Digital Clock Using Java Swing
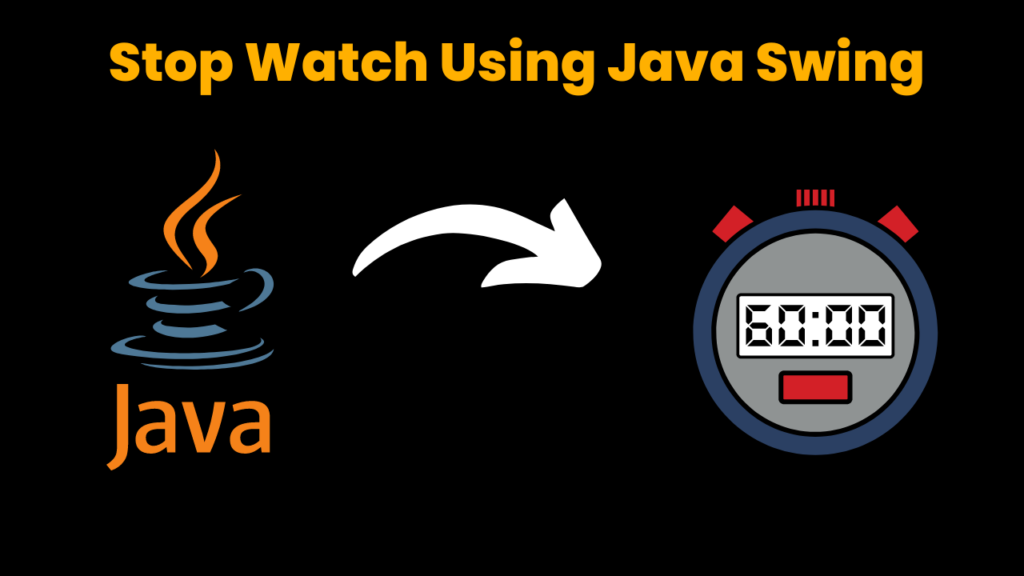
Introduction:
In this project, we will show how to use the Java Swing library to build a digital clock. This digital clock uses a graphical user interface(GUI) to display current date and time. This clock is build using the Java Swing library, which provides a flexible set of components for creating a graphical user interface(GUI). This application has an easy and simple design with Labels to show current date and time. Grab a cup of coffee, sit down and start using the Digital Clock.
Explanation:
This Digital Clock is a Graphical user interface(GUI) application that shows current date and time to the user using labels. The clock updates the date and time automatically. The time is shown in HH:MM:SS format i.e., hours, minutes and seconds respectively. The date is in the format of EEE, MMM dd, yyyy i.e., day, month, day and year respectively.
The Java Swing library which gives a flexible and powerful set of components is used to develop the Digital Clock. The application makes use of Swing components such as JLabel and JPanel among many other Swing components. Date and time is set to the JLabel using setText() Method. Methods of Calendar class in util package is used to get the current date and time.
Methods used in the project:
getTime():
This is the method of Calendar class which belongs the java.util package. It returns the current system date and time in the given format.
Source Code:
//@codewithcurious.com
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import javax.swing.BorderFactory;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.SwingConstants;
import javax.swing.Timer;
public class Digital_clock extends JFrame {
private JLabel timeLabel;
private JLabel dateLabel;
public Digital_clock() {
setTitle("Digital Clock");
setSize(500, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
// Create a panel to hold the time and date labels
JPanel panel = new JPanel();
panel.setBorder(BorderFactory.createEmptyBorder(20, 20, 20, 20));
panel.setLayout(new BorderLayout());
// Create a label to display the time
timeLabel = new JLabel();
timeLabel.setFont(new Font("Arial", Font.PLAIN, 60));
timeLabel.setHorizontalAlignment(SwingConstants.CENTER);
timeLabel.setVerticalAlignment(SwingConstants.CENTER);
timeLabel.setForeground(Color.RED);
// Create a label to display the date
dateLabel = new JLabel();
dateLabel.setFont(new Font("Arial", Font.PLAIN, 20));
dateLabel.setHorizontalAlignment(SwingConstants.CENTER);
dateLabel.setVerticalAlignment(SwingConstants.CENTER);
dateLabel.setForeground(Color.WHITE);
// Add the time and date labels to the panel
panel.add(timeLabel, BorderLayout.CENTER);
panel.add(dateLabel, BorderLayout.SOUTH);
// Set the panel's background color
panel.setBackground(Color.BLACK);
// Add the panel to the frame
add(panel);
// Use a Timer to update the time and date labels every second
Timer timer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
updateTimeAndDate();
}
});
timer.start();
}
private void updateTimeAndDate() {
// Get the current time and format it
Calendar calendar = Calendar.getInstance();
SimpleDateFormat timeFormatter = new SimpleDateFormat("HH:mm:ss");
String time = timeFormatter.format(calendar.getTime());
// Get the current date and format it
SimpleDateFormat dateFormatter = new SimpleDateFormat("EEE, MMM dd, yyyy");
String date = dateFormatter.format(calendar.getTime());
// Update the time and date labels
timeLabel.setText(time);
dateLabel.setText(date);
}
public static void main(String[] args) {
Digital_clock clock = new Digital_clock();
clock.setVisible(true);
}
}
Output:
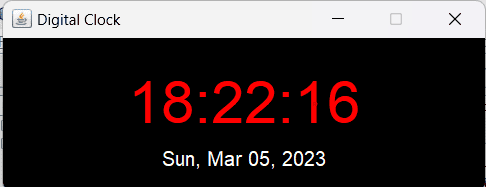
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …