Word Scramble Game Using HTML, CSS and JavaScript With Source Code
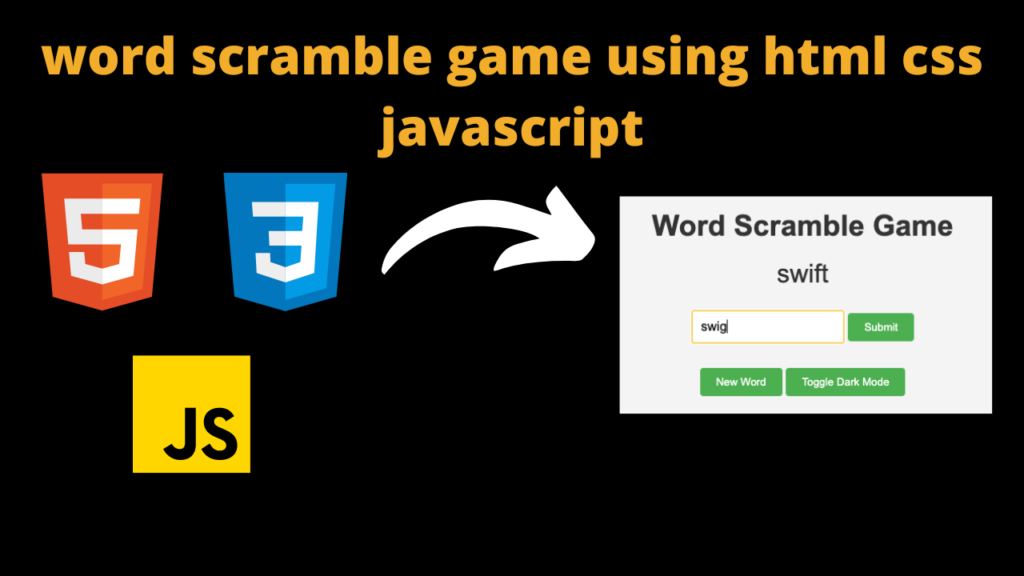
Introduction :
Welcome to the Word Scramble Game project! This simple yet engaging web-based game challenges players to unscramble words and test their vocabulary skills. Developed using HTML, CSS, and JavaScript, this project offers an interactive user interface and provides an enjoyable gaming experience.
Features:
- Word Scramble: Players are presented with a scrambled word that they must unscramble to form the correct word.
- User Input: An input field allows players to enter their guesses for the scrambled word.
- Feedback: Upon submitting a guess, players receive instant feedback indicating whether their guess is correct or incorrect.
- New Word: Players can generate a new scrambled word at any time to continue playing.
- Dark Mode: A toggleable dark mode option enhances user experience by providing an alternative color scheme.
How to run the source code:
To run the code, you’ll need a text editor to create the HTML, CSS, and JavaScript files, and a web browser to view the game. Here’s a step-by-step guide:
Set Up Files:
- Create three files:
index.html
,style.css
, andscript.js
. - Copy the HTML code into
index.html
, CSS code intostyle.css
, and JavaScript code intoscript.js
.
- Create three files:
Open in a Web Browser:
- After saving the files, open
index.html
in a web browser (Chrome, Firefox, Safari, etc.). - You can either double-click the
index.html
file to open it in your default browser or right-click the file and select “Open with” to choose a specific browser.
- After saving the files, open
Play the Game:
- Once the HTML file is opened in the browser, you should see the Word Scramble Game interface.
- You can start playing by typing your guess in the input field and clicking “Submit”. If your guess is correct, it will display “Correct!” otherwise “Incorrect. Try again!”.
- Click “New Word” to generate a new scrambled word to guess.
- You can also toggle between light and dark mode by clicking the “Toggle Dark Mode” button.
Interact with the Game:
- Keep guessing words and enjoying the game! Try to unscramble as many words as you can.
Source code explanation:
HTML (index.html):
- The HTML file defines the structure of our web page.
- Inside the
<body>
tag, we have a<div>
element with the id “container” which holds all the content. - There’s an
<h1>
element for the game title. - A
<div>
with id “word” is where the scrambled word will be displayed. - An
<input>
element with the id “guess” allows the user to input their guess. - A “Submit” button with id “submit” to submit the guess.
- A
<div>
with id “message” to display feedback messages. - A “New Word” button with id “new-word” to generate a new word to guess.
- A “Toggle Dark Mode” button with id “toggle-dark-mode” to switch between light and dark mode.
CSS (style.css):
- Defines the styles for various elements in the game.
- Sets the font family, background color, and text color for the body.
- Provides styling for the container, headings, word display, input field, buttons, and dark mode.
- Uses transitions for smooth color changes in dark mode.
JavaScript (script.js):
- Defines an array
words
containing the words to be used in the game. - Functions:
selectRandomWord()
: Selects a random word from thewords
array.scrambleWord(word)
: Takes a word and returns a scrambled version of it.displayScrambledWord()
: Displays a scrambled word on the page.checkGuess()
: Checks if the user’s guess matches the current word and displays feedback.
- Event listeners:
- Listens for clicks on the “Submit” button and calls
checkGuess()
function. - Listens for clicks on the “New Word” button and generates a new scrambled word.
- Listens for clicks on the “Toggle Dark Mode” button and toggles the
dark-mode
class on the body.
- Listens for clicks on the “Submit” button and calls
- Initializes the game by displaying a scrambled word when the page loads.
This code creates a simple Word Scramble Game with a user-friendly interface, including a dark mode option for better user experience. Players can guess the scrambled word, receive feedback, and toggle dark mode as desired.
Get Discount on Top Educational Courses
Source Code:
HTML (index.html):
Word Scramble Game
Word Scramble Game
CSS (style.css):
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
color: #333;
transition: background-color 0.5s ease, color 0.5s ease;
}
.dark-mode body {
background-color: #333;
color: #f4f4f4;
}
#container {
text-align: center;
margin-top: 50px;
}
h1 {
font-size: 36px;
margin-bottom: 20px;
}
#word {
font-size: 32px;
margin-bottom: 20px;
}
#guess {
margin-bottom: 10px;
padding: 10px;
font-size: 16px;
}
#message {
margin-top: 10px;
font-weight: bold;
}
button {
margin-top: 10px;
padding: 10px 20px;
background-color: #4CAF50;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
.dark-mode button {
background-color: #222;
}
.dark-mode button:hover {
background-color: #333;
}
JavaScript (script.js):
const words = ['javascript', 'html', 'css', 'python', 'java', 'ruby', 'php', 'swift'];
let currentWord, scrambledWord;
function selectRandomWord() {
return words[Math.floor(Math.random() * words.length)];
}
function scrambleWord(word) {
return word.split('').sort(() => Math.random() - 0.5).join('');
}
function displayScrambledWord() {
currentWord = selectRandomWord();
scrambledWord = scrambleWord(currentWord);
document.getElementById('word').innerText = scrambledWord;
}
function checkGuess() {
const guess = document.getElementById('guess').value.toLowerCase();
if (guess === currentWord) {
document.getElementById('message').innerText = 'Correct!';
} else {
document.getElementById('message').innerText = 'Incorrect. Try again!';
}
}
document.getElementById('submit').addEventListener('click', checkGuess);
document.getElementById('new-word').addEventListener('click', () => {
displayScrambledWord();
document.getElementById('guess').value = '';
document.getElementById('message').innerText = '';
});
document.getElementById('toggle-dark-mode').addEventListener('click', () => {
document.body.classList.toggle('dark-mode');
});
// Initialize game
displayScrambledWord();
Output :
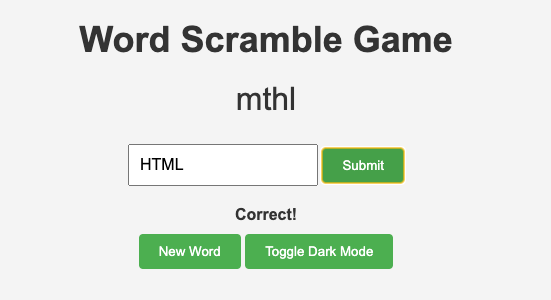
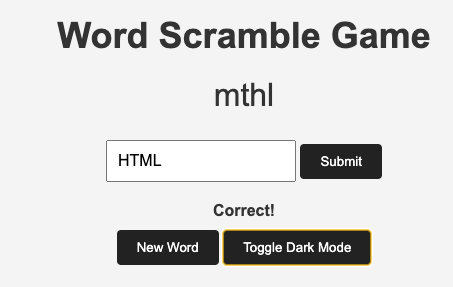
Find More Projects
ludo game in python using gui and thinkter introduction The “ludo game in python“ project is a simplified digital version of the …
hotel management system in python introduction The Hotel Management System is a simple, interactive GUI-based desktop application developed using Python’s Tkinter library. …
cryptography app in python using gui introduction In the digital age, data security and privacy are more important than ever. From messaging …
portfolio generator tool in python using GUI introduction In today’s digital-first world, having a personal portfolio is essential for students, job seekers, …
interactive story game in python using gui introduction The Interactive story game in python using gUI is a visually rich, dynamic, and engaging …
maze generator using Python with Pygame Module GUI introduction In this maze generator using Python, realm of logic, problem-solving, and computer science, …