Car Racing Game Using Python
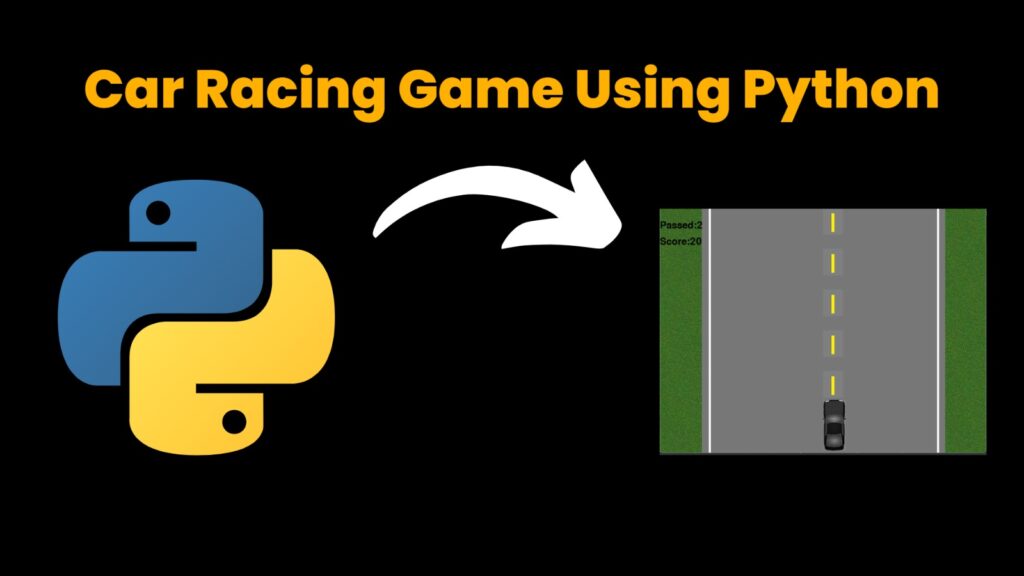
Introduction:
In this project, we have created a “Car Racing Game” by using the pygame module in Python. It is one of the most efficient libraries for game development using python. Starting with an introductory window providing two options that are, to start or to quit, to the user, following this, the user will control the movement of the vehicle accordingly and prevent it from crashing.
Explanation:
First of all, we will import all the necessary modules. For game development with python we will import the pygame module, for controlling the frames will import the time module, for getting random values random module will get used, and the last sys module.
After importing all the modules, we will initialize the pygame by using the “.init()” method. With this, we will create the game window and then set the caption for the one by using “pygame. display.set_mode()” & “pygame.display.set_caption()” respectively.
The main thing about any game development project is images. Under this project, to make it interactive and attractive we will use the following images:
- A background image
- Grass image
- White strip image
- Yellow strip image
- Car images
- Starting city image
Source Code:
# Importing the library
import pygame
import time
import random
import sys
# Initializing the pygame
pygame.init()
# window
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("CAR RACING")
# Loading all images
c1_img = pygame.image.load("car1.jpg")
clock = pygame.time.Clock()
grass = pygame.image.load("grass.jpg")
y_strip = pygame.image.load("y_strip.jpg")
strip = pygame.image.load("strip.jpg")
start = pygame.image.load("start.jpg")
# Function for getting all images
def background():
screen.blit(grass, (0, 0))
screen.blit(grass, (700, 0))
screen.blit(y_strip, (400, 0))
screen.blit(y_strip, (400, 100))
screen.blit(y_strip, (400, 200))
screen.blit(y_strip, (400, 300))
screen.blit(y_strip, (400, 400))
screen.blit(y_strip, (400, 500))
screen.blit(y_strip, (400, 600))
screen.blit(strip, (120, 0))
screen.blit(strip, (680, 0))
# Getting the car on screen
def car(x, y):
screen.blit(c1_img, (x, y))
x_change = 0
x = 400
y = 470
car_width = 56
op_speed = 10
obs = 0
y_change = 0
obs_x = random.randrange(200, 650)
obs_y = -750
op_width = 56
op_height = 125
car_passed = 0
score = 0
level = 0
# Function for enemy cars
def obstacle(obs_x, obs_y, obs):
if obs == 0:
obs_img = pygame.image.load("car2.jpg")
elif obs == 1:
obs_img = pygame.image.load("car3.jpg")
elif obs == 2:
obs_img = pygame.image.load("car4.jpg")
elif obs == 3:
obs_img = pygame.image.load("car5.jpg")
elif obs == 4:
obs_img = pygame.image.load("car6.jpg")
elif obs == 5:
obs_img = pygame.image.load("car7.jpg")
screen.blit(obs_img, (obs_x, obs_y))
# message to display
font = pygame.font.SysFont("None", 150)
render = font.render("CAR CRASHED", True, (0, 0, 0))
# Function to display score
def sc(car_passed, score):
s_font = pygame.font.SysFont(None, 35)
passed = s_font.render("Passed:" + str(car_passed), True, (0, 0, 0))
score = s_font.render("Score:" + str(score), True, (0, 0, 0))
screen.blit(passed, (0, 30))
screen.blit(score, (0, 70))
# Text on Buttons
def text(text, font):
texts = font.render(text, True, (255, 255, 255))
return texts, texts.get_rect()
# Game loop
def game_loop():
global op_speed, x, car_passed, level, x_change, y_change, y, obs_y, obs_x, obs, score, font
running = True
while running:
# for checking the events
for event in pygame.event.get():
if event.type == pygame.QUIT: # QUIT event
running = False
if event.type == pygame.KEYDOWN: # KEYDOWN event
if event.key == pygame.K_LEFT:
x_change = -5
if event.key == pygame.K_RIGHT:
x_change = 5
if event.key == pygame.K_s: # Increase speed
op_speed += 2
if event.key == pygame.K_b: # decrease speed
op_speed -= 2
if event.type == pygame.KEYUP: # KEYUP event
if event.key == pygame.K_LEFT or event.key == pygame.K_RIGHT:
x_change = 0
x += x_change
screen.fill((119, 119, 119))
background()
obs_y -= (op_speed / 4)
obstacle(obs_x, obs_y, obs)
obs_y += op_speed
car(x, y)
sc(car_passed, score)
# Restricting the movement
if x > 680 - car_width or x 600:
obs_y = 0 - op_height
obs_x = random.randrange(170, 600)
obs = random.randrange(0, 6)
car_passed += 1
score = car_passed * 10
if int(car_passed) % 10 == 0:
level += 1
op_speed += 2
font = pygame.font.SysFont(None, 50)
level_text = font.render("Level" + str(level), True, (0, 0, 0))
screen.blit(level_text, (0, 100))
pygame.display.flip()
# car crash logic
if y obs_x and x obs_x and x + car_width 80 and mouse[0] 490 and mouse[1] 580 and mouse[0] 490 and mouse[1] < 590:
pygame.draw.rect(screen, (255, 0, 0), (580, 540, 150, 50))
if click == (1, 0, 0):
pygame.quit()
t = pygame.font.SysFont("arial", 30, "bold")
texts, textr = text("START", t)
textr.center = ((80 + (150 / 2)), (540 + (50 / 2)))
screen.blit(texts, textr)
texts, textr = text("QUIT", t)
textr.center = ((580 + (150 / 2)), (540 + (50 / 2)))
screen.blit(texts, textr)
pygame.display.update()
intro() # calling the intro function
game_loop() # calling the game loop
pygame.quit()
By using “pygame.image.load(<filename>)” we will load all the images in the project. The next step is to blit the image over the screen to be seen and with the help of the “.blit()” function we will blit all the images under a user-defined function named “background()”. We will make another UDF and blit the car image at the coordinates to get the car image.
Continuing in this process, now we will initialize some variables.
- x,y,x_change,y_change : for the coordinates of user’s car
- op_speed: for setting the opponent car speed
- op_width,car_width & op_height: for the width and height of opponent cars
- obs,obs_x,obs_y: for the coordinates of opponent cars
- car_passed: for counting the no of cars passed
- level, score: for determining level and score
In the next step, we will create another UDF named “obstacle()” to show all the opponent cars on the screen. Under this function, we will load the image and then we will blit it by following the same procedure as mentioned above.
For displaying the score, first, we will create the font object by using “pygame.font.Sysfont()” and then we will render it by “.render()” and finally we will blit it on the screen. All of these functionalities will be executed under the UDF named “sc()”.
The most important thing under the game development projects is to run a game loop. For this we will define a UDF named “game_loop()” for this, we will run a for loop for getting the events by using “pygame.event.get()”. Under the “for” block we will check the type of events by the “event. type()” method. Will check for the quit event and keydown event by using “pygame. QUIT” & “pygame. KEYDOWN” respectively because we will press the keys for the movement. Under the keydown event, we will check for the key pressed i.e. left or right by using “pygame.K_LEFT” & “pygame.K_RIGHT”. To show the movement while pressing we will manipulate the coordinate of the user’s car by shifting its x coordinate.
After doing so, we will call all the UDFs under the game loop accordingly. Now, the main logic for our game begins. Let’s code for that. For setting the boundaries of the car movement will use, a use condition according to the car width. The next segment of the code is to get the opponent cars continuously on the window screen, for getting these cars randomly we will make use of random module functions. The last segment of code under this function is to define the car crash logic, we just have to code for this by keeping the width of the cars from the left and right sides in the mind and then we will blit the “CAR CRASHED” message on the screen with the same method. In the end, we will update the screen by “pygame.display.flip()” and to maintain the frames we will use the “.tick()” method.
We will make another UDF named “intro()” to make this game’s introductory window. Under this, we will first load the background image and then blit it. After this will draw two buttons stating “START” &” QUIT” by using the “pygame.draw.rect()” method. For the text to get appeared on that rectangle we will make another UDF named “text()” and with the help of the font object we will follow the same procedure. With the help of “pygame.mouse.get_pos()” we will get the coordinates at which our mouse pointer is present and with the help of that we will show the hover effect on those buttons by drawing another rectangle over them. Now, with the help of “pygame.mouse.get_pressed()” we will come to know the coordinates will the mouse button is pressed, and by using that we will call the “game_loop()” for the start button and “pygame. quit()” for the quit button.
In the end, we will call the intro() and game_loop() functions to run this project successfully.
By following the above-mentioned procedure, you can easily build up a simple car racing game!
Output:
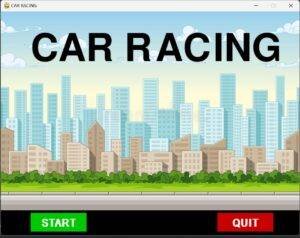
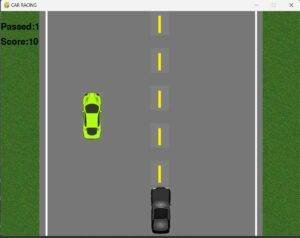
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …