Optical Illusion Game Using Python With Source Code
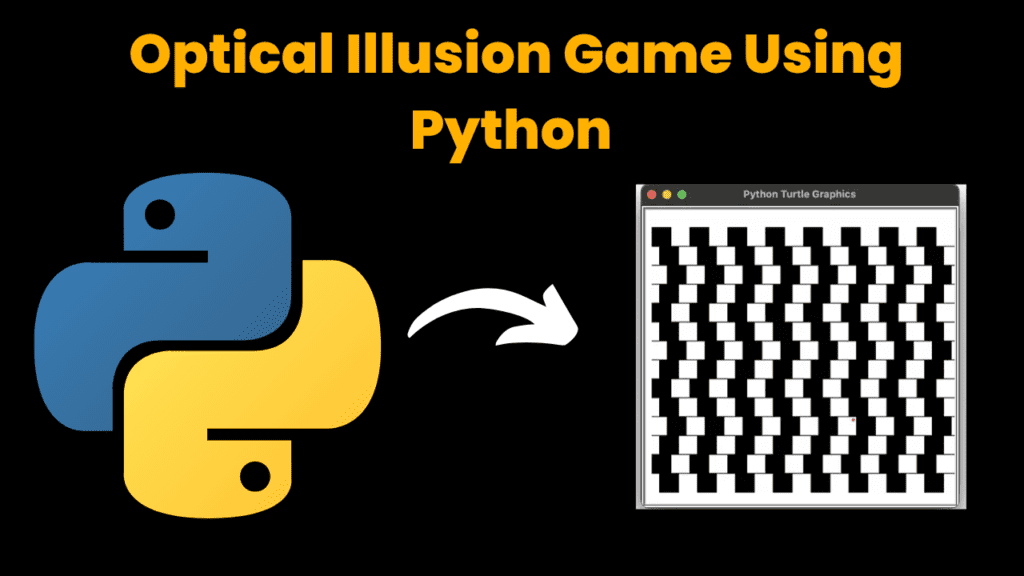
Introduction :
In this tutorial, we’ll create a simple optical illusion using Python’s turtle graphics module. The illusion will consist of rows of squares and lines that create a visually interesting pattern. This project will help you understand how to use basic drawing functions and manage screen graphics. This optical illusion project involves creating a visually engaging pattern using Python’s turtle graphics module. The illusion will feature rows of squares and lines that create an intriguing visual effect. By understanding the code, you’ll learn how to manipulate graphics and create patterns that play with perception.
Required Modules :-
To run this project, you’ll need the turtle and freegames modules. If you haven’t already, install the freegames module using pip
pip install freegames
How to Run the Code :-
1. Install Dependencies:
○ Ensure you have Python installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Save the provided code in a file named optical_illusion.py.
Run the script using Python:
python optical_illusion.py
3. View the Illusion:
○ Thegraphical window will display the optical illusion pattern created by rows of squares and lines.
Code Explanation :-
Let’s break down the key components of the code:
1. Importing Modules
from itertools import cycle
from turtle import *
from freegames import line, square
● cyclefrom itertools is used to create a repeating pattern.
● turtleis used for graphics.
● lineandsquarefrom freegames are used to draw lines and squares.
2. Setting Up the Constants
size = 25
● size: Defines the size of the squares and spacing for the lines.
3. Drawing Rows of Squares
def draw_row(x, y):
for i in range(0, 10):
offset = x + (i * size * 2)
square(offset, y, size, 'black')
● draw_row(x, y): Draws a row of squares starting from (x, y) with a black color. Each square is placed with an offset to the right.
4. Drawing Multiple Rows
def draw_rows():
offsets = [-200,-190,-180,-190]
pairs = zip(cycle(offsets), range(150,-176,-25))
for offset, y in pairs:
draw_row(offset, y)
draw_rows(): Uses cycle to create a repeating pattern for the offsets and draws multiple rows of squares with varying vertical positions
5. Drawing Horizontal Lines
def draw_lines():
x =-200
y = 150
for i in range(0, 14):
line(x, y- i * size, x + 16 * size, y- i * size)
● draw_lines(): Draws horizontal lines across the screen. The lines are spaced vertically and extend horizontally.
6. Setting Up the Graphics Window
setup(420, 400, 30, 0)
hideturtle()
tracer(False)
listen()
draw_rows()
draw_lines()
done()
● setup(): Initializes the graphics window with a width of 420 and a height of 400.
● hideturtle(): Hides the turtle cursor for a cleaner look.
● tracer(False): Disables automatic screen updates for better performance.
● listen(): Prepares the window for events (not used in this basic example but included for potential extensions).
● draw_rows() and draw_lines(): Call the functions to draw the optical illusion.
● done(): Completes the setup and starts the graphics display.
Get Discount on Top Educational Courses
Source Code :
from itertools import cycle
from turtle import *
from freegames import line, square
size = 25
def draw_row(x, y):
for i in range(0, 10):
offset = x + (i * size * 2)
square(offset, y, size, 'black')
def draw_rows():
offsets = [-200, -190, -180, -190]
pairs = zip(cycle(offsets), range(150, -176, -25))
for offset, y in pairs:
draw_row(offset, y)
def draw_lines():
x = -200
y = 150
for i in range(0, 14):
line(x, y - i * size, x + 16 * size, y - i * size)
setup(420, 400, 30, 0)
hideturtle()
tracer(False)
listen()
draw_rows()
draw_lines()
done()
Output :
When you run the code, a window will appear displaying the optical illusion created by rows of squares and lines. The pattern will create a visually interesting effect, making the graphics appear dynamic and engaging
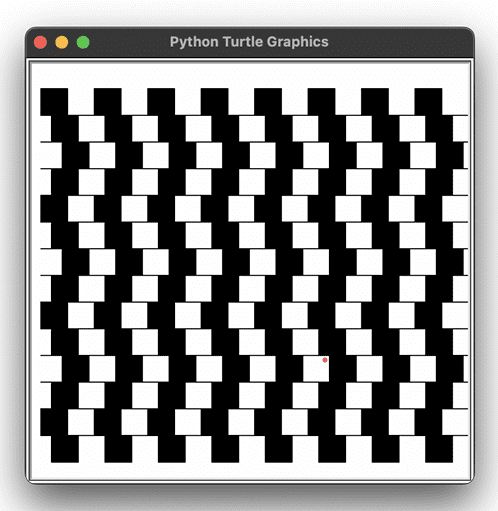
Find More Projects
ludo game in python using gui and thinkter introduction The “ludo game in python“ project is a simplified digital version of the …
hotel management system in python introduction The Hotel Management System is a simple, interactive GUI-based desktop application developed using Python’s Tkinter library. …
cryptography app in python using gui introduction In the digital age, data security and privacy are more important than ever. From messaging …
portfolio generator tool in python using GUI introduction In today’s digital-first world, having a personal portfolio is essential for students, job seekers, …
interactive story game in python using gui introduction The Interactive story game in python using gUI is a visually rich, dynamic, and engaging …
maze generator using Python with Pygame Module GUI introduction In this maze generator using Python, realm of logic, problem-solving, and computer science, …