Draw Doraemon With Python
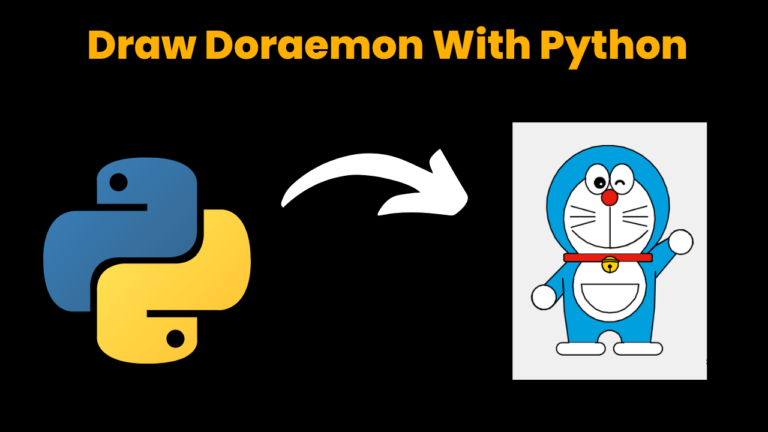
Introduction:
In Python, the turtle is one of the most interesting libraries which enables the user to create pictures on a virtual canvas, with the help of the in-built functions of this library we can see the magic of art. In this project, with the help of this library, we will create a cartoon character “DOREMON”.
Explanation:
To draw any picture, we need to first look at all the small elements which will get combined to form a complete picture that matches our imagination. In the same way, for this project as well, first of all, we will code for all the small elements in various functions accordingly and then will combine them into another function which will sum up all these to get the picture of the cartoon character.
The basic need for drawing this character is to draw
- The Head
- The eyes
- The mouth
- The band
- The whiskers
- The band
- The nose
- The face
- The body
The first function we made in this project is “my_goto(x,y)”. This function will help the turtle( The onscreen pen used for drawing is called turtle) to move at the specified location x,y. Under this function, we made use of two more functions “penup()” & “pendown ()”. The penup() function means that no line will be drawn when the pen moves and the pendown() function means that a line will be drawn when the pen moves. Overall this UDF says that when the function gets called the pen will move to a desired location given by the user and after that, it must draw at that particular position. Now you all are wondering how we should specify the location. So for these, we make use of coordinates. The screen is divided into four quadrants and accordingly, we give the location.
Source Code:
# find more python project source code at Codewithcurious.com
# Importing the library
from turtle import *
# function for position
def my_goto(x, y):
penup()
goto(x, y)
pendown()
# Function for drawing eyes
def eyes():
fillcolor("#ffffff")
begin_fill()
tracer(False)
a = 2.5
for i in range(120):
if 0 <= i < 30 or 60 <= i < 90:
a -= 0.05
lt(3)
fd(a)
else:
a += 0.05
lt(3)
fd(a)
tracer(True)
end_fill()
# Function for drawing whisker
def whisker():
my_goto(-32, 135)
seth(165)
fd(60)
my_goto(-32, 125)
seth(180)
fd(60)
my_goto(-32, 115)
seth(195)
fd(60)
my_goto(37, 135)
seth(15)
fd(60)
my_goto(37, 125)
seth(0)
fd(60)
my_goto(37, 115)
seth(-13)
fd(60)
# Function for drawing mouth
def mouth():
my_goto(5, 148)
seth(270)
fd(100)
seth(0)
circle(120, 50)
seth(230)
circle(-120, 100)
# Function for drawing band
def band():
fillcolor("#e70010")
begin_fill()
seth(0)
fd(200)
circle(-5, 90)
fd(10)
circle(-5, 90)
fd(207)
circle(-5, 90)
fd(10)
circle(-5, 90)
end_fill()
# Function for drawing nose
def nose():
my_goto(-10, 158)
seth(315)
fillcolor("#e70010")
begin_fill()
circle(20)
end_fill()
# Functin for drawing black eyes
def black_eyes():
seth(0)
my_goto(-20, 195)
fillcolor("#000000")
begin_fill()
circle(13)
end_fill()
pensize(6)
my_goto(20, 205)
seth(75)
circle(-10, 150)
pensize(3)
# for drawing the white circle inside
my_goto(-17, 200)
seth(0)
fillcolor("#ffffff")
begin_fill()
circle(5)
end_fill()
my_goto(0, 0)
# Function for drawing face
def face():
fd(183)
lt(45)
fillcolor("#ffffff")
begin_fill()
circle(120, 100)
seth(180)
fd(121)
pendown()
seth(215)
circle(120, 100)
end_fill()
my_goto(63.56, 218.24)
seth(90)
eyes()
seth(180)
penup()
fd(60)
pendown()
seth(90)
eyes()
penup()
seth(180)
fd(64)
# Function for drawing head
def head():
penup()
circle(150, 40)
pendown()
fillcolor("#00a0de")
begin_fill()
circle(150, 280)
end_fill()
# Combining all functions to one
def Doremon():
head()
band()
face()
nose()
mouth()
whisker()
# For drawing the body outline
my_goto(0, 0)
seth(0)
penup()
circle(150, 50)
pendown()
seth(30)
fd(40)
seth(70)
circle(-30, 270)
# For filling the body color
fillcolor("#00a0de")
begin_fill()
seth(230)
fd(80)
seth(90)
circle(1000, 1)
seth(-89)
circle(-1000, 10)
seth(180)
fd(70)
seth(90)
circle(30, 180)
seth(180)
fd(70)
seth(100)
circle(-1000, 9)
seth(-86)
circle(1000, 2)
seth(230)
fd(40)
circle(-30, 230)
seth(45)
fd(81)
seth(0)
fd(203)
circle(5, 90)
fd(10)
circle(5, 90)
fd(7)
seth(40)
circle(150, 10)
seth(30)
fd(40)
end_fill()
# For filling right palm color
seth(70)
fillcolor("#ffffff")
begin_fill()
circle(-30)
end_fill()
# For filling left foot color
my_goto(103.74, -182.59)
seth(0)
fillcolor("#ffffff")
begin_fill()
fd(15)
circle(-15, 180)
fd(90)
circle(-15, 180)
fd(10)
end_fill()
# For filling right foot color
my_goto(-96.26, -182.59)
seth(180)
fillcolor("#ffffff")
begin_fill()
fd(15)
circle(15, 180)
fd(90)
circle(15, 180)
fd(10)
end_fill()
# For filling left palm color
my_goto(-184.67, -61.59)
seth(70)
fillcolor("#ffffff")
begin_fill()
circle(-30)
end_fill()
# For drawing the inner body circle
my_goto(-103.42, 15.09)
seth(0)
fd(38)
seth(230)
begin_fill()
circle(90, 260)
end_fill()
# For drawing the semicircle
my_goto(5, -40)
seth(0)
fd(70)
seth(-90)
circle(-70, 180)
seth(0)
fd(70)
# For drawing the bell
my_goto(-103.42, 15.09)
fd(90)
seth(70)
fillcolor("#ffd200")
begin_fill()
circle(-20)
end_fill()
seth(170)
fillcolor("#ffd200")
begin_fill()
circle(-2, 180)
seth(10)
circle(-100, 22)
circle(-2, 180)
seth(170)
circle(100, 22)
end_fill()
goto(-13.42, 15.09)
seth(250)
circle(20, 110)
seth(90)
fd(15)
dot(10)
my_goto(0, 150)
black_eyes()
# Main function
if __name__ == '__main__':
# Window control
screensize(800, 600, "#f0f0f0")
screen = Screen()
screen.setup(width=1.0, height=1.0)
# Setting teh pen size
pensize(3)
# Setting the speed
speed(10)
Doremon()
my_goto(250, -230)
write("by Codewithcurious.com", font=("Arial", 15))
mainloop()
The next user-defined function is “eyes()”, as the name suggests to draw the eyes of the character. In this function, to fill the drawing with the color we made use of an in-built function “fillcolor(“HEX value”)”. This function accepts the hex-number format as the argument for colors and to start the filling operation we make use of “begin_fill()”. To manage the turtle animation we used the “tracer()” function. With the help of the “lt()” & “fd()” functions we move the turtle left and forward by a specified distance. Then we will make use of “end_fill()” for ending the filling operation. Thus, by playing with coordinates and these above-mentioned functions we will draw the eyes of the character.
The next function is “whisker()” for drawing the whiskers of the character. Under this function first of all we are setting the coordinates for drawing the whisker with the help of “my_goto(x,y)” then we set the orientation of the turtle to a specific angle with the help of the “seth()” function. Then we move forward with a specified distance in that direction via “fd()”. And for drawing all 6 whiskers we do so and change the parameters of the function accordingly.
The next function is the “mouth()” for drawing the mouth of the character. Under this function first of all we set the coordinates, and orientation, and then move forward with a specific distance. All these functionalities will get executed with the same functions. After setting all these we will finally draw the circle for the mouth of the character with the help of “circle(radius, extent, steps)”.
The next function is “band()” to draw the band around the neck of the character. Under this function we will first define the filling color, then start the filling operation. To draw the band we will set the orientations and forward distance and at the end, we will end the filling operation. All of these will be done by the same functions specified above.
The next function is “nose()” for drawing the nose of the character. Under this function, we will set the location for drawing it by “my_goto()” and then follow the same procedure as we have drawn the band for the character.
The next function is “black_eyes()” for drawing the black eyes of the character. In this function, we will again follow the same procedure as before. Going to a desired location “my_goto(x,y)”, setting the orientation “seth()” ,Start the filling operation “begin_fill()”, draw the circle “circle()”, at last end the filling operation “end_fill()”. After this, we will reduce the pen size for drawing the white small circle inside the black eyes. That white circle will be made with the same procedure again.
The next function is “face()” for drawing the face of the character. Again with the help of sam functions, we will move to the desired location, fill the color accordingly, draw the circles and stop the filling operation. For filling the color, the whole drawing is made in the middle of the starting and ending command operation. With this type of project, we just have to use some specific functions again and again for drawing things and the changes are only done when we set the location to draw.
The next function is “head()” for drawing the head of the character, and with the same procedure will do so.
The next function is made to combine and draw these elements one after the other. “Doremon()”, in this function we have called all the above UDF serial-wise to make the picture properly. The series in which the functions are called is mentioned as:
- head()
- band()
- face()
- nose()
- mouth()
- whisker()
After this, to draw the body outline and fill the color in, we followed the same procedure by making use of the same functions. Now, to match the proper visual of the character, we filled the right palm and left palm with white color and both feet as well. We have also made an inner circle, a semicircle inside that, and a bell on the band. To do all these we have again applied the same mechanism, i.e. reached the desired location, started the filling operation and drew the circles accordingly. And after drawing the whole body of the character we called the black_eyes() function to draw the eyes to give the final look to our character.
Until now, we have made all the functions that will be needed to draw this cartoon character. Now to execute all of these we need a main loop. Under this loop, the basic need to run our project is the window screen and with the help of “screensize()” will do so. To get the turtle on the screen we will use “Screen()” and for setting the width and height of that turtle we will use “.setup()”. Then we will set the size and the speed of the turtle by “pensize()” & “speed()” respectively. Now, finally, we will call “Doremon()” to draw the character. To write anything on the screen we will use “write(“text”,font=(“font name”, size”)). And at last, we will call “mainloop()” for the turtle library.
output:
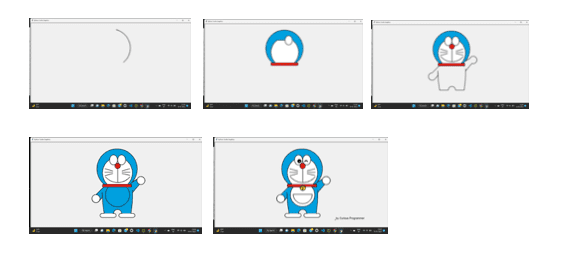
To read more about the turtle and its functions do refer it’s documentation
By following these steps you can easily draw this cartoon character!
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …