Real-Time Chat Application Using HTML , CSS & JavaScript With Source Code
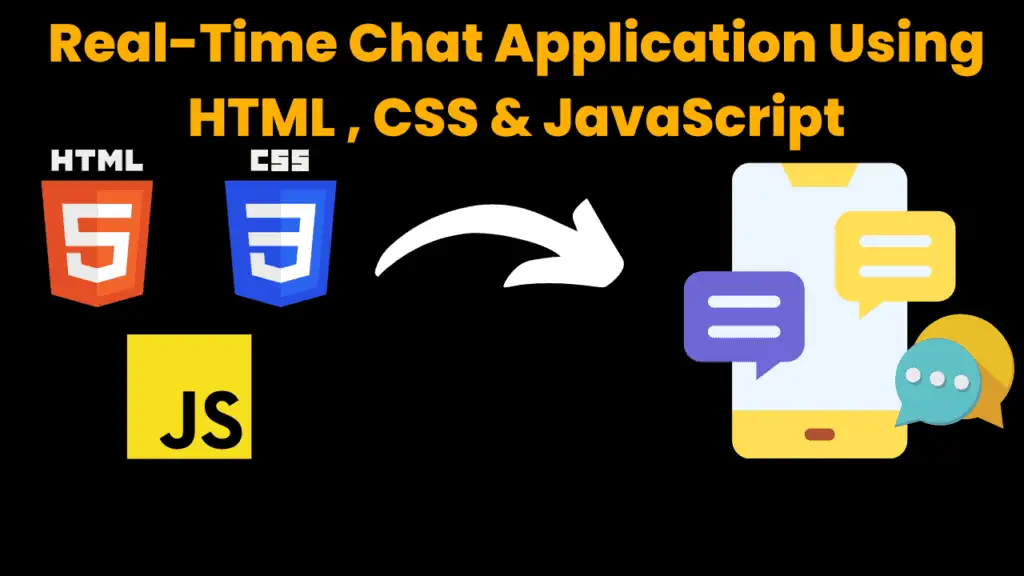
Introduction:
The Real-Time Chat Application is a web-based platform that enables users to communicate with each other in real-time using text messages. It provides a seamless and interactive chatting experience, fostering instant communication and collaboration between users. The application utilizes HTML, CSS, and JavaScript to create an attractive and user-friendly interface.
Key Features:
User Registration and Authentication: Users can create an account and securely log in to the chat application to access the chat features.
Real-Time Messaging: The application allows users to send and receive messages instantly, providing a smooth and responsive chat experience.
User-to-User Communication: Users can search for other registered users and initiate private conversations with them.
Group Chat: Users have the ability to create or join chat rooms where multiple participants can communicate simultaneously.
Emojis and File Sharing: The application supports the use of emojis in messages and allows users to share files such as images or documents.
Message Notifications: Users receive notifications for new messages, ensuring that they stay updated with the ongoing conversations.
User Presence Status: The application displays the online/offline status of users, indicating their availability for chat.
Message History: The chat application maintains a message history, allowing users to scroll back and view previous conversations.
Source Code:
HTML:
Real-Time Chat Application
Real-Time Chat Application
CSS (style.css):
/* @codewithcurious.com */
/* Global Styles */
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
}
header {
background-color: #007bff;
color: #fff;
padding: 20px;
}
.container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
/* Chat Window Styles */
.chat-window {
width: 500px;
background-color: #f0f0f0;
border-radius: 8px;
overflow: hidden;
}
.chat-area {
height: 400px;
overflow-y: scroll;
padding: 20px;
}
.chat-messages {
display: flex;
flex-direction: column;
}
.message {
margin-bottom: 10px;
background-color: #fff;
padding: 10px;
border-radius: 4px;
}
.user-input {
display: flex;
align-items: center;
padding: 20px;
background-color: #fff;
}
#message-input {
flex-grow: 1;
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
}
#send-button {
padding: 8px 16px;
margin-left: 10px;
background-color: #007bff;
border: none;
border-radius: 4px;
color: #fff;
cursor: pointer;
}
#send-button:hover {
background-color: #0056b3;
}
JavaScript (script.js):
//@codewithcurious.com
// Function to handle sending a message
function sendMessage() {
const messageInput = document.getElementById('message-input');
const message = messageInput.value.trim();
if (message !== '') {
const chatMessages = document.querySelector('.chat-messages');
const messageElement = document.createElement('div');
messageElement.classList.add('message');
messageElement.textContent = message;
chatMessages.appendChild(messageElement);
messageInput.value = '';
chatMessages.scrollTop = chatMessages.scrollHeight;
}
}
// Event listener
document.getElementById('send-button').addEventListener('click', sendMessage);
document.getElementById('message-input').addEventListener('keydown', (event) => {
if (event.key === 'Enter') {
event.preventDefault();
sendMessage();
}
});
Find Another Real-Time Chat App on Code Pen :
See the Pen Simple Chat UI by Sajad Hashemian (@sajadhsm) on CodePen.
See the Pen Chat App UI by Luke (@CodeLlama) on CodePen.
Output:
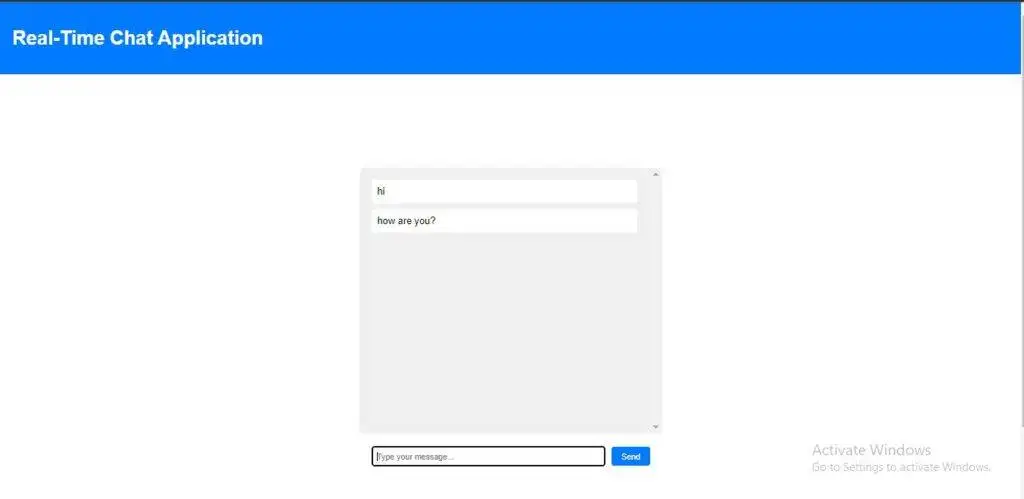
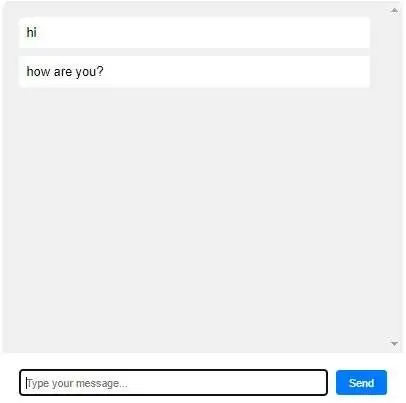
Find More Projects
Bank Management System Using Python Django Introduction The Bank Management System V2.0.2 is a feature-rich online banking solution built using the Python …
Pharmacy Management System Using Python Django Introduction The Pharmacy Dispensing Management System is a robust and user-friendly software solution developed using Python …
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …