Temperature Convertor Using Java
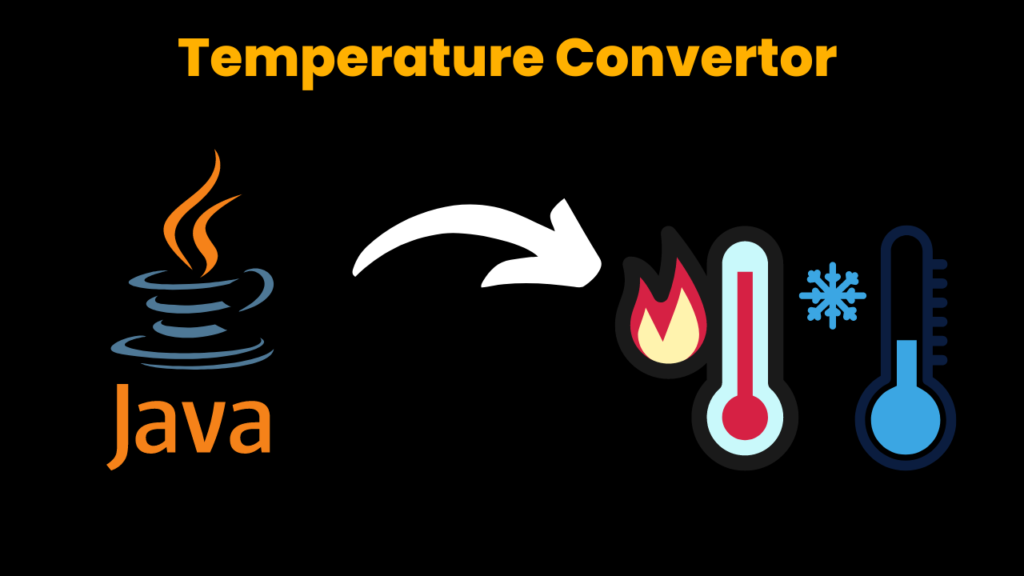
Introduction:
In this project, we will show how to build a Temperature Convertor using Java. This Temperature Converter allows to convert the temperature in various units like Celsius, Fahrenheit and Kelvin. This is the Command Line user Interface(CLI) based application. This system is build with an easy and simple design with Command Line user Interface. So grab a seat, pour a cup of coffee and start converting temperatures using Temperature Converter!
Explanation:
This Temperature Converter is a Command Line user Interface that allows you to easily convert the temperatures in various Units like celsius, Fahrenheit and Kelvin. Users may very quickly convert temperatures using this Temperature Converter. The temperature converter has very simple design with options like celsius to Fahrenheit, Fahrenheit to celsius, celsius to Kelvin, Kelvin to celsius, Kelvin to Fahrenheit and Fahrenheit to kelvin. The system converts the temperature and display it to the user.
The user may convert the temperature using the options like celsius to Fahrenheit, Fahrenheit to celsius, celsius to Kelvin, Kelvin to celsius, Kelvin to Fahrenheit and Fahrenheit to kelvin. The system will quickly and accurately convert the temperature and display it to user.
Java is used to build this conversion system. The system makes use of while loop, switch case and scanner class. The system will initially display the option to users such as celsius to Fahrenheit, Fahrenheit to celsius, celsius to Kelvin, Kelvin to celsius, Kelvin to Fahrenheit, Fahrenheit to kelvin and exit. Then asks temperature input value and displays the converted temperature to the user.
Mathematical Formulas Used for Temperature Conversion
To convert the temperature in Celsius to Fahrenheit, “(temperature * 9 / 5) + 32” is used.
To convert the temperature in Fahrenheit to Celsius, “(temperature – 32) * 5 / 9” is used.
To convert the temperature in Celsius to Kelvin, “temperature + 273.15” is used.
To convert the temperature in Kelvin to Fahrenheit, “temperature – 273.15” is used.
To convert the temperature in Kelvin to Celsius, “(temperature – 273.15) * 9 / 5 + 32” is used.
To convert the temperature in Fahrenheit, “(temperature – 32) * 5 / 9 + 273.15;” is used.
Source Code:
import java.util.Scanner;
public class temperature_con {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double temperature;
int choice;
while (true) {
System.out.println("Choose conversion type: ");
System.out.println("1. Celsius to Fahrenheit");
System.out.println("2. Fahrenheit to Celsius");
System.out.println("3. Celsius to Kelvin");
System.out.println("4. kelvin to Celsius");
System.out.println("5. Kelvin to Fahrenheit");
System.out.println("6. Fahrenheit to Kelvin");
System.out.println("7. Exit");
choice = sc.nextInt();
switch (choice) {
case 1:
System.out.println("Enter the temperature: ");
temperature = sc.nextDouble();
double CF = (temperature * 9 / 5) + 32;
System.out.println(temperature + " Celsius is equal to " + CF + " Fahrenheit.");
break;
case 2:
System.out.println("Enter the temperature: ");
temperature = sc.nextDouble();
double FC = (temperature - 32) * 5 / 9;
System.out.println(temperature + " Fahrenheit is equal to " + FC + " Celsius.");
break;
case 3:
System.out.println("Enter the temperature: ");
temperature = sc.nextDouble();
double CK = temperature + 273.15;
System.out.println(temperature + " Celsius is equal to " + CK + " Kelvin.");
break;
case 4:
System.out.println("Enter the temperature: ");
temperature = sc.nextDouble();
double KC = temperature - 273.15;
System.out.println(temperature + " Kelvin is equal to " + KC + " Celsius.");
break;
case 5:
System.out.println("Enter the temperature: ");
temperature = sc.nextDouble();
double KF = (temperature - 273.15) * 9 / 5 + 32;
System.out.println(temperature + "Kelvin is equal to " + KF + " Fahrenheit.");
break;
case 6:
System.out.println("Enter the temperature: ");
temperature = sc.nextDouble();
double FK = (temperature - 32) * 5 / 9 + 273.15;
System.out.println(temperature + " Fahrenheit is equal to " + FK + " Kelvin.");
break;
case 7:
System.out.println("Thankyou for visiting");
System.exit(0);
default:
System.out.println("Invalid choice.");
break;
}
}
}
}
Output:
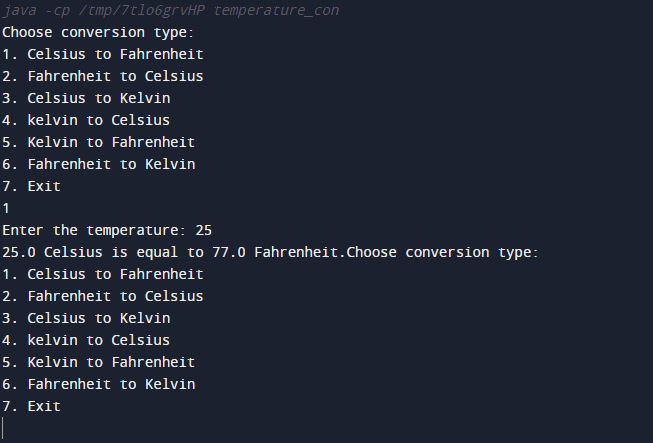
Find More Projects
Hostel Management System Using Python With Source Code by using Python Graphical User Interface GUI Introduction: Managing a hostel efficiently can be …
Contra Game Using Python with source code using Pygame Introduction: Remember the super fun Contra game where you fight aliens and jump …
Restaurant Billing Management System Using Python with Tkinter (Graphical User Interface) Introduction: In the bustling world of restaurants, efficiency is paramount, especially …
Jungle Dash Game Using Python with source code with pygame module Introduction: Dive into the excitement of jungle adventures with our new …
Building a Tetris Game with Python with source code Introduction: Welcome to the world of Tetris, where falling blocks meet strategic maneuvers …
Super Mario Game Using Python with source code Introduction: Remember playing Super Mario as a kid? It’s that classic game where you …