Currency Converter using HTML, CSS and JavaScript With Source Code
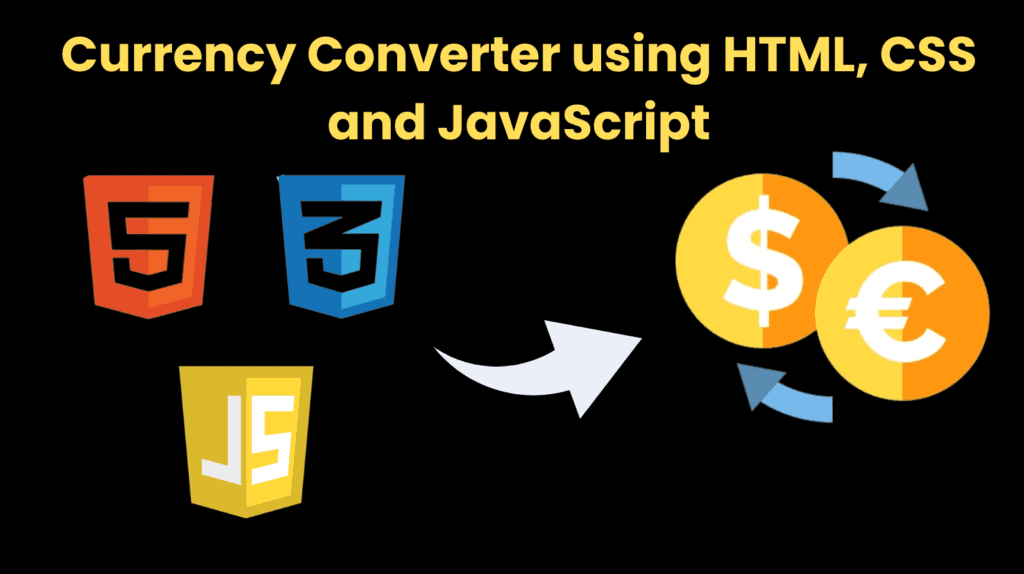
Introduction :
This Currency Converter is a web application created using HTML, CSS, and JavaScript. It allows users to convert an amount from one currency to another using real-time exchange rates fetched from an API (exchangerate-api.com). The Currency Converter is a dynamic web application that leverages the power of HTML, CSS, and JavaScript to provide users with a seamless and intuitive platform for converting currency values. Built with a modern and responsive design, the application ensures an optimal user experience across various devices. The application integrates with the exchangerate-api.com
API to fetch up-to-date exchange rates. This API serves as a crucial component, enabling the Currency Converter to deliver accurate and reliable conversion results based on the latest global currency exchange values. It supports a comprehensive list of currencies, giving users the flexibility to convert between a wide range of international monetary units. The dropdown menus are populated with currency codes, making the selection process straightforward for users. Upon user interaction, the application dynamically fetches exchange rates from the API, calculates the converted amount based on the selected currencies and user-entered value, and presents the result in a clear and visually appealing manner.
Explanation :
HTML Structure:
- The HTML file consists of the standard structure with a
<head>
section containing meta tags, title, and external stylesheet and scripts links. - Bootstrap and Google Fonts are imported for styling purposes.
- The body contains a heading, a form with input fields and dropdowns for currency selection, convert and reset buttons, and a section to display the converted amount.
CSS (style.css):
- The styling is kept minimal, and Bootstrap classes are utilized for layout and responsiveness.
JavaScript Logic (script.js):
- The API endpoint (
api
) is defined for fetching exchange rates. The API used here ishttps://api.exchangerate-api.com/v4/latest/USD
. - Various DOM elements are selected using
document.querySelector
and stored in variables for later use. - Event listeners are added to the “From” and “To” dropdowns to update the selected currencies (
resultFrom
andresultTo
) when the user changes them. - An input event listener (
updateValue
) is added to the input field to capture the amount entered by the user. - When the convert button is clicked, the
getResults
function is called. - Inside
getResults
, a fetch request is made to the API, and the response is processed in thedisplayResults
function. - The exchange rates for the selected currencies are used to calculate the converted amount, which is then displayed in the designated HTML element (
finalValue
). - A reset button (
clearVal
) is implemented to reload the page and clear the converted amount.
Currency Selection:
The Currency Converter supports a comprehensive list of currencies, giving users the flexibility to convert between a wide range of international monetary units. The dropdown menus are populated with currency codes, making the selection process straightforward for users.
Conversion Logic:
Upon user interaction, the application dynamically fetches exchange rates from the API, calculates the converted amount based on the selected currencies and user-entered value, and presents the result in a clear and visually appealing manner.
Source Code :
Get Discount on Top Educational Courses
HTML (index.html)
Currency Converter
Currency Converter
Currency Converter
From
Select One …
USD
AED
ARS
AUD
BGN
BRL
BSD
CAD
CHF
CLP
CNY
COP
CZK
DKK
DOP
EGP
EUR
FJD
GBP
GTQ
HKD
HRK
HUF
IDR
ILS
INR
ISK
JPY
KRW
KZT
MVR
MXN
MYR
NOK
NZD
PAB
PEN
PHP
PKR
PLN
PYG
RON
RUB
SAR
SEK
SGD
THB
TRY
TWD
UAH
UYU
ZAR
To
Select One …
USD
AED
ARS
AUD
BGN
BRL
BSD
CAD
CHF
CLP
CNY
COP
CZK
DKK
DOP
EGP
EUR
FJD
GBP
GTQ
HKD
HRK
HUF
IDR
ILS
INR
ISK
JPY
KRW
KZT
MVR
MXN
MYR
NOK
NZD
PAB
PEN
PHP
PKR
PLN
PYG
RON
RUB
SAR
SEK
SGD
THB
TRY
TWD
UAH
UYU
ZAR
Converted Amount :
CSS (style.css)
body {
background-color: #18FFFF;
background-position: center;
background-size: cover;
background-attachment: fixed;
background-repeat: no-repeat;
background-image: url(global-currency-background_115579-405.avif);
background-position: center;
background-repeat: no-repeat;
background-size: cover;
}
.heading {
/* font-family: 'Pacifico', cursive; */
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
font-weight: bold;
font-size: 60px;
color: antiquewhite;
margin: 35px auto 20px;
}
hr {
border-top: 2px solid black;
width: 40%;
margin-bottom: 55px;
}
.main {
width: 50vw;
margin: auto;
padding: 30px;
border-radius: 5px;
background-color: rgba(0, 0, 0, 0.5);
color: white;
}
label {
font-size: 20px;
}
.btn {
width: 200px;
}
#finalAmount {
font-family: 'Lobster', cursive;
display: none;
margin: 50px auto;
}
#finalAmount h2 {
font-size: 50px;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
color: antiquewhite;
}
.finalValue {
font-family: 'Amiri', serif;
color: antiquewhite;
}
@media (max-width: 768px) {
hr {
width: 60%;
}
.main {
width: 100%;
}
}
@media (max-width: 400px) {
.heading {
font-size: 60px;
}
hr {
width: 75%;
}
#finalAmount h2,
.finalValue {
font-size: 40px;
}
}
JavaScript (script.js)
// include api for currency change
const api = "https://api.exchangerate-api.com/v4/latest/USD";
// for selecting different controls
var search = document.querySelector(".searchBox");
var convert = document.querySelector(".convert");
var fromCurrecy = document.querySelector(".from");
var toCurrecy = document.querySelector(".to");
var finalValue = document.querySelector(".finalValue");
var finalAmount = document.getElementById("finalAmount");
var resultFrom;
var resultTo;
var searchValue;
// Event when currency is changed
fromCurrecy.addEventListener('change', (event) => {
resultFrom = `${event.target.value}`;
});
// Event when currency is changed
toCurrecy.addEventListener('change', (event) => {
resultTo = `${event.target.value}`;
});
search.addEventListener('input', updateValue);
// function for updating value
function updateValue(e) {
searchValue = e.target.value;
}
// when user clicks, it calls function getresults
convert.addEventListener("click", getResults);
// function getresults
function getResults() {
fetch(`${api}`)
.then(currency => {
return currency.json();
}).then(displayResults);
}
// display results after convertion
function displayResults(currency) {
let fromRate = currency.rates[resultFrom];
let toRate = currency.rates[resultTo];
finalValue.innerHTML =
((toRate / fromRate) * searchValue).toFixed(2);
finalAmount.style.display = "block";
}
// when user click on reset button
function clearVal() {
window.location.reload();
document.getElementsByClassName("finalValue").innerHTML = "";
};
Purpose of Functions:
updateValue
: Updates thesearchValue
variable with the amount entered by the user.getResults
: Fetches exchange rates from the API and callsdisplayResults
to display the converted amount.displayResults
: Calculates and displays the converted amount based on the exchange rates and user-entered amount.clearVal
: Reloads the page and clears the converted amount.
Output :
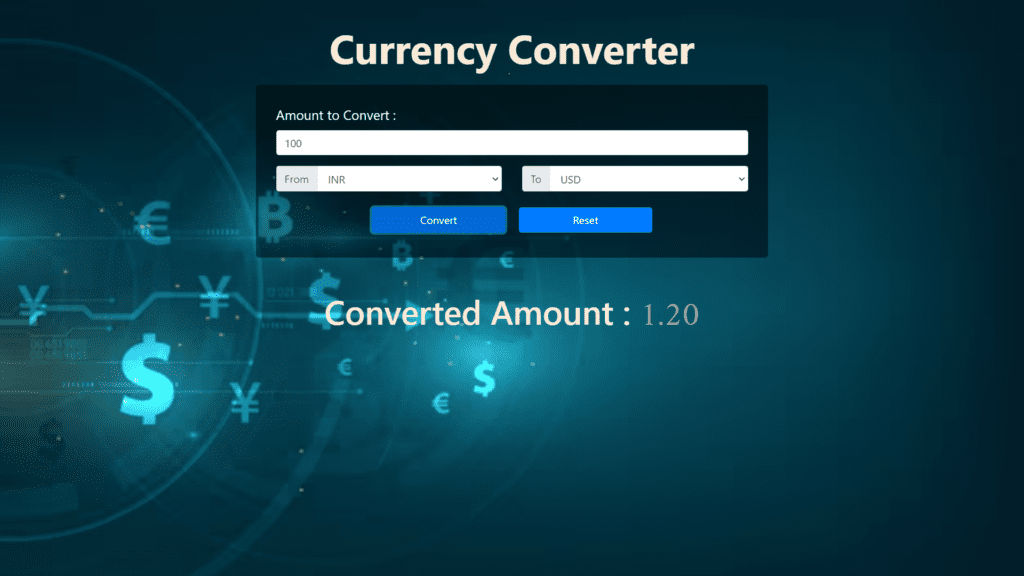
Find More Projects
how to create a video background with html CSS JavaScript Introduction Hello friends, you all are welcome to today’s new blog post. …
Auto Text Effect Animation Using Html CSS & JavaScript Introduction Hello friends, welcome to today’s new blog post. Today we have created …
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …