Shape Clicker Game Using HTML CSS And JavaScript
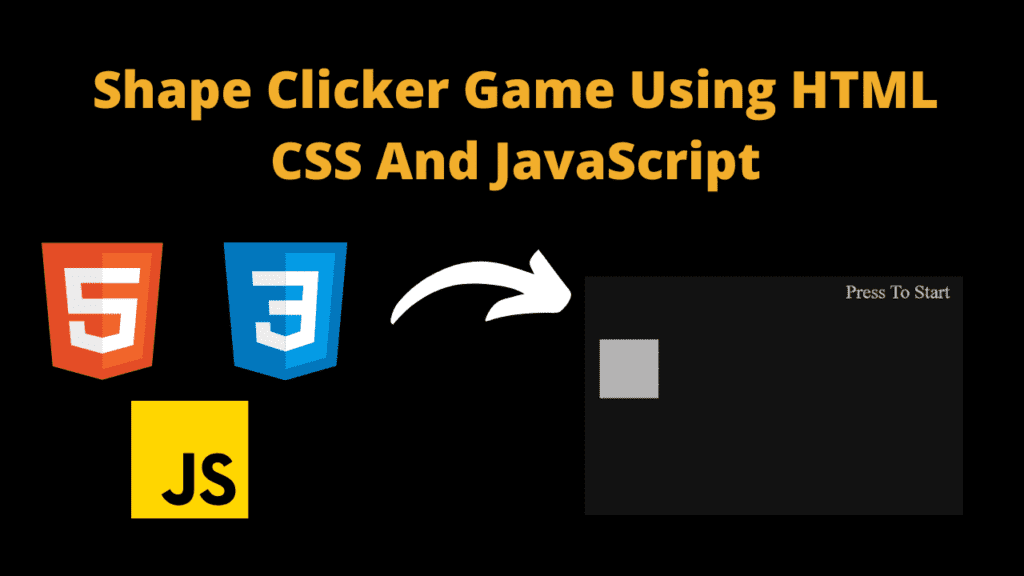
Introduction
Hey coders, welcome to another new blog. Today in this article we’ve made a simple shape clicker game which is a dynamic and easy to build game. In this game, the different shapes displays on your screen and you have to simply just click on them. The heading will display your clicking time on the shape.
We’ve used HTML CSS and JavaScript to build this shape clicker game. Using HTML we’ve set the structure of the game. After the HTML code we’ve designed the shape clicker game. CSS designs our shape clicker game. In the end the JavaScript code adds functionality to our game makes it more interactive.
If you want to build this type of game, all you need is the knowledge and basics of HTML CSS and JavaScript. If you’re good enough in these 3 technologies then you’re good to go. This game is very simple and basic game but it is dynamic as well. Let’s see and understand code of our game.
HTML (index.html)
Get Discount on Top Educational Courses
This is our short and simple HTML code which sets the structure of our game. As you can see our code is not that too long. Using this code we’ve build the basic structure of our game. Let’s understand the code.
- <!DOCTYPE html> : This defines the type of our document and ensures that our file is a HTML code document file.
- <title> : The title tag sets the title of our webpage as “Shape Clicker Game”.
- <link> : The link tag adds external files to our code. It adds a favicon file for our webpage and links a external CSS file in our document.
- <body> : This is the main part of our code as it contains main content of our code.
- <div class=”output”> : This class is to show the output when we click on any shape. We’ll edit this later in our JavaScript code.
- <script> : This tag links external JavaScript file to our code which adds functionality to our game.
CodeWithCurious.com - Shape Clicker Game
CSS (Style.css)
This is our CSS code which designs our shape clicker game. This is not a long CSS code but this code we’ll implement using JavaScript. Let’s understand the code.
- The body sets a background color of black and text color of white.
- The .message class centers the text using text-align property. It sets the padding of 10px with a font size of 2rem.
- The .box class takes a width and height of 100%. It sets position relative to the parent. 50px from the top and 20% from the left sets to the .box class. A background color of cornsilk, font-size of 1.5em, line height of 100px with a 1px of solid black border set to this class.
This is all about our short CSS code.
body {
background: black;
color: white;
}
/* JavaScript */
.message {
text-align: center;
padding: 10px;
font-size: 2rem;
}
.box {
width: 100px;
height: 100px;
position: relative;
top: 50px;
left: 20%;
background-color: cornsilk;
border: 1px solid black;
font-size: 1.5em;
line-height: 100px;
}
Javascript (Script.js)
This is our JavaScript code which adds functionality in our game. Using this code we’ve made our game functional. Let’s understand our JavaScript code.
- First we creates a object named game using const keyword which stores a timer and start time for tracking user response time.
- Next, we creates a message element which displays “Press to Start” at the top of our game page.
- Then we creates a box element and adds box CSS class to it.
- Next, we append the box element into our output element.
- Next we adds an event listener to the .box element. On the click of .box it hides the box and starts a time for showing new box after a random delay. If it’ll the first click, the message will update otherwise it’ll calculate and shows how long it took the user to click the box.
- randomNumbers function generates random number between 0 and max.
- The addBox function shows box with random size, position, and color in the .output container. It stores the current time which track how long it takes the user to click the box.
This is how our JavaScript code works for the shape clicker game.
const game = { timer: 0, start: null };
// Create Message Element
const message = document.createElement("div");
message.classList.add("message");
message.textContent = "Press To Start";
document.body.prepend(message);
// Create a Box
const box = document.createElement("div");
box.classList.add("box");
const output = document.querySelector(".output");
output.append(box);
box.addEventListener("click", () => {
box.textContent = "";
box.style.display = "none";
game.timer = setTimeout(addBox, randomNumbers(3000));
if (!game.start) {
message.textContent = "Watch for element and click it";
} else {
const current = new Date().getTime();
const duration = (current - game.start) / 1000;
message.textContent = `It took ${duration} seconds to click`;
}
});
function randomNumbers(max) {
return Math.floor(Math.random() * max);
}
function addBox() {
game.start = new Date().getTime();
const container = output.getBoundingClientRect();
const dim = [randomNumbers(50) + 20, randomNumbers(50) + 20];
box.style.display = "block";
box.style.width = `${dim[0]}px`;
box.style.height = `${dim[1]}px`;
box.style.backgroundColor = "#" + Math.random().toString(16).substr(-6);
box.style.left = randomNumbers(container.width - dim[0]) + "px";
box.style.top = randomNumbers(container.height - dim[1]) + "px";
box.style.borderRadius = randomNumbers(50) + "%";
}
Output:
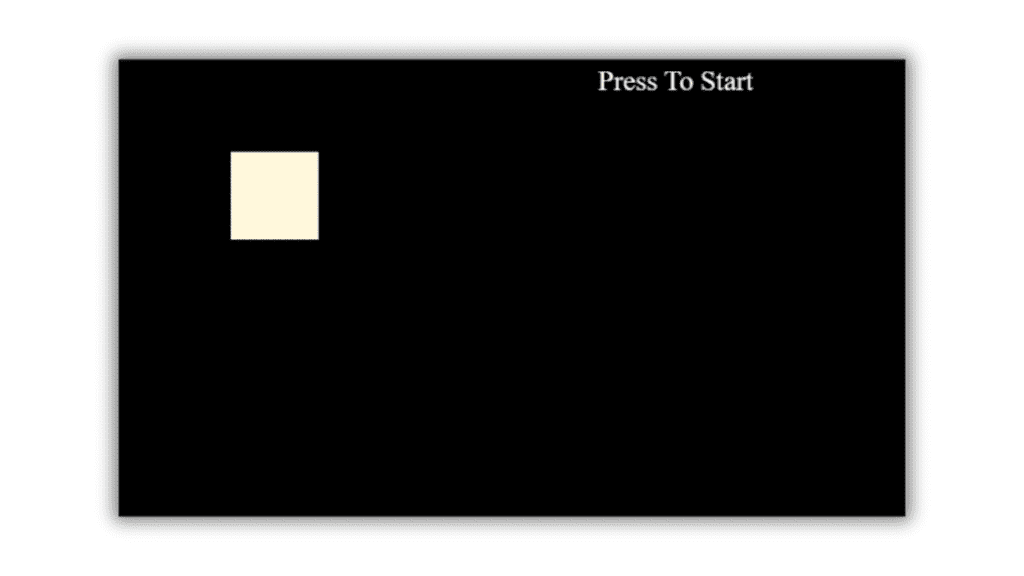
Disclaimer: The code provided in this post is sourced from GitHub and is distributed under the MIT License. All credits for the original code go to the respective owner. We have shared this code for educational purposes only. Please refer to the original repository for detailed information and any additional usage rights or restrictions.
Windows 12 Notepad Using Python Introduction: In this article, we will create a Windows 12 Notepad using Python. If you are a …
Animated Search Bar using Html CSS And JavaScript Introduction Hello friends, all of you developers are welcome to today’s beautiful blog post. …
Best Quiz Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this article we’ll learn …
Tower Blocks Game Using HTML CSS And JavaScript Introduction Hello coders, welcome to another new blog. Today in this blog we’ll learn …