Easy projects in python- Beginner friendly
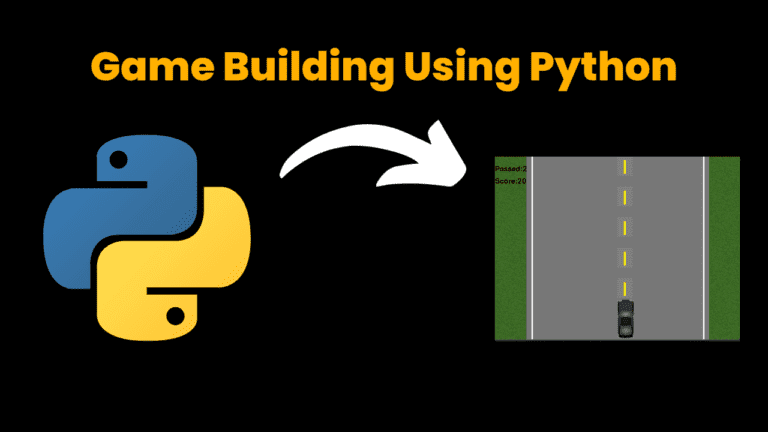
Introduction :
One of the best ways to learn Python is by working on mini-projects that can be completed in a short period of time. They are a great way to practice your coding skills and learn new concepts.
In this blog, we will discuss a simple Python project of rock paper scissors – first make it on a terminal then on a web page, that is finally into a web app.
So gear up your IDE and lets march!
Explanation :
Starting with the basic program that would output on your editors terminal :
1. The code starts by initializing two variables, `count_h` and `count_c`, which will keep track of the number of wins for the human player and the computer player, respectively.
2. The `keep_playing` variable is set to `True` to start a loop that will allow the player to play multiple rounds of the game.
3. The computer’s move is generated using `random.choice([‘rock’, ‘paper’, ‘scissors’])`, which randomly selects one of the three options: rock, paper, or scissors. The human player’s move is also randomly generated using the same method and stored in the variable `h_choice`.
4. The code checks the different combinations of choices to determine the winner. After each round, the code asks the player if they want to play again.
5. If the player answers “no,” indicating they don’t want to play again, the `keep_playing` variable is set to `False`, and the code prints the final scores for both the computer and the human and displays the result.
That’s it! The code allows you to play multiple rounds of Rock, Paper, Scissors against the computer and keeps track of the scores.
Source Code :
#Simple automated game in python where
#computer randomly chooses for both players
count_h =0
count_c =0
print("__________________________Rock...Paper...Scissors__________________________\n")
print("Hey! Welcome to the game Lets begin...\n")
keep_playing = True
while keep_playing:
import random
c_choice = random.choice(['rock', 'paper', 'scissors'])
print('the computer chooses ' + c_choice)
h_choice = random.choice(['rock', 'paper', 'scissors'])
print('human chooses ' + h_choice)
if((h_choice=='rock' and c_choice=='scissors') or (h_choice=='scissors' and c_choice=='paper') or(h_choice=='paper' and c_choice=='rock')):
print("human wins")
count_h += 1
elif(h_choice == c_choice):
print("draw")
else :
print("computer wins")
count_c += 1
print("\nDo you want to play again?")
answer = input()
if answer == "no":
keep_playing = False
print("\nThanks for playing!")
print("Computer score is:"+str(count_c))
print("Human score is :" + str(count_h))
print("\nOverall results:")
if count_c > count_h:
print("Better luck next time!\n")
elif count_c == count_h:
print("It is a draw\n")
else :
print("You win!\n")
print("_________________________Rock...Paper...Scissors_________________________")
Output :
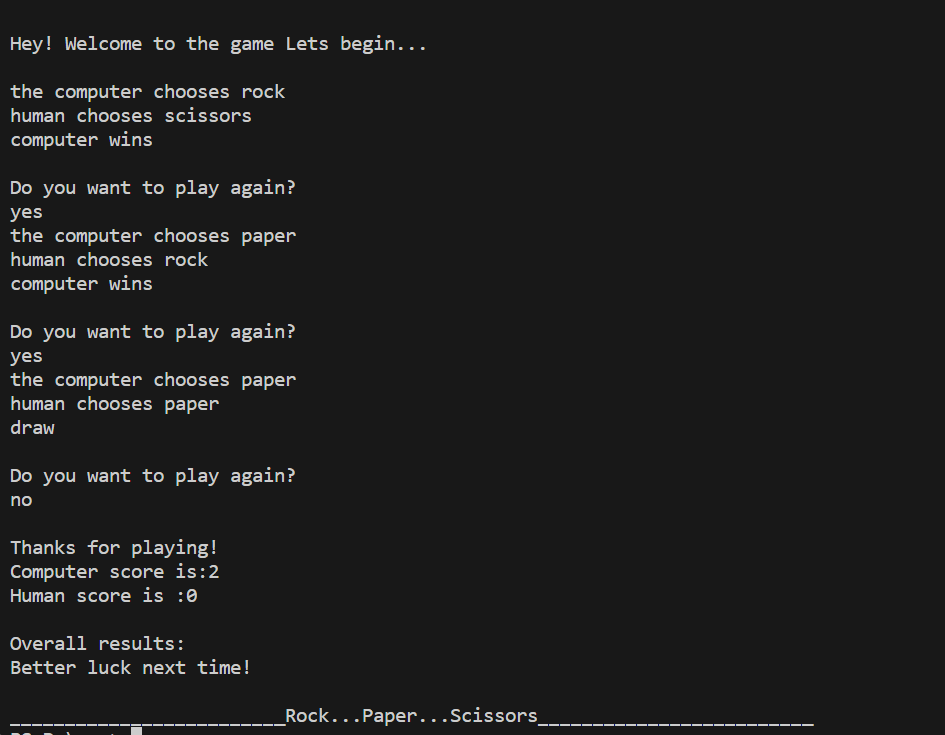
Explanation :
Next, now we will learn how to deploy this on a web page.
For this, we will need to create a folder named templates where we can store HTML files – index.html and results.html. Also, create a new file called app.py as the above code needs to be transformed.
The index file lets you choose the options and the results file will display the outputs.
Now you will need to install Flask to run your web page on localhost. For this run the following command in the terminal: pip install flask
Now, in the app.py file:
1. The code begins by importing the necessary modules: `Flask` for creating the web application, `render_template` for rendering HTML templates, and `request` for handling HTTP requests.
2. An instance of the `Flask` class is created and assigned to the variable `app`. This will represent our web application.
3. The `@app.route(‘/’)` decorator is used to define a route for the root URL (“/”). When a user visits this URL, the `index()` function is called.
4. The `index()` function returns the result of the `render_template()` function. It renders an HTML template called ‘index.html’, which will be displayed in the user’s web browser.
5. Another route is defined using the `@app.route(‘/play’, methods=[‘POST’])` decorator. This route is accessed when the user submits a form on the webpage. The method specified as `POST` indicates that the route will handle POST requests.
6. The `play()` function is called when the ‘/play’ route is accessed. Inside this function, the user’s choice is retrieved from the submitted form using `request.form[‘choice’]`. The value entered in the form field with the name ‘choice’ will be assigned to the `player_choice` variable.
7. A list called `choices` is created, containing the options ‘rock’, ‘paper’, and ‘scissors’.
8. The computer’s choice is randomly selected using `random.choice(choices)`. The chosen option is stored in the `computer_choice` variable.
9. The code then determines the winner based on the choices made by the player and the computer. It uses a series of `if` and `elif` statements to compare the choices and assign the appropriate result message to the `result` variable.
10. Finally, the `render_template()` function is called with ‘result.html’ as the template name. It passes the player’s choice, computer’s choice, and the result message as arguments. This template will be rendered and displayed in the user’s browser, showing the game’s outcome.
11. The `if __name__ == ‘__main__’:` condition ensures that the app is only run if the script is executed directly (not imported as a module).
12. The `app.run(debug=True)` statement starts the Flask development server. It runs the application and allows it to be accessed through a local host address. The `debug=True` parameter enables debug mode, which provides more detailed error messages if something goes wrong.
That’s it! To see your page working, run the following command in the terminal: python app.py
Remember to style it with a CSS file to make it look fabulous !
Source Code:
Rock Paper Scissors
Rock Paper Scissors
Rock
Paper
Scissors
Result
Result
You chose: {{ player }}
The computer chose: {{ computer }}
{{ result }}
Play again
#app.py
from flask import Flask, render_template, request
import random
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/play', methods=['POST'])
def play():
player_choice = request.form['choice']
choices = ['rock', 'paper', 'scissors']
computer_choice = random.choice(choices)
# Determine the winner
if player_choice == computer_choice:
result = "It's a tie!"
elif (
(player_choice == 'rock' and computer_choice == 'scissors') or
(player_choice == 'paper' and computer_choice == 'rock') or
(player_choice == 'scissors' and computer_choice == 'paper')
):
result = "You win!"
else:
result = "You lose!"
return render_template('result.html', player=player_choice, computer=computer_choice, result=result)
if __name__ == '__main__':
app.run(debug=True)
Output:
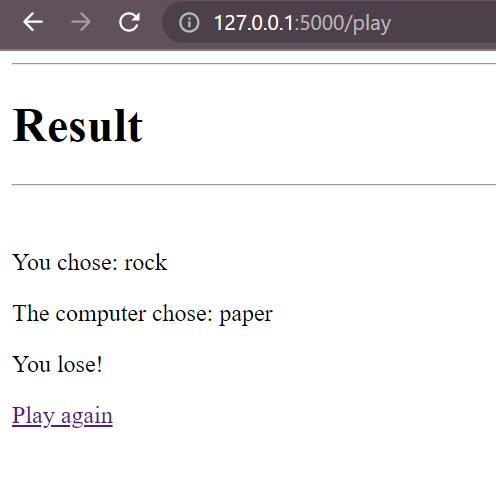
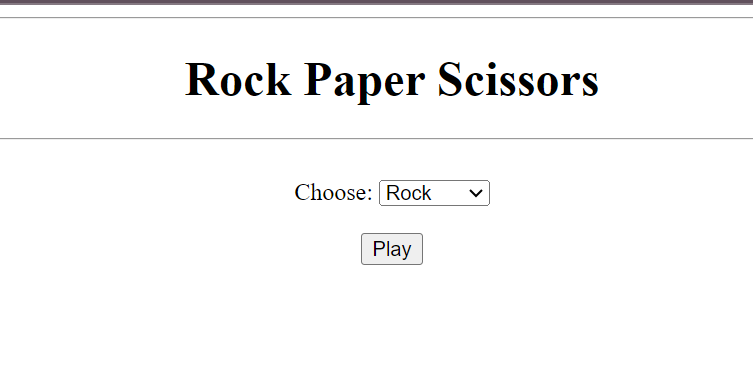
Other Projects :
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …