KBC Game using Python with Source Code
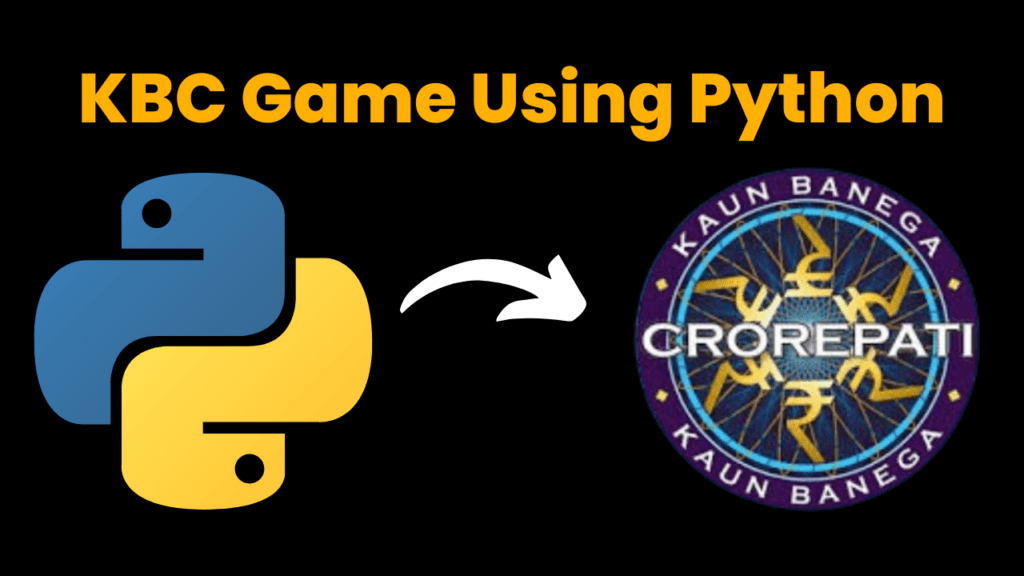
Introduction :
Welcome to this blog post on building a “Kaun Banega Crorepati” (KBC) game using Python! This KBC game is developed with the Tkinter library, a standard GUI toolkit in Python, to create an interactive and engaging experience that mimics the popular TV quiz show. The game offers a series of multiple-choice questions that players must answer correctly to win increasing amounts of money.
In this game, players answer questions to win money, with each correct answer increasing their prize amount. The game includes lifelines, such as the ability to eliminate two incorrect options, adding an extra layer of strategy and excitement. Additionally, players can choose to quit the game at any point and keep the money they have won.
This Python-based KBC game is an excellent way to practice your programming skills, learn more about the Tkinter library, and enjoy a fun quiz game with friends and family!
Required Packages or Modules :
To create the KBC game, we need the following Python modules and packages:
Tkinter: This is a built-in Python module used for creating the graphical user interface (GUI). It provides various widgets like buttons, labels, and message boxes, which are essential for building the game interface.
- Installation (if not pre-installed):
pip install tk
messagebox: A submodule of Tkinter, messagebox
provides functions to create various types of message boxes (such as informational, warning, or error dialogs). It is included with Tkinter, so no separate installation is required.
questions: This is a custom Python module that should contain the list of questions for the game. Ensure that this module is present in the same directory as your main script.
How to Run the Code
To run the KBC game on your computer, follow these steps:
Download the Code
Copy the provided Python code for the KBC game into a file named kbc_game.py
. Ensure you also have a file named questions.py
containing the quiz questions.
Set Up the questions.py Module:
Create a Python file named questions.py
with a list of dictionaries, where each dictionary represents a question. An example format for the questions.py
file is:
QUESTIONS = [
{
"name": "What is the capital of France?",
"option1": "Berlin",
"option2": "Madrid",
"option3": "Paris",
"option4": "Lisbon",
"answer": 3,
"money": 1000
},
# Add more questions in the same format
]
Run the Code:
Command Prompt:
- Open the command prompt and navigate to the directory where
kbc_game.py
is located using thecd
command. - Run the code by typing:
python kbc_game.py
Code Editor:
- Open the file in your preferred code editor (such as Visual Studio Code or PyCharm) and click the “Run” or “Play” button to execute
kbc_game.py
.
- Open the file in your preferred code editor (such as Visual Studio Code or PyCharm) and click the “Run” or “Play” button to execute
Code Explanation:
The KBC game is structured with several key components, each playing an essential role in the game’s functionality. Let’s break down the main parts:
1. Initialising the Game Class:
class KBCGame:
def __init__(self, root):
self.root = root
self.root.title("Kaun Banega Crorepati")
self.current_question = 0
self.money = 0
self.lifeline_used = False
self.setup_gui()
self.load_question()
The KBCGame
class initializes the game by setting up the main window, game variables like the current question, money won, and lifeline usage status. It also calls functions to set up the GUI and load the first question.
2. Setting Up the GUI:
def setup_gui(self):
self.question_label = tk.Label(self.root, text="", font=("Arial", 16), wraplength=600, justify="center")
self.question_label.pack(pady=20)
self.option_buttons = []
for i in range(4):
btn = tk.Button(self.root, text="", font=("Arial", 14), width=20, command=lambda i=i: self.check_answer(i + 1))
btn.pack(pady=5)
self.option_buttons.append(btn)
self.lifeline_button = tk.Button(self.root, text="Lifeline", font=("Arial", 14), command=self.use_lifeline)
self.lifeline_button.pack(side="left", padx=20)
self.quit_button = tk.Button(self.root, text="Quit", font=("Arial", 14), command=self.quit_game)
self.quit_button.pack(side="right", padx=20)
self.money_label = tk.Label(self.root, text="Money: 0", font=("Arial", 14))
self.money_label.pack(pady=20)
This function sets up the graphical interface for the game, including the question label, option buttons, lifeline button, quit button, and a money label to display the current amount won.
3. Loading the Questions:
def load_question(self):
if self.current_question >= len(QUESTIONS):
self.game_over("Congrats!! You answered all the questions correctly!!\nTotal Money Won: {}".format(self.money))
return
question = QUESTIONS[self.current_question]
self.question_label.config(text=f"Question {self.current_question + 1}: {question['name']}")
self.money_label.config(text=f"Money: {self.money}")
for i in range(4):
self.option_buttons[i].config(text=f"Option {i + 1}: {question['option' + str(i + 1)]}", state="normal")
This function loads the current question from the QUESTIONS
module and updates the GUI elements accordingly. If all questions are answered, it ends the game.
4. Checking the Answer:
def check_answer(self, selected_option):
question = QUESTIONS[self.current_question]
correct_answer = question["answer"]
if selected_option == correct_answer:
messagebox.showinfo("Correct!", "You answered correctly!")
self.money += question["money"]
self.current_question += 1
self.load_question()
else:
self.game_over(f"Incorrect! The correct answer was Option {correct_answer}.\nMoney Won: {self.money}")
This function checks whether the selected option matches the correct answer. If correct, it updates the money won and loads the next question. If incorrect, it ends the game.
5. Using a Lifeline:
def use_lifeline(self):
if self.lifeline_used:
messagebox.showwarning("Lifeline Used", "You have already used the lifeline!")
return
self.lifeline_used = True
question = QUESTIONS[self.current_question]
correct_answer = question["answer"]
incorrect_options = [1, 2, 3, 4]
incorrect_options.remove(correct_answer)
hide_options = incorrect_options[:2]
for i in hide_options:
self.option_buttons[i - 1].config(text="", state="disabled")
This function allows the player to use a lifeline to eliminate two incorrect options. The lifeline can only be used once.
6. Quitting the Game:
def quit_game(self):
self.game_over(f"You chose to quit!\nTotal Money Won: {self.money}")
7. Ending the Game:
def game_over(self, message):
messagebox.showinfo("Game Over", message)
self.root.destroy()
These functions handle quitting and ending the game, displaying a message box with the final amount won.
Source Code :
Kbc.py
import tkinter as tk
from tkinter import messagebox
from questions import QUESTIONS
class KBCGame:
def __init__(self, root):
self.root = root
self.root.title("Kaun Banega Crorepati")
self.current_question = 0
self.money = 0
self.lifeline_used = False
self.setup_gui()
self.load_question()
def setup_gui(self):
# Question Label
self.question_label = tk.Label(self.root, text="", font=("Arial", 16), wraplength=600, justify="center")
self.question_label.pack(pady=20)
# Option Buttons
self.option_buttons = []
for i in range(4):
btn = tk.Button(self.root, text="", font=("Arial", 14), width=20, command=lambda i=i: self.check_answer(i + 1))
btn.pack(pady=5)
self.option_buttons.append(btn)
# Lifeline and Quit Buttons
self.lifeline_button = tk.Button(self.root, text="Lifeline", font=("Arial", 14), command=self.use_lifeline)
self.lifeline_button.pack(side="left", padx=20)
self.quit_button = tk.Button(self.root, text="Quit", font=("Arial", 14), command=self.quit_game)
self.quit_button.pack(side="right", padx=20)
# Money Label
self.money_label = tk.Label(self.root, text="Money: 0", font=("Arial", 14))
self.money_label.pack(pady=20)
def load_question(self):
if self.current_question >= len(QUESTIONS):
self.game_over("Congrats!! You answered all the questions correctly!!\nTotal Money Won: {}".format(self.money))
return
question = QUESTIONS[self.current_question]
self.question_label.config(text=f"Question {self.current_question + 1}: {question['name']}")
self.money_label.config(text=f"Money: {self.money}")
for i in range(4):
self.option_buttons[i].config(text=f"Option {i + 1}: {question['option' + str(i + 1)]}", state="normal")
def check_answer(self, selected_option):
question = QUESTIONS[self.current_question]
correct_answer = question["answer"]
if selected_option == correct_answer:
messagebox.showinfo("Correct!", "You answered correctly!")
self.money += question["money"]
self.current_question += 1
self.load_question()
else:
self.game_over(f"Incorrect! The correct answer was Option {correct_answer}.\nMoney Won: {self.money}")
def use_lifeline(self):
if self.lifeline_used:
messagebox.showwarning("Lifeline Used", "You have already used the lifeline!")
return
self.lifeline_used = True
question = QUESTIONS[self.current_question]
correct_answer = question["answer"]
incorrect_options = [1, 2, 3, 4]
incorrect_options.remove(correct_answer)
# Hide two incorrect options
hide_options = incorrect_options[:2]
for i in hide_options:
self.option_buttons[i - 1].config(text="", state="disabled")
def quit_game(self):
self.game_over(f"You chose to quit!\nTotal Money Won: {self.money}")
def game_over(self, message):
messagebox.showinfo("Game Over", message)
self.root.destroy()
if __name__ == "__main__":
root = tk.Tk()
game = KBCGame(root)
root.mainloop()
Questions.py
QUESTIONS = [
{
"name": " India's largest city by population",
"option1": "Delhi",
"option2": "Mumbai",
"option3": "Pune",
"option4": "Bangalore",
"answer": 2,
"money": 1000
},
{
"name": "India is a federal union comprising twenty-nine states and how many union territories?",
"option1": "6",
"option2": "7",
"option3": "8",
"option4": "9",
"answer": 2,
"money": 2000
},
{
"name": "What are the major languages spoken in Andhra Pradesh?",
"option1": " English and Telugu",
"option2": "Telugu and Urdu",
"option3": "Telugu and Kannada",
"option4": "All of the above languages",
"answer": 2,
"money": 3000
},
{
"name": "What is the state flower of Haryana?",
"option1": "Lotus",
"option2": "Rhododendron",
"option3": "Golden Shower",
"option4": "Not declared",
"answer": 2,
"money": 5000
},
{
"name": " Where was the International Conference on 'Yoga for Diabetes' organized from January 4-6, 2017?",
"option1": "New Delhi ",
"option2": "Jharkhand",
"option3": "Jammu and Kashmir",
"option4": "Haryana",
"answer": 1,
"money": 10000
},
{
"name": "Name the tower which was lighted up in Tricolour of India on Republic Day, 2017?",
"option1": "WTC Towers",
"option2": "Jeddah Tower",
"option3": "Burj Khalifa",
"option4": "Burj Al Arab",
"answer": 3,
"money": 20000
},
{
"name": "Which of the following states is not located in the North?",
"option1": "Himachal Pradesh",
"option2": "Jharkhand",
"option3": "Jammu and Kashmir",
"option4": "Haryana",
"answer": 2,
"money": 40000
},
{
"name": "In what state is the Elephant Falls located?",
"option1": "Meghalaya",
"option2": "Mizoram",
"option3": "Orissa",
"option4": "Manipur",
"answer": 2,
"money": 80000
},
{
"name": "Which state has the largest population?",
"option1": "Maharastra",
"option2": "Uttar Pradesh",
"option3": "Bihar",
"option4": "Andra Pradesh",
"answer": 2,
"money": 160000
},
{
"name": " Which state has the largest area?",
"option1": "Maharashtra",
"option2": "Rajasthan",
"option3": "Bihar",
"option4": "Andra Pradesh",
"answer": 2,
"money": 320000
},
{
"name": "Which is the largest coffee producing state of India?",
"option1": "Maharastra",
"option2": "Karnataka",
"option3": "Maharashtra",
"option4": "Rajasthan",
"answer": 2,
"money": 640000
},
{
"name": "In which state is the main language Khasi?",
"option1": "Nagaland",
"option2": "Rajasthan",
"option3": "Maharashtra",
"option4": "Meghalaya",
"answer": 4,
"money": 1250000
},
{
"name": "Which of the following created history in 2016 by winning all three gold medals on offer in the 1st Western Asia Youth Chess Championship?",
"option1": "Nihal Sarin",
"option2": "Kush Bhagat",
"option3": "Praggnanandhaa",
"option4": "Vidit Gujrathi",
"answer": 2,
"money": 2500000
},
{
"name": " Which Bollywood actress is the new ambassador for Swachh Bharat Mission's youth-based 'Swachh Saathi' programme?",
"option1": "Vidya Balan ",
"option2": "Dia Mirza ",
"option3": "Priyanka Chopra",
"option4": "Sonakshi Sinha",
"answer": 2,
"money": 5000000
},
{
"name": "When is the Indian Air Force Day celebrated?",
"option1": "October 9",
"option2": "October 10",
"option3": "October 8",
"option4": "October 11",
"answer": 3,
"money": 10000000
}
]
Output :
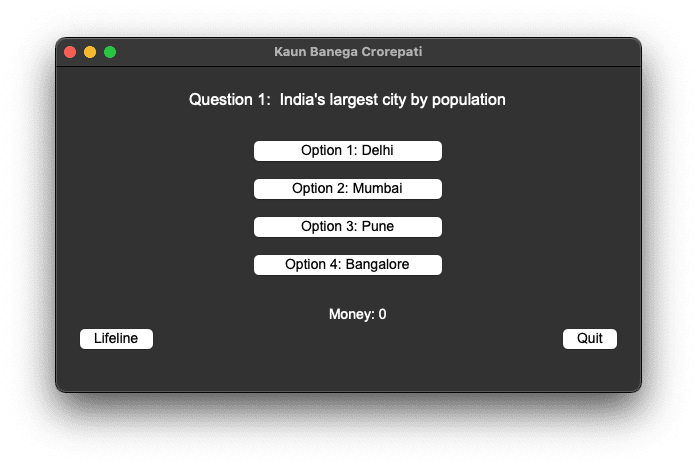
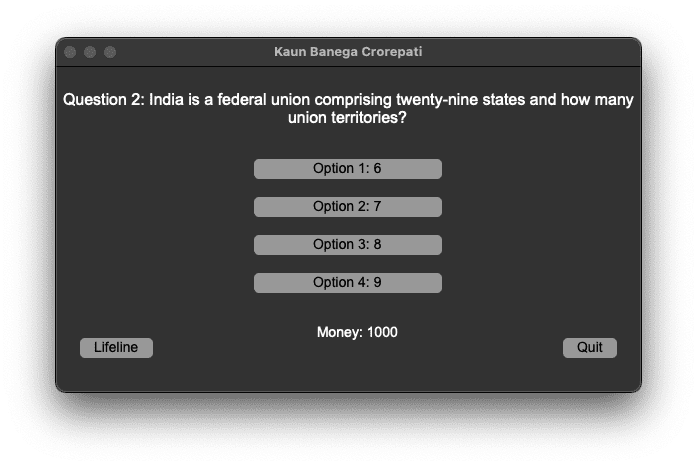
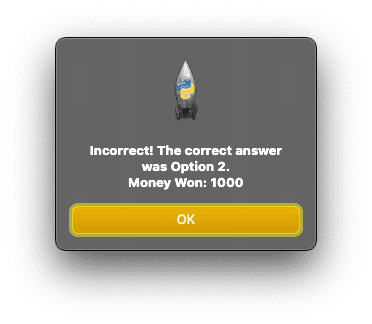
Find More Projects
Drawing Ganesha Using Python Turtle Graphics[Drawing Ganapati Using Python] Introduction In this blog post, we will learn how to draw Lord Ganesha …
Contact Management System in Python with a Graphical User Interface (GUI) Introduction: The Contact Management System is a Python-based application designed to …
KBC Game using Python with Source Code Introduction : Welcome to this blog post on building a “Kaun Banega Crorepati” (KBC) game …
Basic Logging System in C++ With Source Code Introduction : It is one of the most important practices in software development. Logging …
Snake Game Using C++ With Source Code Introduction : The Snake Game is one of the most well-known arcade games, having its …
C++ Hotel Management System With Source Code Introduction : It is very important to manage the hotels effectively to avail better customer …