Detect Plagiarism in files using Python With Source Code
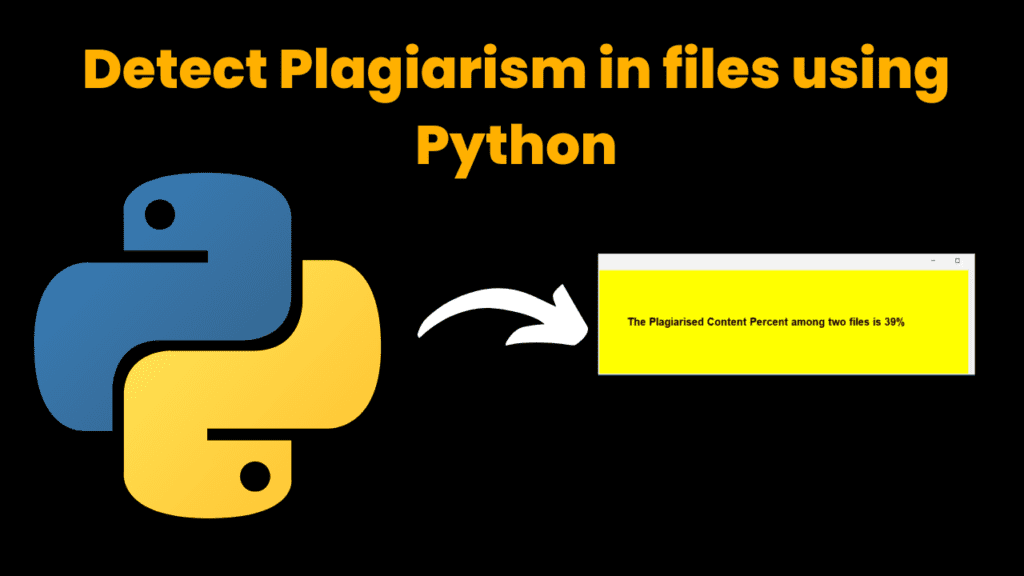
Introduction
Hello Curious Coders,
In this project we are going to discuss how to check the plagiarism between the
contents present in two different text files. In python this can be done using
predefined package difflib but we are going to check it manually. Let’s get into it….
Source Code :
# import required library
from tkinter import *
# First we need to read the contents of two files
with open('File_1.txt') as f1:
s1=f1.read().lower().split()
l1=[]
for i in s1:
if i.isalnum():
l1.append(i)
with open('File_2.txt') as f2:
s2=f2.read().lower().split()
l2=[]
for i in s2:
if i.isalnum():
l2.append(i)
# Finding how many words are common in two files
plag_words=len(set(l1).intersection(set(l2)))
# Finding total number of words in two files
total_words=len(l1)+len(l2)
# Formula to calculate the plagarism percent
plag_percent=100-round((total_words-plag_words*2)/total_words*100)
# Displaying the result
result=" The Plagarized Content Percent among two files is "+str(plag_percent)+"%"
if plag_percent30 and plag_percent<=60:
win= Tk()
win.geometry("800x200")
canvas= Canvas(win, width= 700, height= 650, bg="Yellow")
canvas.create_text(300, 100, text=result, fill="black", font=('Helvetica 15 bold'))
canvas.pack()
win.mainloop()
else:
win= Tk()
win.geometry("800x200")
canvas= Canvas(win, width= 700, height= 650, bg="Red")
canvas.create_text(300, 100, text=result, fill="black", font=('Helvetica 15 bold'))
canvas.pack()
win.mainloop()
Code Explanation :
1. First we read the contents of two files using open() method
2. We read the contents of lines using read() function which return as string.
So we splitted that string into list of words by excluding punctuation marks(,).
3. We extacted the common words from two lists by conveting them to sets.
4. Next we calcualted the length of plagarised words and total words present in two files.
5. Finally we applied a formual there to calculate the plagarised content from two files and printed it on the screen
Output :
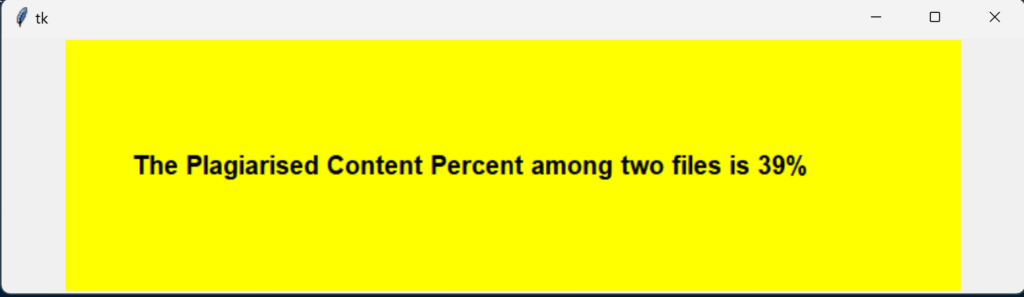
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …