Hospital Management System using Python with Complete Source code [By using Flask Framework]
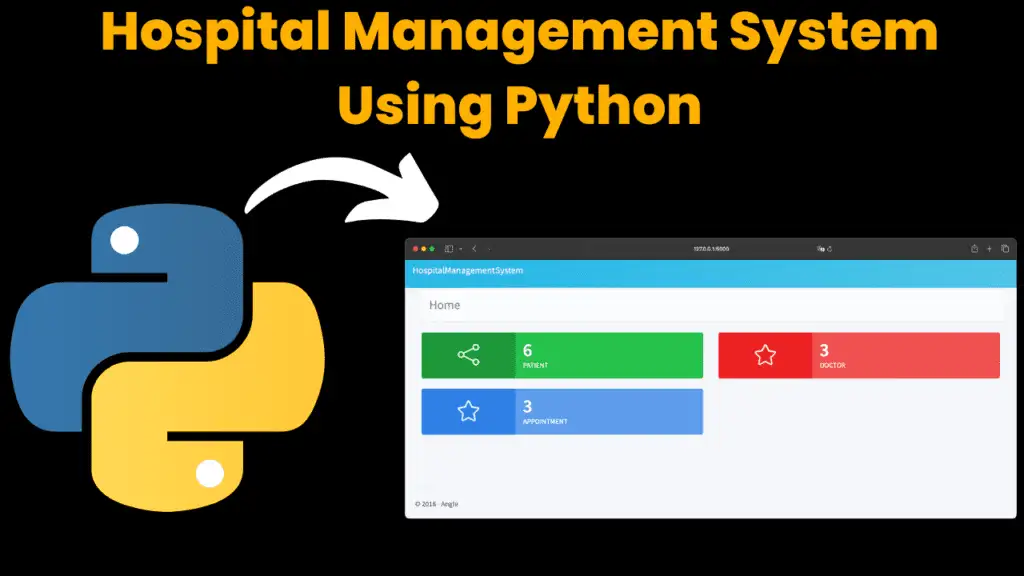
Introduction :
The Hospital Management System is a web-based application designed to streamline the management of medical practices, providing a comprehensive platform for handling patient records, doctor information, appointment scheduling, and common administrative tasks. Built on the Flask framework with RESTful API endpoints, this system offers a modern and efficient solution for healthcare providers to organize their operations seamlessly.
Key Features:
Patient Management: Efficiently manage patient records, including personal information, medical history, and appointments. With dedicated endpoints for creating, updating, and retrieving patient data, healthcare providers can easily access vital patient information.
Doctor Management: Maintain a database of doctors, including their specialties, contact details, and availability. The system allows for the creation, modification, and retrieval of doctor profiles, facilitating effective communication and coordination among medical staff.
Appointment Scheduling: Streamline appointment scheduling processes with intuitive features for booking, rescheduling, and canceling appointments. The system provides a user-friendly interface for patients to request appointments and for administrators to manage appointment calendars.
Common Functionalities: In addition to patient and doctor management, the system includes common functionalities such as authentication, authorization, and error handling. These features ensure the security and reliability of the application while providing a seamless user experience.
Technologies Used:
Flask Framework: The application is built using the Flask framework, a lightweight and modular framework for building web applications in Python. Flask provides the foundation for creating RESTful APIs and serving dynamic web content.
Flask-Restful: To simplify the development of RESTful APIs, the application leverages Flask-Restful, an extension for Flask that provides tools for quickly building API endpoints.
JSON Configuration: Configuration settings such as host and port are stored in a JSON file, allowing for easy customization and deployment of the application.
Code Explanation:
This Python code sets up a Flask web application with RESTful API endpoints for managing patients, doctors, appointments, and common functionalities. Here’s a breakdown of what each part of the code does:
Imports:
Flask
: This is the main Flask class, used to create the Flask web application.send_from_directory
: A Flask function for serving static files.render_template
: A Flask function for rendering HTML templates.Flask-Restful
: An extension for Flask that adds support for quickly building REST APIs.- Various custom modules (
Patients
,Patient
,Doctors
,Doctor
,Appointments
,Appointment
,Common
) from thepackage
directory, which presumably contain the implementations for handling different resources in the API. json
: For working with JSON data.
Loading configuration:
- The code loads configuration settings from a JSON file named
config.json
using thejson.load()
function. These settings likely include the host and port on which the Flask application should run.
- The code loads configuration settings from a JSON file named
Creating the Flask application and API:
- An instance of the Flask class is created with the name
app
. - An instance of the Flask-Restful
Api
class is created with theapp
instance.
- An instance of the Flask class is created with the name
Adding RESTful resources:
- RESTful resources for managing patients (
Patients
,Patient
), doctors (Doctors
,Doctor
), appointments (Appointments
,Appointment
), and common functionalities (Common
) are added to the API using theapi.add_resource()
method.
- RESTful resources for managing patients (
Routes:
- A route for the root URL
'/'
is defined using the@app.route()
decorator. When users visit the root URL, the application will serve theindex.html
file from the static directory.
- A route for the root URL
Running the application:
- Finally, the application is run using the
app.run()
method. Thedebug=True
parameter enables debug mode, and thehost
andport
are set based on the values loaded from theconfig.json
file.
- Finally, the application is run using the
Required Modules:
In the provided project, several modules are required to build and run the Flask-based web application with RESTful API endpoints. Here are the required modules along with instructions on how to install them:
Flask: This is the main web framework used for building the application.
- Installation: You can install Flask using pip, the Python package installer.
pip install Flask
- Installation: You can install Flask using pip, the Python package installer.
Flask-Restful: This extension simplifies the process of building RESTful APIs in Flask.
- Installation:
pip install flask-restful
- Installation:
JSON: This module is used for working with JSON data, particularly for loading configuration settings from a JSON file.
- JSON is a built-in module in Python, so no installation is required.
- JSON is a built-in module in Python, so no installation is required.
How to Run the Code:
To run the provided Python code, which sets up a Flask web application with RESTful API endpoints, follow these steps:
- Download the project Code: Download the Project code from the given link, extract the folder then open the file app.py
Install Required Modules: Ensure that you have installed Flask and Flask-Restful by running the following commands in your terminal or command prompt:
pip install Flask
pip install flask-restful
Run the Application: Execute the Python script to start the Flask application. You can do this by running the script from the command line:
python app.py
Get Discount on Top Educational Courses
Source Code:
Output:
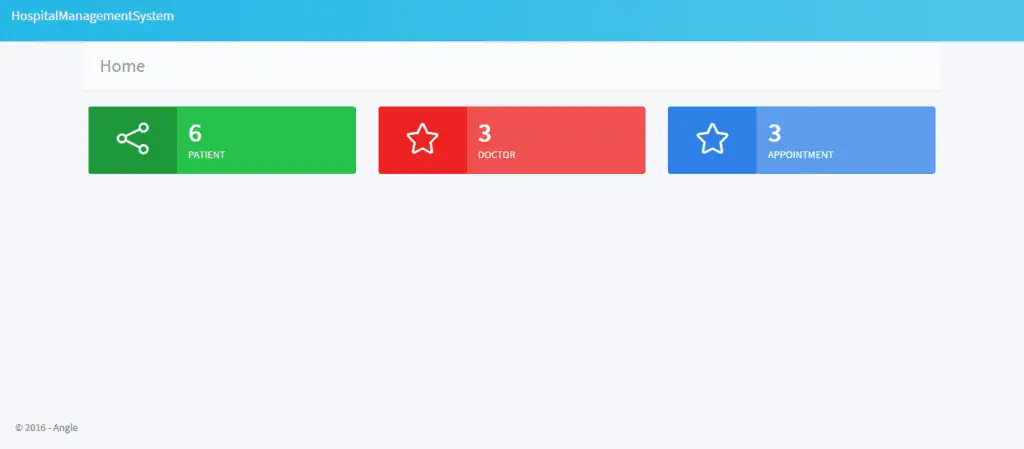
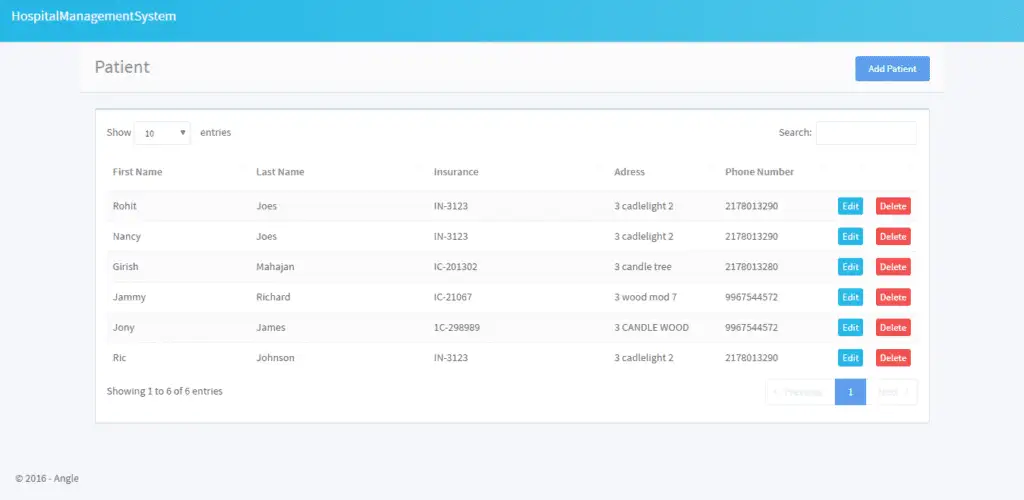
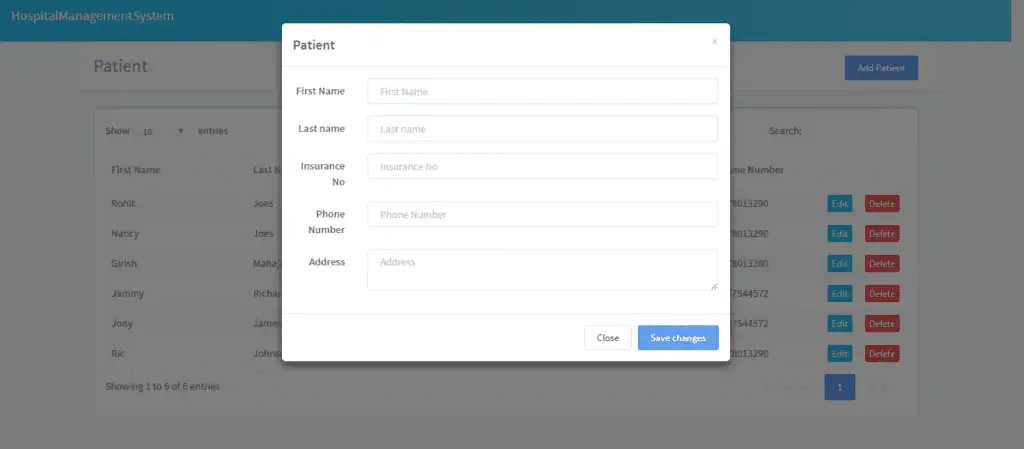
More Projects:
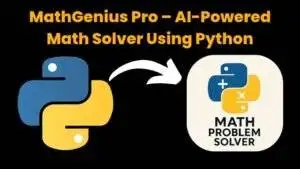
MathGenius Pro – AI-Powered Math Solver Using Python
MathGenius Pro – AI-Powered Math Solver Using Python Introduction: From simple arithmetic to more complicated college-level subjects like integration, differentiation, algebra, matrices, and graph plotting,
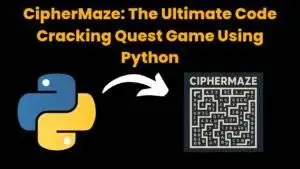
CipherMaze: The Ultimate Code Cracking Quest Game Using Python
CipherMaze: The Ultimate Code Cracking Quest Game Using Python Introduction: You can make CipherMaze, a fun and brain-boosting puzzle game, with Python and tkinter. The
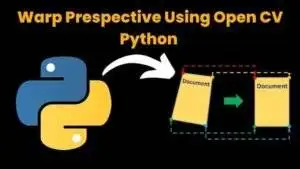
Warp Perspective Using Open CV Python
Warp Perspective Using Open CV Python Introduction: In this article, we are going to see how to Create a Warp Perspective System Using Python .
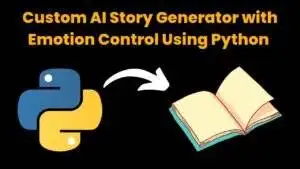
Custom AI Story Generator With Emotion Control Using Python
Custom AI Story Generator With Emotion Control Using Python Introduction: With the help of this AI-powered story generator, users can compose stories based on a
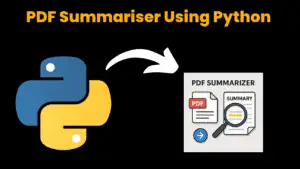
AI Powered PDF Summarizer Using Python
AI Powered PDF Summarizer Using Python Introduction: AI-Powered PDF Summarizer is a tool that extracts and summarizes research papers from ArXiv PDFs using Ollama (Gemma 3 LLM). The system provides structured,
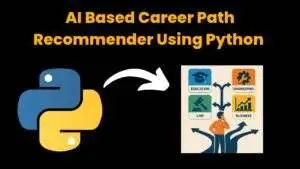
AI Based Career Path Recommender Using Python
AI Based Career Path Recommender Using Python Introduction: One of the most significant and frequently perplexing decisions in a person’s life is selecting the appropriate