Cannon Game Using Python With Source Code
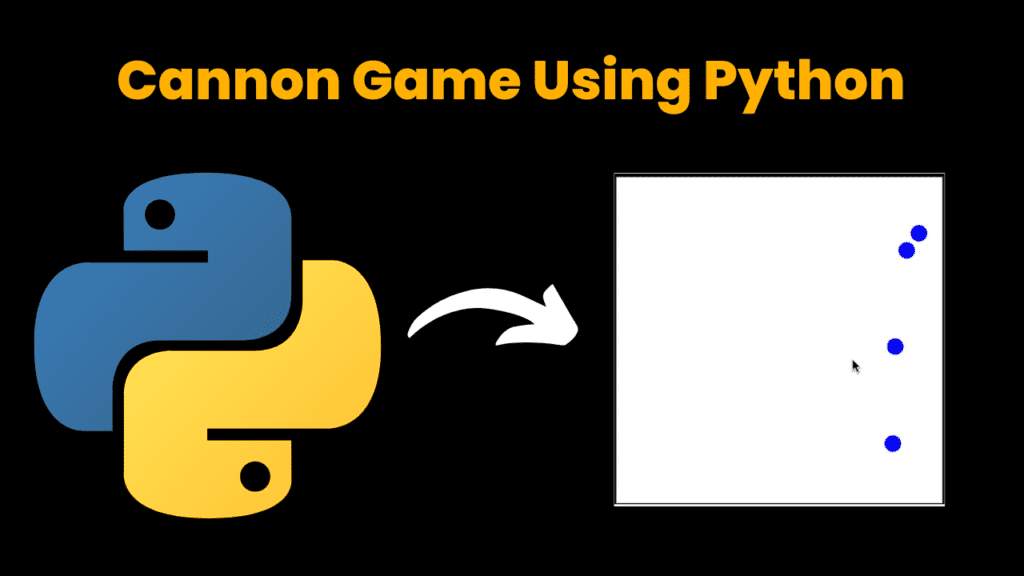
Introduction :
In this tutorial, we’ll create a simple cannon game using Python. The game will involve a cannonball that players shoot towards moving targets. We’ll use the turtle graphics module for rendering and the free games module to manage the game logic. This project will teach you how to handle screen interactions, animations, and basic game mechanics This cannon game is a fun, interactive project that demonstrates basic game developmentco ncepts using Python. In this game, a cannonball is fired when you tap the screen, and your goal is to hit moving targets. The game will keep track of the ball’s movement, handle collisions with targets, and update the game display accordingly
Required Modules :
To run this project, you’ll need the turtle and free games modules. Install the free games module using pip
pip install freegames
How to Run the Code :-
1. Install Dependencies:
○ Ensure you have Python installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Save the provided code in a file named cannon_game.py.
Run the script using Python:
python cannon_game.py
3. Use the Game :
○ Tapanywhere on the screen to fire the cannonball.
○ Thecannonball will move towards the tapped position and attempt to hit the targets.
Code Explanation :-
Let’s break down the key components of the code:
1. Importing Modules
from random import randrange
from turtle import *
from freegames import vector
Weimport the turtle module for graphics, the random module to generate random targets,
and vector from freegames to handle points in the game.
2. Initializing Game Variables
ball = vector(-200,-200)
speed = vector(0, 0)
targets = []
● ball: Theposition of the cannonball.
● speed: The speed and direction of the cannonball.
● targets: List of targets to hit.
3. Handling Screen Taps
def tap(x, y):
"Respond to screen tap."
if not inside(ball):
ball.x =-199
ball.y =-199
speed.x = (x + 200) / 25
speed.y = (y + 200) / 25
tap(x, y): Called when the screen is tapped. It sets the cannonball’s speed based on the tap position
4. Checking If a Point Is Inside the Screen
def inside(xy):
"Return True if xy within screen."
return-200 < xy.x < 200 and-200 < xy.y < 200
inside(xy): Returns True if the point is within the game screen bounds.
5. Drawing the Game Elements
def draw():
"Draw ball and targets."
clear()
for target in targets:
goto(target.x, target.y)
dot(20, 'blue')
if inside(ball):
goto(ball.x, ball.y)
dot(6, 'red')
update()
draw(): Clears the screen and redraws the ball and targets
6. Moving the Ball and Targets
def move():
"Move ball and targets."
if randrange(40) == 0:
y = randrange(-150, 150)
target = vector(200, y)
targets.append(target)
for target in targets:
target.x-= 0.5
if inside(ball):
speed.y-= 0.35
ball.move(speed)
dupe = targets.copy()
targets.clear()
for target in dupe:
if abs(target- ball) > 13:
targets.append(target)
draw()
for target in targets:
if not inside(target):
return
ontimer(move, 50)
move(): Moves the cannonball and targets, updates their positions, and handles collisions. If a target is hit, it’s removed from the game.
7. Setting Up the Game
setup(420, 420, 370, 0)
hideturtle()
up()
tracer(False)
onscreenclick(tap)
move()
done()
setup(): Initializes the game window.
● hideturtle(): Hides the turtle cursor.
● up(): Moves the turtle to the “pen up” state.
● tracer(False): Disables automatic screen updates for better performance.
● onscreenclick(tap): Registers a click event handler for the game.
● move(): Starts the game loop.
● done(): Completes the setup and starts the game.
Get Discount on Top Educational Courses
Source Code :
from random import randrange
from turtle import *
from freegames import vector
ball = vector(-200, -200)
speed = vector(0, 0)
targets = []
def tap(x, y):
"Respond to screen tap."
if not inside(ball):
ball.x = -199
ball.y = -199
speed.x = (x + 200) / 25
speed.y = (y + 200) / 25
def inside(xy):
"Return True if xy within screen."
return -200 < xy.x < 200 and -200 < xy.y < 200
def draw():
"Draw ball and targets."
clear()
goto(ball.x, ball.y)
dot(10, 'blue')
for target in targets:
goto(target.x, target.y)
dot(20, 'red')
update()
def move():
"Move ball and targets."
if inside(ball):
speed.y -= 0.35
ball.move(speed)
for target in targets:
target.x -= 0.5
if randrange(40) == 0:
y = randrange(-150, 150)
target = vector(200, y)
targets.append(target)
draw()
for target in targets:
if not inside(target):
return
ontimer(move, 50)
setup(420, 420, 370, 0)
hideturtle()
up()
tracer(False)
onscreenclick(tap)
move()
done()
Output :
When you run the code, a window will appear where you can tap to fire the cannonball. The cannonball will travel towards the tapped location, attempting to hit moving targets. The targets will appear randomly and move horizontally across the screen. The game will continue running until you close the window.
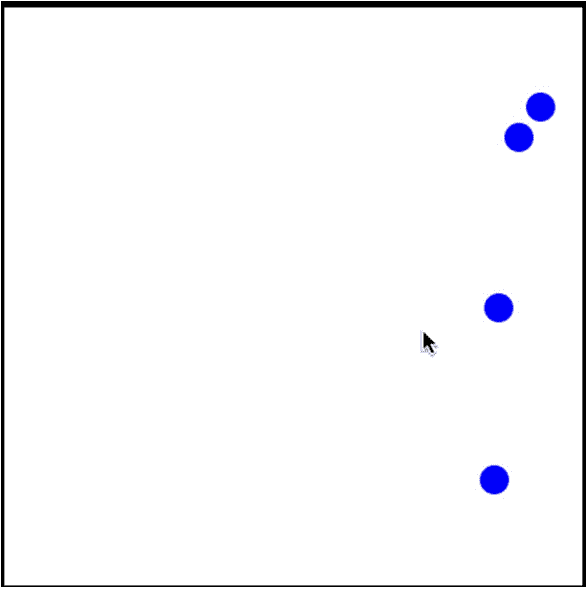
Reference
For more information on the turtle and freegames modules, you can refer to their respective documentation:
● Turtle Graphics Documentation
Find More Projects
MathGenius Pro – AI-Powered Math Solver Using Python Introduction: From simple arithmetic to more complicated college-level subjects like integration, differentiation, algebra, matrices, …
CipherMaze: The Ultimate Code Cracking Quest Game Using Python Introduction: You can make CipherMaze, a fun and brain-boosting puzzle game, with Python …
Warp Perspective Using Open CV Python Introduction: In this article, we are going to see how to Create a Warp Perspective System …
Custom AI Story Generator With Emotion Control Using Python Introduction: With the help of this AI-powered story generator, users can compose stories …
AI Powered PDF Summarizer Using Python Introduction: AI-Powered PDF Summarizer is a tool that extracts and summarizes research papers from ArXiv PDFs using Ollama (Gemma 3 LLM). The …
AI Based Career Path Recommender Using Python Introduction: One of the most significant and frequently perplexing decisions in a person’s life is …