Parking System using Java With Source Code
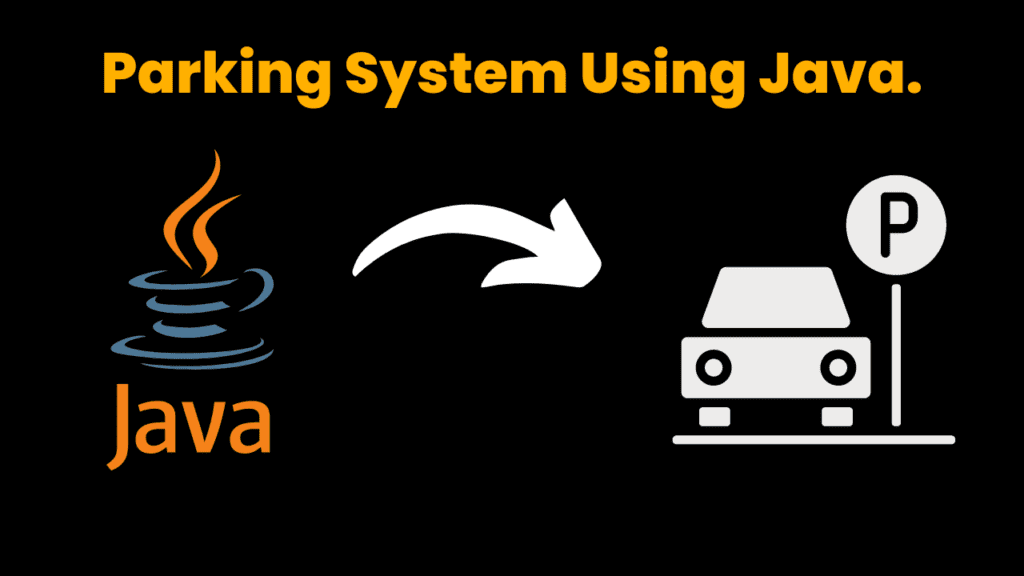
Introduction:
Welcome to Car Parking System using Java! This system allows you to make your task to park the cars easy. Java is a popular programming language that is widely used for developing parking management systems due to its simplicity, versatility, and platform independence. This system analyses for an available space in parking area and on the basis of it gives the output to user. If all slots are full then it will simply tell the user to not park car in that particular area. It increments the available slots if any user removed his/her car from parking area and decrements slots if any user park his/her car. Overall, a parking system in Java provides a flexible and powerful platform for managing parking spaces, improving traffic flow, and enhancing the overall parking experience for drivers. By leveraging the strengths of Java, developers can create robust and scalable parking systems that meet the unique needs of their users.
Explanation:
The Parking System we will build will be a simple console-based application that allows users to park their cars, removed their cars and view all parked cars. It presents a menu with four options: park a car, remove a car, view parked cars, and exit the program. It is very simple with coding point of view as it has only one java class in it. We need to import Scanner class and Array List class. Scanner class used to take input through the keyboard from user while Array List is used to make things simple for adding the cars and deleting them. User needs to provide total number of parking slots and then main menu will be displayed for further operations. In park car method, we will check for availability of slots. If slots are available then user will provide car license number and message will be displayed as “Car parked successfully”. In remove car method, we will check for number of parked cars if it appears as zero then process will not go further. By entering license number of parked car one can simply removed it. Inbuilt functions of Array List ‘add’ and ‘remove’ are used to add cars in array list and remove it from the array list, respectively. Along with the process of adding and removing the available slots will get decrement and increment accordingly. For displaying all parked cars we have used for each loop and displaying Array List named as parked cars. All these three methods have been called for particular case of switch case. We have tried our best to make a code as simple as possible for better understanding. Just some basic knowledge of java and even beginners can code it easily. Happy Coding!
Source Code:
import java.util.ArrayList;
import java.util.Scanner;
public class ParkingSystem {
static int totalSlots, availableSlots;
static ArrayList parkedCars = new ArrayList();
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the total number of parking slots:");
totalSlots = sc.nextInt();
availableSlots = totalSlots;
while (true) {
System.out.println("\nWhat would you like to do?");
System.out.println("1. Park a car");
System.out.println("2. Remove a car");
System.out.println("3. View parked cars");
System.out.println("4. Exit");
int choice = sc.nextInt();
switch (choice) {
case 1:
parkCar();
break;
case 2:
removeCar();
break;
case 3:
viewParkedCars();
break;
case 4:
System.exit(0);
default:
System.out.println("Invalid choice. Please try again.");
}
}
}
public static void parkCar() {
if (availableSlots == 0) {
System.out.println("Sorry, there are no available parking slots.");
return;
}
Scanner sc = new Scanner(System.in);
System.out.println("Enter the license plate number of the car:");
String licensePlate = sc.nextLine();
parkedCars.add(licensePlate);
availableSlots--;
System.out.println("Car parked successfully. Available slots: " + availableSlots);
}
public static void removeCar() {
if (availableSlots == totalSlots) {
System.out.println("There are no parked cars.");
return;
}
Scanner sc = new Scanner(System.in);
System.out.println("Enter the license plate number of the car to be removed:");
String licensePlate = sc.nextLine();
if (parkedCars.contains(licensePlate)) {
parkedCars.remove(licensePlate);
availableSlots++;
System.out.println("Car removed successfully. Available slots: " + availableSlots);
} else {
System.out.println("The car is not parked here.");
}
}
public static void viewParkedCars() {
if (availableSlots == totalSlots) {
System.out.println("There are no parked cars.");
return;
}
System.out.println("Parked cars:");
for (String licensePlate : parkedCars) {
System.out.println(licensePlate);
}
}
}
Output:
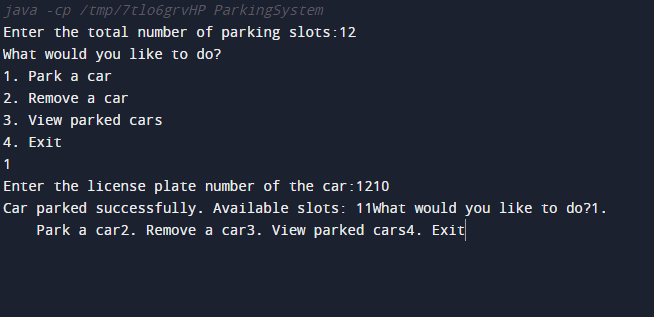
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …