Weather App using HTML, CSS, JavaScript & ReactJs With Source Code
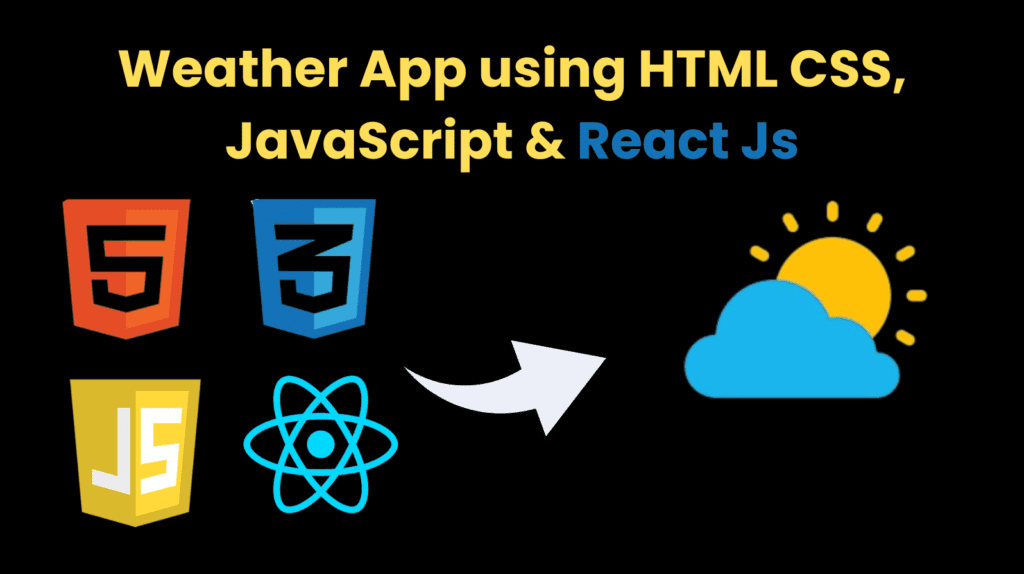
Introduction :
The React Weather App is a web application designed to provide users with up-to-date weather information for various cities around the world. Leveraging React.js, a popular JavaScript library for building user interfaces, this app offers an intuitive and dynamic user experience. By integrating with the OpenWeatherMap API, users can access real-time weather data simply by inputting the name of a city.
The primary goal of the React Weather App is to deliver accurate weather forecasts to users in a convenient and accessible manner. Key functionalities include:
City Input: Users can enter the name of a city into the app’s interface.
Weather Data Retrieval: Upon submitting a city name, the app asynchronously fetches weather data from the OpenWeatherMap API.
Dynamic Display: The app dynamically updates its interface to display the retrieved weather information, including temperature, weather conditions, and corresponding weather icons.
Visual Representation: Weather conditions are visually represented using custom icons corresponding to various weather states such as sunny, cloudy, rainy, etc.
The React Weather App utilizes a modern technology stack to achieve its functionality:
React.js: The core of the application, React.js facilitates the creation of reusable UI components and manages the app’s state and rendering logic efficiently.
styled-components: Styling is handled using styled-components, a CSS-in-JS library that allows developers to write CSS directly within their JavaScript code.
Axios: For making HTTP requests to the OpenWeatherMap API, Axios, a promise-based HTTP client, is employed to fetch weather data asynchronously.
OpenWeatherMap API: The app leverages the OpenWeatherMap API to access real-time weather data based on the user’s input city.
Explanation :
App.js
Imports:
- React: To define React components and utilize React hooks like useState.
- styled-components: For styling components with CSS-in-JS approach.
- Axios: To make HTTP requests for fetching weather data.
- CityComponent and WeatherComponent: Custom components for handling city input and displaying weather information.
WeatherIcons Object:
- This object maps weather condition codes to corresponding icon paths.
Container styled-component:
- It defines the styling for the main container of the app.
AppLabel styled-component:
- This is a styled span element for displaying the label of the app.
CloseButton styled-component:
- Although it’s defined, it’s not used in the current code.
App Component:
- It’s a functional component named “App”.
- Uses React’s useState hook to manage state for city and weather data.
- Defines a fetchWeather function that makes an asynchronous call to the OpenWeatherMap API to fetch weather data based on the entered city.
- Renders a Container component that holds either the WeatherComponent or CityComponent based on whether city and weather data are available.
index.js
Imports:
- React: Required for defining React components.
- ReactDOM: Necessary for rendering React components into the DOM.
- ‘./index.css’: Imports the CSS file for styling.
- App: Imports the main component of the application.
ReactDOM.render():
- This function renders the root component
<App />
into the DOM. - It’s wrapped in
<React.StrictMode>
for additional development mode checks and warnings. - The rendered component is placed inside the HTML element with the id ‘root’, which is typically a
<div>
element in the HTML file where the React app is mounted.
- This function renders the root component
JavaScript Logic:
- useState hook is used to manage state for city and weather data.
- fetchWeather function makes an asynchronous HTTP request using Axios to fetch weather data based on the entered city.
- Conditional rendering is employed to display either the CityComponent or WeatherComponent based on the availability of city and weather data.
Purpose of Functions:
- useState: To manage state variables for city and weather data.
- fetchWeather: To fetch weather data from the OpenWeatherMap API based on the entered city.
- App Component: To render the main structure of the Weather App and manage state transitions between CityComponent and WeatherComponent.
Instructions to run this project :
Step 01 : Download the zip file of source code (given below) and extract it.
Step 02 : Navigate to the Project Directory:
- Once the repository is cloned, navigate into the project directory using the
cd
command: cd <project_directory> - Replace
<project_directory>
with the name of the directory where the project was cloned.
Step 03 : Install Dependencies:
- Inside the project directory, run the following command to install project dependencies using Yarn : yarn install
- This command will read the
package.json
file and install all required dependencies listed in it.
Step 04 : Run the Project:
- After installing dependencies, you can start the development server using the following command: yarn start
- This command will start the development server and automatically open the project in your default web browser.
- Once the development server is up and running, you can access the project by opening your web browser and navigating to the specified address (usually
http://localhost:3000
). - You can now explore the e-commerce app, navigate through different pages, interact with components, and experience the functionality firsthand.
Get Discount on Top Educational Courses
Source Code :
Output :
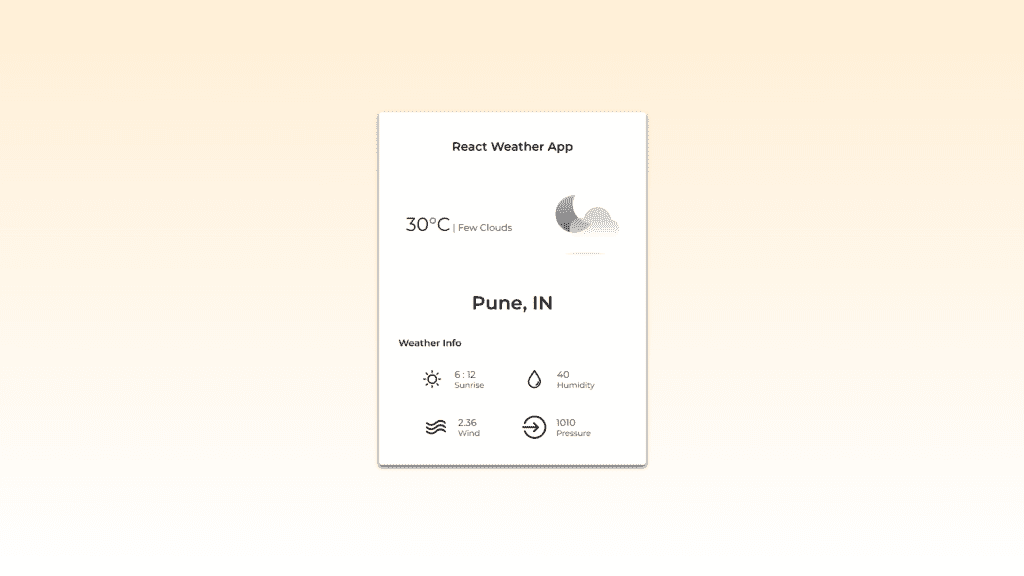
Find More Projects
how to create a video background with html CSS JavaScript Introduction Hello friends, you all are welcome to today’s new blog post. …
Auto Text Effect Animation Using Html CSS & JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …