Flappy Bird Game Using Python With Source Code
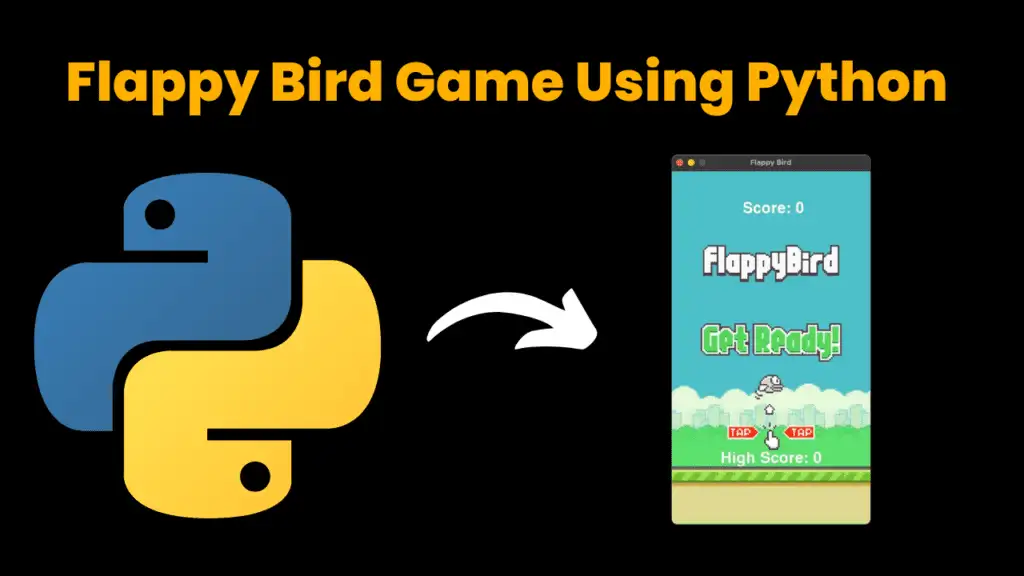
Introduction:
In this project, we have created a game using the “Pygame” module in python. The game is named “Flappy Bird”. Most of you have played this game on your mobile phones and now it’s time to code this game by yourself. If you haven’t played this before, not an issue, let’s cover this introduction with these few lines. The game is a side-scroller where the player controls a bird, attempting to fly between columns of green pipes without hitting them, and scores for the same.
Required Modules
pygame: Pygame is a set of Python modules designed for writing video games. It provides functionality for graphics, sound, and input handling, making it a popular choice for hobbyist and indie game developers.
Installation: You can install pygame using pip, Python’s package manager. Open a terminal or command prompt and type:
pip install pygame
Documentation: Pygame Documentation
sys: The sys
module provides access to some variables used or maintained by the Python interpreter and to functions that interact strongly with the interpreter.
Installation: sys
is a built-in module, meaning it comes pre-installed with Python and doesn’t require any additional installation steps.
Documentation: sys Documentation
time: The time
module provides various time-related functions. It’s commonly used for measuring time intervals, delaying execution, or converting between different time representations.
Installation: Like sys
, time
is a standard library module and doesn’t need to be installed separately.
Documentation: time Documentation
random: The random
module implements pseudo-random number generators for various distributions. It’s frequently used in scenarios where randomization or sampling is required.
Installation: Similarly, random
is a standard library module and is available without additional installation steps.
Documentation: random Documentation
Source Code:
Get Discount on Top Educational Courses
# Importing the libraries
import pygame
import sys
import time
import random
# Initializing the pygame
pygame.init()
# Frames per second
clock = pygame.time.Clock()
# Function to draw
def draw_floor():
screen.blit(floor_img, (floor_x, 520))
screen.blit(floor_img, (floor_x + 448, 520))
# Function to create pipes
def create_pipes():
pipe_y = random.choice(pipe_height)
top_pipe = pipe_img.get_rect(midbottom=(467, pipe_y - 300))
bottom_pipe = pipe_img.get_rect(midtop=(467, pipe_y))
return top_pipe, bottom_pipe
# Function for animation
def pipe_animation():
global game_over, score_time
for pipe in pipes:
if pipe.top < 0:
flipped_pipe = pygame.transform.flip(pipe_img, False, True)
screen.blit(flipped_pipe, pipe)
else:
screen.blit(pipe_img, pipe)
pipe.centerx -= 3
if pipe.right < 0:
pipes.remove(pipe)
if bird_rect.colliderect(pipe):
game_over = True
# Function to draw score
def draw_score(game_state):
if game_state == "game_on":
score_text = score_font.render(str(score), True, (255, 255, 255))
score_rect = score_text.get_rect(center=(width // 2, 66))
screen.blit(score_text, score_rect)
elif game_state == "game_over":
score_text = score_font.render(f" Score: {score}", True, (255, 255, 255))
score_rect = score_text.get_rect(center=(width // 2, 66))
screen.blit(score_text, score_rect)
high_score_text = score_font.render(f"High Score: {high_score}", True, (255, 255, 255))
high_score_rect = high_score_text.get_rect(center=(width // 2, 506))
screen.blit(high_score_text, high_score_rect)
# Function to update the score
def score_update():
global score, score_time, high_score
if pipes:
for pipe in pipes:
if 65 < pipe.centerx < 69 and score_time:
score += 1
score_time = False
if pipe.left <= 0:
score_time = True
if score > high_score:
high_score = score
# Game window
width, height = 350, 622
clock = pygame.time.Clock()
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Flappy Bird")
# setting background and base image
back_img = pygame.image.load("img_46.png")
floor_img = pygame.image.load("img_50.png")
floor_x = 0
# different stages of bird
bird_up = pygame.image.load("img_47.png")
bird_down = pygame.image.load("img_48.png")
bird_mid = pygame.image.load("img_49.png")
birds = [bird_up, bird_mid, bird_down]
bird_index = 0
bird_flap = pygame.USEREVENT
pygame.time.set_timer(bird_flap, 200)
bird_img = birds[bird_index]
bird_rect = bird_img.get_rect(center=(67, 622 // 2))
bird_movement = 0
gravity = 0.17
# Loading pipe image
pipe_img = pygame.image.load("greenpipe.png")
pipe_height = [400, 350, 533, 490]
# for the pipes to appear
pipes = []
create_pipe = pygame.USEREVENT + 1
pygame.time.set_timer(create_pipe, 1200)
# Displaying game over image
game_over = False
over_img = pygame.image.load("img_45.png").convert_alpha ()
over_rect = over_img.get_rect(center=(width // 2, height // 2))
# setting variables and font for score
score = 0
high_score = 0
score_time = True
score_font = pygame.font.Font("freesansbold.ttf", 27)
# Game loop
running = True
while running:
clock.tick(120)
# for checking the events
for event in pygame.event.get():
if event.type == pygame.QUIT: # QUIT event
running = False
sys.exit()
if event.type == pygame.KEYDOWN: # Key pressed event
if event.key == pygame.K_SPACE and not game_over: # If space key is pressed
bird_movement = 0
bird_movement = -7
if event.key == pygame.K_SPACE and game_over:
game_over = False
pipes = []
bird_movement = 0
bird_rect = bird_img.get_rect(center=(67, 622 // 2))
score_time = True
score = 0
# To load different stages
if event.type == bird_flap:
bird_index += 1
if bird_index > 2:
bird_index = 0
bird_img = birds[bird_index]
bird_rect = bird_up.get_rect(center=bird_rect.center)
# To add pipes in the list
if event.type == create_pipe:
pipes.extend(create_pipes())
screen.blit(floor_img, (floor_x, 550))
screen.blit(back_img, (0, 0))
# Game over conditions
if not game_over:
bird_movement += gravity
bird_rect.centery += bird_movement
rotated_bird = pygame.transform.rotozoom(bird_img, bird_movement * -6, 1)
if bird_rect.top < 5 or bird_rect.bottom >= 550:
game_over = True
screen.blit(rotated_bird, bird_rect)
pipe_animation()
score_update()
draw_score("game_on")
elif game_over:
screen.blit(over_img, over_rect)
draw_score("game_over")
# To move the base
floor_x -= 1
if floor_x < -448:
floor_x = 0
draw_floor()
# Update the game window
pygame.display.update()
# quiting the pygame and sys
pygame.quit()
sys.exit()
The most basic need to work under the module is to import them. With the help of the “import” keyword, we will import all the libraries needed. Along with “pygame”, we will also import the “sys”, “time”, and “random” modules.
Before talking about the process in which we have to code, let’s discuss the raw elements first and along with them the objectives as well:
*Firstly, for making this game the raw elements which will be needed:
- A bird
- A background image
- A floor image
- Pipe image
- Game over message image
* After discussing the elements, let’s discuss the objectives under which these elements will be used:
- We need to create an animation that will treat the user’s eye as if the bird is moving ahead and by moving the base we will do so.
- To make the bird fly and rotate.
- Create the pipes at the top and bottom and show them in animation as well.
- For maintaining the rules, we have to code for setting the parameters for scoring a point and losing the game by hitting the pipes and surfaces
The basic steps are to initialize the pygame module by the “.init()” method and set the frames per second by the “pygame.time.Clock() “method of the “random” module.
First of all, to create a game window we will use the “pygame.display.set_mode(width, height)” method and to set the caption of our window we will use “pygame.display.set_caption( )”.
Now we will make a game loop using a “while” loop under this we will run a “for” loop for getting the events with the help of “pygame.event.get()” and to check the event type we will make use of “.type”. firstly, we will check for the quit event with the help of “pygame. QUIT”.
In this project, we will make 5 user-defined functions named:
- draw_floor( )
- create_pipes( )
- pipe_animation( )
- draw_score( )
- score_update( )
Now starting with the game, the most basic need is to set a background image for our window screen. Firstly, we will load the image and then we will blit it on the screen, the blitting means to draw that particular image. To load the image we will use “pygame.image.load(‘image path or image name’)” and “screen. blit(‘source’,’ position’)” is being used to blit the particular image on the screen under the game loop. With the help of these two functions and the variable named “back_img” we will set the background image, and to update the game window with the changes we will use the “pygame.display.update()” function.
After drawing the background image we will need a floor image and with the same process under the variable named “floor_img”, we will load and then blit the floor image on the game window.
The next step is to move the floor and for doing so we will create a variable called “floor_x” and declare it initially as 0. Now under the game loop we will subtract the 1 from our variable and to move it continuously we will blit the floor image by adding 448 to our x position. All of this will be done under our first user-defined function “draw_floor( )” and the floor may not get disappeared so under the game loop we will check the condition of the floor_x will be less than -448 then again the floor_x will be set as 0. Due to this condition, we can see that the base on our game window will move continuously
The next most important step is to draw a bird on the screen and for showing the movement of the bird we will use three images of a bird at different stages. That is a bird with an up flap, a bid with a mid flap, and a bird with a down flap. We will load these images and blit them with the help of the same functions. Now to show these different images we will create a bird list and store these images in that and initially declare the bird index as 0. Now we will also create a bird rectangle with the help of “image-variable.get_rect(center=(position))”. Due to this step we just draw the bird on the game window
To give this bird some motion, we will make two variables named bird movement and bird gravity and they will be initialized as 0 and 0.17 respectively. Now in the game loop, we will increase the bird movement by bird gravity, and then to make our bird move downwards we will use “rect-variable(bird_rect).centery” and incrementing it by bird movement will do so. This functionality will let our bird move downwards.
To get the ability to control the bird’s motion with the space key, firstly we will check the key down event under the game loop with the help of “pygame. KEYDOWN”. If the event type condition satisfies then we will initialize the bird_movement variable as 0 and then to -7. Thus with help of the space key, we will be able to control our bird. Now to flap our bird we will make use of that list that we have created earlier storing different stages of the bird.
To make the wings flap we will create an event by using “.USEREVENT” and we will trigger the event by using the “pygame.time.set_timer()” method. After doing so and to make the bird bodies exchange we will check for the created event under the game loop and if the event is the one then we want the bird index to get incremented by 1. And if the bird index exceeds 2 then we want to create another rectangle with the same method and for that our center parameter will be bird_rect.center.
To make the bird rotate we will make use of the function “pygame. transform.rotozoom(‘surface, angle,scale’)” . and under the game loop where we are blitting the bird image instead of that, we will now blit the rotated bird.
The next major step is to make the pipes for that first, we will load the image and then blit it on the screen under the game loop we will store the different heights of the pipe and also create an empty list as pipes. To create pipes after a timestamp. We will create a user event named “create_pipes”. Now at this stage, we will create our 2nd UDF named “create_pipes( )”. Under this function, we will first randomly select the pipe height values to be drawn with the help of “.choice” under the “random” module. Then after that, we want to create two pipes, the top pipe, and the bottom pipe and for both of them, we will create the rectangles by “.get_rect( )” by seeing the necessary positions. For the top pipe will use midbottom arg in the method and for the bottom pipe midtop will be used and at the end of the function, both of the pipe variables will be returned. Again to call this function we will check the event type under the game loop and then we will call the function with the .extend() function which will append the pipe in the pipes list
To draw the pipes we will run a for loop in the pipes list and we will blit the pipe image under that. Now to move those pipes we will use “.centerx”. Now to flip our pipes we will check for the condition, if the top is less than 0 then we want to draw the flipped pipe and with the help of “pygame. transform. flip( )” we will do so. And if the condition stated above is not true then just draw a simple pipe. Now, all of these steps are used to make our next UDF named “pipe_animation( )” under which we are showing the movement of our pipes and now under this function itself we will set the conditions for collision. With help of “.colliderect( )” we will do so. Whenever the bird will hit the pipe the person will lose the game. In the end, we will call this function under the game loop.
To show the user the above condition, we will load a game over message image, so whenever the user hit the pipes the image will get displayed.
To restart the game, once the user loses the game we will check the condition under the space key event. That after losing the game if the user presses the space key then again the game will start. With this, we will also set the boundary conditions for the bird for losing the game.
Now, the last part is to show the scores. For doing so we will create a font variable first with the help of “pygame. font.Font(‘fontname.ttf’,size)” . For this, we will create another function named “draw_score” to draw the score. So first of all we will render the font variable by using “.render() “ and then with the help of “.blit()” we will draw it on screen. In this game, we have set the parameter for keeping the high score as well and we will check the condition for that if the present score is more than the previous score then the present score will be updated as a high score
To update the score we will make another function named “update_score( )”. So under this, we will check the condition with the help of position, if it satisfies the condition then we will increment the score by 1. Now we will call both of these functions under the game loop.
By following all these objectives step by step and using all these functions, the flappy bird game is ready!
Output:
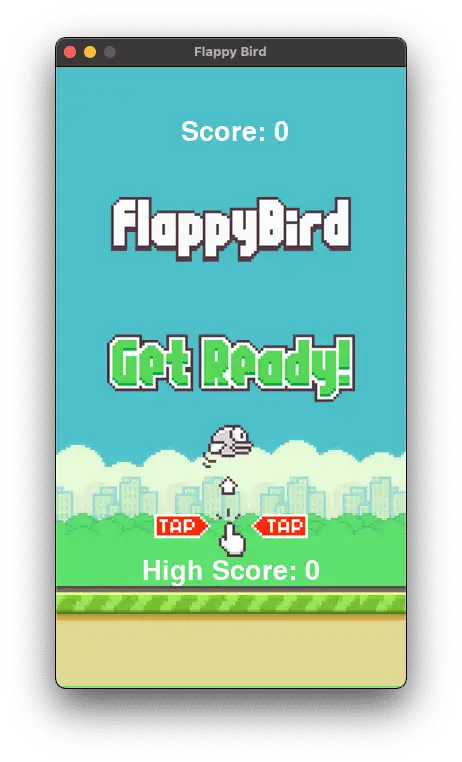
Find More Projects
MathGenius Pro – AI-Powered Math Solver Using Python Introduction: From simple arithmetic to more complicated college-level subjects like integration, differentiation, algebra, matrices, …
CipherMaze: The Ultimate Code Cracking Quest Game Using Python Introduction: You can make CipherMaze, a fun and brain-boosting puzzle game, with Python …
Warp Perspective Using Open CV Python Introduction: In this article, we are going to see how to Create a Warp Perspective System …
Custom AI Story Generator With Emotion Control Using Python Introduction: With the help of this AI-powered story generator, users can compose stories …
AI Powered PDF Summarizer Using Python Introduction: AI-Powered PDF Summarizer is a tool that extracts and summarizes research papers from ArXiv PDFs using Ollama (Gemma 3 LLM). The …
AI Based Career Path Recommender Using Python Introduction: One of the most significant and frequently perplexing decisions in a person’s life is …