Pacman Game using Python With Source Code
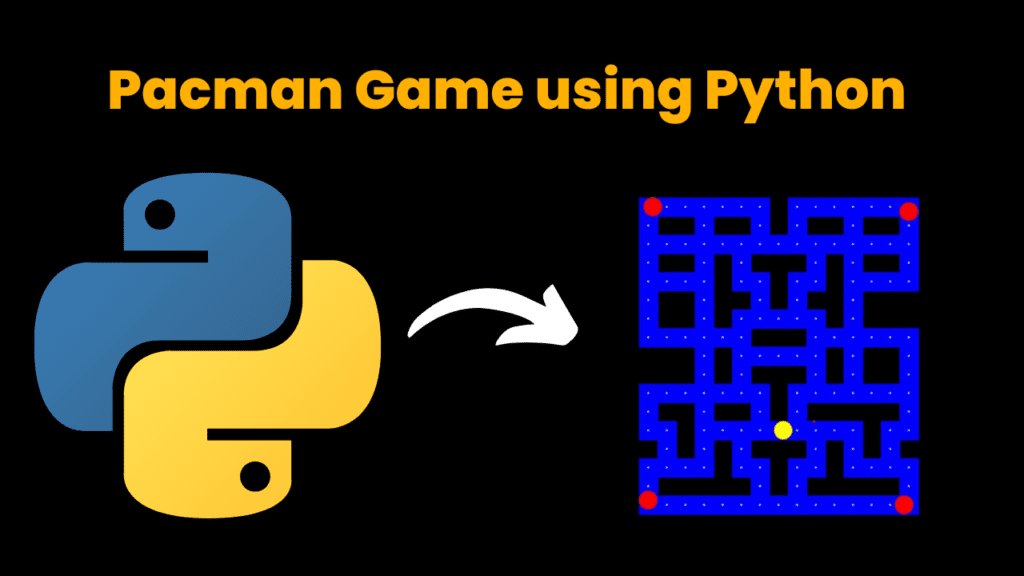
Introduction :
In this tutorial, we’ll create a classic Pacman game using Python. This project will use the turtle graphics module for drawing and the free games module for handling game logic. Our Pacman game will include movement, collision detection, and scorekeeping. This Pacman game is a simplified version of the classic arcade game. In this version, Pacman moves around a grid, eating pellets while avoiding ghosts. The game keeps track of the score and allows for basic movement in four directions. This project is a great exercise in understanding game development concepts using Python
Required Modules :
To run this project, you’ll need the turtle and freegames modules. Install the freegames module using pip
pip install freegames
How to Run the Code :
1. Install Dependencies:
○ Ensure you have Python installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Save the provided code in a file named pacman_game.py.
Run the script using Python
python pacman_game.py
Use the Game:
○ Usethearrow keys to move Pacman:
■ RightArrow: Move right
■ LeftArrow: Move left
■ UpArrow:Moveup
■ DownArrow:Movedown
○ Pacmaneats pellets, and the score is updated accordingly.
Code Explanation :-
Let’s walk through the key parts of the code:
1. Importing Modules
from random import choice
from turtle import *
from freegames import floor, vector
Weimport the turtle module for graphics, the random module for random ghost movement, and vector for handling points in the game.
2. Game Setup
state = {'score': 0}
path = Turtle(visible=False)
writer = Turtle(visible=False)
aim = vector(5, 0)
pacman = vector(-40,-80)
ghosts = [
[vector(-180, 160), vector(5, 0)],
[vector(-180,-160), vector(0, 5)],
[vector(100, 160), vector(0,-5)],
[vector(100,-160), vector(-5, 0)],
]
state: Dictionary to keep track of the score.
● pathandwriter: Turtle objects used for drawing and score display.
● aim: Vector representing Pacman’s current direction.
● pacman: Vector for Pacman’s position.
● ghosts: List of ghost positions and movement vectors
3. Drawing Functions :
● square(x, y): Draws a square at the specified position to represent walls and pellets
def square(x, y):
"Draw square using path at (x, y)."
path.up()
path.goto(x, y)
path.down()
path.begin_fill()
for count in range(4):
path.forward(20)
path.left(90)
path.end_fill()
offset(point): Converts a point’s coordinates to a tile index in the grid.
def offset(point):
"Return offset of point in tiles."
x = (floor(point.x, 20) + 200) / 20
y = (180- floor(point.y, 20)) / 20
index = int(x + y * 20)
return index
valid(point): Checks if a point is valid for movement (not a wall).
def valid(point):
"Return True if point is valid in tiles."
index = offset(point)
if tiles[index] == 0: return False
index = offset(point + 19)
if tiles[index] == 0:
return False
return point.x % 20 == 0 or point.y % 20 == 0
world(): Draws the game world including walls and pellets
def world():
"Draw world using path."
bgcolor('black')
path.color('blue')
for index in range(len(tiles)):
tile = tiles[index]
if tile > 0:
x = (index % 20) * 20- 200
y = 180- (index // 20) * 20
square(x, y)
if tile == 1:
path.up()
path.goto(x + 10, y + 10)
path.dot(2, 'white')
4. Game Logic
● move(): Moves Pacman and ghosts, updates the game state
def move():
"Move pacman and all ghosts."
writer.undo()
writer.write(state['score'])
clear()
if valid(pacman + aim):
pacman.move(aim)
index = offset(pacman)
if tiles[index] == 1:
tiles[index] = 2
state['score'] += 1
x = (index % 20) * 20- 200
y = 180- (index // 20) * 20
square(x, y)
up()
goto(pacman.x + 10, pacman.y + 10)
dot(20, 'yellow')
for point, course in ghosts:
if valid(point + course):
point.move(course)
else:
options = [vector(5, 0), vector(-5, 0), vector(0, 5),
vector(0,-5)]
plan = choice(options)
course.x = plan.x
course.y = plan.y
up()
goto(point.x + 10, point.y + 10)
dot(20, 'red')
update()
for point, course in ghosts:
if abs(pacman- point) < 20:
return
ontimer(move, 100)
change(x, y): Changes Pacman’s direction if valideo.
def change(x, y):
"Change pacman aim if valid."
if valid(pacman + vector(x, y)):
aim.x = x
aim.y = y
5. Initializing the Game
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
writer.goto(160, 160)
writer.color('white')
writer.write(state['score'])
listen()
onkey(lambda: change(5, 0), 'Right')
onkey(lambda: change(-5, 0), 'Left')
onkey(lambda: change(0, 5), 'Up')
onkey(lambda: change(0,-5), 'Down')
world()
move()
done()
setup(): Initializes the window.
● hideturtle(): Hides the turtle cursor.
● tracer(False): Disables automatic screen updates for better performance.
● writer.write(): Displays the score.
● onkey(): Registers key bindings for movement.
● world()andmove(): Draw the game world and start the game loop
Source Code :
from random import choice
from turtle import *
from freegames import floor, vector
state = {'score': 0}
path = Turtle(visible=False)
writer = Turtle(visible=False)
aim = vector(5, 0)
pacman = vector(-40, -80)
ghosts = [
[vector(-180, 160), vector(5, 0)],
[vector(-180, -160), vector(0, 5)],
[vector(100, 160), vector(0, -5)],
[vector(100, -160), vector(-5, 0)],
]
tiles = [
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1,
1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1,
0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 1,
0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 1,
1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0,
0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 0, 0, 0,
0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1,
1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0,
0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1,
1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0,
]
def square(x, y):
"Draw square using path at (x, y)."
path.up()
path.goto(x, y)
path.down()
path.begin_fill()
for count in range(4):
path.forward(20)
path.left(90)
path.end_fill()
def offset(point):
"Return offset of point in tiles."
x = (floor(point.x, 20) + 200) / 20
y = (180 - floor(point.y, 20)) / 20
index = int(x + y * 20)
return index
def valid(point):
"Return True if point is valid in tiles."
index = offset(point)
if tiles[index] == 0:
return False
index = offset(point + 19)
if tiles[index] == 0:
return False
return point.x % 20 == 0 or point.y % 20 == 0
def world():
"Draw world using path."
bgcolor('black')
path.color('blue')
for index in range(len(tiles)):
tile = tiles[index]
if tile > 0:
x = (index % 20) * 20 - 200
y = 180 - (index // 20) * 20
square(x, y)
if tile == 1:
path.up()
path.goto(x + 10, y + 10)
path.dot(2, 'white')
def move():
"Move pacman and all ghosts."
writer.undo()
writer.write(state['score'])
clear()
if valid(pacman + aim):
pacman.move(aim)
index = offset(pacman)
if tiles[index] == 1:
tiles[index] = 2
state['score'] += 1
x = (index % 20) * 20 - 200
y = 180 - (index // 20) * 20
square(x, y)
up()
goto(pacman.x + 10, pacman.y + 10)
dot(20, 'yellow')
for point, course in ghosts:
if valid(point + course):
point.move(course)
else:
options = [vector(5, 0), vector(-5, 0), vector(0, 5), vector(0, -5)]
plan = choice(options)
course.x = plan.x
course.y = plan.y
up()
goto(point.x + 10, point.y + 10)
dot(20, 'red')
update()
for point, course in ghosts:
if abs(pacman - point) < 20:
return
ontimer(move, 100)
def change(x, y):
"Change pacman aim if valid."
if valid(pacman + vector(x, y)):
aim.x = x
aim.y = y
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
writer.goto(160, 160)
writer.color('white')
writer.write(state['score'])
listen()
onkey(lambda: change(5, 0), 'Right')
onkey(lambda: change(-5, 0), 'Left')
onkey(lambda: change(0, 5), 'Up')
onkey(lambda: change(0, -5), 'Down')
world()
move()
done()
Output :
When you run the code, you will see a window displaying the Pacman game. Pacman will navigate a grid, consuming pellets and increasing the score. Ghosts will move randomly, adding a challenge to the game
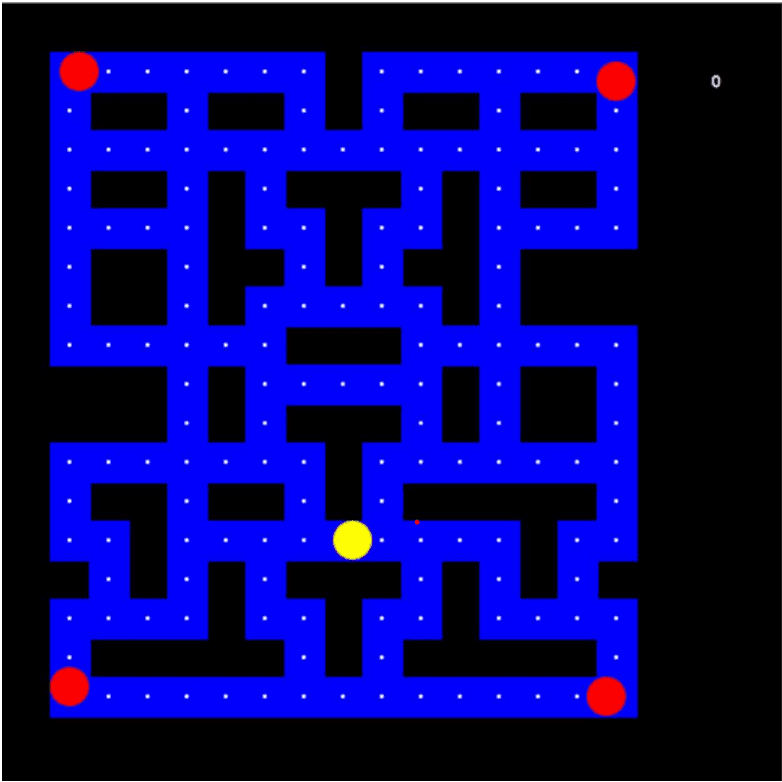
Reference
For more detailed information on the turtle and freegames modules, you can refer to their respective documentation:
● Turtle Graphics Documentation
Find More Projects
Custom Music Player with Playlist Using JavaScript Introduction Hello friends , welcome to another new blog. Hope you all are doing good. …
Country Guide App using HTML, CSS & JavaScript Introduction Hello friends, welcome to our new blog post. Today we have created a …
Personal Finance Manager using java swing with complete source code Introduction: The Personal Finance Manager is a Java-based application designed to help …
Gym Website Using HTML, CSS, and JavaScript Introduction Hello friends, all of you are welcome to today’s beautiful project. Today we have …
Jarvis Chatbot Using HTML Jarvis Chatbot Using HTML Jarvis Chatbot Using HTML CSS & javaScript Introduction Hello friends, hope you all are …
movie Website using HTML, CSS, and JavaScript Introduction Hello friends my name is Gautam and you all are welcome to today’s beautiful …