Stopwatch Using Java Swing
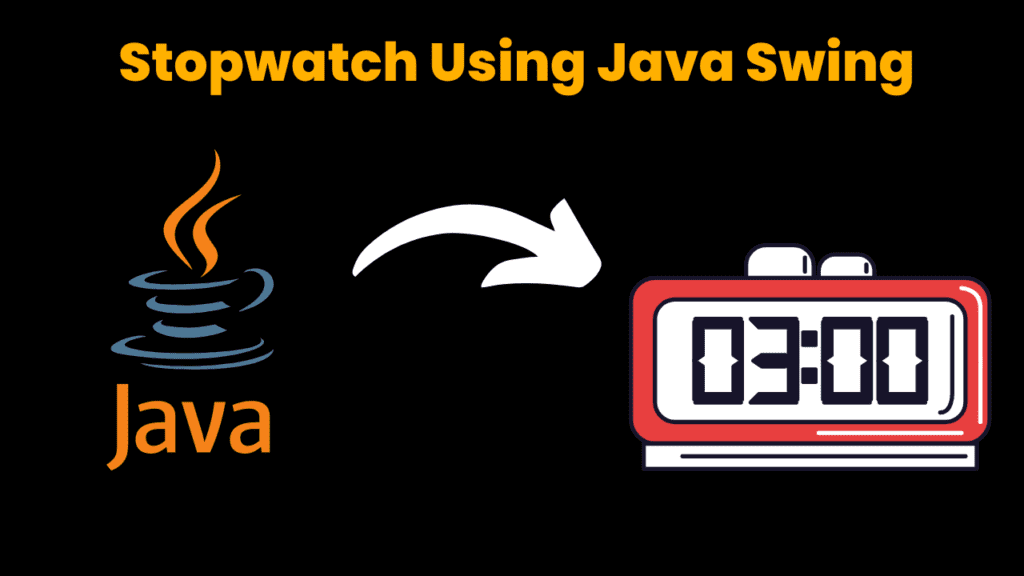
Introduction:
In this article, we will show how to use the Java Swing library to develop a Stop Watch. This Stop Watch uses a graphical user interface(GUI) to display current time. This stop watch is build using the Java Swing library, which provides a flexible set of components for creating a graphical user interface(GUI). This application has an easy and simple design with Label and buttons to start, stop and reset the timer. Grab a cup of coffee, sit down and start using the Stop Watch.
Explanation:
This Stop Watch is a Graphical user interface(GUI) application that shows current time to the user using label. The watch updates the elapsed time every second and updates the time label accordingly. Users may very easily use the stop watch with the system’s simple design. The stop watch has simple design label and buttons. The user can enter start and timer, stop the timer and reset the timer to zero.
The users can use three buttons in the application i.e., start, stop and reset button. Start button is used to start the timer, stop button stops the timer and reset button is used to reset the elapsed time to zero.
The Java Swing library which gives a flexible and powerful set of components is used to develop the Stop Watch. The application makes use of Swing components such as JLabel, JButton andJPanel among many other Swing components. Time is set to the JLabel using setText() Method. Timer class is used to start, stop and reset the time.
Methods used in the project:
actionPerformed():
The action or events of the buttons are handled using this method. Buttons in the stop watch such as start, stop and reset are handled using this method. This method describe how the components should behave after the button is click.
updateTimeLabel():
This method is used to update the elapsed time every second and also updates the time label accordingly.
Source Code:
//@codewithcurious.com
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Stop_watch extends JFrame implements ActionListener {
private JLabel timeLabel;
private JButton startButton, stopButton, resetButton;
private Timer timer;
private int elapsedTime;
public Stop_watch() {
// Set up the user interface
timeLabel = new JLabel("00:00:00", JLabel.CENTER);
timeLabel.setFont(new Font("Arial", Font.PLAIN, 50));
timeLabel.setForeground(Color.red);
startButton = new JButton("Start");
startButton.addActionListener(this);
stopButton = new JButton("Stop");
stopButton.addActionListener(this);
resetButton = new JButton("Reset");
resetButton.addActionListener(this);
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(1, 3));
buttonPanel.add(startButton);
buttonPanel.add(stopButton);
buttonPanel.add(resetButton);
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
contentPane.add(timeLabel, BorderLayout.CENTER);
contentPane.add(buttonPanel, BorderLayout.SOUTH);
// Set up the timer
timer = new Timer(1000, new ActionListener() {
public void actionPerformed(ActionEvent e) {
elapsedTime += 1000;
updateTimeLabel();
}
});
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
timer.start();
} else if (e.getSource() == stopButton) {
timer.stop();
} else if (e.getSource() == resetButton) {
timer.stop();
elapsedTime = 0;
updateTimeLabel();
}
}
private void updateTimeLabel() {
int hours = elapsedTime / 3600000;
int minutes = (elapsedTime % 3600000) / 60000;
int seconds = (elapsedTime % 60000) / 1000;
String time = String.format("%02d:%02d:%02d", hours, minutes, seconds);
timeLabel.setText(time);
}
public static void main(String[] args) {
Stop_watch frame = new Stop_watch();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 250);
frame.setVisible(true);
}
}
Output:
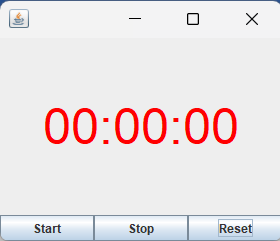
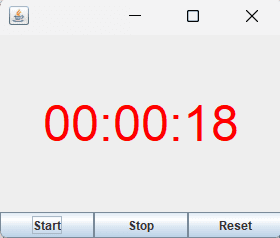
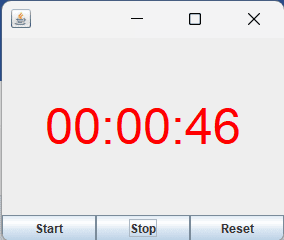
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …