A Simple Maze Drawing Game in Python With Source Code

Introduction :
In this game, the maze is generated randomly, and you interact with it by clicking on the screen. The maze is drawn with lines that form a grid, and you can see how the maze is structured. This project is a great way to practice Python programming and understand basic graphics handling Maze is an intriguing game where players navigate through a maze, starting from one side and aiming to reach the other. In this Python project, we’ll create a simple maze generator using the turtle graphics module. This maze game will allow you to draw a maze and interact with it by clicking on the screen.
In this blog post, we’ll walk through the process of creating the Maze game and explain how the code works
Required Modules :
To run this project, you need the turtle and freegames modules. If you don’t have freegames installed, you can install it using:
pip install freegames
How to Run the Code :
1. Install Dependencies:
○ Ensure Python is installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Savetheprovided code in a file named maze_game.py.
Run the script using Python
python maze_game.py
3. Interact with the Game:
○ Agraphical window will open displaying the maze.
○ Click anywhere within the window to draw a red dot on the maze.
Code Explanation :-
Let’s break down the code to understand how the Maze game is implemented:
1. Importing Modules
from random import random
from turtle import *
from freegames import line
● random.random: Used to generate random values for maze generation.
● turtle: Used for graphical output and user interaction.
● freegames.line: Provides a utility function to draw lines.
2. Drawing the Maze
def draw():
"""Draw maze."""
color('black')
width(5)
for x in range(-200, 200, 40):
for y in range(-200, 200, 40):
if random() > 0.5:
line(x, y, x + 40, y + 40)
else:
line(x, y + 40, x + 40, y)
update()
draw(): Generates the maze by drawing lines in a grid pattern. The maze is created
with a series of random lines that either go diagonally or in the opposite direction. The
random() function determines whether the line will go from (x, y) to (x + 40, y +
40) or (x, y + 40)to(x + 40, y).
3. Handling Clicks
def tap(x, y):
"""Draw line and dot for screen tap."""
if abs(x) > 198 or abs(y) > 198:
up()
else:
down()
width(2)
color('red')
goto(x, y)
dot(4)
tap(x, y):Responds to mouse clicks. It draws a small red dot where the user clicks.
The function also checks if the click is within the bounds of the window; if it is, it will draw
a dot. If not, it will move the turtle to the click position without drawing.
4. Setting Up the Game
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
draw()
onscreenclick(tap)
done()
setup(): Initializes the graphical window with a width and height of 420.
● hideturtle(): Hides the turtle cursor for a cleaner look.
● tracer(False): Disables automatic screen updates for better performance.
● draw(): Draws the initial maze.
● onscreenclick(tap): Sets up the tap() function to handle mouse clicks.
● done(): Completes the setup and starts the game loop
Source Code :
from random import random
from turtle import *
from freegames import line
def draw():
"""Draw maze."""
color('black')
width(5)
for x in range(-200, 200, 40):
for y in range(-200, 200, 40):
if random() > 0.5:
line(x, y, x + 40, y + 40)
else:
line(x, y + 40, x + 40, y)
update()
def tap(x, y):
"""Draw line and dot for screen tap."""
if abs(x) > 198 or abs(y) > 198:
up()
else:
down()
width(2)
color('red')
goto(x, y)
dot(4)
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
draw()
onscreenclick(tap)
done()
Output :
Running the code will open a graphical window displaying a randomly generated maze. You can
interact with the maze by clicking on the screen to draw red dots
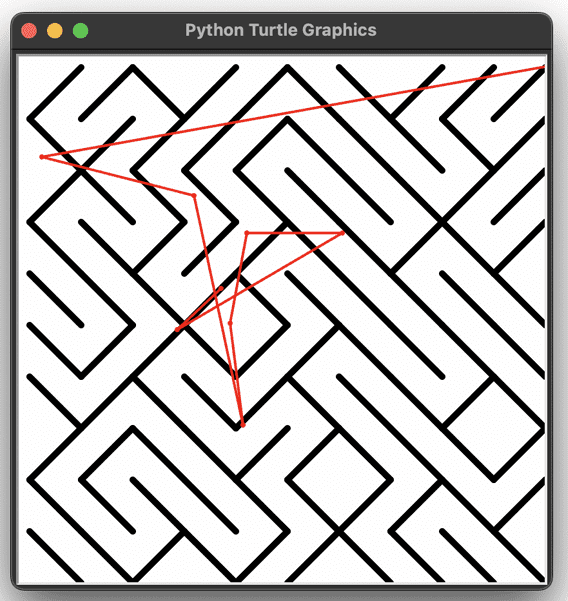
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …