Simon Says– Classic Memory Puzzle Game Using Python With Source Code
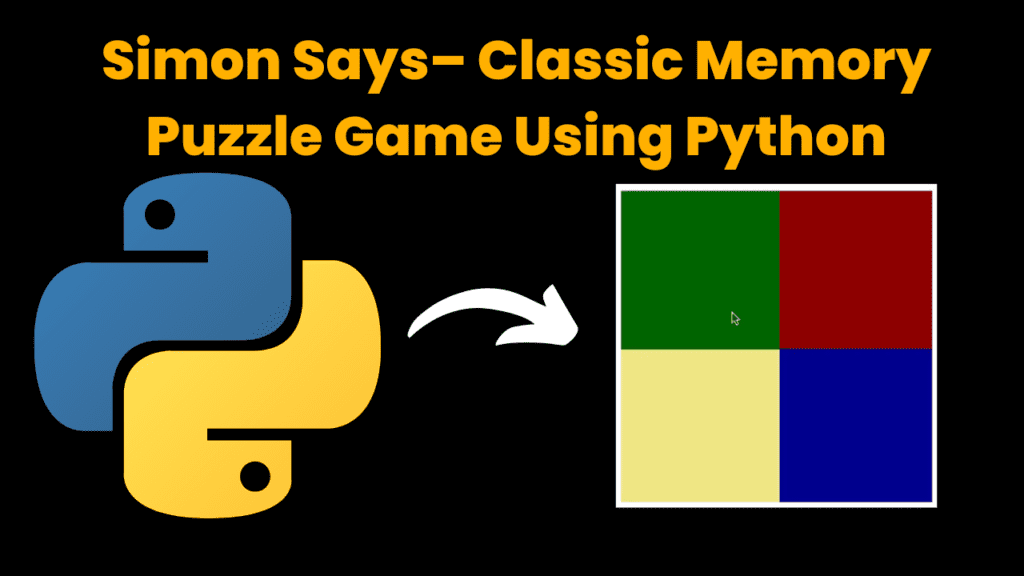
Introduction :
Simon Says is a classic memory puzzle game that challenges players to remember and reproduce sequences of flashing tiles. Each time the player correctly matches the sequence, it becomes longer, testing their memory and attention. In this tutorial, we will create a simple version of Simon Says using Python and the turtle graphics module. Simon Says is a fun and engaging memory game where the player must replicate a sequence of flashing tiles. The game starts with a short sequence and gradually increases in length as the player correctly follows the pattern. This project demonstrates how to build a Simon Says game using Python’s turtle graphics module.
Required Modules :-
To run this project, you need the turtle and freegames modules. If you don’t have free games installed, you can install it using
pip install freegames
How to Run the Code :-
1. Install Dependencies:
○ Ensure Python is installed.
○ Install the freegames module using pip.
2. Run the Code:
○ Save the provided code in a file named simon_says.py.
Run the script using Python
python simon_says.py
Play the Game:
○ Agraphical window will open displaying the Simon Says game.
○ Click anywhere on the screen to start the game.
○ Watchthe pattern as it flashes and then click the tiles in the same order to replicate the sequence.
Code Explanation :-
Let’s break down the code to understand how the Simon Says game is implemented:
1. Importing Modules
from random import choice
from time import sleep
from turtle import *
from freegames import floor, square, vector
● random.choice: Used to randomly select a tile for the pattern.
● time.sleep: Used to create delays between tile flashes.
● turtle: Used for graphical output.
● freegames: Provides utilities for drawing and managing game elements.
2. Initializing Variables
pattern = []
guesses = []
tiles = {
vector(0, 0): ('red', 'dark red'),
vector(0,-200): ('blue', 'dark blue'),
vector(-200, 0): ('green', 'dark green'),
vector(-200,-200): ('yellow', 'khaki'),
}
● pattern: Stores the sequence of tiles that the player must remember.
● guesses: Stores the player’s current guesses.
● tiles:Defines the colors for each tile position on the grid.
3. Drawing the Grid
def grid():
"""Draw grid of tiles."""
square(0, 0, 200, 'dark red')
square(0,-200, 200, 'dark blue')
square(-200, 0, 200, 'dark green')
square(-200,-200, 200, 'khaki')
update()
● grid(): Draws the four tiles of the game board. Each tile is colored differently and positioned in a grid format.
4. Flashing Tiles
def flash(tile):
"""Flash tile in grid."""
glow, dark = tiles[tile]
square(tile.x, tile.y, 200, glow)
update()
sleep(0.5)
square(tile.x, tile.y, 200, dark)
update()
sleep(0.5)
● flash(tile): Lights up the tile by changing its color to a brighter shade, then returns it to its original color. This simulates the tile flashing.
5. Growing the Pattern
def grow():
"""Grow pattern and flash tiles."""
tile = choice(list(tiles))
pattern.append(tile)
for tile in pattern:
flash(tile)
print('Pattern length:', len(pattern))
guesses.clear()
● grow(): Adds a new random tile to the pattern, flashes the entire sequence, and clears the player’s guesses. The pattern grows with each correct sequence
6. Handling Clicks
def tap(x, y):
"""Respond to screen tap."""
onscreenclick(None)
x = floor(x, 200)
y = floor(y, 200)
tile = vector(x, y)
index = len(guesses)
if tile != pattern[index]:
exit()
guesses.append(tile)
flash(tile)
if len(guesses) == len(pattern):
grow()
onscreenclick(tap)
● tap(x, y):Handles mouse clicks. Converts the click position into a tile, checks if it matches the current position in the pattern, and updates the game state. If the player completes the sequence, it grows the pattern.
7. Starting the Game
def start(x, y):
"""Start game."""
grow()
onscreenclick(tap)
● start(x, y):Starts the game by initializing the pattern and setting up the click handler for tile interactions
8. Setting Up the Game
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
grid()
onscreenclick(start)
done()
● setup(): Initializes the graphical window with a width and height of 420.
● hideturtle(): Hides the turtle cursor for a cleaner look.
● tracer(False): Disables automatic screen updates for better performance.
● grid(): Draws the game grid.
● onscreenclick(start): Sets up the start() function to handle the initial game start.
● done(): Completes the setup and starts the game loop.
Source Code :-
from random import choice
from time import sleep
from turtle import *
from freegames import floor, square, vector
pattern = []
guesses = []
tiles = {
vector(0, 0): ('red', 'dark red'),
vector(0, -200): ('blue', 'dark blue'),
vector(-200, 0): ('green', 'dark green'),
vector(-200, -200): ('yellow', 'khaki'),
}
def grid():
"""Draw grid of tiles."""
square(0, 0, 200, 'dark red')
square(0, -200, 200, 'dark blue')
square(-200, 0, 200, 'dark green')
square(-200, -200, 200, 'khaki')
update()
def flash(tile):
"""Flash tile in grid."""
glow, dark = tiles[tile]
square(tile.x, tile.y, 200, glow)
update()
sleep(0.5)
square(tile.x, tile.y, 200, dark)
update()
sleep(0.5)
def grow():
"""Grow pattern and flash tiles."""
tile = choice(list(tiles))
pattern.append(tile)
for tile in pattern:
flash(tile)
print('Pattern length:', len(pattern))
guesses.clear()
def tap(x, y):
"""Respond to screen tap."""
onscreenclick(None)
x = floor(x, 200)
y = floor(y, 200)
tile = vector(x, y)
index = len(guesses)
if tile != pattern[index]:
exit()
guesses.append(tile)
flash(tile)
if len(guesses) == len(pattern):
grow()
onscreenclick(tap)
def start(x, y):
"""Start game."""
grow()
onscreenclick(tap)
setup(420, 420, 370, 0)
hideturtle()
tracer(False)
grid()
onscreenclick(start)
done()
Output :
Running the code will open a graphical window displaying the Simon Says game. Click anywhere on the screen to start the game, then follow the sequence of flashing tiles by clicking on them in the correct order. The game will continue to grow more challenging as the pattern length increases.
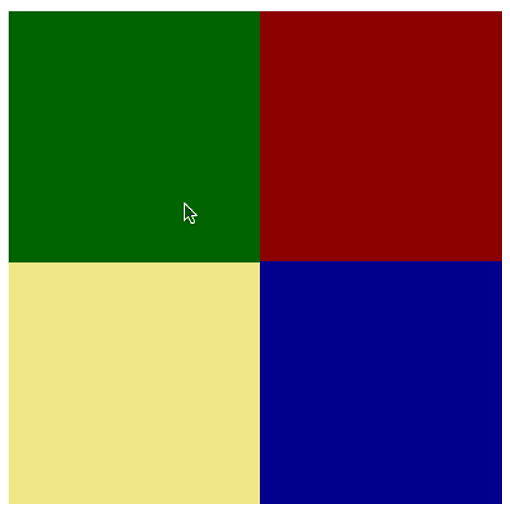
Find More Projects
Build a Quiz Game Using HTML CSS and JavaScript Introduction Hello coders, you might have played various games, but were you aware …
Emoji Catcher Game Using HTML CSS and JavaScript Introduction Hello Coders, Welcome to another new blog. In this article we’ve made a …
Typing Challenge Using HTML CSS and JavaScript Introduction Hello friends, all you developer friends are welcome to our new project. If you …
Breakout Game Using HTML CSS and JavaScript With Source Code Introduction Hello friends, welcome to today’s new blog post. All of you …
Digital and Analog Clock using HTML CSS and JavaScript Introduction : This project is a digital clock and stopwatch system, which allows …
Coffee Shop Website using HTML, CSS & JavaScript Introduction : This project is a website for coffee house business. It uses HTML …