Typing Challenge Using HTML CSS and JavaScript
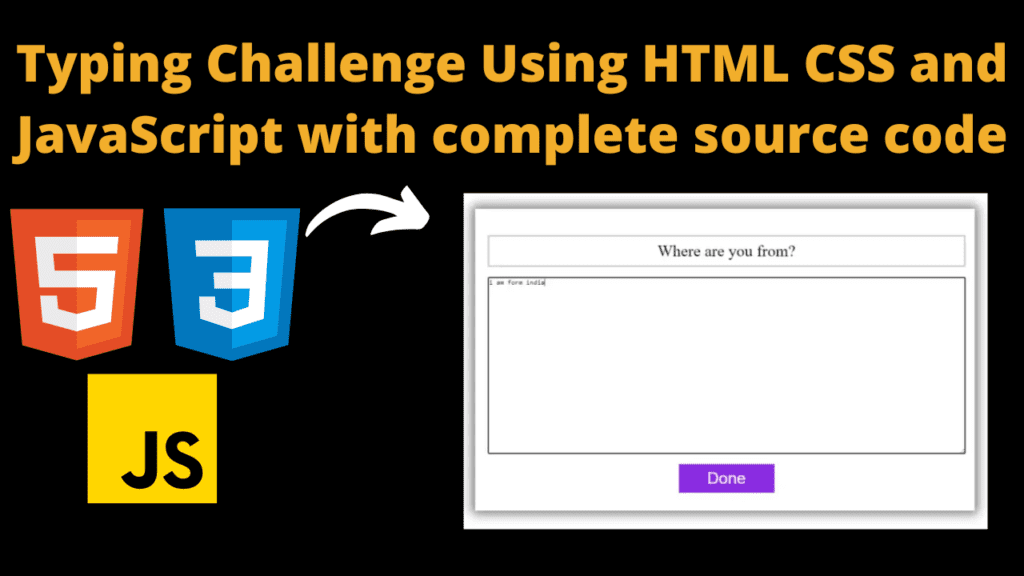
Introduction
Hello friends, all you developer friends are welcome to our new project. If you are also new to the world of coding then you have come to the right website because today we have created a beautiful project for you which is a game which we have created with the help of html, css and javascript. Creating this game is a very easy task. If you have even a little knowledge of coding, you can easily make such games. By making such games, we get a lot of coding knowledge, with the help of which we can create more advanced games and many different projects as much as you have knowledge of coding. The more you gain knowledge of questions, the more beneficial it is for you which will also improve your skills. The game we have made today is quite interesting and useful. It is a typing game in which we get some questions which we have to answer. The faster you answer, the higher will be your score.
Our advantage with this is that we will type quickly to give the answers which will also increase our typing speed which is quite good. So, let’s understand the code of this typing game.
HTML (index.html)
Get Discount on Top Educational Courses
First of all we have used html for our typing game, with which we have created a structure which is not looking good at all right now, to make it look good we have to use css which we will see later, by the way friends we have not written much code in html, but whatever code we have written in html it is very important for us to understand it, we are going to understand only that code which is important for us, so let’s understand the code.
- <title>CodeWithCurious.com – Typing Challenge</title> We have added a title to our game which you can change as per your choice from which you can know what this project is about
- <link rel=”icon” href=”…”> We have added an icon to our game. If you want to change this icon, you can do it. You just have to replace the url of your icon with the url that was entered.
- Friends, as I just told you that you can create structure with HTML, but to design the game, we have to use CSS, so for that we need to link CSS with the HTML file, so <link rel=”stylesheet” href=”style.css” /> you have to use this tag
- Then comes the <body> tag of our HTML in which we are going to write our code. This is necessary for everyone to do this.
- First of all we have created a container <div class=”container”> so that we don’t have any problem in designing the typing game
- <div class=”main”></div> We have used another <div> because in this container we will show our question to the user with the help of JavaScript
- <textarea name=”words” class=”typingArea”></textarea> We have used text area because whatever question the user will answer in this, he can do it inside this.
- <br /> We have used this tag so that our element comes in the next line
- <button class=”btn”>Start</button> We have also created a button which will start our game
- To link our JavaScript file to html we have used <script src=”app.js”></script> element which is very important
CodeWithCurious.com - Typing Challenge
CSS (Style.css)
Now we have created the structure of the game with the help of HTML but it does not look good at all, so to design it well we have to take the help of CSS. With the help of CSS we can also make a very good animated game, so let’s understand the CSS code now.
- First of all we have flexed <body> display: flex; so that all our elements are in one line
- justify-content: center; in our game we have Content placed all the in the center
- After centering the content, we centered all the items in our game with align-items: center; which gives a slightly better design
- We have centered all text in the game text-align: center;
- We have created a container that will contain our game code. Width of this container: 70%; we have kept it like this
- In the container we have used some padding padding: 10px;
- We have created another <div> in our game in which some of our quotations are displayed, the text of which we have placed in the center text-align: center; and have used padding padding: 10px; , font size font-size: 2em; and border: 3px solid white; have added border 3px and have kept the color white
- We have kept the width of text area width: 100%; full and the height as height: 350px; 350px We have kept 20px from text area top margin-top: 20px;
- We have kept the width of the button 20%; For background we have used this color background-color: blueviolet;
body {
display: flex;
justify-content: center;
align-items: center;
text-align: center;
}
.container {
width: 70%;
padding: 10px;
}
.main {
text-align: center;
padding: 10px;
font-size: 2em;
border: 3px solid white;
}
.typingArea {
width: 100%;
height: 350px;
margin-top: 20px;
}
.btn {
width: 20%;
outline: none;
border: none;
font-size: 2em;
padding: 10px;
color: white;
background-color: blueviolet;
margin-top: 20px;
}
Javascript (Script.js)
Friends, the code that you are getting to see below is the JavaScript code which is an important part of our game, without it you can make a game but no user can play that game. We have added some questions in our game which are displayed with the help of JavaScript. When a user enters the game, when he starts the game, then he is shown a random question for which the user has to write the answer. The faster he writes the answer, the higher the score he gets and it is also important that the answer is correct.
- First we target the date of our HTML which is named”main”. const i = document.querySelector(“.i”);
- We have done the same in the other <div> as well, but friends, we can target only one element with one “id”. If you make some mistake then there can be a problem in running your code.
- const typeArea = document.querySelector(“.typingArea”);
const btn = document.querySelector(“.btn”); - “Cust Words” We have added some questions using custom words, you can edit them
const main = document.querySelector(".main");
const typeArea = document.querySelector(".typingArea");
const btn = document.querySelector(".btn");
const words = [
"A day in the life of programmer",
"What is JavaScript?",
"What is React?",
"What is Programming Language?",
"What's your name?",
"Where are you from?",
"This is just random word",
"What is Remix.js?",
"New Technologies",
"Is programming hard?",
"Why do you wanna become a programmer?",
"Which programming language you like the most?",
"What is Golang? and why do you wanna learn it?",
"What is CSS",
];
const game = {
start: 0,
end: 0,
user: "",
arrText: "",
};
btn.addEventListener("click", () => {
if (btn.textContent === "Start") {
play();
typeArea.value = "";
typeArea.disabled = false;
} else if (btn.textContent === "Done") {
typeArea.disabled = true;
main.style.borderColor = "white";
end();
}
});
function play() {
let randText = Math.floor(Math.random() * words.length);
main.textContent = words[randText];
game.arrText = words[randText];
main.style.borderColor = "#c8c8c8";
btn.textContent = "Done";
const duration = new Date();
game.start = duration.getTime(); // unix timestamp
}
function end() {
const duration = new Date();
game.end = duration.getTime();
const totalTime = (game.end - game.start) / 1000;
game.user = typeArea.value;
const correct = results();
main.style.borderColor = "white";
main.innerHTML = `Time: ${totalTime} Score: ${correct.score} out of ${correct.total}`;
btn.textContent = "Start";
}
function results() {
let valueOne = game.arrText.split(" ");
let valueTwo = game.user.split(" ");
let score = 0;
valueOne.forEach((word, idx) => {
if (word === valueTwo[idx]) {
score++;
}
});
return { score, total: valueOne.length };
}
Output:
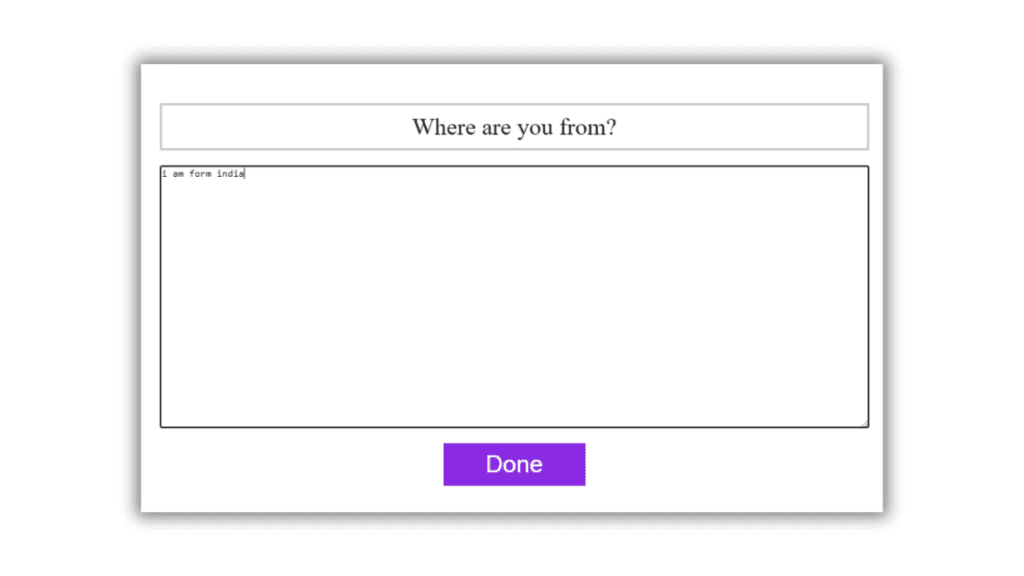
Disclaimer: The code provided in this post is sourced from GitHub and is distributed under the MIT License. All credits for the original code go to the respective owner. We have shared this code for educational purposes only. Please refer to the original repository for detailed information and any additional usage rights or restrictions.
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …