Windows 12 Notepad Using Python
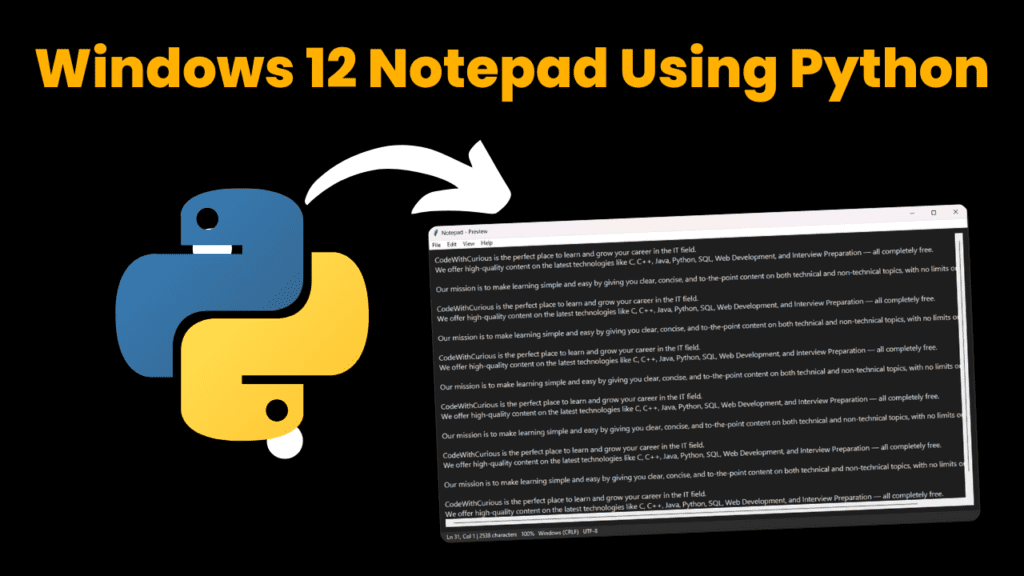
Introduction:
In this article, we will create a Windows 12 Notepad using Python.
If you are a beginner who wants to learn how to make desktop apps, or someone with experience who wants to try a modern project, this guide is for you. We will use Python’s built-in Tkinter library to create a nice-looking text editor that looks like Windows 12 style. Our goal is to build an app that is easy to use, looks modern, and has all the basic features needed for writing and editing text.
In the next parts, we will look at which Python tools we need, how to run the code, and understand each part step by step. At the end, we will see the full code and what the final result should look like. Everything is explained in a very simple and clear way, so even beginners can easily understand and follow along.
Required Modules or Packages:
To make our Windows 12 Notepad, we will use these built-in Python modules:
tkinter
This is Python’s built-in tool for creating desktop apps. We will use it to make the main window, buttons, text area, and menu bar.filedialog
This is a part of tkinter. It lets us open and save files by showing a file selection box on the screen.messagebox
This is also part of tkinter. It shows small popup messages, like asking the user if they want to save before closing the app.os
This module helps us work with files and folders on the computer. We use it to manage file names and paths.
You don’t need to install anything extra. These modules already come with Python.
How to Run the Code:
Follow these simple steps to run your Windows 12 Notepad application:
Ensure Python is Installed:
Verify that Python (preferably version 3.6 or above) is installed on your system by typing the following in your command prompt or terminal:
python --version
Copy the Code:
Copy the complete source code (provided in the Source Code section below) into a file named, for example, windows12_notepad.py
.
Navigate to Your Code Directory:
Open a terminal or command prompt and use the cd
command to change to the directory where you saved the file.
cd path/to/directory
Run the Script:
Execute the script by typing:
python windows12_notepad.py
The Notepad application window will launch, displaying the modern, dark-themed interface similar to Windows 12.
Begin Using the Application:
Use the menu options at the top to create new files, open existing ones, save your work, or exit the program. You can also utilize keyboard shortcuts for a smoother experience.
Code Explanation:
Let’s go step by step to understand how the Notepad works:
Setting Up the Application Window
from tkinter import *
root = Tk()
root.title("Notepad - Preview")
root.geometry("1920x1080") # Full screen
root.config(bg="#252526")
We first import everything from Tkinter, which is the library used for making the GUI. Then we create the main window with Tk()
. The window title is set to “Notepad – Preview,” and the geometry (size) of the window is set to fill the entire screen. We also set a dark background color with root.config(bg="#252526")
to give it a modern look.
Creating the Text Area
text_area = Text(root, font="Segoe UI 12", bg="#252526", fg="white", undo=True)
text_area.pack(expand=True, fill=BOTH)
Here, we create a text area where users can type. The Text()
widget is used for this purpose. We set the font to “Segoe UI” at size 12 for a modern look, a dark background, and white text for better visibility. The undo=True
allows users to undo text changes. Finally, we use pack(expand=True, fill=BOTH)
to make the text area fill the entire window.
Adding Scrollbars
scrollbar_v = Scrollbar(root, orient=VERTICAL, command=text_area.yview)
scrollbar_v.pack(side=RIGHT, fill=Y)
text_area.config(yscrollcommand=scrollbar_v.set)
scrollbar_h = Scrollbar(root, orient=HORIZONTAL, command=text_area.xview)
scrollbar_h.pack(side=BOTTOM, fill=X)
text_area.config(xscrollcommand=scrollbar_h.set)
We add both vertical and horizontal scrollbars to the text area. The vertical scrollbar is placed on the right, and the horizontal scrollbar is placed at the bottom. We also connect each scrollbar to the text area so that they move when the user scrolls.
File Operations: New File
def new_file():
text_area.delete(1.0, END)
The new_file()
function clears the text area so users can start typing a new document. The text_area.delete(1.0, END)
command removes all text, starting from the first character (1.0) to the last (END).
File Operations: Open File
from tkinter import filedialog
def open_file():
file = filedialog.askopenfilename(defaultextension=".txt", filetypes=[("Text files", "*.txt")])
if file:
with open(file, "r") as f:
text_area.delete(1.0, END)
text_area.insert(END, f.read())
The open_file()
function opens a file dialog to let the user choose a file. Once the file is selected, it reads the file’s contents and loads them into the text area. The filedialog.askopenfilename()
function opens the file selection window, and we use with open(file, "r")
to read the file. The text area is first cleared before loading the file’s content.
File Operations: Save File
def save_file():
file = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text files", "*.txt")])
if file:
with open(file, "w") as f:
f.write(text_area.get(1.0, END))
The save_file()
function opens a “Save As” dialog where the user can choose where to save the file. The content of the text area is then written to the selected file. text_area.get(1.0, END)
gets all the text from the text area, and with open(file, "w")
writes that text to the file.
Exit Application with Save Check
def exit_app():
if messagebox.askyesno("Save", "Do you want to save changes?"):
save_file()
root.quit()
The exit_app()
function checks if there are unsaved changes before closing the app. A message box appears asking if the user wants to save their changes. If they click “Yes,” the save_file()
function is called. Finally, root.quit()
closes the application.
Creating the Menu Bar
menu_bar = Menu(root)
file_menu = Menu(menu_bar, tearoff=0)
file_menu.add_command(label="New", command=new_file)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
file_menu.add_command(label="Exit", command=exit_app)
menu_bar.add_cascade(label="File", menu=file_menu)
root.config(menu=menu_bar)
We create a menu bar using Menu(root)
. The “File” menu is added with options like New, Open, Save, and Exit. Each menu item is linked to a function. For example, clicking “New” will run the new_file()
function. Finally, the menu bar is added to the window using root.config(menu=menu_bar)
.
Status Bar
status_bar = Label(root, text="Line 1, Column 1", bd=1, relief=SUNKEN, anchor=W)
status_bar.pack(side=BOTTOM, fill=X)
A status bar is added at the bottom of the window. It shows information about the current line and column number. The Label()
widget is used to create this, and pack(side=BOTTOM)
places it at the bottom of the window.
Keyboard Shortcuts
root.bind("", lambda event: new_file())
root.bind("", lambda event: open_file())
root.bind("", lambda event: save_file())
root.bind("", lambda event: exit_app())
These lines set up keyboard shortcuts. For example, pressing Ctrl+N will run the new_file()
function, Ctrl+O will open a file, and Ctrl+S will save the file. The root.bind()
function connects a keyboard action to a specific function.
Running the Application
root.mainloop()
The root.mainloop()
keeps the application running and responsive. It listens for events like button clicks and keyboard presses until the user closes the application.
Source Code:
Here you go, bhai! Below is the complete source code for the Windows 12 Notepad project. Just copy-paste it into your Python file (windows12_notepad.py
) and run it! 🔥
import tkinter as tk
from tkinter import filedialog, messagebox
import os
# ----- Basic setup for the main window -----
root = tk.Tk()
root.title("Notepad - Preview") # Window title
root.geometry(f"{root.winfo_screenwidth()}x{root.winfo_screenheight()}+0+0") # Fullscreen
root.configure(bg="#252526") # Dark background for a modern look
# ----- Creating the text area where user writes -----
text_area = tk.Text(
root,
font=("Segoe UI", 12),
wrap="none", # No word wrap by default
undo=True, # Allow undo/redo
bg="#1e1e1e", # Background color of text area
fg="#ffffff", # Text color
insertbackground="white", # Cursor color
relief="flat", # No border
padx=10,
pady=10
)
text_area.pack(expand=True, fill="both") # Take full space
# ----- Adding scrollbars -----
# Vertical scrollbar
scroll_y = tk.Scrollbar(text_area, orient="vertical", command=text_area.yview)
scroll_y.pack(side="right", fill="y")
text_area.configure(yscrollcommand=scroll_y.set)
# Horizontal scrollbar
scroll_x = tk.Scrollbar(text_area, orient="horizontal", command=text_area.xview)
scroll_x.pack(side="bottom", fill="x")
text_area.configure(xscrollcommand=scroll_x.set)
file_path = None # Keeps track of the current file path
# ----- File menu functions -----
def new_file():
# Clears everything for a new file
global file_path
file_path = None
text_area.delete("1.0", tk.END)
root.title("Untitled - Notepad")
update_status_bar()
def open_file():
# Lets the user open a text file
global file_path
path = filedialog.askopenfilename(
defaultextension=".txt",
filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")]
)
if path:
file_path = path
with open(path, "r", encoding="utf-8") as f:
content = f.read()
text_area.delete("1.0", tk.END)
text_area.insert("1.0", content)
root.title(os.path.basename(file_path) + " - Notepad")
update_status_bar()
def save_file():
# Saves the current file (asks location if new)
global file_path
if not file_path:
path = filedialog.asksaveasfilename(
initialfile="Untitled.txt",
defaultextension=".txt",
filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")]
)
if not path:
return
file_path = path
with open(file_path, "w", encoding="utf-8") as f:
f.write(text_area.get("1.0", tk.END))
root.title(os.path.basename(file_path) + " - Notepad")
def exit_app():
# Asks the user if they want to save before exiting
result = messagebox.askyesnocancel("Exit", "Do you want to save before exiting?")
if result is True:
save_file()
root.destroy()
elif result is False:
root.destroy()
# ----- Edit menu functions -----
def cut(): text_area.event_generate("<>")
def copy(): text_area.event_generate("<>")
def paste(): text_area.event_generate("<>")
def delete(): text_area.delete("1.0", tk.END)
def select_all(): text_area.tag_add("sel", "1.0", "end")
def deselect_all(): text_area.tag_remove("sel", "1.0", "end")
# ----- View menu functions -----
def toggle_word_wrap():
# Toggles word wrap on and off
current_wrap = text_area.cget("wrap")
text_area.config(wrap="word" if current_wrap == "none" else "none")
# ----- Help menu function -----
def about_notepad():
# Simple About info popup
messagebox.showinfo("About Notepad", "Windows 12 Notepad (Dark Style)\nCreated with Python + Tkinter")
# ----- Creating the top menu bar -----
menu_bar = tk.Menu(root, bg="#252526", fg="white", activebackground="#3c3c3c", bd=0)
# File menu
file_menu = tk.Menu(menu_bar, tearoff=0, bg="#252526", fg="white", activebackground="#3c3c3c")
file_menu.add_command(label="New", accelerator="Ctrl+N", command=new_file)
file_menu.add_command(label="Open", accelerator="Ctrl+O", command=open_file)
file_menu.add_command(label="Save", accelerator="Ctrl+S", command=save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", accelerator="Alt+F4", command=exit_app)
menu_bar.add_cascade(label="File", menu=file_menu)
# Edit menu
edit_menu = tk.Menu(menu_bar, tearoff=0, bg="#252526", fg="white", activebackground="#3c3c3c")
edit_menu.add_command(label="Undo", accelerator="Ctrl+Z", command=text_area.edit_undo)
edit_menu.add_command(label="Redo", accelerator="Ctrl+Y", command=text_area.edit_redo)
edit_menu.add_separator()
edit_menu.add_command(label="Cut", accelerator="Ctrl+X", command=cut)
edit_menu.add_command(label="Copy", accelerator="Ctrl+C", command=copy)
edit_menu.add_command(label="Paste", accelerator="Ctrl+V", command=paste)
edit_menu.add_command(label="Delete", accelerator="Del", command=delete)
edit_menu.add_separator()
edit_menu.add_command(label="Select All", accelerator="Ctrl+A", command=select_all)
edit_menu.add_command(label="Deselect All", command=deselect_all)
menu_bar.add_cascade(label="Edit", menu=edit_menu)
# View menu
view_menu = tk.Menu(menu_bar, tearoff=0, bg="#252526", fg="white", activebackground="#3c3c3c")
view_menu.add_command(label="Word Wrap", command=toggle_word_wrap)
menu_bar.add_cascade(label="View", menu=view_menu)
# Help menu
help_menu = tk.Menu(menu_bar, tearoff=0, bg="#252526", fg="white", activebackground="#3c3c3c")
help_menu.add_command(label="About Notepad", command=about_notepad)
menu_bar.add_cascade(label="Help", menu=help_menu)
# Attach the menu to the main window
root.config(menu=menu_bar)
# ----- Status bar at the bottom -----
status_bar = tk.Label(
root,
text="Ln 1, Col 1 | 0 characters 100% Windows (CRLF) UTF-8",
bg="#2d2d2d",
fg="white",
anchor="w",
padx=10
)
status_bar.pack(side="bottom", fill="x")
def update_status_bar(event=None):
# Shows line, column, and character count
line, col = text_area.index("insert").split(".")
line = int(line)
col = int(col) + 1
content = text_area.get("1.0", "end-1c")
chars = len(content)
status_text = f"Ln {line}, Col {col} | {chars} characters 100% Windows (CRLF) UTF-8"
status_bar.config(text=status_text)
# ----- Update status bar on user actions -----
text_area.bind("", update_status_bar)
text_area.bind("", update_status_bar)
text_area.bind("", update_status_bar)
# ----- Keyboard shortcuts -----
root.bind("", lambda e: new_file())
root.bind("", lambda e: open_file())
root.bind("", lambda e: save_file())
root.bind("", lambda e: select_all())
root.bind("", lambda e: text_area.edit_undo())
root.bind("", lambda e: text_area.edit_redo())
root.bind("", lambda e: cut())
root.bind("", lambda e: copy())
root.bind("", lambda e: paste())
root.bind("", lambda e: exit_app())
# Show initial status
update_status_bar()
# ----- Start the main loop (run the app) -----
root.mainloop()
Output:
After you run the code, the Notepad app will open in full screen with a modern dark look, just like something from Windows 12. Here’s what you’ll see:
Title Bar:
At the top of the app, it shows the name “Notepad – Preview.” If you open or save a file, the name of that file will also appear there.
Text Area:
In the middle, there’s a big area where you can write and edit your text. The background is dark and the text is white, which looks cool and is easy on the eyes. It gives a new modern look like latest Windows apps.
Scrollbars:
If your text becomes long, don’t worry. You’ll see scrollbars on the side and bottom, so you can easily move up, down, left, or right.
Status Bar:
At the bottom of the app, there’s a small bar that shows which line and column you’re on, and how many characters are there. It updates while you type.
Menu Bar:
At the top, you’ll see menus like File, Edit, View, and Help. You can use them to create new files, open old ones, save your work, cut/copy/paste, and even get help.
Keyboard Shortcuts:
You can also use shortcut keys like Ctrl+N for new file, Ctrl+S for save, etc. It makes using the app faster and easier.
To help you understand what the final app looks like, I have added a screenshot below.
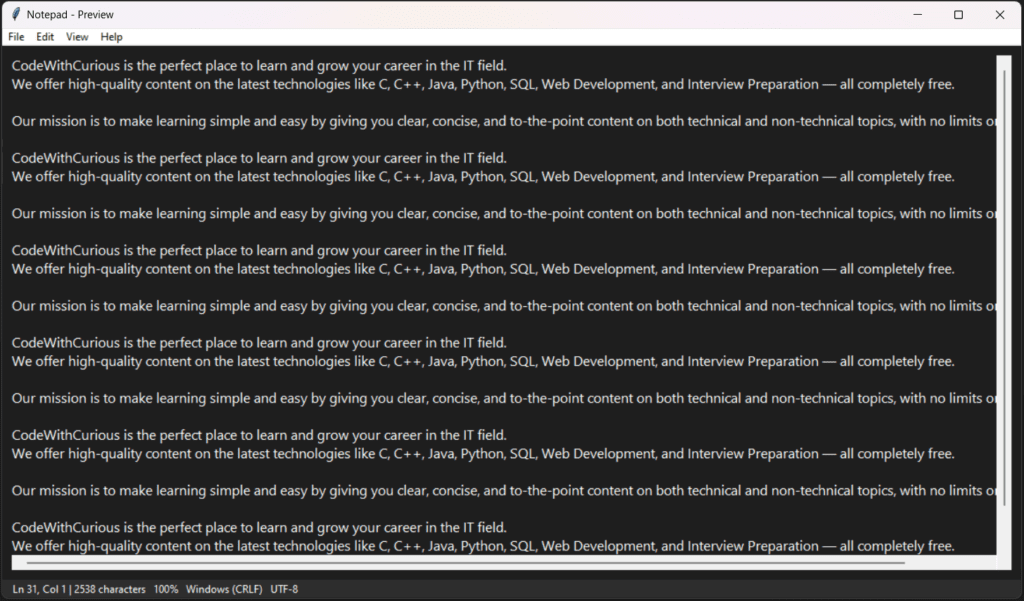
This image shows the clean full-screen design. You can clearly see the title bar at the top, the menu options, the big text area in the center, and the status bar at the bottom — all in one glance.
You can also check how it looks on your own computer and compare it with the screenshot. The design is made to feel familiar but also modern, just like the latest Windows versions.