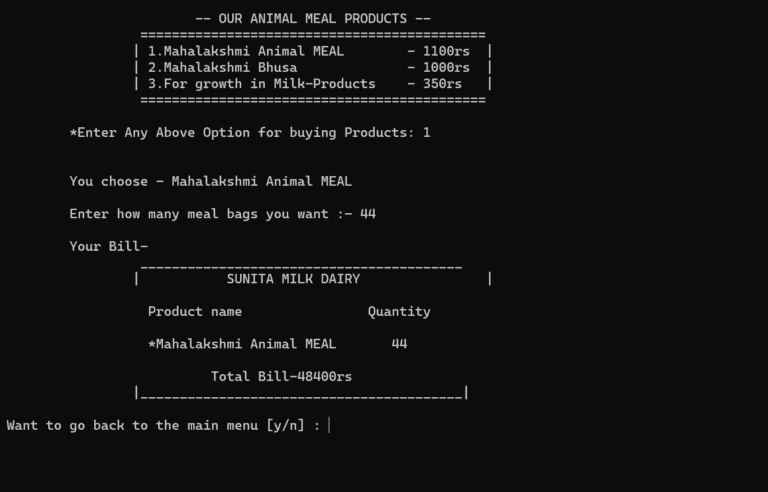
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …