JavaNote - Text-Based Note Taking Application
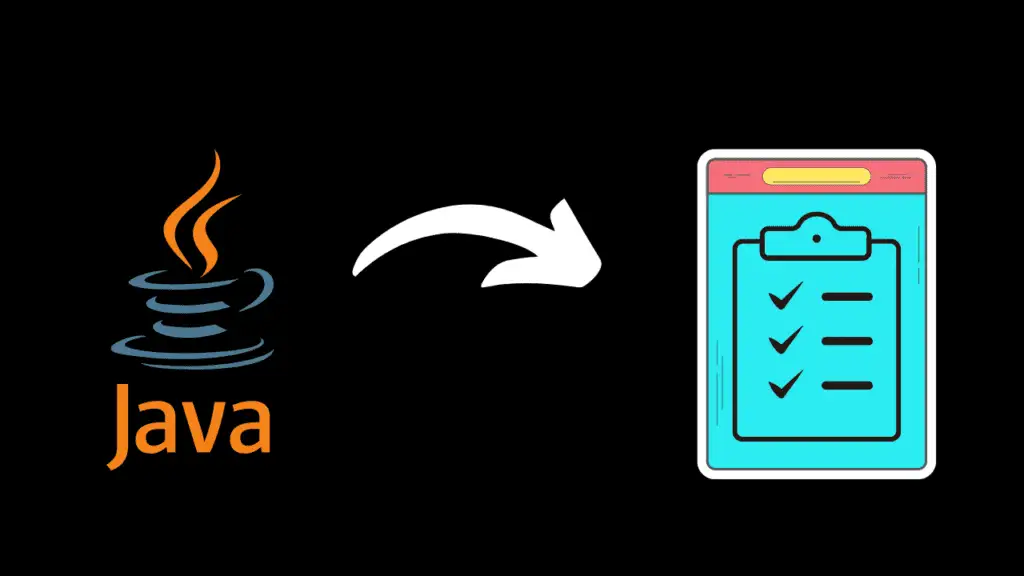
Introduction:
JavaNote is a simple text-based note-taking application designed to help users create, organize, and manage their notes efficiently. With features like formatting, categorization, and basic search functionality, JavaNote provides a user-friendly interface for maintaining digital notes. This project is an excellent opportunity to practice Java programming and GUI development skills.
Key Features:
User Authentication: Users can create accounts or log in securely to access their notes.
Note Creation and Editing: Users can create new notes, edit existing ones, and apply basic formatting (such as bold, italic, and underline) using a user-friendly text editor.
Categorization: Notes can be categorized into different topics or folders, allowing users to better organize their content.
Search Functionality: Users can search for specific notes by keywords, titles, or tags.
User Interface: A graphical user interface (GUI) will be provided for users to interact with the application. The GUI should include buttons, menus, and text areas for creating, editing, and managing notes.
File Persistence: Notes and user data will be saved locally using file storage. Serialization can be used to save and load notes.
Formatting Options: Implement a set of buttons or formatting shortcuts that users can use to apply formatting options to selected text in the note editor.
Responsive Design: Design the application’s GUI to be responsive and user-friendly, ensuring that it works well on different screen sizes.
Data Validation: Implement input validation to ensure that users enter valid data when creating or editing notes.
Source Code:
Note.java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.ArrayList;
public class NoteAppFrame extends JFrame {
private JTextArea noteTextArea;
private JComboBox categoryComboBox;
private ArrayList notes;
public NoteAppFrame() {
setTitle("JavaNote - Note Taking Application");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 400);
setLocationRelativeTo(null);
notes = new ArrayList<>();
noteTextArea = new JTextArea();
categoryComboBox = new JComboBox<>();
JButton createButton = new JButton("Create");
JButton editButton = new JButton("Edit");
JButton deleteButton = new JButton("Delete");
JButton saveButton = new JButton("Save");
JPanel controlPanel = new JPanel();
controlPanel.setLayout(new FlowLayout());
controlPanel.add(createButton);
controlPanel.add(editButton);
controlPanel.add(deleteButton);
controlPanel.add(saveButton);
createButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
createNote();
}
});
editButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
editNote();
}
});
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
deleteNote();
}
});
saveButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
saveNotes();
}
});
add(controlPanel, BorderLayout.NORTH);
add(new JScrollPane(noteTextArea), BorderLayout.CENTER);
loadNotes();
}
private void loadNotes() {
try {
FileInputStream fileIn = new FileInputStream("notes.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
notes = (ArrayList) in.readObject();
in.close();
fileIn.close();
populateCategoryComboBox();
} catch (IOException | ClassNotFoundException ex) {
ex.printStackTrace();
}
}
private void saveNotes() {
try {
FileOutputStream fileOut = new FileOutputStream("notes.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(notes);
out.close();
fileOut.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
private void createNote() {
String title = JOptionPane.showInputDialog(this, "Enter note title:");
String content = noteTextArea.getText();
String category = categoryComboBox.getSelectedItem().toString();
Note note = new Note(title, content, category);
notes.add(note);
populateCategoryComboBox();
saveNotes();
noteTextArea.setText("");
JOptionPane.showMessageDialog(this, "Note created successfully!");
}
private void editNote() {
int selectedNoteIndex = notes.size() > 0 ? categoryComboBox.getSelectedIndex() : -1;
if (selectedNoteIndex >= 0) {
Note selectedNote = notes.get(selectedNoteIndex);
selectedNote.setContent(noteTextArea.getText());
saveNotes();
JOptionPane.showMessageDialog(this, "Note edited successfully!");
} else {
JOptionPane.showMessageDialog(this, "No note selected.");
}
}
private void deleteNote() {
int selectedNoteIndex = notes.size() > 0 ? categoryComboBox.getSelectedIndex() : -1;
if (selectedNoteIndex >= 0) {
notes.remove(selectedNoteIndex);
populateCategoryComboBox();
saveNotes();
noteTextArea.setText("");
JOptionPane.showMessageDialog(this, "Note deleted successfully!");
} else {
JOptionPane.showMessageDialog(this, "No note selected.");
}
}
private void populateCategoryComboBox() {
categoryComboBox.removeAllItems();
for (Note note : notes) {
categoryComboBox.addItem(note.getTitle());
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new NoteAppFrame();
frame.setVisible(true);
});
}
}
NoteAppFrame.java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.util.ArrayList;
public class NoteAppFrame extends JFrame {
private JTextArea noteTextArea;
private JComboBox categoryComboBox;
private ArrayList notes;
public NoteAppFrame() {
setTitle("JavaNote - Note Taking Application");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(600, 400);
setLocationRelativeTo(null);
notes = new ArrayList<>();
noteTextArea = new JTextArea();
categoryComboBox = new JComboBox<>();
JButton createButton = new JButton("Create");
JButton editButton = new JButton("Edit");
JButton deleteButton = new JButton("Delete");
JButton saveButton = new JButton("Save");
JPanel controlPanel = new JPanel();
controlPanel.setLayout(new FlowLayout());
controlPanel.add(createButton);
controlPanel.add(editButton);
controlPanel.add(deleteButton);
controlPanel.add(saveButton);
createButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
createNote();
}
});
editButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
editNote();
}
});
deleteButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
deleteNote();
}
});
saveButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
saveNotes();
}
});
add(controlPanel, BorderLayout.NORTH);
add(new JScrollPane(noteTextArea), BorderLayout.CENTER);
loadNotes();
}
private void loadNotes() {
try {
FileInputStream fileIn = new FileInputStream("notes.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
notes = (ArrayList) in.readObject();
in.close();
fileIn.close();
populateCategoryComboBox();
} catch (IOException | ClassNotFoundException ex) {
ex.printStackTrace();
}
}
private void saveNotes() {
try {
FileOutputStream fileOut = new FileOutputStream("notes.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(notes);
out.close();
fileOut.close();
} catch (IOException ex) {
ex.printStackTrace();
}
}
private void createNote() {
String title = JOptionPane.showInputDialog(this, "Enter note title:");
String content = noteTextArea.getText();
String category = categoryComboBox.getSelectedItem().toString();
Note note = new Note(title, content, category);
notes.add(note);
populateCategoryComboBox();
saveNotes();
noteTextArea.setText("");
JOptionPane.showMessageDialog(this, "Note created successfully!");
}
private void editNote() {
int selectedNoteIndex = notes.size() > 0 ? categoryComboBox.getSelectedIndex() : -1;
if (selectedNoteIndex >= 0) {
Note selectedNote = notes.get(selectedNoteIndex);
selectedNote.setContent(noteTextArea.getText());
saveNotes();
JOptionPane.showMessageDialog(this, "Note edited successfully!");
} else {
JOptionPane.showMessageDialog(this, "No note selected.");
}
}
private void deleteNote() {
int selectedNoteIndex = notes.size() > 0 ? categoryComboBox.getSelectedIndex() : -1;
if (selectedNoteIndex >= 0) {
notes.remove(selectedNoteIndex);
populateCategoryComboBox();
saveNotes();
noteTextArea.setText("");
JOptionPane.showMessageDialog(this, "Note deleted successfully!");
} else {
JOptionPane.showMessageDialog(this, "No note selected.");
}
}
private void populateCategoryComboBox() {
categoryComboBox.removeAllItems();
for (Note note : notes) {
categoryComboBox.addItem(note.getTitle());
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
JFrame frame = new NoteAppFrame();
frame.setVisible(true);
});
}
}
Output:
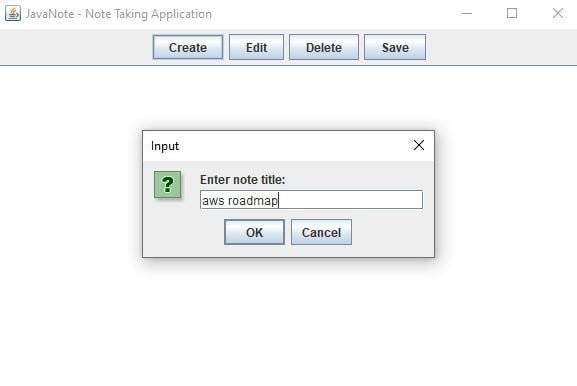
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …