Library Management System Using Java Swing With Source Code
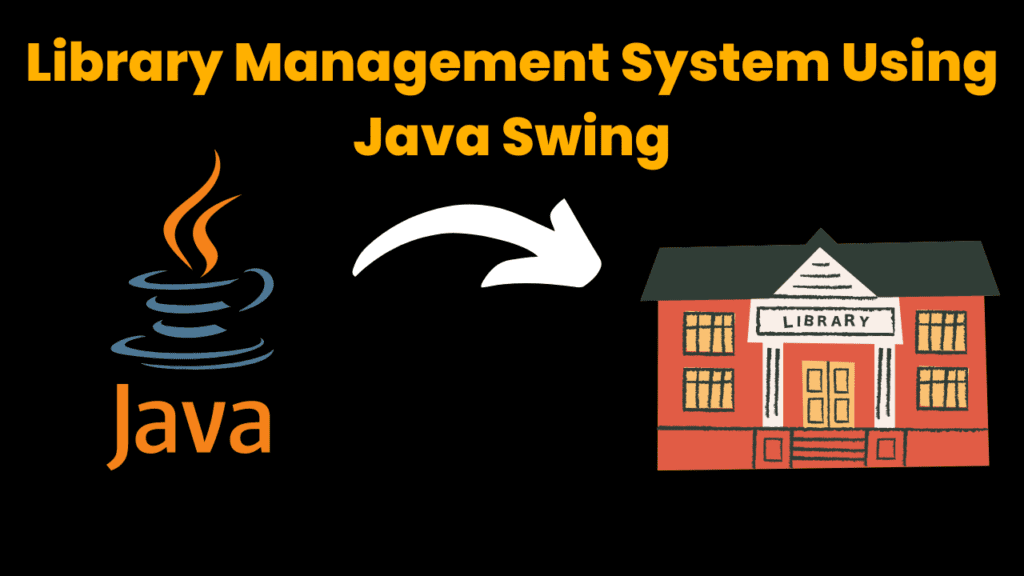
Introduction:
In this project, we will demonstrate how to develop Library Management System using Java Swing library. This Library Management System allows you to add books details using a graphical user interface (GUI) application. This system is build using the Java Swing package which gives a flexible set of components that can be use to build a Graphical user interface(GUI). This application has an easy and simple design with buttons to add books, view books list, edit the details, delete the book, clear the text fields and exit application. So take a seat, pour a cup of coffee and start using Library management system.
Explanation:
This library management System is a Graphical user interface(GUI) system that allows user to easily add the book details, view the list of books, edit the details, delete the book record, clear the fields and exit the application using buttons. Users may very quickly add, view, edit and delete the books using the system’s simple design. The library management System has simple design with buttons and Text Fields. The user can enter details in Text field and then can add the book using add button.
The users may add book details using ‘add’ button, view list of added books using ‘view’ button, edit details using ‘edit’ button, delete record using ‘delete’ button, reset the fields using ‘clear’ button and can exit the application using ‘exit’ button. The user can enter details in Text field and then can add the book using add button.
The Java Swing library which gives a flexible and powerful set of components is used to develop the Library Management System. The system makes use of Swing components such as JLabel, JTextField, JButton, JTable and JPanel among many other Swing components. Button event is handled by the ActionListener interface and button events are defined using ActionListener’s actionPerformed() method.
Methods used in the project:
actionPerformed():
The action or events of the buttons are handled using this method. Buttons in the system to add the book details, to view the list of books, to edit the details, to delete the book record, to clear the fields and to exit the application are handled using this method. This method describe how the components should behave after the button is click.
Source Code:
Get Discount on Top Educational Courses
//@codewithcurious.com
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.*;
public class Library_management extends JFrame implements ActionListener {
private JLabel label1, label2, label3, label4, label5, label6, label7;
private JTextField textField1, textField2, textField3, textField4, textField5, textField6, textField7;
private JButton addButton, viewButton, editButton, deleteButton, clearButton,exitButton;
private JPanel panel;
private ArrayList books = new ArrayList();
public Library_management() {
setTitle("Library Management System");
setSize(600, 300);
setDefaultCloseOperation(EXIT_ON_CLOSE);
label1 = new JLabel("Book ID");
label2 = new JLabel("Book Title");
label3 = new JLabel("Author");
label4 = new JLabel("Publisher");
label5 = new JLabel("Year of Publication");
label6 = new JLabel("ISBN");
label7 = new JLabel("Number of Copies");
textField1 = new JTextField(10);
textField2 = new JTextField(20);
textField3 = new JTextField(20);
textField4 = new JTextField(20);
textField5 = new JTextField(10);
textField6 = new JTextField(20);
textField7 = new JTextField(10);
addButton = new JButton("Add");
viewButton = new JButton("View");
editButton = new JButton("Edit");
deleteButton = new JButton("Delete");
clearButton = new JButton("Clear");
exitButton=new JButton("Exit");
addButton.addActionListener(this);
viewButton.addActionListener(this);
editButton.addActionListener(this);
deleteButton.addActionListener(this);
clearButton.addActionListener(this);
exitButton.addActionListener(this);
panel = new JPanel(new GridLayout(10,2));
panel.add(label1);
panel.add(textField1);
panel.add(label2);
panel.add(textField2);
panel.add(label3);
panel.add(textField3);
panel.add(label4);
panel.add(textField4);
panel.add(label5);
panel.add(textField5);
panel.add(label6);
panel.add(textField6);
panel.add(label7);
panel.add(textField7);
panel.add(addButton);
panel.add(viewButton);
panel.add(editButton);
panel.add(deleteButton);
panel.add(clearButton);
panel.add(exitButton);
add(panel);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addButton) {
String[] book = new String[7];
book[0] = textField1.getText();
book[1] = textField2.getText();
book[2] = textField3.getText();
book[3] = textField4.getText();
book[4] = textField5.getText();
book[5] = textField6.getText();
book[6] = textField7.getText();
books.add(book);
JOptionPane.showMessageDialog(this, "Book added successfully");
clearFields();
}
else if (e.getSource() == viewButton) {
String[] columns = {"Book ID", "Book Title", "Author", "Publisher", "Year of Publication", "ISBN", "Number of Copies"};
Object[][] data = new Object[books.size()][7];
for (int i = 0; i < books.size(); i++) {
data[i][0] = books.get(i)[0];
data[i][1] = books.get(i)[1];
data[i][2] = books.get(i)[2];
data[i][3] = books.get(i)[3];
data[i][4] = books.get(i)[4];
data[i][5] = books.get(i)[5];
data[i][6] = books.get(i)[6];
}
JTable table = new JTable(data, columns);
JScrollPane scrollPane = new JScrollPane(table);
JFrame frame = new JFrame("View Books");
frame.add(scrollPane);
frame.setSize(800, 400);
frame.setVisible(true);
}
else if (e.getSource() == editButton) {
String bookID = JOptionPane.showInputDialog(this, "Enter book ID to edit:");
for (int i = 0; i < books.size(); i++) {
if (books.get(i)[0].equals(bookID)) {
String[] book = new String[7];
book[0] = bookID;
book[1] = textField2.getText();
book[2] = textField3.getText();
book[3] = textField4.getText();
book[4] = textField5.getText();
book[5] = textField6.getText();
book[6] = textField7.getText();
books.set(i, book);
JOptionPane.showMessageDialog(this, "Book edited successfully");
clearFields();
return;
}
}
JOptionPane.showMessageDialog(this, "Book not found");
}
else if (e.getSource() == deleteButton) {
String bookID = JOptionPane.showInputDialog(this, "Enter book ID to delete:");
for (int i = 0; i < books.size(); i++) {
if (books.get(i)[0].equals(bookID)) {
books.remove(i);
JOptionPane.showMessageDialog(this, "Book deleted successfully");
clearFields();
return;
}
}
JOptionPane.showMessageDialog(this, "Book not found");
}
else if (e.getSource() == clearButton) {
clearFields();
}
else if (e.getSource() == exitButton) {
System.exit(0);
}
}
private void clearFields() {
textField1.setText("");
textField2.setText("");
textField3.setText("");
textField4.setText("");
textField5.setText("");
textField6.setText("");
textField7.setText("");
}
public static void main(String[] args) {
new Library_management();
}
}
Output :
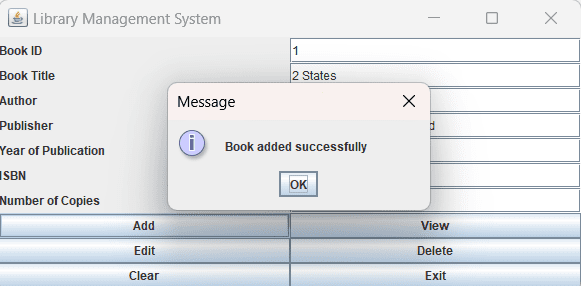
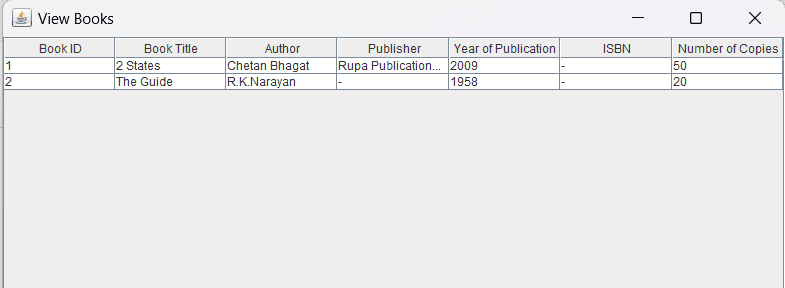
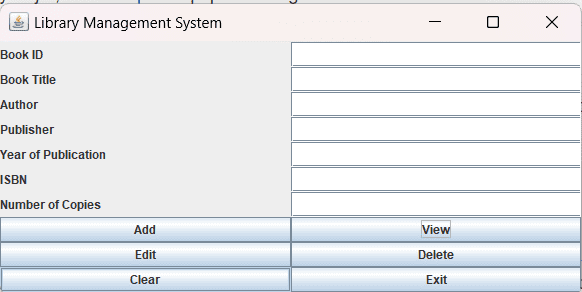
Find More Projects
resume screener in python using python introduction The hiring process often begins with reviewing numerous resumes to filter out the most suitable …
expense tracer in python using GUI introduction Tracking daily expenses is a vital part of personal financial management. Whether you’re a student …
my personal diary in python using GUI introduction Keeping a personal diary in python is one of the oldest and most effective …
interview question app in python using GUI introduction In today’s rapidly evolving tech landscape, landing a job often requires more than just …
sudoko solver in python using GUI introduction Sudoku, a classic combinatorial number-placement puzzle, offers an engaging challenge that blends logic, pattern recognition, …
handwritten digit recognizer in python introduction In an era where artificial intelligence and deep learning are transforming industries, real-time visual recognition has …