Build a Quiz Game Using HTML CSS and JavaScript
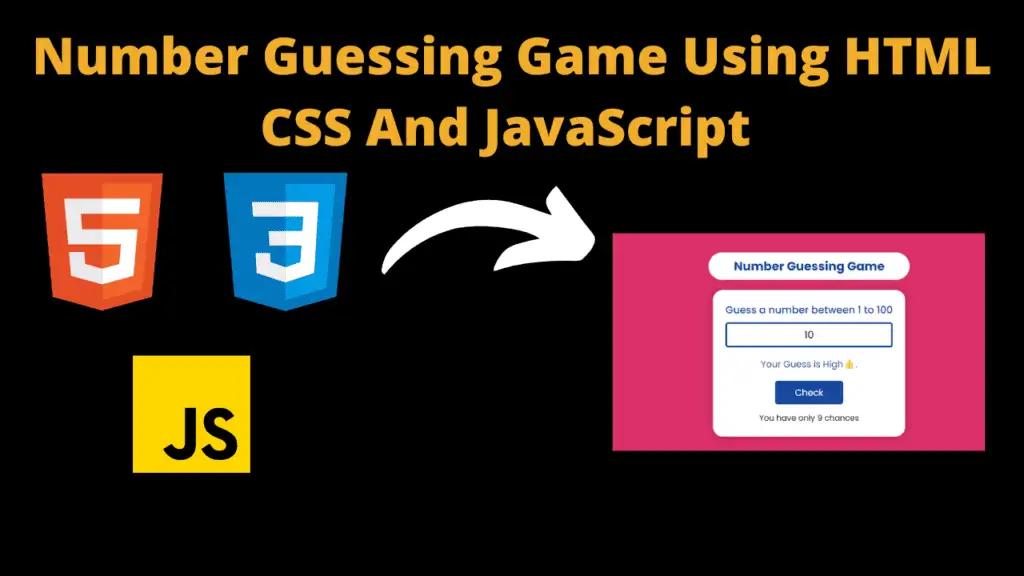
Introduction
Hello coders, you might have played various games, but were you aware that you can create your own games using HTML, CSS, and JavaScript which can be played by both you and the computer.
Today in this article, you’ll get to know that how to create a Number Guessing Game using HTML, CSS and JavaScript. As we know that by developing these type of game or project we can enhance our coding skills, as it requires using logic and problem-solving skills.
In our project we’ve build a number guessing game. In this game, the computer will randomly select a number between 1 to 100 that you will not know, and you have to guess the number based on the hint that will be provided and you’ll get only 10 chances to make the correct guess and find the answer.
HTML (index.html)
Get Discount on Top Educational Courses
This code is our HTML code. Our HTML code sets up the basic structure of our number guessing game. As we all know that HTML code is the code which sets the basic structure of any website or webpage.
- Our code starts with <! DOCTYPE html> which defines the type of our document and declare that our code is a HTML code document.
- <html lang=”en”> starts the HTML document with the language set to English.
- <title>: Sets the title of the webpage as Talha – Number Guessing Game.
- <link>: These tags links external CSS file and Favicon to our webpage.
- <body>: This section contains the content of our Number guessing game.
- <h1>: It contains main heading of the page as Number Guessing Game.
- <div class=”container”>: This contains main content of the game.
- <h2> : A secondary heading which tells user to guess the number as prompt.
- <input>: This is the area where user enter the number.
- <p class=”guess”></p>: This is for the the result or feedback of the user’s input number.
- <button class=”checkBtn”>: This button is to submit the guessed number by user.
- <p class=”chances”>: This displays that how many chance are left for user, starting at 10.
- <script src=”index.js”>: This links the external JavaScript file that will control the functionality of the game.
<title>CodeWithCurious.com - Number Guessing Game</title>
<h1>Number Guessing Game</h1>
<div class="container">
<h2 class="heading">Guess a number between 1 to 100</h2>
<p class="guess"></p>
<button class="checkBtn">Check</button>
<p class="chances">You have only <span class="chance">10</span> chances</p>
</div>
CSS (Style.css)
This is our CSS code which styles our number guessing game and provides our game an authentic look. CSS adds interactivity to any webpage as it provide styling to the webpages.
- Our code starts by importing external font “Poppins” from the google fonts with various font weights.
- Universal selector resets the margin, padding of the webpage and sets box-sizing as border box and applies the Poppins font for all elements.
- For the body tag, Centers all content horizontally and vertically using flexbox. Min height sets as 100vh, a light pink background and content direction sets as column using flex-direction.
- H1 takes the font size of 24px with some margin and padding as well. Color, background color and border-radius is also given to the main heading of the page.
- Container centers its content using text align property. It takes a padding of 25px with a border-radius of 20px. Also it applies a shadow to give a 3d look.
- h2, guess and chances are styled by providing some basic styling to them. They contains some font and color properties.
- Input field takes full width and centers user input. It also sets the font size with a border and border-radius. It Removes the spinner buttons for number input across different browsers (Chrome, Safari, Firefox, etc.).
- Button (.checkBtn) styled by giving blue background, white text, medium font-size of 18px and border-radius. It adds padding of 10px 40px. It Change cursor to pointer on hover.
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@100;200;300;400;500;600;700;800;900&display=swap");
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
min-height: 100vh;
background: #db3069;
}
h1 {
font-size: 24px;
margin-bottom: 20px;
padding: 10px 50px;
color: #1446a0;
background-color: white;
border-radius: 50px;
}
.container {
text-align: center;
padding: 25px;
background: white;
border-radius: 20px;
box-shadow: 0 0 20px rgba(0, 0, 0, 0.2);
}
.container h2 {
font-size: 20px;
color: #1446a0;
font-weight: 500;
}
.container .guess {
color: #1446a0;
font-size: 18px;
margin: 10px 0;
}
.container .chances {
color: black;
font-size: 16px;
margin-top: 5px;
}
.container input {
font-size: 20px;
padding: 0 20px;
text-align: center;
width: 100%;
height: 50px;
margin: 10px 0;
border: 3px solid #1446a0;
border-radius: 5px;
}
input:disabled {
cursor: not-allowed;
}
/* To remove the controls in the input field */
/* Chrome, Safari, Edge, Opera */
input::-webkit-outer-spin-button,
input::-webkit-inner-spin-button {
-webkit-appearance: none;
margin: 0;
}
/* Firefox */
input[type="number"] {
-moz-appearance: textfield;
}
.checkBtn {
font-size: 18px;
margin: 10px 0;
padding: 10px 40px;
color: white;
background: #1446a0;
border: none;
border-radius: 5px;
cursor: pointer;
}
.checkBtn:active {
transform: scale(0.95);
}
Javascript (Script.js)
This is our JavaScript code which adds functionality to our number guessing game and also this is the main part of our game. Without using this code our game will not function properly so that we need JavaScript. It adds logic to our code using which we provide functionality to our number guessing game.
- First it selects the elements from the HTML code and stores them in variables.
- Then using Math.random it generates random number between 1 to 100 and store in a variable.
- Sets the total chances as 10 at initially.
- Adds a event listener on click of Button.
- First it decrease the total chance by 1 after every button click.
- When chances reaches the 0, it disables the input field and updates the message. It changes the button text to “Play again”.
- If user guess the number correctly, It disables input, displays a win message and changes the button to “Play Again”.
- If the guess is high or low, it displays a message that either user’s guess is low or high and updates remaining chances.
- If user enters an invalid number which is out of range or keeps the input filed empty then it’ll show an error message.
- At last it resets the game when play again is clicked.
const inputEl = document.querySelector("input");
const guessEl = document.querySelector(".guess");
const checkBtnEl = document.querySelector("button");
const remainingChancesTextEl = document.querySelector(".chances");
const remainingChancesEl = document.querySelector(".chance");
let randomNumber = Math.floor(Math.random() * 100);
totalChances = 10;
checkBtnEl.addEventListener("click", () => {
totalChances--;
let inputValue = inputEl.value;
if (totalChances === 0) {
inputValue = "";
inputEl.disabled = true;
guessEl.textContent = "Oops...! Bad luck, You lost the game."
guessEl.style.color = "red";
checkBtnEl.textContent = "Play Again...";
remainingChancesTextEl.textContent = "No chances left"
}
else if (totalChances randomNumber && inputValue < 100) {
guessEl.textContent = "Your Guess is High.";
remainingChancesEl.textContent = totalChances;
guessEl.style.color = "#1446a0";
} else if (inputValue 0) {
guessEl.textContent = "Your Guess is low.";
remainingChancesEl.textContent = totalChances;
guessEl.style.color = "#1446a0";
} else {
guessEl.textContent = "Your number is invalid.";
remainingChancesEl.textContent = totalChances;
guessEl.style.color = "red";
}
});
Output:
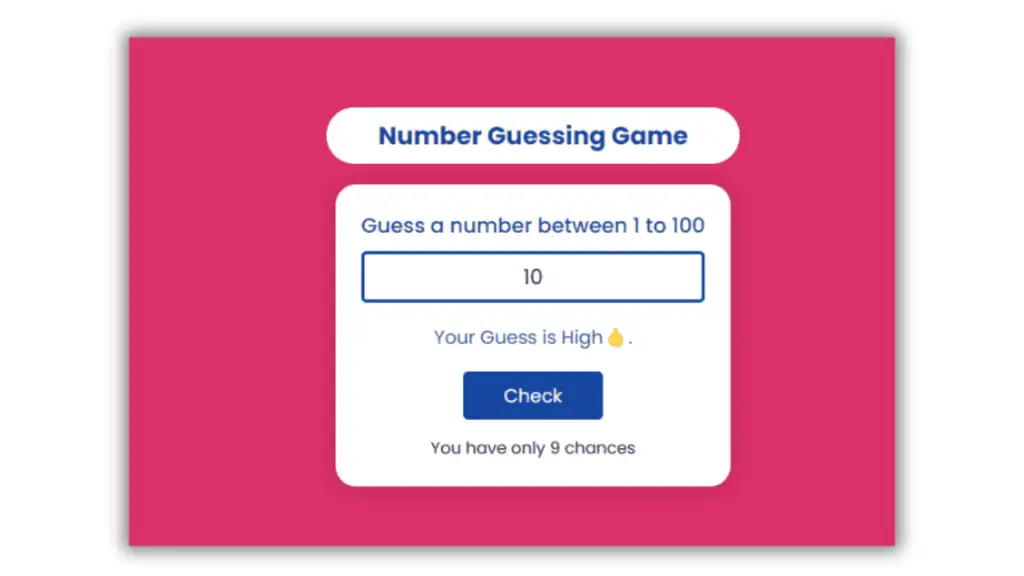
Disclaimer: The code provided in this post is sourced from GitHub and is distributed under the MIT License. All credits for the original code go to the respective owner. We have shared this code for educational purposes only. Please refer to the original repository for detailed information and any additional usage rights or restrictions.
Drawing Chhatrapati Shivaji Maharaj Using Python Chhatrapati Shivaji Maharaj, the legendary Maratha warrior and founder of the Maratha Empire, is an inspiration …
Resume Builder Application using Java With Source Code Graphical User Interface [GUI] Introduction: The Resume Builder Application is a powerful and user-friendly …
Encryption Tool using java with complete source Code GUI Introduction: The Encryption Tool is a Java-based GUI application designed to help users …
Movie Ticket Booking System using Java With Source Code Graphical User Interface [GUI] Introduction: The Movie Ticket Booking System is a Java …