Currency Converter Using Java Swing With Source Code
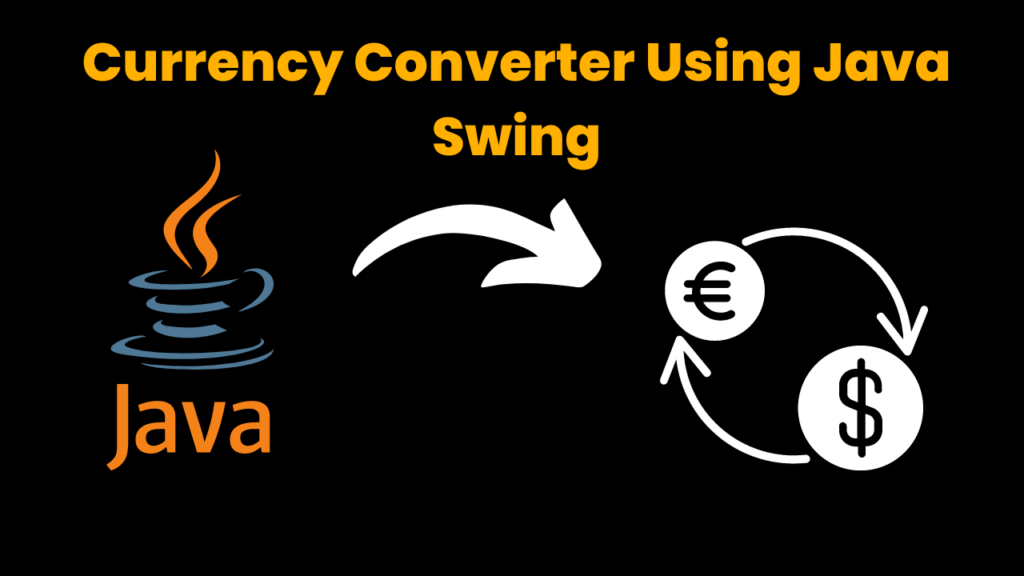
Introduction :
In this article, we will demonstrate how to develop Currency Converter using Java Swing library. This Currency Converter allows you to convert the currency using a graphical user interface (GUI) application. This application is build using the Java Swing library which gives a flexible set of components that can be use to build a Graphical user interface(GUI). This application has an easy and simple design with button to convert the entered currency amount in targeted currency. So take a seat, pour a cup of coffee and start using currency converter.
Explanation:
This Currency Converter is a Graphical user interface(GUI) system that allows user to easily convert the entered currency amount in the targeted currency using button. Users may very quickly convert the currency using the system’s simple design. The Currency Converter has simple design with TextField, combo box and button. The user can enter the currency in Text field then select the targeted currency and can convert using convert button.
The users may convert the currency using ‘convert’ button. The user can enter the currency in Text field then select the targeted currency and can convert using convert button.
The Java Swing library which gives a flexible and powerful set of components is used to develop the currency Converter. The system makes use of Swing components such as JLabel, JTextField, JComboBox and JButton among many other Swing components. Button event is handled by the ActionListener interface and button events are defined using ActionListener’s actionPerformed() method.
Methods used in the project:
actionPerformed():
The action or events of the buttons are handled using this method. Buttons in the system to convert the currency is handled using this method. This method describe how the components should behave after the button is click.
Source Code:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.DecimalFormat;
public class Currency_Converter extends JFrame {
private JLabel amountLabel, fromLabel, toLabel, resultLabel;
private JTextField amountField;
private JComboBox fromComboBox, toComboBox;
private JButton convertButton;
private DecimalFormat decimalFormat = new DecimalFormat("#,##0.00");
private final String[] currencies = {"USD", "EUR", "JPY", "GBP", "CAD", "AUD", "CHF", "CNY","INR"};
private double[] exchangeRates = {1.00, 0.84, 109.65, 0.72, 1.27, 1.30, 0.92, 6.47,87.14};
public Currency_Converter() {
setTitle("Currency Converter");
setLayout(new GridLayout(4, 2));
amountLabel = new JLabel("Amount:");
add(amountLabel);
amountField = new JTextField();
add(amountField);
fromLabel = new JLabel("From:");
add(fromLabel);
fromComboBox = new JComboBox<>(currencies);
add(fromComboBox);
toLabel = new JLabel("To:");
add(toLabel);
toComboBox = new JComboBox<>(currencies);
add(toComboBox);
convertButton = new JButton("Convert");
add(convertButton);
resultLabel = new JLabel();
add(resultLabel);
convertButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
double amount = Double.parseDouble(amountField.getText());
String fromCurrency = (String) fromComboBox.getSelectedItem();
String toCurrency = (String) toComboBox.getSelectedItem();
double exchangeRate = exchangeRates[getIndex(toCurrency)] / exchangeRates[getIndex(fromCurrency)];
double result = amount * exchangeRate;
resultLabel.setText(decimalFormat.format(result) + " " + toCurrency);
} catch (Exception ex) {
resultLabel.setText("Invalid input");
}
}
});
setSize(300, 200);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
private int getIndex(String currency) {
for (int i = 0; i < currencies.length; i++) {
if (currency.equals(currencies[i])) {
return i;
}
}
return -1;
}
public static void main(String[] args) {
new Currency_Converter();
}
}
Output:
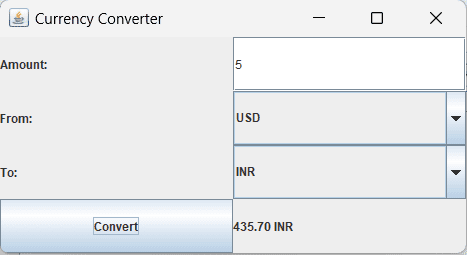
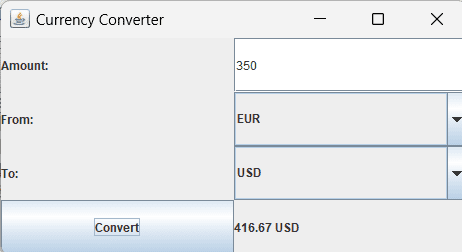
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …