Birthday Wishes Using Python
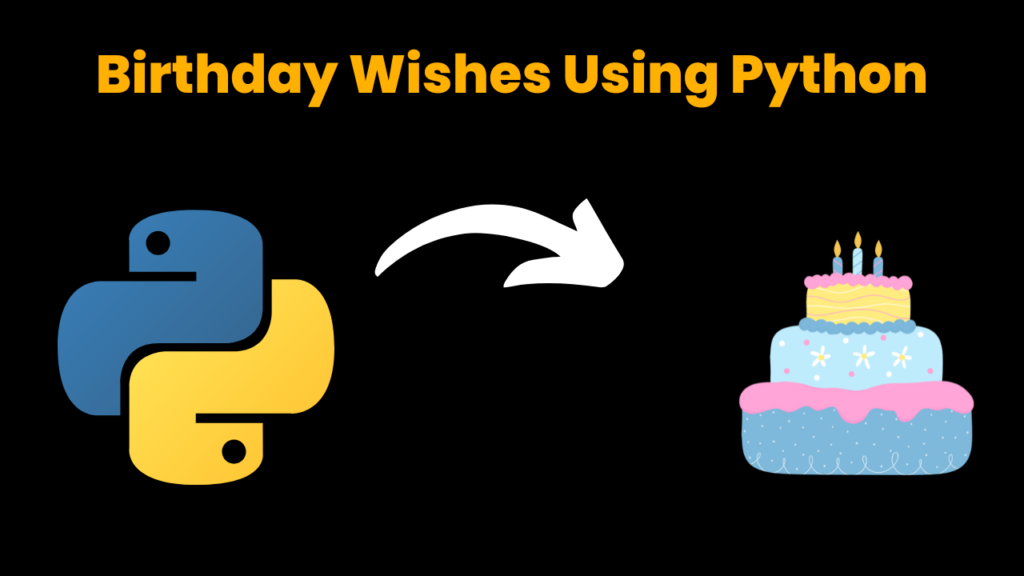
Introduction
Hello Curious Coders,
In this Project we are going to generate simple Birthday wishes using turtle package in python. Turtle is a package in python which is responsible to produce some graphics. So here is the Code….
Code
import turtle
# Create a turtle object
my_turtle = turtle.Turtle()
# Set the turtle's speed to fast
my_turtle.speed(10)
# Set the turtle's pen color to red
my_turtle.pencolor("red")
# Move the turtle to the starting position
my_turtle.penup()
my_turtle.goto(-150, 0)
my_turtle.pendown()
# Write the word "Happy"
my_turtle.write("Happy", font=("Arial", 36, "bold"))
# Move the turtle to the next position
my_turtle.penup()
my_turtle.forward(200)
# Write the word "Birthday"
my_turtle.write("Birthday", font=("Arial", 36, "bold"))
# Hide the turtle when done
my_turtle.hideturtle()
# Keep the turtle window open
turtle.done()
Code Explanation
- First, we imported the turtle module using the line
import turtle
. - Then, we created a turtle object using the line
my_turtle = turtle.Turtle()
. This creates an instance of the turtle class, which we can use to control the turtle’s movements and drawing. - Next, we set the turtle’s speed to fast using the line
my_turtle.speed(10)
. This sets the turtle’s animation speed, with 10 being the fastest setting. - After that, it sets the turtle’s pen color to red using the line
my_turtle.pencolor("red")
. This sets the color of the turtle’s pen, which is used to draw on the screen. - Next, it moves the turtle to the starting position using the lines
my_turtle.penup()
,my_turtle.goto(-150, 0)
, andmy_turtle.pendown()
. The turtle’s starting position is set to (-150, 0) on the x-y plane. Thepenup()
method is used to lift the turtle’s pen off the screen so it can move to the new position without drawing. Thependown()
method is used to put the pen back on the screen so it can begin drawing again. - Then, it writes the word “Happy” using the line
my_turtle.write("Happy", font=("Arial", 36, "bold"))
. This uses the turtle’swrite()
method to write the word “Happy” in red, bold font using Arial font of size 36. - After that, it moves the turtle to the next position using the line
my_turtle.penup()
,my_turtle.forward(200)
. This moves the turtle 200 units forward from its current position. - Finally, it writes the word “Birthday” using the line
my_turtle.write("Birthday", font=("Arial", 36, "bold"))
. This uses the turtle’swrite()
method to write the word “Birthday” in red, bold font using Arial font of size 36. - Then, it hides the turtle using the line
my_turtle.hideturtle()
. This makes the turtle invisible on the screen. - Finally, it keeps the turtle window open using the line
turtle.done()
. This keeps the turtle window open until the user closes it. - Overall, this code uses the turtle module to create a turtle object, set its properties, and use its methods to write the words “Happy” and “Birthday” on the screen.
Output:
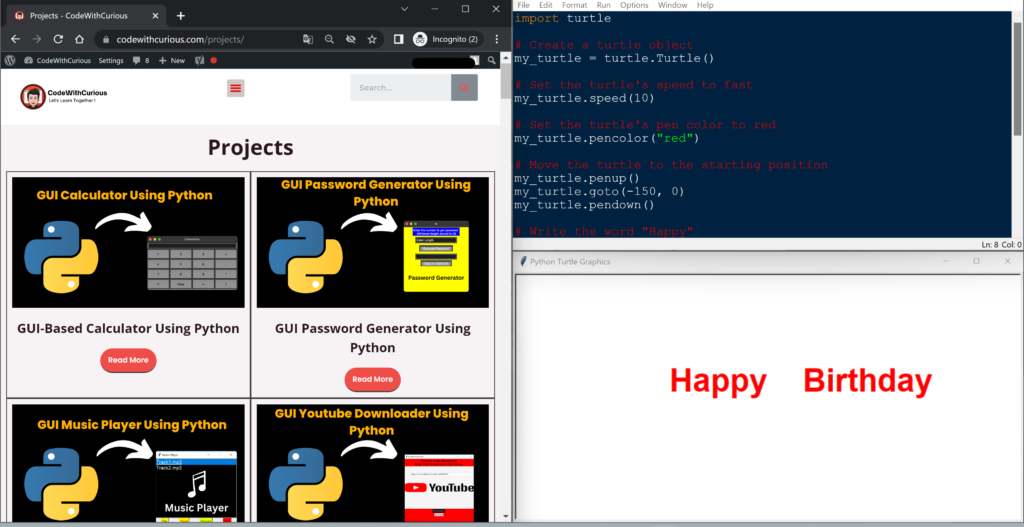
Find More Projects
library management system using python with source code using Python GUI Tkinter (Graphical User Interface) How to Run the code: Introduction: Imagine …
Space Shooter Game Using Python with source code Overview: A space shooter game typically involves controlling a spaceship to navigate through space …
Hotel Management System Using Python with source code Introduction: Welcome to our blog post introducing a helpful tool for hotels: the Tkinter-based …
Student Management System Using Python Introduction: The Student Management System is a comprehensive software solution designed to streamline the process of managing …
Billing Management System using Python introduction: The Billing software using python is a simple yet effective Python application designed to facilitate the …
Medical Management System using Python with Complete Source code [By using Flask Framework] Introduction Hospital Management System The Hospital Management System is …